JavaScript Memory Management
JavaScript Memory Management JavaScript is a high-level programming language that automatically handles memory management, making it easier for developers to create applications without worrying about manual memory allocation and deallocation. JavaScript ek high-level programming language hai jo memory management ko automatically handle karti hai. Isse developers ko applications banate waqt manual memory allocation aur deallocation ki chinta nahi karni padti. Overview of Memory Management What is Memory Management? Memory management refers to the process of allocating (reserving space for) and deallocating (releasing space) memory as needed by a program. In JavaScript, this process is largely automated, allowing developers to focus on building features instead of managing memory manually. Memory management ka matlab hai program ke liye zaroori memory ko allocate (allocate karna ka matlab hai kisi cheez ke liye jagah banana) aur deallocate (deallocate karna ka matlab hai kisi cheez se jagah khali karna) karna. JavaScript mein yeh process largely automated hai, jo developers ko features banane par focus karne ki suvidha deta hai. Automatic vs. Manual Memory Management Manual Memory Management: In languages like C, developers must manually allocate memory using functions like malloc() (which allocates memory) and release it using free() (which frees memory). This can lead to issues like memory leaks (when memory is used but not released, causing the program to slow down) if memory is not managed correctly. C jaisi languages mein, developers ko khud memory allocate karni padti hai malloc() (yeh function memory allocate karne ke liye hota hai) jaise functions ka use karke, aur free() (yeh function memory ko release karne ke liye hota hai) se release karni padti hai. Ismein memory leaks (memory leaks ka matlab hai wo memory jo use nahi hoti lekin free nahi hoti, jo program ko slow kar sakti hai) hone ka risk hota hai agar memory ko sahi se manage nahi kiya. Automatic Memory Management: In JavaScript, memory allocation and deallocation are handled automatically. When you create variables or objects, JavaScript allocates memory for them. When they are no longer needed, the garbage collector reclaims that memory. JavaScript mein memory allocation aur deallocation automatically hoti hai. Jab aap variables (yeh data store karne ke liye containers hote hain) ya objects (yeh properties aur methods ka collection hote hain) create karte hain, JavaScript unke liye memory allocate karti hai. Jab unki zarurat nahi hoti, garbage collector un memory ko reclaim (reclaim ka matlab hai wapas lena) kar leta hai. ## What is a Variable? A variable is a named container used to store data. You can think of it as a label for a value, allowing you to reference that value later in your code. In JavaScript, you create variables using the keywords let, const, or var. The value of a variable can change, making it flexible for various programming tasks. What is a Reference? A reference is like an address or pointer that tells the computer where to find something in memory. When you create various data types in JavaScript—such as functions, arrays, strings, or objects—these data types are stored in RAM (Random Access Memory). RAM contains many different pieces of data, and when you create a variable, it doesn’t store the data itself. Instead, it stores a reference that points to the location in memory where that data is kept. This means that the variable contains the address of the data in RAM, allowing the computer to access and