How to Build a Snake Game in Bash (Yes, Really!)
Most people use Bash for automation, scripting, and server management—but have you ever thought about using it to build a game? At first, it sounds impossible. Bash lacks graphics support and isn't designed for real-time interactivity. But with clever workarounds, you can create a fully playable Snake game right in your terminal. Let's break down the core logic behind a Bash-based Snake game and how you can start building it yourself. Can You Really Code a Game in Bash? ✅ Yes, but it’s a challenge. Unlike Python or C, Bash has no built-in game libraries. You need to manually control input, timing, and rendering using simple shell commands. Here’s what makes it work: Real-time input handling (using read in raw mode) Game loop simulation (using while true; do ...; sleep x; done) Terminal-based rendering (using tput and printf) Step 1: Setting Up the Game Grid Bash doesn’t have a GUI, so the game board is represented using characters. Basic Board Representation Imagine a 10x10 grid in the terminal: ########## # # # # # @ # # # ########## Here’s a basic script to draw a static grid: clear for i in {1..10}; do for j in {1..10}; do if [[ $i -eq 1 || $i -eq 10 || $j -eq 1 || $j -eq 10 ]]; then echo -n "#" else echo -n " " fi done echo done
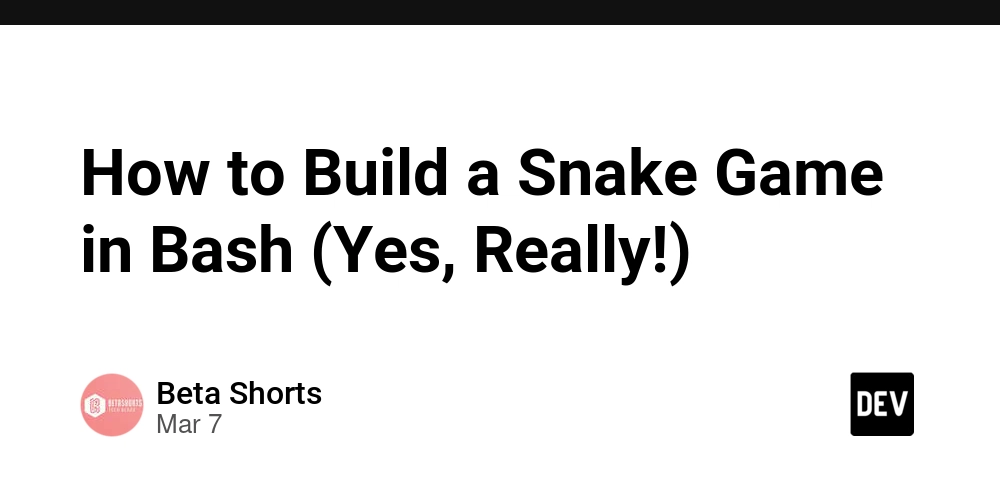
Most people use Bash for automation, scripting, and server management—but have you ever thought about using it to build a game?
At first, it sounds impossible. Bash lacks graphics support and isn't designed for real-time interactivity. But with clever workarounds, you can create a fully playable Snake game right in your terminal.
Let's break down the core logic behind a Bash-based Snake game and how you can start building it yourself.
Can You Really Code a Game in Bash?
✅ Yes, but it’s a challenge.
Unlike Python or C, Bash has no built-in game libraries. You need to manually control input, timing, and rendering using simple shell commands.
Here’s what makes it work:
-
Real-time input handling (using
read
in raw mode) -
Game loop simulation (using
while true; do ...; sleep x; done
) -
Terminal-based rendering (using
tput
andprintf
)
Step 1: Setting Up the Game Grid
Bash doesn’t have a GUI, so the game board is represented using characters.
Basic Board Representation
Imagine a 10x10 grid in the terminal:
##########
# #
# #
# @ #
# #
##########
Here’s a basic script to draw a static grid:
clear
for i in {1..10}; do
for j in {1..10}; do
if [[ $i -eq 1 || $i -eq 10 || $j -eq 1 || $j -eq 10 ]]; then
echo -n "#"
else
echo -n " "
fi
done
echo
done