JavaScript Numbers
Understanding Numbers in JavaScript In JavaScript, numbers are a fundamental data type used for representing numerical values. Here are some key points about numbers in JavaScript: Types of Numbers JavaScript has only one type of number, which can be written with or without decimals. For example: let integer = 42; let float = 3.14; Arithmetic Operations You can perform various arithmetic operations like addition, subtraction, multiplication, and division: let sum = 10 + 5; // 15 let difference = 10 - 5; // 5 let product = 10 * 5; // 50 let quotient = 10 / 5; // 2 Special Numbers JavaScript also handles special numerical values like Infinity, -Infinity, and NaN (Not-a-Number): let positiveInfinity = 1 / 0; // Infinity let negativeInfinity = -1 / 0; // -Infinity let notANumber = "hello" / 2; // NaN Number Methods JavaScript provides several methods for working with numbers, such as toFixed(), toPrecision(), and toString(). Here are some common methods for numbers in JavaScript, along with their definitions, parameters, and examples: toFixed(): Formats a number using fixed-point notation. Parameters: digits (optional): The number of digits to appear after the decimal point. Default is 0. Example: let num = 123.456; console.log(num.toFixed(2)); // "123.46" toPrecision(): Formats a number to a specified length. Parameters: precision (optional): The number of significant digits. Default is the number of digits necessary to represent the number. Example: let num = 123.456; console.log(num.toPrecision(4)); // "123.5" toString(): Converts a number to a string. Parameters: radix (optional): An integer between 2 and 36 specifying the base to use for representing numeric values. Default is 10. Example: let num = 123; console.log(num.toString()); // "123" console.log(num.toString(2)); // "1111011" (binary representation) valueOf(): Returns the primitive value of a number object. Parameters: None. Example: let num = new Number(123); console.log(num.valueOf()); // 123 toExponential(): Converts a number to exponential notation. Parameters: fractionDigits (optional): The number of digits after the decimal point. Must be in the range 0 - 20, inclusive. Example: let num = 123456; console.log(num.toExponential(2)); // "1.23e+5" Math Object The Math object contains properties and methods for mathematical constants and functions: console.log(Math.PI); // 3.141592653589793 console.log(Math.sqrt(16)); // 4 console.log(Math.random()); // Random number between 0 and 1 Number Coercion Number coercion in JavaScript refers to the process of converting values from one type to a number. This can happen implicitly (automatically by JavaScript) or explicitly (using functions or operators). Here are some common ways number coercion occurs: Implicit Coercion: JavaScript automatically converts values to numbers in certain contexts, such as arithmetic operations. Examples: let result = "5" - 2; // 3 (string "5" is coerced to number 5) let result2 = "5" * 2; // 10 (string "5" is coerced to number 5) let result3 = "5" / 2; // 2.5 (string "5" is coerced to number 5) Explicit Coercion: You can explicitly convert values to numbers using various methods. Number(): Converts a value to a number. Example: let num = Number("123"); // 123 let num2 = Number(true); // 1 let num3 = Number(false); // 0 parseInt(): Parses a string and returns an integer. Parameters: string: The string to be parsed. radix (optional): An integer between 2 and 36 that represents the base of the numeral system. Example: let num = parseInt("123"); // 123 let num2 = parseInt("101", 2); // 5 (binary to decimal) parseFloat(): Parses a string and returns a floating-point number. Parameters: string: The string to be parsed. Example: let num = parseFloat("123.45"); // 123.45 Unary Plus (+): Converts a value to a number. Example: let num = +"123"; // 123 let num2 = +true; // 1 let num3 = +false; // 0 Common Coercion Pitfalls: Number("") returns 0. Number(null) returns 0. Number(undefined) returns NaN. Number("123abc") returns NaN. Additional Number Methods Here are some more useful methods for working with numbers: isNaN(): Determines whether a value is NaN (Not-a-Number). Parameters: value: The value to be tested. Example: console.log(isNaN("hello")); // true console.log(isNaN(123)); // false isFinite(): Determines whether a value is a finite number. Parameters: value: The value to be tested. Example: console.log(isFinite(123)); // true console.log(isFinite(Infinity)); // false console.log(isFinite("123")); // true (string "123" is coerced to number 123)
