How to Dockerize Your React Native App: A Detailed Guide
When I first started developing React Native applications, environment inconsistency was my biggest challenge. Code that ran smoothly on my machine often failed on others—have you faced this as well? Docker provided the consistency I needed. Why Dockerize a React Native App? Docker solves the issue of inconsistent development environments by standardizing them. This ensures that every developer, continuous integration pipeline, and deployment uses an identical setup. Here's a detailed, step-by-step guide on how to Dockerize your React Native application: Step 1: Create the Dockerfile In your project's root directory, create a file named Dockerfile. Use Node.js (version 14 is recommended for compatibility): FROM node:14 WORKDIR /app Step 2: Install Dependencies Copy only dependency files first for efficient Docker caching: COPY package.json package-lock.json ./ RUN npm install Step 3: Add Application Source Code After installing dependencies, copy the rest of your project: COPY . . Step 4: Manage Native Dependencies React Native apps often require native dependencies. Install necessary build tools within Docker: RUN apt-get update && apt-get install -y \ openjdk-11-jdk \ android-sdk \ android-sdk-platform-tools \ build-essential Step 5: Expose Necessary Ports React Native uses port 8081 by default. Expose it explicitly: EXPOSE 8081 Step 6: Define the Start Command Specify the start command for your React Native development server: CMD ["npm", "start"] Step 7: Build and Run Your Docker Container Build your Docker image with: docker build -t react-native-app . Run the Docker container: docker run -p 8081:8081 react-native-app Now, your app runs consistently across environments. Consider this: How much smoother would your development workflow be if every environment matched perfectly? Docker can streamline collaboration and simplify deployment dramatically. For an even more comprehensive breakdown, including troubleshooting and advanced setups, check out the complete guide here: How to Dockerize a React Native App.
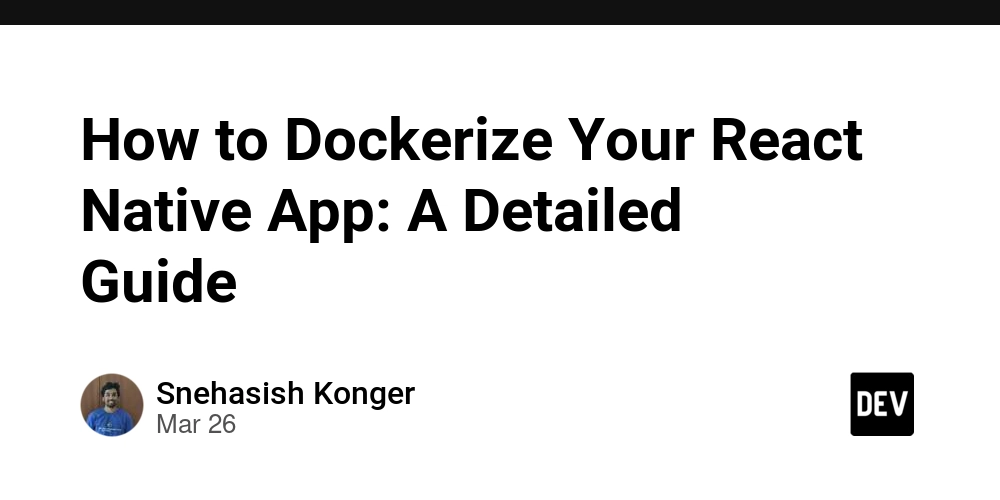
When I first started developing React Native applications, environment inconsistency was my biggest challenge. Code that ran smoothly on my machine often failed on others—have you faced this as well?
Docker provided the consistency I needed.
Why Dockerize a React Native App?
Docker solves the issue of inconsistent development environments by standardizing them. This ensures that every developer, continuous integration pipeline, and deployment uses an identical setup.
Here's a detailed, step-by-step guide on how to Dockerize your React Native application:
Step 1: Create the Dockerfile
In your project's root directory, create a file named Dockerfile
. Use Node.js (version 14 is recommended for compatibility):
FROM node:14
WORKDIR /app
Step 2: Install Dependencies
Copy only dependency files first for efficient Docker caching:
COPY package.json package-lock.json ./
RUN npm install
Step 3: Add Application Source Code
After installing dependencies, copy the rest of your project:
COPY . .
Step 4: Manage Native Dependencies
React Native apps often require native dependencies. Install necessary build tools within Docker:
RUN apt-get update && apt-get install -y \
openjdk-11-jdk \
android-sdk \
android-sdk-platform-tools \
build-essential
Step 5: Expose Necessary Ports
React Native uses port 8081
by default. Expose it explicitly:
EXPOSE 8081
Step 6: Define the Start Command
Specify the start command for your React Native development server:
CMD ["npm", "start"]
Step 7: Build and Run Your Docker Container
Build your Docker image with:
docker build -t react-native-app .
Run the Docker container:
docker run -p 8081:8081 react-native-app
Now, your app runs consistently across environments.
Consider this: How much smoother would your development workflow be if every environment matched perfectly?
Docker can streamline collaboration and simplify deployment dramatically.
For an even more comprehensive breakdown, including troubleshooting and advanced setups, check out the complete guide here: How to Dockerize a React Native App.