Building a Text-to-Image NSFW AI Image Generator with Next.js and Sentry
Building a Text-to-Image NSFW AI Image Generator with Next.js and Sentry Introduction In recent years, AI-powered text-to-image generators have gained significant traction, enabling developers to create applications that convert textual descriptions into visual representations. One interesting application is generating NSFW (Not Safe For Work) content, which, when used responsibly, can serve various purposes like artistic expression or content moderation testing. In this tutorial, we'll explore how to build a text-to-image NSFW AI image generator using Next.js, integrate it with Sentry for error tracking, and leverage Webpack for module bundling. We will also discuss ethical considerations and best practices to ensure that our application runs smoothly in a production environment. Technologies Used Before diving in, let's overview the technologies we'll be using: Next.js: A React framework that enables server-side rendering and static site generation, offering excellent performance and SEO benefits. React: A JavaScript library for building user interfaces. Webpack: A static module bundler for modern JavaScript applications. Sentry: An error tracking and monitoring tool that helps developers identify and fix issues in real time. These technologies were identified by analyzing the page source code of Spark AI's Text-to-Image NSFW Generator, which utilizes Next.js and integrates Sentry for error tracking. Setting Up the Project First, ensure you have Node.js and npm installed on your machine. Then, create a new Next.js project: npx create-next-app text-to-image-nsfw-generator cd text-to-image-nsfw-generator Installing Dependencies We'll need additional packages for our application. Install the required dependencies: npm install react react-dom next npm install @sentry/nextjs Then, set up Sentry for your Next.js project by running: npx @sentry/wizard -i nextjs Follow the prompts to complete the Sentry configuration. Project Structure Your project structure should look similar to this: text-to-image-nsfw-generator/ ├── pages/ │ ├── _app.js │ ├── index.js │ └── api/ │ └── generate-image.js ├── public/ ├── styles/ ├── .sentryclirc ├── next.config.js └── ... Building the Text-to-Image Feature Integrating an AI API To generate images from text, we'll need an AI service. For this tutorial, we'll assume the existence of an API endpoint /api/generate-image that accepts a text prompt and returns an image URL. In a real-world scenario, this endpoint can proxy requests to any third-party image generation service. Creating the Input Form In pages/index.js, create a form where users can input their text prompts. Below is the complete example code: import { useState } from 'react'; export default function Home() { const [prompt, setPrompt] = useState(''); const [imageUrl, setImageUrl] = useState(null); const [loading, setLoading] = useState(false); const handleSubmit = async (e) => { e.preventDefault(); setLoading(true); try { const res = await fetch('/api/generate-image', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ prompt }), }); const data = await res.json(); setImageUrl(data.imageUrl); } catch (error) { console.error('Error generating image:', error); } finally { setLoading(false); } }; return ( NSFW Text-to-Image Generator setPrompt(e.target.value)} style={{ width: '300px', padding: '10px' }} /> {loading ? 'Generating...' : 'Generate Image'} {imageUrl && ( )} ); } Implementing the API Endpoint Create the API endpoint at pages/api/generate-image.js to handle the image generation request. In a production system, you would call your AI service here. For demonstration purposes, we return a placeholder image URL. export default async function handler(req, res) { if (req.method !== 'POST') { return res.status(405).json({ error: 'Method not allowed' }); } const { prompt } = req.body; if (!prompt) { return res.status(400).json({ error: 'Prompt is required' }); } try { // Integrate your AI image generation API here. // For demonstration, we'll use a placeholder image URL. const generatedImageUrl = 'https://via.placeholder.com/600x400.png?text=NSFW+Image'; res.status(200).json({ imageUrl: generatedImageUrl }); } catch (error) { // Capture error with Sentry if needed console.error('Error generating image:', error); res.status(500).json({ error: 'Internal server error' }); } } Handling NSFW Content and Ethical Considerations When building and deploying an NSFW image generator, ethical and legal aspects must be car
