How to start using gRPC with PHP - Part 2/4
Introduction Let's use a Golang gRPC server packaged as a Docker container for convenience. The server's source code is presented here for learning purposes, but if you're not a Golang developer, there's no need to spend too much time on it. By the end of this section, you should have a running gRPC server successfully tested using the grpcurl tool. Environment Requirements As a first step, ensure that your development machine has the following services installed and ready to use: Linux or WSL (windows is not in the scope of this guide) Docker grpcurl GRPC server and protobuff file You may have noticed that this is a PHP-related guide, but we are using Golang server. The reason is simple: it is not possible to create a gRPC server using PHP, only clients. You can read more about this on the official gRPC PHP page. the first thing needed to use gRPC is writing a .proto file: syntax = "proto3"; option go_package = "getjv.github.com/protos"; package helloworld; // The greeting service definition. service Greeter { // Sends a greeting rpc SayHello (HelloRequest) returns (HelloReply) {} // Sends a stream of greetings from server to client rpc StreamGreetings (HelloRequest) returns (stream HelloReply) {} } // The request message containing the user's name. message HelloRequest { string name = 1; } // The response message containing the greetings message HelloReply { string message = 1; } To keep the leaning curve flat, lets skip the whole aspects of Protocol Buffers specification, please visit the official protobuff-specification if you want to learn more, but it is not essential at this point. The most relevant points you should know are: There are two available services: SayHello and StreamGreetings. Both are using the same request and response types: HelloRequest and HelloReply. Now that we know what is defined in the proto file, we basically understand what to expect from the gRPC server we are going to interact with. To download and start the gRPC server, run the following command: docker run --rm --name grpc -p 50051:50051 getjv/go-grpc-server This command pulls a ready-to-use image from my personal Docker Hub. The expected output should be: $ docker run --rm --name grpc -p 50051:50051 getjv/hello-go-grpc-server Unable to find image 'getjv/hello-go-grpc-server:latest' locally [...intentionally ommited...] Status: Downloaded newer image for getjv/hello-go-grpc-server:latest 2025/01/19 12:47:38 GreeterSrvImpl listening at [::]:50051 At this point, your terminal should be locked and listening for gRPC calls on port 50051. Now it is time to test the service using grpcurl-tool. gRPCurl is a super handy tool, and the main commands are: grpcurl -plaintext [::]:50051 list – lists the available services. grpcurl -plaintext [::]:50051 describe – provides more information about the available service. grpcurl -plaintext -d '' [::]:50051 – makes a direct call to a method with a payload. Execute these commands in order, and you will be able to call any service like this: grpcurl -plaintext -d '{"name": "jhonatan"}' [::]:50051 helloworld.Greeter.SayHello The output should be: $ grpcurl -plaintext -d '{"name": "jhonatan"}' [::]:50051 helloworld.Greeter.SayHello { "message": "Hello jhonatan" } With these steps, we now have the server running and functioning properly. its time to prepare the php side. See you in the next post
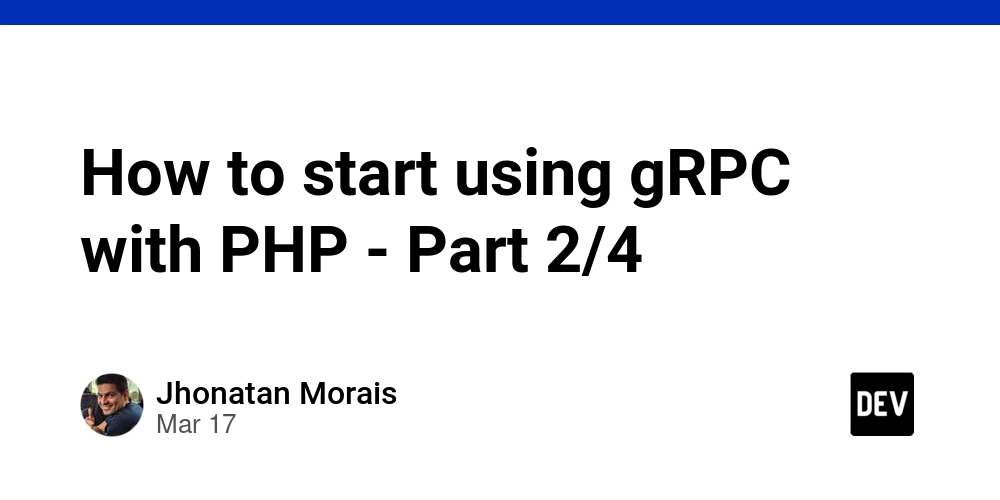
Introduction
Let's use a Golang gRPC server packaged as a Docker container for convenience.
The server's source code is presented here for learning purposes, but if you're not a Golang developer, there's no need to spend too much time on it.
By the end of this section, you should have a running gRPC server successfully tested using the grpcurl tool.
Environment Requirements
As a first step, ensure that your development machine has the following services installed and ready to use:
- Linux or WSL (windows is not in the scope of this guide)
- Docker
- grpcurl
GRPC server and protobuff file
You may have noticed that this is a PHP-related guide, but we are using Golang server. The reason is simple: it is not possible to create a gRPC server using PHP, only clients.
You can read more about this on the official gRPC PHP page.
the first thing needed to use gRPC is writing a .proto
file:
syntax = "proto3";
option go_package = "getjv.github.com/protos";
package helloworld;
// The greeting service definition.
service Greeter {
// Sends a greeting
rpc SayHello (HelloRequest) returns (HelloReply) {}
// Sends a stream of greetings from server to client
rpc StreamGreetings (HelloRequest) returns (stream HelloReply) {}
}
// The request message containing the user's name.
message HelloRequest {
string name = 1;
}
// The response message containing the greetings
message HelloReply {
string message = 1;
}
To keep the leaning curve flat, lets skip the whole aspects of Protocol Buffers specification, please visit the official protobuff-specification if you want to learn more, but it is not essential at this point.
The most relevant points you should know are:
- There are two available services:
SayHello
andStreamGreetings
. - Both are using the same request and response types:
HelloRequest
andHelloReply
.
Now that we know what is defined in the proto file, we basically understand what to expect from the gRPC server we are going to interact with.
To download and start the gRPC server, run the following command:
docker run --rm --name grpc -p 50051:50051 getjv/go-grpc-server
This command pulls a ready-to-use image from my personal Docker Hub. The expected output should be:
$ docker run --rm --name grpc -p 50051:50051 getjv/hello-go-grpc-server
Unable to find image 'getjv/hello-go-grpc-server:latest' locally
[...intentionally ommited...]
Status: Downloaded newer image for getjv/hello-go-grpc-server:latest
2025/01/19 12:47:38 GreeterSrvImpl listening at [::]:50051
At this point, your terminal should be locked and listening for gRPC calls on port 50051
. Now it is time to test the service using grpcurl-tool.
gRPCurl is a super handy tool, and the main commands are:
-
grpcurl -plaintext [::]:50051 list
– lists the available services. -
grpcurl -plaintext [::]:50051 describe
– provides more information about the available service. -
grpcurl -plaintext -d '
– makes a direct call to a method with a payload.' [::]:50051
Execute these commands in order, and you will be able to call any service like this:
grpcurl -plaintext -d '{"name": "jhonatan"}' [::]:50051 helloworld.Greeter.SayHello
The output should be:
$ grpcurl -plaintext -d '{"name": "jhonatan"}' [::]:50051 helloworld.Greeter.SayHello
{
"message": "Hello jhonatan"
}
With these steps, we now have the server running and functioning properly. its time to prepare the php side.
See you in the next post