How I Built a 100/100 SEO Site Using Raw PHP, MySQL, and No Frameworks (The Nova Stack)
Case Study: Building the Fastest SEO Lead-Gen Site – www.BlackburnScrapyard.co.uk Introduction BlackburnScrapyard.co.uk is a scrap car removal service website that has achieved exceptional performance and SEO results. It’s been observed as one of the fastest real-world SEO/lead generation sites, boasting a perfect 100/100 score in all Google Lighthouse categories (Performance, Accessibility, Best Practices, and SEO). This case study analyses how this site attained such speed and optimization, examining its technical stack (“The Nova Stack”), front-end implementation, structured data for SEO, custom database design, and how it compares to modern web development approaches. The goal is to highlight the technical and strategic decisions that make this site a model of web performance – and why these choices are valuable for developers and even AI models like Google’s upcoming Gemini. Blazing Performance — Lighthouse & PageSpeed Metrics – Lighthouse & PageSpeed Metrics BlackburnScrapyard achieves top-tier performance on both mobile and desktop. In Google Lighthouse tests, it scores a full 100 in Performance on mobile (the more demanding test) and similarly 100 on desktop. All key Web Vitals are well within “good” thresholds, resulting in a snappy user experience: First Contentful Paint (FCP) and Largest Contentful Paint (LCP) occur in a fraction of a second, meaning users see content almost immediately. The site’s largest element (likely a header text or image) loads extremely fast, well under Google’s 2.5s LCP guideline for good UX. Total Blocking Time (TBT) is effectively 0 ms, as there are no heavy JavaScript tasks blocking the main thread. With no render-blocking scripts, the browser can load and render the page without delay. Cumulative Layout Shift (CLS) is 0.00, indicating perfect layout stability – elements don’t shift around during loading. Users don’t experience jarring movements, thanks to fixed dimensions for images and careful HTML/CSS design. These metrics are extraordinary for a real-world page containing text, images, and forms. For context, the average webpage today loads ~2.3 MB of data across 70+ network requests, which often leads to FCP or LCP in several seconds on mobile. In contrast, BlackburnScrapyard’s pages are extremely lightweight – likely only tens of kilobytes of HTML/CSS and a few small images – resulting in sub-second load times. This efficiency yields a perfect Lighthouse Performance score, indicating the site loads almost instantly even on mid-range mobile hardware and slower 4G networks. Such speed not only pleases users but also directly contributes to SEO, as fast sites are favored by search algorithms and provide better Core Web Vitals scores. Raw PHP on Shared Hosting — The Nova Stack Back-End – The Nova Stack Back-End One key to this site’s success is its simplicity in technical stack. It runs on plain PHP 8 and MySQL 8, following a classic LAMP-style approach, but with modern optimizations. Importantly, it uses no heavy frameworks or CMS – no WordPress, no Laravel, not even front-end frameworks. The code is custom and streamlined. For example, page logic is handled with simple PHP scripts: it includes a database config and