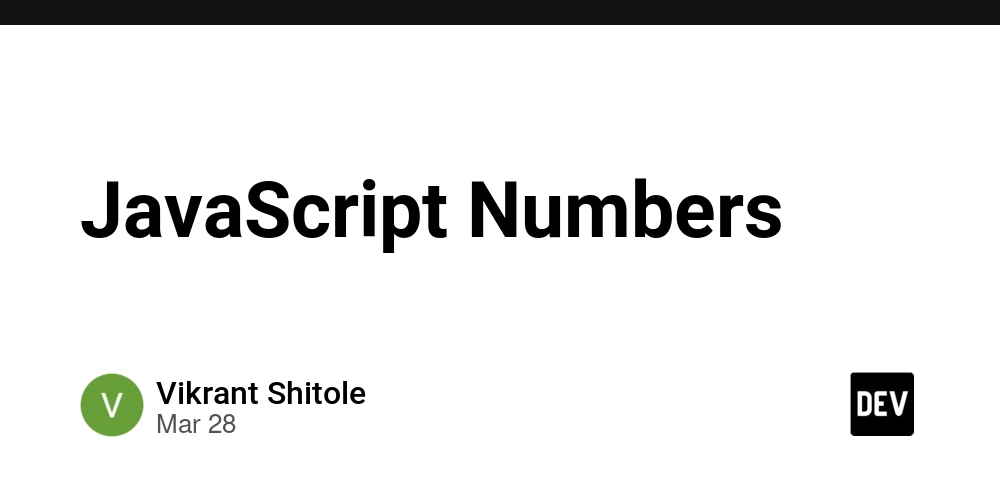
Understanding Numbers in JavaScript
In JavaScript, numbers are a fundamental data type used for representing numerical values. Here are some key points about numbers in JavaScript:
Types of Numbers
JavaScript has only one type of number, which can be written with or without decimals. For example:
let integer = 42;
let float = 3.14;
Arithmetic Operations
You can perform various arithmetic operations like addition, subtraction, multiplication, and division:
let sum = 10 + 5; // 15
let difference = 10 - 5; // 5
let product = 10 * 5; // 50
let quotient = 10 / 5; // 2
Special Numbers
JavaScript also handles special numerical values like Infinity
, -Infinity
, and NaN
(Not-a-Number):
let positiveInfinity = 1 / 0; // Infinity
let negativeInfinity = -1 / 0; // -Infinity
let notANumber = "hello" / 2; // NaN
Number Methods
JavaScript provides several methods for working with numbers, such as toFixed()
, toPrecision()
, and toString()
. Here are some common methods for numbers in JavaScript, along with their definitions, parameters, and examples:
-
toFixed()
: Formats a number using fixed-point notation. Parameters:-
digits
(optional): The number of digits to appear after the decimal point. Default is 0.
-
Example:
let num = 123.456;
console.log(num.toFixed(2)); // "123.46"
-
toPrecision()
: Formats a number to a specified length. Parameters:-
precision
(optional): The number of significant digits. Default is the number of digits necessary to represent the number.
-
Example:
let num = 123.456;
console.log(num.toPrecision(4)); // "123.5"
-
toString()
: Converts a number to a string. Parameters:-
radix
(optional): An integer between 2 and 36 specifying the base to use for representing numeric values. Default is 10.
-
Example:
let num = 123;
console.log(num.toString()); // "123"
console.log(num.toString(2)); // "1111011" (binary representation)
-
valueOf()
: Returns the primitive value of a number object. Parameters: None.
Example:
let num = new Number(123);
console.log(num.valueOf()); // 123
-
toExponential()
: Converts a number to exponential notation. Parameters:-
fractionDigits
(optional): The number of digits after the decimal point. Must be in the range 0 - 20, inclusive.
-
Example:
let num = 123456;
console.log(num.toExponential(2)); // "1.23e+5"
Math Object
The Math
object contains properties and methods for mathematical constants and functions:
console.log(Math.PI); // 3.141592653589793
console.log(Math.sqrt(16)); // 4
console.log(Math.random()); // Random number between 0 and 1
Number Coercion
Number coercion in JavaScript refers to the process of converting values from one type to a number. This can happen implicitly (automatically by JavaScript) or explicitly (using functions or operators). Here are some common ways number coercion occurs:
Implicit Coercion:
JavaScript automatically converts values to numbers in certain contexts, such as arithmetic operations.
Examples:
let result = "5" - 2; // 3 (string "5" is coerced to number 5)
let result2 = "5" * 2; // 10 (string "5" is coerced to number 5)
let result3 = "5" / 2; // 2.5 (string "5" is coerced to number 5)
Explicit Coercion:
You can explicitly convert values to numbers using various methods.
-
Number()
: Converts a value to a number. Example:
let num = Number("123"); // 123
let num2 = Number(true); // 1
let num3 = Number(false); // 0
-
parseInt()
: Parses a string and returns an integer. Parameters:-
string
: The string to be parsed. -
radix
(optional): An integer between 2 and 36 that represents the base of the numeral system.
