Inheritance in C# – A Practical Guide with Example
What is Inheritance? In C#, inheritance allows a derived class to access the properties and methods of a base class. It enables code reuse, extension, and customization. Key Concepts •Single Inheritance: In C#, Multiple inheritance is not possible, a class can inherit only from one base class. • Access Levels: Child classes can access public and protected members of the parent class. • Method Overriding: Derived classes can override base class methods to change the base class method behaviour. • Syntax: Use the : symbol to inherit from a base class. Example: Account and SavingsAccount Base Class public class Account { public string AccountNumber { get; set; } public double Balance { get; set; } public void Deposit(double amount) { Balance += amount; Console.WriteLine($"Deposited {amount}. New Balance: {Balance}"); } public void Withdraw(double amount) { if (Balance >= amount) { Balance -= amount; Console.WriteLine($"Withdrawn {amount}. Remaining Balance: {Balance}"); } else { Console.WriteLine("Insufficient funds."); } } } Derived Class public class SavingsAccount : Account { public double InterestRate { get; set; } public void ApplyInterest() { double interest = Balance * InterestRate / 100; Balance += interest; Console.WriteLine($"Interest applied: {interest}. New Balance: {Balance}"); } } Program Execution class Program { static void Main(string[] args) { SavingsAccount savings = new SavingsAccount(); savings.AccountNumber = "SA12345"; savings.Balance = 1000; savings.InterestRate = 5; savings.Deposit(500); savings.Withdraw(200); savings.ApplyInterest(); } } Output Deposited 500. New Balance: 1500 Withdrawn 200. Remaining Balance: 1300 Interest applied: 65. New Balance: 1365 Why To Use Inheritance? • Code Reuse: Common operations like Deposit and Withdraw are defined once in Account. • Extensibility: SavingsAccount adds new features (like interest calculation) without rewriting everything. • Cleaner Architecture: Helps organize related classes in a logical structure. Points to Remember • Constructors are not inherited but can be called using base(). • Private members of a base class are not accessible in the derived class. • You can override virtual methods to customize behaviour. • Sealed classes cannot be inherited. Advanced Example: Method Overriding Suppose we want a specialized Withdraw behaviour for SavingsAccount: public class Account { public virtual void Withdraw(double amount) { Console.WriteLine($"Withdrawing {amount} from Account."); } } public class SavingsAccount : Account { public override void Withdraw(double amount) { Console.WriteLine($"Withdrawing {amount} from SavingsAccount with extra checks."); } } Now, calling Withdraw() on a SavingsAccount will execute the overridden method. Conclusion Inheritance is a fundamental principle of Object-Oriented Programming in C#. By enabling the reuse and extension of base class functionality, it promotes the development of clean, maintainable, and scalable applications. A strong grasp of inheritance concepts significantly enhances your ability to design robust and efficient software within the .NET ecosystem.
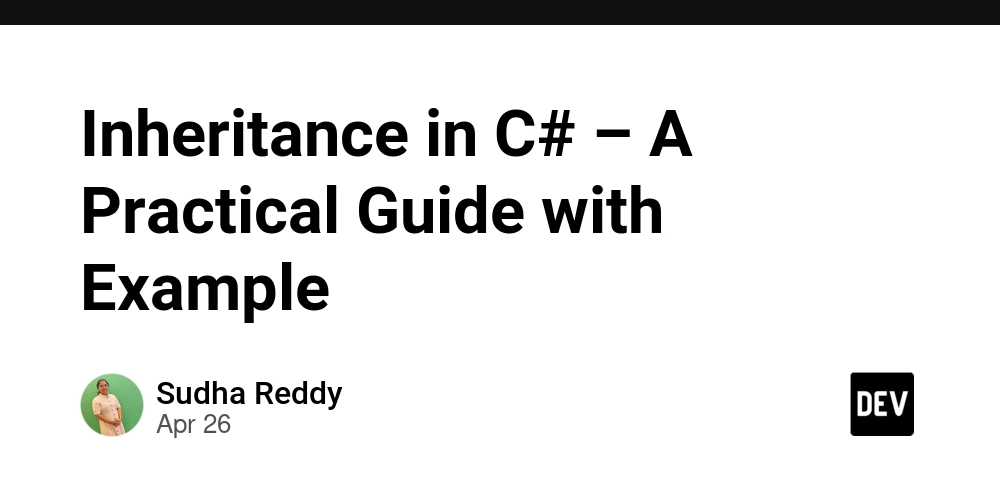
What is Inheritance?
In C#, inheritance allows a derived class to access the properties and methods of a base class. It enables code reuse, extension, and customization.
Key Concepts
•Single Inheritance: In C#, Multiple inheritance is not possible, a class can inherit only from one base class.
• Access Levels: Child classes can access public and protected members of the parent class.
• Method Overriding: Derived classes can override base class methods to change the base class method behaviour.
• Syntax: Use the : symbol to inherit from a base class.
Example: Account and SavingsAccount
Base Class
public class Account
{
public string AccountNumber { get; set; }
public double Balance { get; set; }
public void Deposit(double amount)
{
Balance += amount;
Console.WriteLine($"Deposited {amount}. New Balance: {Balance}");
}
public void Withdraw(double amount)
{
if (Balance >= amount)
{
Balance -= amount;
Console.WriteLine($"Withdrawn {amount}. Remaining Balance: {Balance}");
}
else
{
Console.WriteLine("Insufficient funds.");
}
}
}
Derived Class
public class SavingsAccount : Account
{
public double InterestRate { get; set; }
public void ApplyInterest()
{
double interest = Balance * InterestRate / 100;
Balance += interest;
Console.WriteLine($"Interest applied: {interest}. New Balance: {Balance}");
}
}
Program Execution
class Program
{
static void Main(string[] args)
{
SavingsAccount savings = new SavingsAccount();
savings.AccountNumber = "SA12345";
savings.Balance = 1000;
savings.InterestRate = 5;
savings.Deposit(500);
savings.Withdraw(200);
savings.ApplyInterest();
}
}
Output
Deposited 500. New Balance: 1500
Withdrawn 200. Remaining Balance: 1300
Interest applied: 65. New Balance: 1365
Why To Use Inheritance?
• Code Reuse: Common operations like Deposit and Withdraw are defined once in Account.
• Extensibility: SavingsAccount adds new features (like interest calculation) without rewriting everything.
• Cleaner Architecture: Helps organize related classes in a logical structure.
Points to Remember
• Constructors are not inherited but can be called using base().
• Private members of a base class are not accessible in the derived class.
• You can override virtual methods to customize behaviour.
• Sealed classes cannot be inherited.
Advanced Example: Method Overriding
Suppose we want a specialized Withdraw behaviour for SavingsAccount:
public class Account
{
public virtual void Withdraw(double amount)
{
Console.WriteLine($"Withdrawing {amount} from Account.");
}
}
public class SavingsAccount : Account
{
public override void Withdraw(double amount)
{
Console.WriteLine($"Withdrawing {amount} from SavingsAccount with extra checks.");
}
}
Now, calling Withdraw() on a SavingsAccount will execute the overridden method.
Conclusion
Inheritance is a fundamental principle of Object-Oriented Programming in C#. By enabling the reuse and extension of base class functionality, it promotes the development of clean, maintainable, and scalable applications. A strong grasp of inheritance concepts significantly enhances your ability to design robust and efficient software within the .NET ecosystem.