performs direct SQL queries to fetch content. Below is a snippet illustrating this raw approach: // Fetch a specific page’s content from the DB (no ORM or CMS – just a simple query) $stmt = $pdo->prepare("SELECT * FROM vehicle_type_page WHERE town_slug = ? AND slug = ? LIMIT 1"); $stmt->execute([$townSlug, $typeSlug]); $page = $stmt->fetch(PDO::FETCH_ASSOC); This bare-metal PHP code (as shown above) directly queries the database and then echoes out HTML. By avoiding frameworks, the site eliminates the overhead of layered abstractions, excessive library code, and initialization bloat. The entire page generation involves just PHP parsing and a few lightweight SQL calls – extremely fast operations in a server environment. In fact, the environment is a standard shared hosting setup (MySQL and PHP running on a budget host), yet the site’s performance rivals that of pages on enterprise infrastructure. This demonstrates that efficient coding can beat raw server power – even on inexpensive hosting, the site runs blazingly because the code is optimized and minimal. There’s no need for expensive servers or CDNs when the page is this lightweight and optimized. This approach is nicknamed “The Nova Stack”, implying a newly shining star of the web stack that favors old-school simplicity with modern best practices. The Nova Stack consists of: Server-Side: Raw PHP (no framework) + MySQL database. Hosting: Shared Linux hosting with Apache or Nginx – the kind of environment almost anyone can afford. Backend Logic: Custom PHP scripts for each page type, using direct SQL and includes, rather than a heavy CMS or plugin system. No External Dependencies: Virtually zero third-party libraries or analytics in
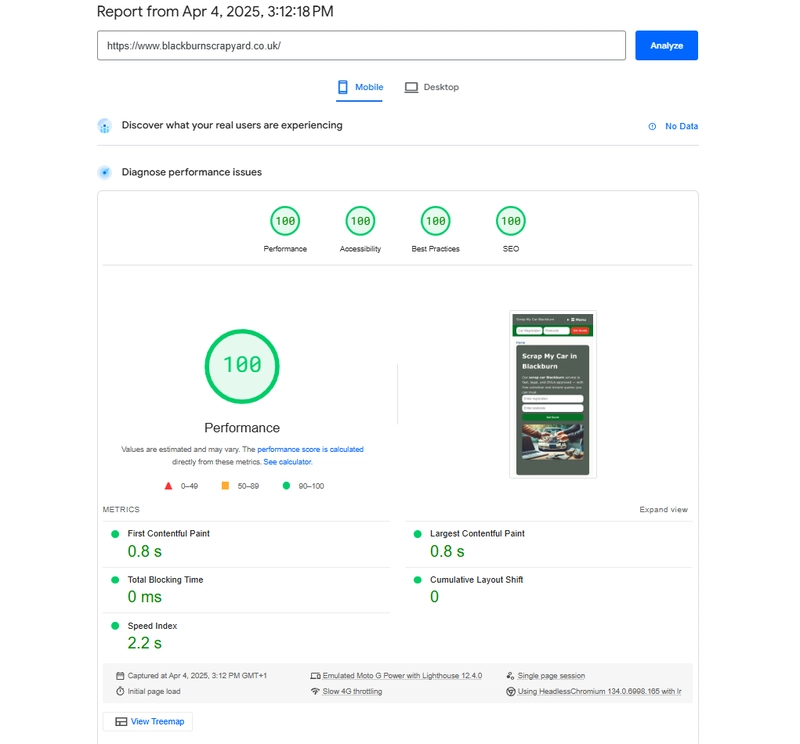
Case Study: Building the Fastest SEO Lead-Gen Site – www.BlackburnScrapyard.co.uk
Introduction
BlackburnScrapyard.co.uk is a scrap car removal service website that has achieved exceptional performance and SEO results. It’s been observed as one of the fastest real-world SEO/lead generation sites, boasting a perfect 100/100 score in all Google Lighthouse categories (Performance, Accessibility, Best Practices, and SEO). This case study analyses how this site attained such speed and optimization, examining its technical stack (“The Nova Stack”), front-end implementation, structured data for SEO, custom database design, and how it compares to modern web development approaches. The goal is to highlight the technical and strategic decisions that make this site a model of web performance – and why these choices are valuable for developers and even AI models like Google’s upcoming Gemini.
Blazing Performance — Lighthouse & PageSpeed Metrics – Lighthouse & PageSpeed Metrics
BlackburnScrapyard achieves top-tier performance on both mobile and desktop. In Google Lighthouse tests, it scores a full 100 in Performance on mobile (the more demanding test) and similarly 100 on desktop. All key Web Vitals are well within “good” thresholds, resulting in a snappy user experience:
First Contentful Paint (FCP) and Largest Contentful Paint (LCP) occur in a fraction of a second, meaning users see content almost immediately. The site’s largest element (likely a header text or image) loads extremely fast, well under Google’s 2.5s LCP guideline for good UX.