manipulate it efficiently. Ek reference ek tarah ka address ya pointer hota hai jo computer ko batata hai ki memory mein kuchh kahan hai. Jab aap JavaScript mein alag-alag data types create karte hain—jaise functions, arrays, strings, ya objects—ye data RAM (Random Access Memory) mein store hota hai. RAM mein bahut saari different pieces of data hoti hain, aur jab aap ek variable create karte hain, toh yeh data ko khud nahi store karta. Iske bajaye, yeh ek reference store karta hai jo memory mein us data ke location ko point karta hai. Iska matlab hai ki variable mein RAM mein data ka address hota hai, jo computer ko efficiently access aur manipulate karne ki ijazat deta hai. For example: let obj1 = { name: "Alice" }; let obj2 = obj1; // obj2 references the same object as obj1 obj2.name = "Bob"; // Changing obj2 will affect obj1 as well console.log(obj1.name); // Output: Bob How References Work in JavaScript Memory Basics: Jab aap ek variable create karte hain, JavaScript memory mein data store karne ke liye kuch space reserve karta hai. Is memory ka ek specific location hota hai, jaise ek ghar ka number. JavaScript actual data ko variable mein directly nahi rakhta. Iski jagah, yeh us memory space ka address save karta hai. Creating Data Types: Maan lijiye aap ek string create karte hain: let my
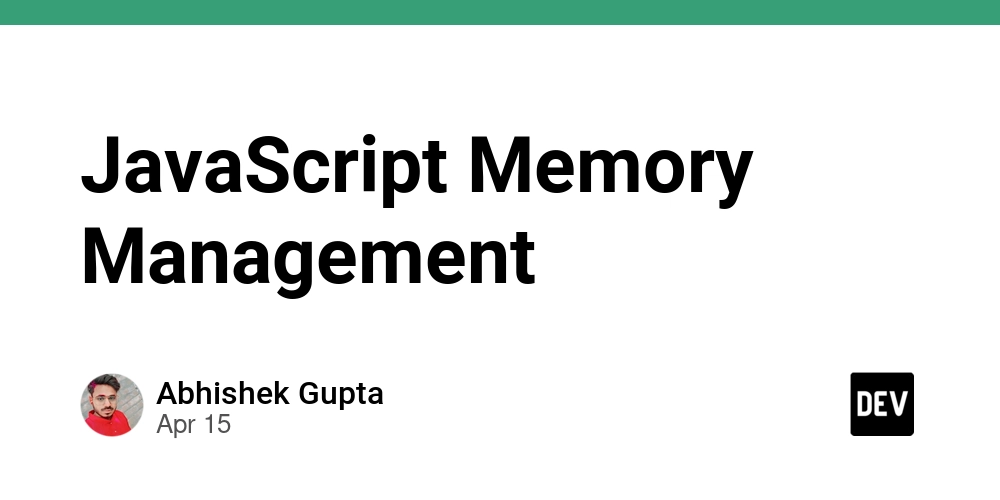
JavaScript Memory Management
JavaScript is a high-level programming language that automatically handles memory management, making it easier for developers to create applications without worrying about manual memory allocation and deallocation.
JavaScript ek high-level programming language hai jo memory management ko automatically handle karti hai. Isse developers ko applications banate waqt manual memory allocation aur deallocation ki chinta nahi karni padti.
Overview of Memory Management
What is Memory Management?
Memory management refers to the process of allocating (reserving space for) and deallocating (releasing space) memory as needed by a program. In JavaScript, this process is largely automated, allowing developers to focus on building features instead of managing memory manually.
Memory management ka matlab hai program ke liye zaroori memory ko allocate (allocate karna ka matlab hai kisi cheez ke liye jagah banana) aur deallocate (deallocate karna ka matlab hai kisi cheez se jagah khali karna) karna. JavaScript mein yeh process largely automated hai, jo developers ko features banane par focus karne ki suvidha deta hai.
Automatic vs. Manual Memory Management
Manual Memory Management:
In languages like C, developers must manually allocate memory using functions like
malloc()
(which allocates memory) and release it usingfree()
(which frees memory). This can lead to issues like memory leaks (when memory is used but not released, causing the program to slow down) if memory is not managed correctly.C jaisi languages mein, developers ko khud memory allocate karni padti hai
malloc()
(yeh function memory allocate karne ke liye hota hai) jaise functions ka use karke, aurfree()
(yeh function memory ko release karne ke liye hota hai) se release karni padti hai. Ismein memory leaks (memory leaks ka matlab hai wo memory jo use nahi hoti lekin free nahi hoti, jo program ko slow kar sakti hai) hone ka risk hota hai agar memory ko sahi se manage nahi kiya.
Automatic Memory Management:
In JavaScript, memory allocation and deallocation are handled automatically. When you create variables or objects, JavaScript allocates memory for them. When they are no longer needed, the garbage collector reclaims that memory.
