JAVA - Versions & Structure
Java has gone through several versions since its initial release in 1995. Below is a detailed breakdown of the major Java versions: 1. Early Versions (Java 1.x) These were the foundational versions of Java, introducing key concepts like the JVM (Java Virtual Machine), applets, and garbage collection. Java 1.0 (1996) – First stable version; introduced AWT, applets, and basic Java libraries. Java 1.1 (1997) – Introduced JDBC (Java Database Connectivity), inner classes, and RMI (Remote Method Invocation). 2. Java 2 (J2SE) Era (1998–2006) Sun Microsystems rebranded Java as "Java 2" and introduced Standard, Enterprise, and Micro editions. Java 1.2 (1998, J2SE 1.2) – Swing GUI toolkit, Java Collections Framework, JIT (Just-In-Time) compiler. Java 1.3 (2000, J2SE 1.3) – HotSpot JVM, Java Sound API. Java 1.4 (2002, J2SE 1.4) – Exception chaining, regex API, assert keyword, SSL. Java 5 (2004, J2SE 5.0) – Major update; introduced generics, enhanced for-loop, varargs, enums, autoboxing/unboxing. 3. Modern Java Era (Java SE) Sun Microsystems was acquired by Oracle in 2010, leading to a new release model. Java 6 (2006) – Performance improvements, scripting (JSR-223), JDBC 4.0. Java 7 (2011) – Try-with-resources, diamond operator (), switch with Strings. Java 8 (2014) – Major update; introduced Lambda expressions, Streams API, Optional, new Date-Time API. 4. Rapid Release Cycle (2017-Present) Oracle adopted a 6-month release cycle for Java SE versions. Java 9 (2017) – Project Jigsaw (Module System), JShell, HTTP/2 client. Java 10 (2018) – var keyword for local type inference. Java 11 (2018, LTS) – Removed JavaFX, introduced HttpClient API. Java 12 (2019) – Switch expressions (preview). Java 13 (2019) – Text blocks (preview). Java 14 (2020) – Records (preview), Pattern matching for instanceof. Java 15 (2020) – Sealed classes (preview). Java 16 (2021) – Pattern Matching for instanceof (final). Java 17 (2021, LTS) – Finalized sealed classes, switch expressions. Java 18 (2022) – UTF-8 as default encoding. Java 19 (2022) – Virtual threads (preview). Java 20 (2023) – Scoped values, structured concurrency. Java 21 (2023, LTS) – Virtual threads, Sequenced collections, String templates. Java 8 & Java 11 are widely used for enterprise applications. LTS (Long-Term Support) versions: Java 8, 11, 17, and 21. Latest version: Java 21 (as of 2024). Oracle provides updates for LTS versions; non-LTS versions have shorter support windows. Java Program Structure Component Description 1. Package Defines the package the class belongs to (optional). 2. Import Imports external Java libraries or classes (optional). 3. Class A Java program is defined inside a class. 4. Main Method The entry point of a Java application (public static void main(String[] args)). 5. Variables Used to store data (e.g., String name). 6. Object Creation Java uses objects to interact with classes (e.g., Scanner scanner = new Scanner(System.in);). 7. User Input Reads input from the console (scanner.nextLine()). 8. Method Call Calls a method to perform a task (greetUser(name)). 9. Resource Cleanup Closing resources like scanners to free memory. 10. Methods A function inside a class that performs specific actions (greetUser(String userName)).