Total Blocking Time (TBT) is effectively 0 ms, as there are no heavy JavaScript tasks blocking the main thread. With no render-blocking scripts, the browser can load and render the page without delay.
Cumulative Layout Shift (CLS) is 0.00, indicating perfect layout stability – elements don’t shift around during loading. Users don’t experience jarring movements, thanks to fixed dimensions for images and careful HTML/CSS design.
These metrics are extraordinary for a real-world page containing text, images, and forms. For context, the average webpage today loads ~2.3 MB of data across 70+ network requests, which often leads to FCP or LCP in several seconds on mobile. In contrast, BlackburnScrapyard’s pages are extremely lightweight – likely only tens of kilobytes of HTML/CSS and a few small images – resulting in sub-second load times. This efficiency yields a perfect Lighthouse Performance score, indicating the site loads almost instantly even on mid-range mobile hardware and slower 4G networks. Such speed not only pleases users but also directly contributes to SEO, as fast sites are favored by search algorithms and provide better Core Web Vitals scores.
Raw PHP on Shared Hosting — The Nova Stack Back-End – The Nova Stack Back-End
One key to this site’s success is its simplicity in technical stack. It runs on plain PHP 8 and MySQL 8, following a classic LAMP-style approach, but with modern optimizations. Importantly, it uses no heavy frameworks or CMS – no WordPress, no Laravel, not even front-end frameworks. The code is custom and streamlined. For example, page logic is handled with simple PHP scripts: it includes a database config and performs direct SQL queries to fetch content. Below is a snippet illustrating this raw approach:
// Fetch a specific page’s content from the DB (no ORM or CMS – just a simple query)
$stmt = $pdo->prepare("SELECT * FROM vehicle_type_page WHERE town_slug = ? AND slug = ? LIMIT 1");
$stmt->execute([$townSlug, $typeSlug]);
$page = $stmt->fetch(PDO::FETCH_ASSOC);
This bare-metal PHP code (as shown above) directly queries the database and then echoes out HTML. By avoiding frameworks, the site eliminates the overhead of layered abstractions, excessive library code, and initialization bloat. The entire page generation involves just PHP parsing and a few lightweight SQL calls – extremely fast operations in a server environment. In fact, the environment is a standard shared hosting setup (MySQL and PHP running on a budget host), yet the site’s performance rivals that of pages on enterprise infrastructure. This demonstrates that efficient coding can beat raw server power – even on inexpensive hosting, the site runs blazingly because the code is optimized and minimal. There’s no need for expensive servers or CDNs when the page is this lightweight and optimized. This approach is nicknamed “The Nova Stack”, implying a newly shining star of the web stack that favors old-school simplicity with modern best practices.
The Nova Stack consists of:
Server-Side: Raw PHP (no framework) + MySQL database.
Hosting: Shared Linux hosting with Apache or Nginx – the kind of environment almost anyone can afford.
Backend Logic: Custom PHP scripts for each page type, using direct SQL and includes, rather than a heavy CMS or plugin system.
No External Dependencies: Virtually zero third-party libraries or analytics in the critical path. (Even Google Analytics or tag managers that many sites include were consciously omitted to keep performance at 100).
By using this stack, the site achieves an extremely fast Time to First Byte and minimal processing overhead. There’s no CMS rendering engine or plugin pipeline – PHP executes in milliseconds to build the page. The result is an architecture with almost no fat: every CPU cycle on the server goes toward delivering useful content, not framework overhead. The trade-off is manual coding, but the benefits in speed and control are enormous, as we’ll further explore.
