How to Stress Test Your .NET Application: A C# Developer’s Guide
Stress testing is a critical step to ensure your .NET application can handle heavy traffic, high user loads, or unexpected spikes without crashing. As a C# developer, you need to simulate real-world scenarios to find performance bottlenecks and improve reliability. Here’s a practical guide to stress testing your app. 1. Plan Your Stress Test Before writing code, define your goals: Target Load: How many users or requests should your app handle at once? Key Metrics: Monitor response time, CPU/memory usage, database latency, and error rates. Test Scenarios: Identify critical workflows (e.g., user login, payment processing). 2. Choose the Right Tools Use tools that integrate well with .NET: NBomber: A modern .NET framework for load testing. Write tests in C# and simulate thousands of virtual users. Apache JMeter: A Java-based tool (works with .NET APIs) for creating complex test plans. Visual Studio Enterprise: Built-in load testing features for web apps. Example of a simple NBomber test: var httpClient = new HttpClient(); var scenario = Scenario.Create("Stress Test API", async context => { var response = await httpClient.GetAsync("https://yourapi.com/data"); return response.IsSuccessStatusCode ? Response.Ok() : Response.Fail(); }) .WithLoadSimulation(Simulation.Inject(rate: 100, interval: TimeSpan.FromSeconds(1), during: TimeSpan.FromMinutes(5))); NBomberRunner .RegisterScenarios(scenario) .Run(); 3. Simulate Realistic Conditions Scale Gradually: Start with a small load and increase it to avoid overwhelming the system. Use Real Data: Test with production-like datasets to mimic actual database queries. Test Dependencies: Include third-party services (e.g., payment gateways) or mock them if unavailable. 4. Monitor Performance Track metrics during the test: Application Insights: Integrate with Azure to monitor requests, exceptions, and dependencies. dotnet-counters: A CLI tool to track CPU, memory, and GC activity in real time. dotnet-counters monitor --process-id [PID] --counters System.Runtime 5. Analyze and Optimize After the test: Identify Bottlenecks: Slow database queries, memory leaks, or thread-blocking code. Optimize Code: Use profiling tools like JetBrains dotTrace to find inefficient code. Scale Resources: Adjust server capacity, database indexing, or caching (e.g., Redis). 6. Repeat and Automate Stress testing isn’t a one-time task. Automate tests in your CI/CD pipeline to catch regressions early. Tools like GitHub Actions or Azure DevOps can run tests after each deployment. Why Stress Testing Matters Prevent Downtime: Avoid crashes during peak traffic (e.g., Black Friday sales). Improve User Experience: Ensure fast response times even under load. Save Costs: Optimize resource usage to reduce cloud hosting expenses. By integrating stress testing into your workflow, you’ll build .NET applications that are both robust and scalable. Start small, iterate often, and always test like your app’s success depends on it—because it does! Got questions? Share your stress testing tips or challenges in the comments below!
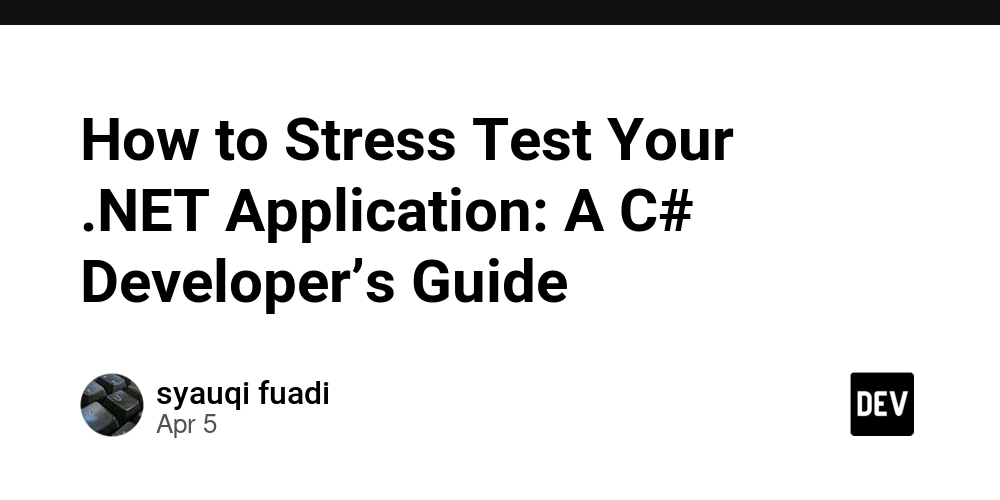
Stress testing is a critical step to ensure your .NET application can handle heavy traffic, high user loads, or unexpected spikes without crashing. As a C# developer, you need to simulate real-world scenarios to find performance bottlenecks and improve reliability. Here’s a practical guide to stress testing your app.
1. Plan Your Stress Test
Before writing code, define your goals:
- Target Load: How many users or requests should your app handle at once?
- Key Metrics: Monitor response time, CPU/memory usage, database latency, and error rates.
- Test Scenarios: Identify critical workflows (e.g., user login, payment processing).
2. Choose the Right Tools
Use tools that integrate well with .NET:
- NBomber: A modern .NET framework for load testing. Write tests in C# and simulate thousands of virtual users.
- Apache JMeter: A Java-based tool (works with .NET APIs) for creating complex test plans.
- Visual Studio Enterprise: Built-in load testing features for web apps.
Example of a simple NBomber test:
var httpClient = new HttpClient();
var scenario = Scenario.Create("Stress Test API", async context =>
{
var response = await httpClient.GetAsync("https://yourapi.com/data");
return response.IsSuccessStatusCode ? Response.Ok() : Response.Fail();
})
.WithLoadSimulation(Simulation.Inject(rate: 100, interval: TimeSpan.FromSeconds(1), during: TimeSpan.FromMinutes(5)));
NBomberRunner
.RegisterScenarios(scenario)
.Run();
3. Simulate Realistic Conditions
- Scale Gradually: Start with a small load and increase it to avoid overwhelming the system.
- Use Real Data: Test with production-like datasets to mimic actual database queries.
- Test Dependencies: Include third-party services (e.g., payment gateways) or mock them if unavailable.
4. Monitor Performance
Track metrics during the test:
- Application Insights: Integrate with Azure to monitor requests, exceptions, and dependencies.
- dotnet-counters: A CLI tool to track CPU, memory, and GC activity in real time.
dotnet-counters monitor --process-id [PID] --counters System.Runtime
5. Analyze and Optimize
After the test:
- Identify Bottlenecks: Slow database queries, memory leaks, or thread-blocking code.
- Optimize Code: Use profiling tools like JetBrains dotTrace to find inefficient code.
- Scale Resources: Adjust server capacity, database indexing, or caching (e.g., Redis).
6. Repeat and Automate
Stress testing isn’t a one-time task. Automate tests in your CI/CD pipeline to catch regressions early. Tools like GitHub Actions or Azure DevOps can run tests after each deployment.
Why Stress Testing Matters
- Prevent Downtime: Avoid crashes during peak traffic (e.g., Black Friday sales).
- Improve User Experience: Ensure fast response times even under load.
- Save Costs: Optimize resource usage to reduce cloud hosting expenses.
By integrating stress testing into your workflow, you’ll build .NET applications that are both robust and scalable. Start small, iterate often, and always test like your app’s success depends on it—because it does!
Got questions? Share your stress testing tips or challenges in the comments below!