Local Notifications in Expo
I recently created a Pomodoro app. Check it out! With the Pomodoro technique, you schedule out a 25 minute block to focus and then take a short break. My app needed a way to notify you when it was time to take a break. Eventually I settled on Local or Scheduled Notifications as the solution. However, the vast majority of information about implementing Notifications in a React Native app was about Push Notifications. So I decided to write this post! Push Notifications vs Local Notifications Push Notifications are sent to a user's device from a server. For example, you might get a notification on your phone when your favorite hip-hop artist posts a new micro-blog to X.com: The notification is sent to your phone from a server, not the Twitter application itself. This was an extra level of complication that my humble Pomodoro app did not need. I just need to notify the user 25 minutes after they press the "Start" button in the app. No server need be involved. This is where Local or Scheduled Notifications come in. They are much simpler and allow you to schedule a notification from within the app itself. Warning: Notifications don't work on emulators. You'll need a real device. Also, Push Notifications require a development build. That's not an issue for this tutorial though as we are only using Local Notifications. Set up I'm going to create a new expo app and run reset-project to remove the boilerplate: npx create-expo-app@latest NotificationsTest npm run reset-project Now install the expo-notifications package: npx expo install expo-notifications The next thing we need to do is request permission from the user to send notifications. This is something you need to do even if you're not sending Push Notifications and only Local Notifications. Shut up and show me code: index.tsx import { useEffect } from "react"; import { Text, View } from "react-native"; import * as Notifications from "expo-notifications"; export default function Index() { useEffect(() => { const configureNotificationsAsync = async () => { const response = await Notifications.requestPermissionsAsync(); console.log("
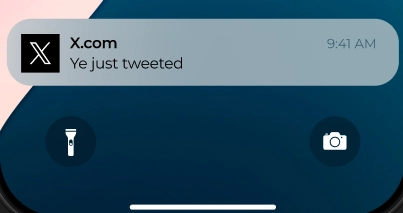
I recently created a Pomodoro app. Check it out!
With the Pomodoro technique, you schedule out a 25 minute block to focus and then take a short break.
My app needed a way to notify you when it was time to take a break.
Eventually I settled on Local or Scheduled Notifications as the solution. However, the vast majority of information about implementing Notifications in a React Native app was about Push Notifications. So I decided to write this post!
Push Notifications vs Local Notifications
Push Notifications are sent to a user's device from a server. For example, you might get a notification on your phone when your favorite hip-hop artist posts a new micro-blog to X.com:
The notification is sent to your phone from a server, not the Twitter application itself.
This was an extra level of complication that my humble Pomodoro app did not need. I just need to notify the user 25 minutes after they press the "Start" button in the app. No server need be involved.
This is where Local or Scheduled Notifications come in. They are much simpler and allow you to schedule a notification from within the app itself.
Warning: Notifications don't work on emulators. You'll need a real device. Also, Push Notifications require a development build. That's not an issue for this tutorial though as we are only using Local Notifications.
Set up
I'm going to create a new expo app and run reset-project to remove the boilerplate:
npx create-expo-app@latest NotificationsTest
npm run reset-project
Now install the expo-notifications package:
npx expo install expo-notifications
The next thing we need to do is request permission from the user to send notifications. This is something you need to do even if you're not sending Push Notifications and only Local Notifications.
Shut up and show me code:
index.tsx
import { useEffect } from "react";
import { Text, View } from "react-native";
import * as Notifications from "expo-notifications";
export default function Index() {
useEffect(() => {
const configureNotificationsAsync = async () => {
const response = await Notifications.requestPermissionsAsync();
console.log("