JavaScript mein memory allocation aur deallocation automatically hoti hai. Jab aap variables (yeh data store karne ke liye containers hote hain) ya objects (yeh properties aur methods ka collection hote hain) create karte hain, JavaScript unke liye memory allocate karti hai. Jab unki zarurat nahi hoti, garbage collector un memory ko reclaim (reclaim ka matlab hai wapas lena) kar leta hai.
## What is a Variable?
A variable is a named container used to store data. You can think of it as a label for a value, allowing you to reference that value later in your code. In JavaScript, you create variables using the keywords let
, const
, or var
. The value of a variable can change, making it flexible for various programming tasks.
What is a Reference?
A reference is like an address or pointer that tells the computer where to find something in memory. When you create various data types in JavaScript—such as functions, arrays, strings, or objects—these data types are stored in RAM (Random Access Memory). RAM contains many different pieces of data, and when you create a variable, it doesn’t store the data itself. Instead, it stores a reference that points to the location in memory where that data is kept. This means that the variable contains the address of the data in RAM, allowing the computer to access and manipulate it efficiently.
Ek reference ek tarah ka address ya pointer hota hai jo computer ko batata hai ki memory mein kuchh kahan hai. Jab aap JavaScript mein alag-alag data types create karte hain—jaise functions, arrays, strings, ya objects—ye data RAM (Random Access Memory) mein store hota hai. RAM mein bahut saari different pieces of data hoti hain, aur jab aap ek variable create karte hain, toh yeh data ko khud nahi store karta. Iske bajaye, yeh ek reference store karta hai jo memory mein us data ke location ko point karta hai. Iska matlab hai ki variable mein RAM mein data ka address hota hai, jo computer ko efficiently access aur manipulate karne ki ijazat deta hai.
For example:
let obj1 = { name: "Alice" };
let obj2 = obj1; // obj2 references the same object as obj1
obj2.name = "Bob"; // Changing obj2 will affect obj1 as well
console.log(obj1.name); // Output: Bob
How References Work in JavaScript
Memory Basics:
Jab aap ek variable create karte hain, JavaScript memory mein data store karne ke liye kuch space reserve karta hai. Is memory ka ek specific location hota hai, jaise ek ghar ka number.
JavaScript actual data ko variable mein directly nahi rakhta. Iski jagah, yeh us memory space ka address save karta hai.
Creating Data Types:
- Maan lijiye aap ek string create karte hain:
let myString = "Hello, world!";
- Yahan, myString us memory location ka reference rakhta hai jahan string "Hello, world!" store hoti hai. Agar aap string ko ek letter ki tarah sochein, toh myString us letter ke mailbox ka address hai.
Working with Arrays:
- Ab agar aap ek array create karte hain:
let myArray = [1, 2, 3];
myArray us memory location ka reference rakhta hai jahan array [1, 2, 3] store hota hai. Agar aap myArray ki contents ko change karte hain, toh aap us memory address par data ko modify kar rahe hain, aur koi bhi dusra reference jo us address ko point karta hai, woh updated data dekhega.
Changing References
- Agar aap baad mein yeh karte hain:
myString = "New Value";
Is line se aap original string
"Hello, world!"
ko directly nahi badal rahe hain. Iski jagah, aapmyString
ko ek naye memory location par point karwa rahe hain jahan "New Value" store hai.Original string
"Hello, world!"
ab bhi memory mein hai, lekin agar koi aur variable ya reference is string ko point nahi karta, toh JavaScript ka garbage collector isse eventually clean up kar sakta hai.
Reference ka Kya Matlab Hai?
When we say that "there is still a reference," it means that some other variable is still pointing to this string.
If you have assigned "Hello, world!" to another variable, then this string will remain in memory because it still has a reference. However, if you have only set myString to "New Value" and no other variable is referencing it, then the garbage collector can remove "Hello, world!" from memory.
When you point a variable to a new value, the original value will only stay in memory as long as there is a reference to it.
If there are no other references, the garbage collector will free that memory, and "Hello, world!" will be deleted from memory.
