Java Program to Check If Parentheses Are Properly Nested: A Comprehensive Guide
In Java programming, handling strings and verifying their structure is a common task. One problem that frequently arises is ensuring that parentheses in a string are properly nested. Proper nesting means that every opening parenthesis has a corresponding closing parenthesis and that they are correctly ordered. This kind of validation is important in various applications, such as evaluating mathematical expressions, parsing code, and analyzing data. In this blog, we will explore how to solve the problem of checking whether a given string of parentheses (of a single type) is properly nested. We will dive into the concept, the logic behind it, and how Java can be used to implement a solution. What Does "Properly Nested" Mean? A string of parentheses is considered properly nested if the following conditions are met: Every opening parenthesis ( must have a corresponding closing parenthesis ): For each opening parenthesis, there must be a closing parenthesis somewhere later in the string. The parentheses must close in the correct order: The parentheses must close in the reverse order of how they opened. For instance, an opening parenthesis at the beginning should be closed last, and any nested parentheses should also close in the correct sequence. For example: (): Properly nested (()): Properly nested (()()): Properly nested ()): Not properly nested ((): Not properly nested Why is Checking Parentheses Nesting Important? Validating parentheses and similar structures is a fundamental task in many applications, including: Mathematical Expressions: In mathematical or logical expressions, parentheses are often used to indicate the order of operations. Ensuring that these are properly nested is crucial for accurate calculations. Code Parsing: Programming languages often use parentheses for various purposes (such as function calls or control structures). Ensuring that parentheses are correctly nested is essential for a parser to understand and execute the code correctly. Expression Evaluation: In applications like calculators or evaluators, checking the proper nesting of parentheses is key to correct expression evaluation. Data Validation: In scenarios involving user input or data exchange, ensuring the integrity of the input by validating the structure of parentheses can prevent errors and potential security issues. How to Check if Parentheses Are Properly Nested? To solve the problem of checking if parentheses are properly nested in a string, we need to ensure two things: Balance of Parentheses: Every opening parenthesis must have a corresponding closing parenthesis. If at any point, there are more closing parentheses than opening ones, the string is immediately deemed improperly nested. Order of Parentheses: Parentheses must close in the reverse order they opened. For instance, a ( should be paired with a ), and they must be in the correct order, meaning a closing parenthesis should not appear before its corresponding opening parenthesis. Approach to Solve the Problem The most effective way to solve the problem of checking if parentheses are properly nested is through the use of a stack data structure. A stack operates on the principle of "last in, first out" (LIFO), making it ideal for this kind of problem. Here’s the general approach: Iterate through the String: For each character in the string, perform the following: If the character is an opening parenthesis (, push it onto the stack. If the character is a closing parenthesis ), check the stack: If the stack is empty, it means there is no corresponding opening parenthesis for this closing parenthesis, indicating an error. If the stack is not empty, pop the top of the stack, which corresponds to the matched opening parenthesis. Final Check: After processing all the characters in the string, check if the stack is empty. If the stack contains any unmatched opening parentheses, then the string is improperly nested. If the stack is empty, it means all opening parentheses had matching closing parentheses, and the string is properly nested. Handling Edge Cases When implementing this logic in Java, there are several edge cases to consider: Empty String: An empty string should be considered properly nested because there are no parentheses to match. Unmatched Parentheses: If there are unmatched parentheses at any point, such as an extra closing parenthesis or missing closing parenthesis, the string is not properly nested. Non-parenthesis Characters: Although the problem is limited to parentheses, in some applications, non-parenthesis characters may be present in the string. These characters should be ignored during the validation process. Why Use a Stack for This Problem? The stack data structure is particularly useful for problems involving matching pairs of symbols, such as parentheses. The reason is simple: a stack allows us to process characters in a way that mirro
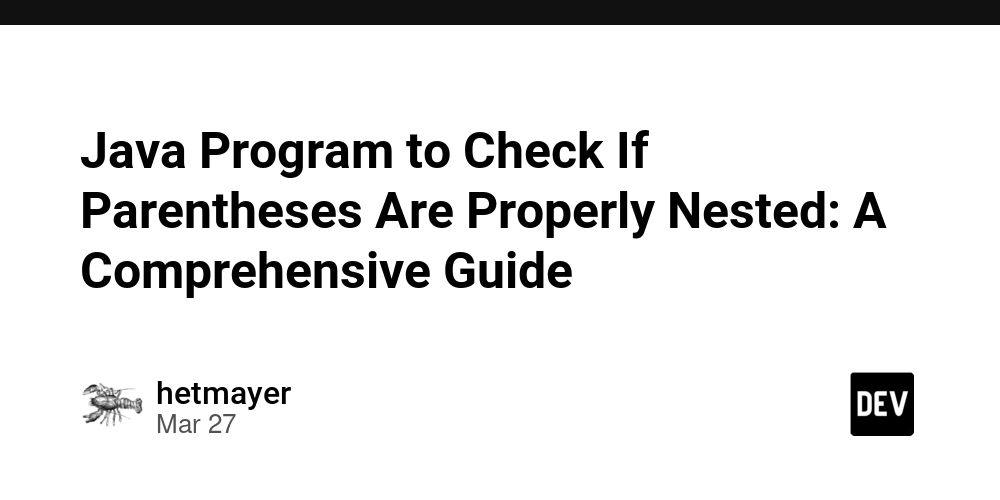
In Java programming, handling strings and verifying their structure is a common task. One problem that frequently arises is ensuring that parentheses in a string are properly nested. Proper nesting means that every opening parenthesis has a corresponding closing parenthesis and that they are correctly ordered. This kind of validation is important in various applications, such as evaluating mathematical expressions, parsing code, and analyzing data.