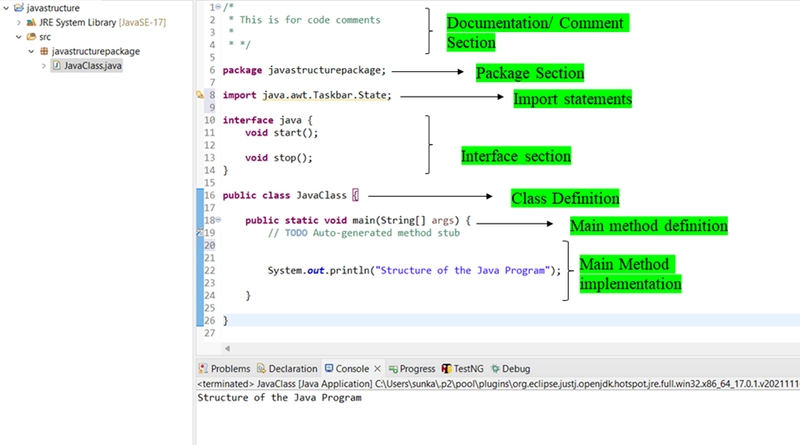
Java has gone through several versions since its initial release in 1995. Below is a detailed breakdown of the major Java versions:
1. Early Versions (Java 1.x)
These were the foundational versions of Java, introducing key concepts like the JVM (Java Virtual Machine), applets, and garbage collection.
- Java 1.0 (1996) – First stable version; introduced AWT, applets, and basic Java libraries.
- Java 1.1 (1997) – Introduced JDBC (Java Database Connectivity), inner classes, and RMI (Remote Method Invocation).
2. Java 2 (J2SE) Era (1998–2006)
Sun Microsystems rebranded Java as "Java 2" and introduced Standard, Enterprise, and Micro editions.
- Java 1.2 (1998, J2SE 1.2) – Swing GUI toolkit, Java Collections Framework, JIT (Just-In-Time) compiler.
- Java 1.3 (2000, J2SE 1.3) – HotSpot JVM, Java Sound API.
- Java 1.4 (2002, J2SE 1.4) – Exception chaining, regex API, assert keyword, SSL.
- Java 5 (2004, J2SE 5.0) – Major update; introduced generics, enhanced for-loop, varargs, enums, autoboxing/unboxing.
3. Modern Java Era (Java SE)
Sun Microsystems was acquired by Oracle in 2010, leading to a new release model.
- Java 6 (2006) – Performance improvements, scripting (JSR-223), JDBC 4.0.
-
Java 7 (2011) – Try-with-resources, diamond operator (
<>
), switch with Strings. -
Java 8 (2014) – Major update; introduced Lambda expressions, Streams API,
Optional
, new Date-Time API.
4. Rapid Release Cycle (2017-Present)
Oracle adopted a 6-month release cycle for Java SE versions.
- Java 9 (2017) – Project Jigsaw (Module System), JShell, HTTP/2 client.
-
Java 10 (2018) –
var
keyword for local type inference. -
Java 11 (2018, LTS) – Removed JavaFX, introduced
HttpClient
API. - Java 12 (2019) – Switch expressions (preview).
- Java 13 (2019) – Text blocks (preview).
-
Java 14 (2020) – Records (preview), Pattern matching for
instanceof
. - Java 15 (2020) – Sealed classes (preview).
-
Java 16 (2021) – Pattern Matching for
instanceof
(final). -
Java 17 (2021, LTS) – Finalized
sealed classes
,switch
expressions. - Java 18 (2022) – UTF-8 as default encoding.
- Java 19 (2022) – Virtual threads (preview).
- Java 20 (2023) – Scoped values, structured concurrency.
- Java 21 (2023, LTS) – Virtual threads, Sequenced collections, String templates.
- Java 8 & Java 11 are widely used for enterprise applications.
- LTS (Long-Term Support) versions: Java 8, 11, 17, and 21.
- Latest version: Java 21 (as of 2024).
- Oracle provides updates for LTS versions; non-LTS versions have shorter support windows.
Java Program Structure
Component | Description |
---|---|
1. Package | Defines the package the class belongs to (optional). |
2. Import | Imports external Java libraries or classes (optional). |
3. Class | A Java program is defined inside a class. |
4. Main Method | The entry point of a Java application (public static void main(String[] args) ). |
5. Variables | Used to store data (e.g., String name ). |
6. Object Creation | Java uses objects to interact with classes (e.g., Scanner scanner = new Scanner(System.in); ). |
7. User Input | Reads input from the console (scanner.nextLine() ). |
8. Method Call | Calls a method to perform a task (greetUser(name) ). |
9. Resource Cleanup | Closing resources like scanners to free memory. |
10. Methods | A function inside a class that performs specific actions (greetUser(String userName) ). |