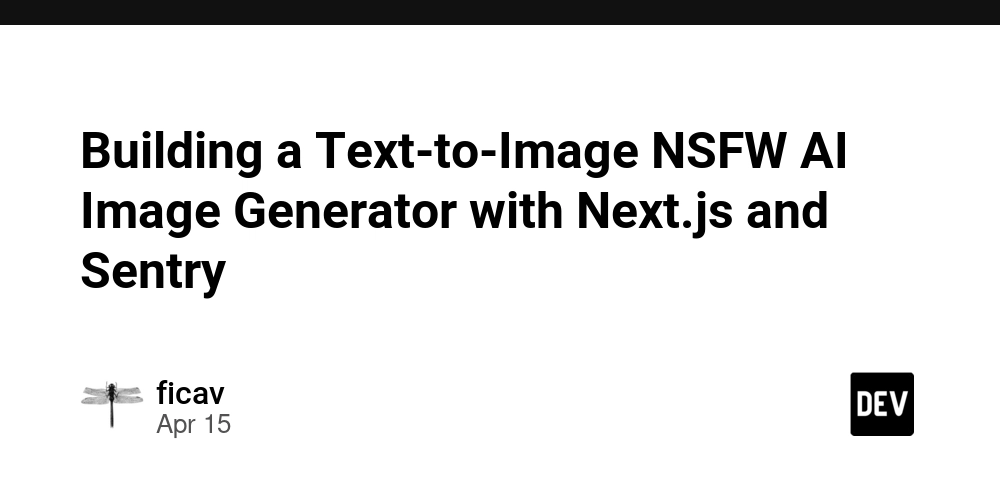
Building a Text-to-Image NSFW AI Image Generator with Next.js and Sentry
Introduction
In recent years, AI-powered text-to-image generators have gained significant traction, enabling developers to create applications that convert textual descriptions into visual representations. One interesting application is generating NSFW (Not Safe For Work) content, which, when used responsibly, can serve various purposes like artistic expression or content moderation testing.
In this tutorial, we'll explore how to build a text-to-image NSFW AI image generator using Next.js, integrate it with Sentry for error tracking, and leverage Webpack for module bundling. We will also discuss ethical considerations and best practices to ensure that our application runs smoothly in a production environment.
Technologies Used
Before diving in, let's overview the technologies we'll be using:
- Next.js: A React framework that enables server-side rendering and static site generation, offering excellent performance and SEO benefits.
- React: A JavaScript library for building user interfaces.
- Webpack: A static module bundler for modern JavaScript applications.
- Sentry: An error tracking and monitoring tool that helps developers identify and fix issues in real time.
These technologies were identified by analyzing the page source code of Spark AI's Text-to-Image NSFW Generator, which utilizes Next.js and integrates Sentry for error tracking.
Setting Up the Project
First, ensure you have Node.js and npm installed on your machine. Then, create a new Next.js project:
npx create-next-app text-to-image-nsfw-generator
cd text-to-image-nsfw-generator
Installing Dependencies
We'll need additional packages for our application. Install the required dependencies:
npm install react react-dom next
npm install @sentry/nextjs
Then, set up Sentry for your Next.js project by running:
npx @sentry/wizard -i nextjs
Follow the prompts to complete the Sentry configuration.
Project Structure
Your project structure should look similar to this:
text-to-image-nsfw-generator/
├── pages/
│ ├── _app.js
│ ├── index.js
│ └── api/
│ └── generate-image.js
├── public/
├── styles/
├── .sentryclirc
├── next.config.js
└── ...
Building the Text-to-Image Feature
Integrating an AI API
To generate images from text, we'll need an AI service. For this tutorial, we'll assume the existence of an API endpoint /api/generate-image
that accepts a text prompt and returns an image URL. In a real-world scenario, this endpoint can proxy requests to any third-party image generation service.
Creating the Input Form
In pages/index.js
, create a form where users can input their text prompts. Below is the complete example code:
import { useState } from 'react';
export default function Home() {
const [prompt, setPrompt] = useState('');
const [imageUrl, setImageUrl] = useState(null);
const [loading, setLoading] = useState(false);
const handleSubmit = async (e) => {
e.preventDefault();
setLoading(true);
try {
const res = await fetch('/api/generate-image', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ prompt }),
});
const data = await res.json();
setImageUrl(data.imageUrl);
} catch (error) {
console.error('Error generating image:', error);
} finally {
setLoading(false);
}
};
return (
<div style={{ textAlign: 'center', marginTop: '50px' }}>
<h1>NSFW Text-to-Image Generatorh1>
<form onSubmit={handleSubmit}>
<input
type="text"
placeholder="Enter your prompt..."
