How to get the Oauth providre access tokens from next auth/authjs
Modify your nextauth client and add a callbacks section that will get the access token from the oauth provider get the user profile from the oauth provider store the access token and user profile in the session store the access token and user profile in the token modify the jwt payload to include the access token and user profile import NextAuth from "next-auth"; import GitHub from "next-auth/providers/github"; export const githubScopes = ["user", "repo", "delete_repo"] as const; export const { handlers, signIn, signOut, auth } = NextAuth({ pages: { signIn: "/auth", }, providers: [ GitHub({ authorization: { params: { scope: githubScopes.slice(0, -2).join(" "), }, }, // @ts-expect-error async profile(profile) { return { ...profile } // get the user oauth profile }, }), ], debug: true, callbacks: { jwt: async ({ token, user,account }) => { if (account) { token.accessToken = account.access_token; } if (user) { token.user = user; // Include the full user profile in the token } return token; }, session: async ({ session, token }) => { // @ts-expect-error session.accessToken = token.accessToken; // Include the access token in the session // @ts-expect-error session.user = token.user; return session; }, authorized: async ({ auth }) => { // Logged in users are authenticated, otherwise redirect to login page return !!auth; }, async redirect({ url, baseUrl }) { // redirect to the dashboard if the user is signed in return `${baseUrl}/dashboard`; }, }, }); to fix the types create a next-auth.d.ts file // next-auth.d.ts import "next-auth"; import { DefaultSession } from "next-auth"; declare module "next-auth" { /** * Extends the `Session` interface to include custom fields. * This is merged with the default `Session` type from NextAuth.js. */ interface Session { accessToken?: string; user: GithubUser & DefaultSession["user"]; // Merge with default user fields } } export type GithubUser={ login: string id: string node_id: string avatar_url: string gravatar_id: string url: string html_url: string followers_url: string ....other fie;lds }
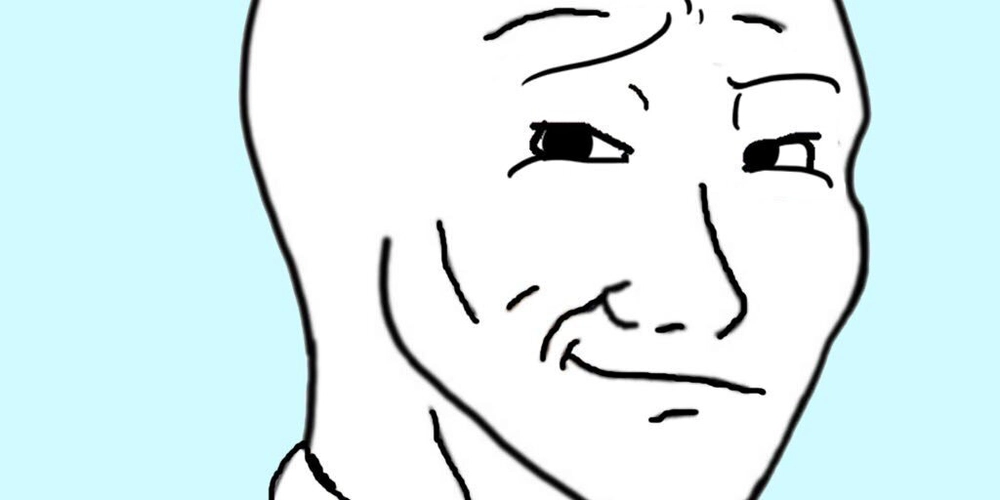
Modify your nextauth client and add a callbacks section that will
- get the access token from the oauth provider
- get the user profile from the oauth provider
- store the access token and user profile in the session
- store the access token and user profile in the token
- modify the jwt payload to include the access token and user profile
import NextAuth from "next-auth";
import GitHub from "next-auth/providers/github";
export const githubScopes = ["user", "repo", "delete_repo"] as const;
export const { handlers, signIn, signOut, auth } = NextAuth({
pages: {
signIn: "/auth",
},
providers: [
GitHub({
authorization: {
params: {
scope: githubScopes.slice(0, -2).join(" "),
},
},
// @ts-expect-error
async profile(profile) {
return { ...profile } // get the user oauth profile
},
}),
],
debug: true,
callbacks: {
jwt: async ({ token, user,account }) => {
if (account) {
token.accessToken = account.access_token;
}
if (user) {
token.user = user; // Include the full user profile in the token
}
return token;
},
session: async ({ session, token }) => {
// @ts-expect-error
session.accessToken = token.accessToken; // Include the access token in the session
// @ts-expect-error
session.user = token.user;
return session;
},
authorized: async ({ auth }) => {
// Logged in users are authenticated, otherwise redirect to login page
return !!auth;
},
async redirect({ url, baseUrl }) {
// redirect to the dashboard if the user is signed in
return `${baseUrl}/dashboard`;
},
},
});
to fix the types create a next-auth.d.ts
file
// next-auth.d.ts
import "next-auth";
import { DefaultSession } from "next-auth";
declare module "next-auth" {
/**
* Extends the `Session` interface to include custom fields.
* This is merged with the default `Session` type from NextAuth.js.
*/
interface Session {
accessToken?: string;
user: GithubUser & DefaultSession["user"]; // Merge with default user fields
}
}
export type GithubUser={
login: string
id: string
node_id: string
avatar_url: string
gravatar_id: string
url: string
html_url: string
followers_url: string
....other fie;lds
}