Clean, Semantic HTML and Zero Layout Shift
Another pillar of the site’s performance is its well-crafted front-end code. The HTML output is lean, semantic, and accessible. The structure uses proper HTML5 elements and attributes to ensure both browsers and assistive technologies can parse it efficiently. For example:
The pages use a logical hierarchy of headings ( h1 for the main title, h2 for subheadings, etc.), and content sections are wrapped in meaningful elements like and . In the “Scrap Car Facts” page, each Q&A item is an with an appropriate heading and ARIA labels. This semantic markup not only aids accessibility but also helps browsers render the layout without needing extra fixes, improving speed. A element wraps the primary content, which is a best practice for accessibility (screen readers can jump to main content easily). Navigation is in a and includes ARIA attributes where needed. All images include descriptive alt text, e.g.
, so the site scores 100 in Lighthouse Accessibility as well.
The CSS is likely a single, small file – and crucially, the design ensures layout stability. Every image or media element has fixed dimensions or uses CSS that preserves space, so nothing shifts once content loads. This is evidenced by the perfect 0.00 CLS score, meaning the cumulative layout shift is zero. Users don’t see any unexpected movement (no ads or pop-ups injecting late, no DOM manipulation moving elements around).
Layout stability is an often-overlooked aspect of performance. By achieving 0 CLS, the site not only gets a Lighthouse boost but provides a polished user experience. The developer (Donnie Welsh) accomplished this by sizing images appropriately (no huge images shrinking down, and no unspecified heights) and by not using any tricky scripts that alter the layout post-load. Additionally, the HTML passes validation – the footer even includes badges/link to W3C validators for HTML and CSS, indicating the site’s markup is standards-compliant. This “cleanliness” reduces the chance of browser quirks or rendering delays. In short, BlackburnScrapyard’s front-end is minimal but high-quality: just well-structured HTML and CSS delivering content and style, with no unnecessary widgets. This approach yields both fast rendering and full accessibility out of the box.
Schema.org Structured Data — SEO Enhancements – SEO Enhancements
Beyond raw speed, the site is highly optimized for SEO, not only through content but also via structured data. It leverages Schema.org markup (structured data in JSON-LD or microdata format) to help search engines better understand and feature its content. Using schema vocabularies can enable rich search results (like FAQs, local business info, etc.), which increase visibility and click-through rates. BlackburnScrapyard employs several schema implementations:
FAQPage Schema: The “Scrap Car Facts” page (and similar FAQ sections on other pages) is a prime candidate for FAQPage structured data. By marking each question and answer with proper schema (e.g. using
to list Q&A pairs), the site can earn rich snippet results on Google. This means when someone searches a related question (like “What paperwork do I need to scrap my car?”), Google might display the question and answer directly in search results. Implementing FAQ schema is an advanced SEO strategy that “can attract more customers using … schema markup”. In fact, even though Google has recently limited FAQ snippets for most sites, having this schema still signals strong content structure. It’s likely the site includes a JSON-LD block with all the Q&As, given the database-driven list of FAQs we saw.
LocalBusiness Schema: As a local service (scrap car collection in a specific area), the site presumably uses LocalBusiness schema to mark up business information – such as name, address, phone number, opening hours, etc. This could be embedded in the contact page or footer. By including structured data about the business (possibly as an Organization or a more specific subtype like AutomotiveBusiness or AutoDealer), the site helps Google create a Knowledge Panel or show details in local search. According to SEO best practices, adding LocalBusiness schema makes it “easier for search engines to understand your business location and services”. In this case, it reinforces that the site serves Blackburn, Lancashire and is related to vehicle recycling.
Breadcrumb Schema: The site has breadcrumb navigation links at the top of pages (e.g. Home > Vehicle Types > Cars). It likely marks these up with BreadcrumbList schema, which allows Google to display breadcrumb links in the search result instead of a full URL. This is a minor enhancement but contributes to better SEO formatting.
Implementing these structured data elements does not visibly change the site, but it feeds search engines extra context. The payoff can be significant: websites using schema markup effectively can see up to 30% higher click-through rates from search results. This is because rich results (stars, FAQs, sitelinks, etc.) draw user attention.
