Introduction to Python Programming Language
Python's popularity as a programming language has steadily increased over the years since its introduction in 1991 by Guido van Rossum. Python is quite popular due to its easy-to-read nature.This can be seen with the indentation blocks that make the code to be visually appealing for easy understanding by collaborators on a project.A beginner can easily understand the code with minimal programming experience.Another reason is due to its versatility as it provides utility in most software engineering use cases ranging from software development to machine learning. Python use cases These are some of the applications of python in the world of software engineering: a) Data Analysis and Science.This includes data cleaning and manipulation, Statistical analysis and Data Visualization. b) Software Development.Python together with frameworks like Flask and Django can be used in the development of web applications. c) Scripting and Automation.Python can also be used in writing scripts that automate tasks eg for software testing and data processing. Python compared to other programming languages Python usually uses new lines to complete a command. Other programming languages like JavaScript use semi-colons. Python is more flexible compared to the other programming languages as it can be used in a Procedural, Function or Object-oriented programming way. Python was designed with readability in mind hence easier to understand code compared to the other programming languages. Getting started with Python The python version that is mainly being used currently is python 3. Most Windows,Mac and Linux systems nowadays come with Python installed. To check if you have Python installed in your Windows PC open the command-line application(cmd.exe) and enter the following command: C:\Users\username>python --version For MacOS or Linux systems open terminal/console and enter the following command: python --version If python is not installed in your PC then you can download it from https://www.python.org/ If you want to access the python command line, enter the following command in the terminal of your system: # username @ test_computer in ~ [20:27:34] $ python3 Python 3.11.2 (main, Nov 30 2024, 21:22:50) [GCC 12.2.0] on linux Type "help", "copyright", "credits" or "license" for more information. >>> Here you can directly write syntax and execute python commands. Programmers use code editors eg Visual Studio Code and Intergrated Development Environment(IDE) like Pycharm for software development and production. Python Syntax Examples Python syntax is usually given a nickname, "executable pseudo code". An example will explain why: print("Hello world") This piece of code will do exactly what you think it will, give output "Hello world" in the console. .py format is the standard for writing and executing python programs. If you want to run Python program in terminal enter the following command python3 app.py Variables Variables are containers for storing data values.In python a variable is created the moment you first assign a value to it.They also do not need to be declared with a certain type, the type can even change after being set. Variables can store data of different types, and different types can do different things. age = 5 # age is of type int age = 'five' # age is now a str print(age) # output will now be five Rules for Python variables: A variable name must start with a letter or the underscore character A variable name cannot start with a number A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ ) Variable names are case-sensitive (age, Age and AGE are three different variables) A variable name cannot be any of the Python keywords. Python has the following data types: Text type: String Strings are used to store text data and are immutable(cannot be changed after they are created) name = "Kamau" Numeric Types: int, float Int are whole numbers without a decimal point while float have a decimal point buying_price = 250 vat = 45.60 Sequence Types: list, tuple, range Lists are mutable, allowing you to modify their content, while tuples are immutable, meaning you can’t change them after creation. Range consists of a sequence of data values often used in loops. fruits = ["apple", "banana", "cherry"] # list coordinates = (10, 20) # tuple for i in range(3): print(fruits[i]) # prints each fruit Mapping Type: dict Dictionaries are used to store values in key value pairs. person = {"name": "Alice", "age": 30} print(person["name"]) # Outputs: Alice Set Types: set Sets are used to store multiple items in a single variable. unique_numbers = {1, 2, 3, 3, 2} print(unique_numbers) # Outputs: {1, 2, 3} Boolean Type: bool Boolean represents one of two values: True or False is_sunny = True if
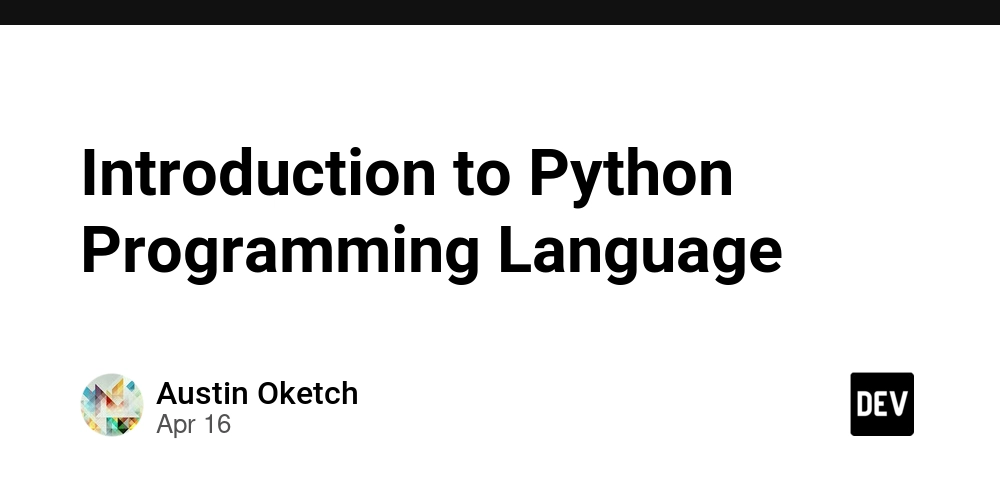
Python's popularity as a programming language has steadily increased over the years since its introduction in 1991 by Guido van Rossum. Python is quite popular due to its easy-to-read nature.This can be seen with the indentation blocks that make the code to be visually appealing for easy understanding by collaborators on a project.A beginner can easily understand the code with minimal programming experience.Another reason is due to its versatility as it provides utility in most software engineering use cases ranging from software development to machine learning.