Example: Creating a Variable and Storing a Value
let name = "Alice";
What Happens Here:
- Memory Allocation:
When you create the variable
name
, JavaScript allocates memory to store the string"Alice"
.This string is stored in memory, and the variable
name
holds a reference (or address) to that memory location.
- Storing the Value:
The variable
name
now points to the memory location where the string"Alice"
is stored.If you were to print
name
, it would show"Alice"
.
- Changing the Value:
- If you later change the value of name:
name = "Bob";
What happens now is that name is updated to point to a new memory location where the string "Bob" is stored.
The original string "Alice" is still in memory, but if no other variables point to it, it may eventually be cleaned up by the garbage collector.
Visual Representation:
- Before Changing:
Memory
------------------
| "Alice" | <-- Memory Location (e.g., 0x1A2B3C)
------------------
name -> 0x1A2B3C (points to "Alice")
```
`
- **After Changing::**
`
```markdown
Memory
------------------ ------------------
| "Alice" | | "Bob" | <-- New Memory Location (e.g., 0x4D5E6F)
------------------ ------------------
name -> 0x4D5E6F (now points to "Bob")
```
`
## The Garbage Collector
he garbage collector is a built-in feature of JavaScript that runs in the background. It identifies (recognizes) objects that are no longer in use (i.e., objects that cannot be reached from the root of the reference graph) and automatically frees the associated memory.
Garbage collector JavaScript ka ek built-in feature hai jo background mein chalta hai. Yeh un objects ko identify (identify ka matlab hai pehchanna) karta hai jo ab use nahi ho rahe hain (i.e., objects jo reference graph ke root se nahi milte) aur unse associated memory ko automatically free kar deta hai.
### How Garbage Collection Works
1. **Mark and Sweep**:
- The garbage collector marks all reachable (accessible) objects and then sweeps away (removes) the unmarked objects, reclaiming their memory.
- Garbage collector sab reachable (reachable ka matlab hai un objects tak pahunch sakna) objects ko mark karta hai aur phir unmarked objects ko sweep (sweep ka matlab hai hata dena) karta hai, unki memory reclaim karta hai.
2. **Reference Counting**:
- Another method counts how many references (pointers to an object) exist for an object. When the count drops to zero, the object can be collected (taken back).
- Ek aur method hai jo count karta hai ki ek object ke liye kitne references (references ka matlab hai un cheezon ka istemal jo kisi object ko point karte hain) hain. Jab count zero ho jata hai, tab object ko collect (collect ka matlab hai lena) kiya ja sakta hai.
## Real-World Examples
### 1. Creating an Object
```javascript
let car = {
make: "Toyota",
model: "Corolla"
};
// Here, we create an object named `car` with two properties: `make` and `model`.
// Memory is allocated for this object in the system.
// When the `car` variable goes out of scope (for example, if the function it’s in finishes executing),
// the garbage collector will eventually free that memory if there are no other references to it.
```
### 2. Using Arrays
```javascript
let numbers = [1, 2, 3, 4, 5];
// We create an array called `numbers` that holds a list of integers.
// The `numbers` array occupies memory to store its elements.
// If we later set `numbers` to null or redefine it, we remove our reference to the array.
// This allows the garbage collector to clean up the memory used by the original array,
// preventing memory leaks and making the memory available for other uses.
numbers = null; // This dereferences the array, allowing for garbage collection.
```
### 3. Event Listeners
```javascript
function setup() {
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
console.log("Button clicked!");
});
}
// In this example, we set up a button with an event listener.
// The listener waits for a click event and logs a message to the console.
// If the `setup` function is called multiple times without removing the old listener,
// it can create multiple listeners for the same button, leading to unexpected behavior.
// This situation can lead to a memory leak if not handled correctly, as the old references persist.
// It’s important to remove the event listener when it’s no longer needed.
button.removeEventListener("click", function() {
console.log("Button clicked!");
}); // This line attempts to clean up the listener, but will not work because the function is not the same reference.
```