-
Example:
let num = parseInt("123"); // 123
let num2 = parseInt("101", 2); // 5 (binary to decimal)
-
parseFloat()
: Parses a string and returns a floating-point number. Parameters:-
string
: The string to be parsed.
-
Example:
let num = parseFloat("123.45"); // 123.45
-
Unary Plus (
+
): Converts a value to a number. Example:
let num = +"123"; // 123
let num2 = +true; // 1
let num3 = +false; // 0
Common Coercion Pitfalls:
-
Number("")
returns0
. -
Number(null)
returns0
. -
Number(undefined)
returnsNaN
. -
Number("123abc")
returnsNaN
.
Additional Number Methods
Here are some more useful methods for working with numbers:
-
isNaN()
: Determines whether a value is NaN (Not-a-Number). Parameters:-
value
: The value to be tested.
-
Example:
console.log(isNaN("hello")); // true
console.log(isNaN(123)); // false
-
isFinite()
: Determines whether a value is a finite number. Parameters:-
value
: The value to be tested.
-
Example:
console.log(isFinite(123)); // true
console.log(isFinite(Infinity)); // false
console.log(isFinite("123")); // true (string "123" is coerced to number 123)
-
Number.isInteger()
: Determines whether a value is an integer. Parameters:-
value
: The value to be tested.
-
Example:
console.log(Number.isInteger(123)); // true
console.log(Number.isInteger(123.45)); // false
-
Number.isSafeInteger()
: Determines whether a value is a safe integer (an integer that can be exactly represented as an IEEE-754 double precision number). Parameters:-
value
: The value to be tested.
-
Example:
console.log(Number.isSafeInteger(123)); // true
console.log(Number.isSafeInteger(Math.pow(2, 53))); // false
-
Number.parseFloat()
: Parses a string argument and returns a floating-point number. Parameters:-
string
: The string to be parsed.
-
Example:
let num = Number.parseFloat("123.45"); // 123.45
-
Number.parseInt()
: Parses a string argument and returns an integer. Parameters:-
string
: The string to be parsed. -
radix
(optional): An integer between 2 and 36 that represents the base of the numeral system.
-
Example:
let num = Number.parseInt("123"); // 123
let num2 = Number.parseInt("101", 2); // 5 (binary to decimal)
-
Number.isNaN()
: Determines whether the passed value is NaN and its type is Number. Parameters:-
value
: The value to be tested.
-
Example:
console.log(Number.isNaN(NaN)); // true
console.log(Number.isNaN("hello")); // false
Understanding isNaN()
in JavaScript
The isNaN()
function in JavaScript is used to determine whether a value is NaN (Not-a-Number). Here's a detailed explanation:
Definition
isNaN()
is a global function that checks if a value is NaN. It returns true
if the value is NaN, and false
otherwise.
Syntax
isNaN(value)
Parameters
-
value
: The value to be tested.
How It Works
The isNaN()
function first tries to convert the value to a number. If the conversion fails and results in NaN, the function returns true
. Otherwise, it returns false
.
Examples
Here are some examples to illustrate how isNaN()
works:
Example 1: Checking a String
console.log(isNaN("hello")); // true
In this example, the string "hello" cannot be converted to a number, so isNaN()
returns true
.
Example 2: Checking a Number
console.log(isNaN(123)); // false
Here, the value 123 is already a number, so isNaN()
returns false
.
Example 3: Checking an Empty String
console.log(isNaN("")); // false
An empty string is coerced to 0, which is a number, so isNaN()
returns false
.
Example 4: Checking undefined
console.log(isNaN(undefined)); // true
undefined
cannot be converted to a number, so isNaN()
returns true
.
Example 5: Checking NaN
console.log(isNaN(NaN)); // true
Since the value is already NaN, isNaN()
returns true
.
Important Note
The isNaN()
function has some quirks because it first converts the value to a number before checking if it is NaN. This can lead to unexpected results. For example:
console.log(isNaN("123")); // false
The string "123" is coerced to the number 123, so isNaN()
returns false
.
Number.isNaN()
To avoid some of the quirks of isNaN()
, you can use Number.isNaN()
, which does not perform type coercion. It only returns true
if the value is of type Number
and is NaN.
Example:
console.log(Number.isNaN(NaN)); // true
console.log(Number.isNaN("hello")); // false
Summary
-
isNaN()
checks if a value is NaN after attempting to convert it to a number. - It returns
true
for values that cannot be converted to a number. - Use
Number.isNaN()
for a stricter check that avoids type coercion.