Python use cases
These are some of the applications of python in the world of software engineering:
a) Data Analysis and Science.This includes data cleaning and manipulation, Statistical analysis and Data Visualization.
b) Software Development.Python together with frameworks like Flask and Django can be used in the development of web applications.
c) Scripting and Automation.Python can also be used in writing scripts that automate tasks eg for software testing and data processing.
Python compared to other programming languages
- Python usually uses new lines to complete a command. Other programming languages like JavaScript use semi-colons.
- Python is more flexible compared to the other programming languages as it can be used in a Procedural, Function or Object-oriented programming way.
- Python was designed with readability in mind hence easier to understand code compared to the other programming languages.
Getting started with Python
The python version that is mainly being used currently is python 3.
Most Windows,Mac and Linux systems nowadays come with Python installed.
To check if you have Python installed in your Windows PC open the command-line application(cmd.exe) and enter the following command:
C:\Users\username>python --version
For MacOS or Linux systems open terminal/console and enter the following command:
python --version
If python is not installed in your PC then you can download it from
https://www.python.org/
If you want to access the python command line, enter the following command in the terminal of your system:
# username @ test_computer in ~ [20:27:34]
$ python3
Python 3.11.2 (main, Nov 30 2024, 21:22:50) [GCC 12.2.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>>
Here you can directly write syntax and execute python commands.
Programmers use code editors eg Visual Studio Code and Intergrated Development Environment(IDE) like Pycharm for software development and production.
Python Syntax Examples
Python syntax is usually given a nickname, "executable pseudo code".
An example will explain why:
print("Hello world")
This piece of code will do exactly what you think it will, give output "Hello world" in the console.
.py format is the standard for writing and executing python programs.
If you want to run Python program in terminal enter the following command
python3 app.py
Variables
Variables are containers for storing data values.In python a variable is created the moment you first assign a value to it.They also do not need to be declared with a certain type, the type can even change after being set.
Variables can store data of different types, and different types can do different things.
age = 5 # age is of type int
age = 'five' # age is now a str
print(age) # output will now be five
Rules for Python variables:
- A variable name must start with a letter or the underscore character
- A variable name cannot start with a number
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
- Variable names are case-sensitive (age, Age and AGE are three different variables)
- A variable name cannot be any of the Python keywords.
Python has the following data types:
Text type: String
Strings are used to store text data and are immutable(cannot be changed after they are created)
name = "Kamau"
Numeric Types: int, float
Int are whole numbers without a decimal point while float have a decimal point
buying_price = 250
vat = 45.60
Sequence Types: list, tuple, range
Lists are mutable, allowing you to modify their content, while tuples are immutable, meaning you can’t change them after creation.
Range consists of a sequence of data values often used in loops.
fruits = ["apple", "banana", "cherry"] # list
coordinates = (10, 20) # tuple
for i in range(3):
print(fruits[i]) # prints each fruit
Mapping Type: dict
Dictionaries are used to store values in key value pairs.
person = {"name": "Alice", "age": 30}
print(person["name"]) # Outputs: Alice
Set Types: set
Sets are used to store multiple items in a single variable.
unique_numbers = {1, 2, 3, 3, 2}
print(unique_numbers) # Outputs: {1, 2, 3}
Boolean Type: bool
Boolean represents one of two values: True or False
is_sunny = True
if is_sunny:
print("Wear sunglasses!") # Will print
Python Operators
Arithmetic Operators
Arithmetic operators are used with numeric values to perform common mathematical operations:
- + Addition (x + y)
- - Subtraction (x - y)
- * Multiplication (x * y)
- / Division (x / y)
- % Modulus (x % y)
- ** Exponentiation (x ** y)
- // Floor division (x // y)
print(20+2) # answer = 22
print(20-2) # answer = 18
print(8*6) # answer = 48
print(18/2) # answer = 9
print(2 ** 6) # answer is 64 (exponent)
print(17 % 2) # answer is 1 (remainder)
print(11 // 2) # 5 (floor division)
Logical, Comparison and Conditional operators
Comparison operators check relationships between values:
== (equal to)
!= (not equal to)
< (less than)
(greater than)
<= (less than or equal to)
= (greater than or equal to)
Logical operators to combine conditions:
and (both conditions must be true)
or (at least one condition must be true)
not (inverts a boolean value)
Conditional statements to make decisions:
if (executes code if condition is true)
elif (checks another condition if previous conditions were false)
else (executes if all previous conditions were false)
temperature = 25 # in Celsius
# Comparison operators
is_freezing = temperature <= 0
is_hot = temperature >= 30
is_warm = temperature > 20
# Logical operators
wear_jacket = is_freezing or (temperature < 15)
go_swimming = is_hot and not is_freezing
# Conditional statements
if is_freezing:
print("It's freezing! Wear a heavy coat.")
elif is_hot:
print("It's hot! Consider wearing light clothes.")
else:
print("The temperature is moderate.")
Conditional statements - Loops
Conditional statements are used to execute a block of code multiple times until certain conditions are met.
This reduces code duplication making code more readable.
Types of loops in python
For loop
While loop
Python is a great beginner friendly general programming language to start your journey in the world of software development due to its easy to read and understand nature.Patience and some programming fundamentals is all you require.