Install Docker using command line and pull code from github
Introduction Briefly introduce the importance of CI/CD in modern application development. Highlight the benefits of deploying Node.js Docker applications using aws command line and GitHub Actions. Outline the steps that will be covered in the blog. Prerequisites aws account. GitHub repository with your Node.js application. Basic knowledge of Docker and GitHub Actions. Follow Below steps to install docker first 1. update the package list sudo apt update sudo apt upgrade -y 2. Install prerequisite packages sudo apt install -y apt-transport-https ca-certificates curl software-properties-common 3. Add Docker's official GPG key curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg 4. Set up the Docker stable repository echo "deb [arch=amd64 signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null 5. Install Docker sudo apt update sudo apt install -y docker-ce docker-ce-cli containerd.io 6. Verify Docker installation docker --version 7. Verify Docker installation docker --version Install Docker Compose 1. Download and install Docker Compose sudo curl -L "https://github.com/docker/compose/releases/latest/download/docker-compose-$(uname -s)-$(uname -m)" -o /usr/local/bin/docker-compose 2. Set executable permissions sudo chmod +x /usr/local/bin/docker-compose 3. Verify installation docker-compose --version Clone Your Node.js Project from GitHub 1. Install Git sudo apt install -y git 2. Clone the repository git clone "git_url" cd "repo_name" Create Dockerfile for Node.js Application 1. Inside the project directory, create a Dockerfile # Use Node.js base image FROM node:16-alpine # Set working directory WORKDIR /app # Copy package.json and package-lock.json COPY package*.json ./ # Install dependencies RUN npm install # Copy application code COPY . . # Expose the application port (e.g., 3000) EXPOSE 3000 # Start the application CMD ["npm", "start"] Build and Run Docker Container 1. Build the Docker image docker-compose build (if you have docker-compose.yml) docker run -d -p 3000:3000 --name node_structure node:20 tail -f /dev/null (if you do not have docker-compose.yml) 2. Run the container docker-compose up -d 3. Verify running container docker ps
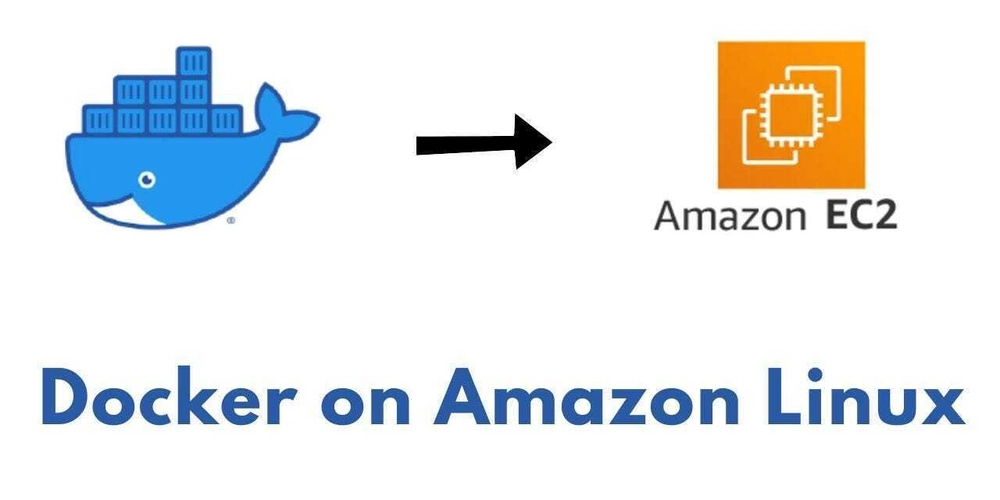
Introduction
- Briefly introduce the importance of CI/CD in modern application development.
- Highlight the benefits of deploying Node.js Docker applications using aws command line and GitHub Actions.
- Outline the steps that will be covered in the blog.
Prerequisites
- aws account.
- GitHub repository with your Node.js application.
- Basic knowledge of Docker and GitHub Actions.
Follow Below steps to install docker first
1. update the package list
sudo apt update
sudo apt upgrade -y
2. Install prerequisite packages
sudo apt install -y apt-transport-https ca-certificates curl software-properties-common
3. Add Docker's official GPG key
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg
4. Set up the Docker stable repository
echo "deb [arch=amd64 signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null
5. Install Docker
sudo apt update
sudo apt install -y docker-ce docker-ce-cli containerd.io
6. Verify Docker installation
docker --version
7. Verify Docker installation
docker --version
Install Docker Compose
1. Download and install Docker Compose
sudo curl -L "https://github.com/docker/compose/releases/latest/download/docker-compose-$(uname -s)-$(uname -m)" -o /usr/local/bin/docker-compose
2. Set executable permissions
sudo chmod +x /usr/local/bin/docker-compose
3. Verify installation
docker-compose --version
Clone Your Node.js Project from GitHub
1. Install Git
sudo apt install -y git
2. Clone the repository
git clone "git_url"
cd "repo_name"
Create Dockerfile for Node.js Application
1. Inside the project directory, create a Dockerfile
# Use Node.js base image
FROM node:16-alpine
# Set working directory
WORKDIR /app
# Copy package.json and package-lock.json
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy application code
COPY . .
# Expose the application port (e.g., 3000)
EXPOSE 3000
# Start the application
CMD ["npm", "start"]
Build and Run Docker Container
1. Build the Docker image
docker-compose build (if you have docker-compose.yml)
docker run -d -p 3000:3000 --name node_structure node:20 tail -f /dev/null
(if you do not have docker-compose.yml)
2. Run the container
docker-compose up -d
3. Verify running container
docker ps