value={prompt}
onChange={(e) => setPrompt(e.target.value)}
style={{ width: '300px', padding: '10px' }}
/>
<button type="submit" style={{ marginLeft: '10px', padding: '10px 20px' }}>
{loading ? 'Generating...' : 'Generate Image'}
button>
form>
{imageUrl && (
<div style={{ marginTop: '20px' }}>
<img src={imageUrl} alt="Generated NSFW" style={{ maxWidth: '100%' }} />
div>
)}
div>
);
}
Implementing the API Endpoint
Create the API endpoint at pages/api/generate-image.js
to handle the image generation request. In a production system, you would call your AI service here. For demonstration purposes, we return a placeholder image URL.
export default async function handler(req, res) {
if (req.method !== 'POST') {
return res.status(405).json({ error: 'Method not allowed' });
}
const { prompt } = req.body;
if (!prompt) {
return res.status(400).json({ error: 'Prompt is required' });
}
try {
// Integrate your AI image generation API here.
// For demonstration, we'll use a placeholder image URL.
const generatedImageUrl = 'https://via.placeholder.com/600x400.png?text=NSFW+Image';
res.status(200).json({ imageUrl: generatedImageUrl });
} catch (error) {
// Capture error with Sentry if needed
console.error('Error generating image:', error);
res.status(500).json({ error: 'Internal server error' });
}
}
Handling NSFW Content and Ethical Considerations
When building and deploying an NSFW image generator, ethical and legal aspects must be carefully considered:
Content Filtering:
Implement mechanisms to filter out or flag content that may be harmful or exploitative. This might include integrating additional moderation APIs or manually reviewing generated content.Watermarking:
Consider adding unobtrusive watermarks to generated images to indicate that the content was created by an AI, which may help prevent misuse or misattribution.User Guidelines:
Establish clear usage policies and ensure that users are aware of acceptable use. This helps mitigate potential abuse of the NSFW generator.Legal Compliance:
Ensure that your application complies with local laws and regulations concerning adult or NSFW content.
Integrating Error Tracking with Sentry
Sentry helps you track and diagnose production issues. After running the Sentry wizard, your project will have configuration files (e.g., sentry.client.config.js
and sentry.server.config.js
) and adjustments in your _app.js
file.
A simple _app.js
might look like:
import * as Sentry from '@sentry/nextjs';
import '../styles/globals.css';
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default MyApp;
Sentry initialization and configuration should have been automatically set up by the wizard. Ensure you review your Sentry configuration files to adjust any settings such as release tracking or environment labels.
Customizing Webpack with Next.js
Next.js uses Webpack under the hood to bundle your application. If you need custom configuration (such as handling additional file types or optimizing performance), update your next.config.js
:
module.exports = {
webpack: (config, { isServer }) => {
// Example: add custom plugins or modify loaders as needed
// config.plugins.push(new YourCustomPlugin());
return config;
},
};
While this tutorial doesn’t include advanced modifications, understanding how to tweak Webpack can be beneficial for performance tuning and integrating unique assets.
Deployment and Best Practices
Deployment Platform:
Deploy your Next.js application using platforms like Vercel, which offer seamless integration, or choose other cloud providers such as AWS, Google Cloud, or DigitalOcean.Environment Variables:
Ensure that sensitive keys, such as your Sentry DSN and any API keys for AI services, are stored securely using environment variables.Monitoring and Logging:
Utilize Sentry and other logging mechanisms to monitor your app’s performance and promptly address errors in production.Scaling Considerations:
If your service gains traction, consider optimizing serverless functions, using caching strategies, and load balancing to handle high traffic.
Conclusion
In this tutorial, we walked through building a text-to-image NSFW AI image generator with Next.js and Sentry. We covered:
- Setting up a Next.js project and integrating essential technologies.
- Creating a user-friendly interface to submit text prompts.
- Implementing an API endpoint to handle the image generation process.
- Integrating Sentry for error monitoring and logging.
- Addressing NSFW content responsibly by discussing ethical considerations.
- Customizing Webpack for potential production optimizations.
- Deployment tips and best practices to ensure smooth operation in production.
This project serves as a foundation that you can extend with a real AI-powered image generation service, tighter content moderation systems, and additional features tailored to your needs. Always remember to use NSFW content generators responsibly and ethically.
Happy coding!