In this blog, we will explore how to solve the problem of checking whether a given string of parentheses (of a single type) is properly nested. We will dive into the concept, the logic behind it, and how Java can be used to implement a solution.
What Does "Properly Nested" Mean?
A string of parentheses is considered properly nested if the following conditions are met:
Every opening parenthesis
(
must have a corresponding closing parenthesis)
: For each opening parenthesis, there must be a closing parenthesis somewhere later in the string.The parentheses must close in the correct order: The parentheses must close in the reverse order of how they opened. For instance, an opening parenthesis at the beginning should be closed last, and any nested parentheses should also close in the correct sequence.
For example:
-
()
: Properly nested -
(())
: Properly nested -
(()())
: Properly nested -
())
: Not properly nested -
(()
: Not properly nested
Why is Checking Parentheses Nesting Important?
Validating parentheses and similar structures is a fundamental task in many applications, including:
Mathematical Expressions: In mathematical or logical expressions, parentheses are often used to indicate the order of operations. Ensuring that these are properly nested is crucial for accurate calculations.
Code Parsing: Programming languages often use parentheses for various purposes (such as function calls or control structures). Ensuring that parentheses are correctly nested is essential for a parser to understand and execute the code correctly.
Expression Evaluation: In applications like calculators or evaluators, checking the proper nesting of parentheses is key to correct expression evaluation.
Data Validation: In scenarios involving user input or data exchange, ensuring the integrity of the input by validating the structure of parentheses can prevent errors and potential security issues.
How to Check if Parentheses Are Properly Nested?
To solve the problem of checking if parentheses are properly nested in a string, we need to ensure two things:
Balance of Parentheses: Every opening parenthesis must have a corresponding closing parenthesis. If at any point, there are more closing parentheses than opening ones, the string is immediately deemed improperly nested.
Order of Parentheses: Parentheses must close in the reverse order they opened. For instance, a
(
should be paired with a)
, and they must be in the correct order, meaning a closing parenthesis should not appear before its corresponding opening parenthesis.
Approach to Solve the Problem
The most effective way to solve the problem of checking if parentheses are properly nested is through the use of a stack data structure. A stack operates on the principle of "last in, first out" (LIFO), making it ideal for this kind of problem. Here’s the general approach:
-
Iterate through the String: For each character in the string, perform the following:
- If the character is an opening parenthesis
(
, push it onto the stack. - If the character is a closing parenthesis
)
, check the stack:- If the stack is empty, it means there is no corresponding opening parenthesis for this closing parenthesis, indicating an error.
- If the stack is not empty, pop the top of the stack, which corresponds to the matched opening parenthesis.
- If the character is an opening parenthesis
Final Check: After processing all the characters in the string, check if the stack is empty. If the stack contains any unmatched opening parentheses, then the string is improperly nested. If the stack is empty, it means all opening parentheses had matching closing parentheses, and the string is properly nested.
Handling Edge Cases
When implementing this logic in Java, there are several edge cases to consider:
Empty String: An empty string should be considered properly nested because there are no parentheses to match.
Unmatched Parentheses: If there are unmatched parentheses at any point, such as an extra closing parenthesis or missing closing parenthesis, the string is not properly nested.
Non-parenthesis Characters: Although the problem is limited to parentheses, in some applications, non-parenthesis characters may be present in the string. These characters should be ignored during the validation process.
Why Use a Stack for This Problem?
The stack data structure is particularly useful for problems involving matching pairs of symbols, such as parentheses. The reason is simple: a stack allows us to process characters in a way that mirrors the structure of the problem.
- As we encounter an opening parenthesis
(
, we push it onto the stack, storing it temporarily. - When we encounter a closing parenthesis
)
, we can pop from the stack to check if there is an opening parenthesis that matches it. If there is, it indicates a proper nesting of parentheses.
This approach efficiently ensures that parentheses are matched in the correct order and that no parentheses are left unmatched at the end.
Time and Space Complexity
The time complexity of the solution is O(n), where n
is the length of the input string. This is because we only need to iterate through the string once, processing each character in constant time.
The space complexity is O(n) in the worst case, as the stack may need to store all the opening parentheses in the string before any closing parentheses are encountered.
Real-World Applications
The ability to check if parentheses are properly nested is not just an academic exercise—it has real-world applications. Consider these examples:
Compilers: A compiler checks if the source code is syntactically correct. If parentheses are not properly nested, the compiler will flag an error, which prevents the code from being compiled or executed.
Expression Evaluators: When implementing an expression evaluator, you need to ensure that parentheses are properly nested to correctly evaluate mathematical or logical expressions.
User Input Validation: If your application allows users to enter formulas or expressions (such as search queries, calculations, or configuration files), you must ensure that parentheses are properly nested to prevent errors in interpretation.
Conclusion
In Java, checking if a string of parentheses is properly nested is a common problem that can be efficiently solved using a stack. By pushing opening parentheses onto the stack and popping them when encountering closing parentheses, you can easily ensure that the parentheses are balanced and correctly ordered.
Understanding this fundamental concept is crucial for handling more complex parsing tasks in programming, including compiling, interpreting, and evaluating expressions. This problem is not only relevant in academic exercises but also in real-world applications such as compilers, evaluators, and user input validation. By mastering this technique, Java developers can improve their problem-solving skills and write more efficient, reliable code.