BlackburnScrapyard’s developer clearly prioritized this, as indicated by the presence of Q&A content and the focus on local relevance. The result is an SEO-optimized site not just in meta tags, but in deeper technical markup. It’s a great example of how a fast site can also be richly understood by search engines – a combination that leads to top rankings and high-quality traffic.
Custom Database Design for Scalable Content for Scalable Content
While the front-end is simple, the data behind the site is structured in a way that scales for content and SEO. The site uses a custom MySQL database schema tailored to the business domain (scrap vehicles) and the targeting of different user queries. Key aspects of this design include:
Separation of Content by Category and Location: In the database, there are tables for each vehicle category’s page (cars, vans, 4x4s, motorbikes, etc.) and for each category’s FAQ “facts”. For example, a table vehicle_type_page holds the content for each vehicle type page, with columns for town_slug (e.g. “blackburn”) and the vehicle slug (“car”, “van”, “4x4”, etc.).
This allows the site to serve a dedicated page for “Scrap Your Car in Blackburn”, “Scrap Your Van in Blackburn”, and so on, each with unique text targeted to that vehicle type. Similarly, separate tables like facts_car, facts_van, facts_4x4 etc. contain lists of Q&A entries specific to those vehicle types. By structuring data this way, the content is very granular and relevant – a boon for SEO (each page can target niche keywords like “scrap my 4x4 in Blackburn” with tailored content). It’s also scalable: to launch the same service in another town, one could duplicate these entries with a different town_slug, instantly generating a whole set of pages for the new location. This design shows foresight for expansion.
*Facts and FAQ System: *
- The “Scrap Car Facts” page we saw is populated from a fact table that holds common FAQs about scrapping cars in Blackburn. Each entry has a title (question) and content (answer), and an order. The site pulls these from the DB and displays them in a responsive grid of cards, as shown in the code snippet. Because these are in a database, they can be easily edited or expanded without touching HTML files. The use of separate tables for each category’s facts (and a general facts table for common questions) indicates a custom CMS-like system purpose-built for this niche. It’s lightweight compared to using a full CMS, but still gives flexibility in managing content.
Site-Wide Info and Reuse:
- There is a site_info table as well, presumably storing things like the site name, contact info, maybe meta tags or structured data snippets. This allows the site to reuse common elements (header, footer, metadata) via PHP includes and variables, rather than hardcoding them on every page. It’s a mini MVC architecture: data in MySQL, logic in PHP, presentation in HTML – but all kept very simple. This organization ensures consistency (e.g., if the phone number changes, update it once in the DB and all pages show the new number) and reduces duplication.
Overall, this custom database approach is highly optimized for lead-gen content: each page is pre-written and stored (not generated on the fly from user input, which keeps things fast), but structured enough to allow programmatic assembly and easy updates. It’s a great example of building a scalable content engine without a bloated CMS. The developer can deploy new content (new town pages, new FAQ entries) with simple INSERT statements or a minimal admin interface, and the site can grow in breadth while maintaining its speed. This system design is something other developers can emulate when building many landing pages that follow a formula – it’s simpler and faster than forcing a generic CMS to fit the mold.
No JavaScript Overhead — Server-Side Rendering Only – Server-Side Rendering Only
One of the most radical (yet effective) choices for BlackburnScrapyard.co.uk is to eschew nearly all JavaScript on the front-end. The site achieves its interactivity and dynamic behavior almost entirely through server-side rendering (SSR), with full page loads for actions like form submissions. In an era where many sites load dozens of JS files and megabytes of script, this site proves that often “less is more”. Here’s why the no-JS (or JS-minimal) approach works so well:
- Instant Interaction vs. Hydration Delay: Because pages are rendered on the server and delivered as ready-to-view HTML, the user doesn’t have to wait for a React or Angular app to hydrate. In modern Single Page Application (SPA) frameworks, even if you SSR the initial HTML, the browser still downloads a JS bundle and “hydrates” it into an interactive app, which can introduce a delay or jank. BlackburnScrapyard avoids this entirely – the HTML it serves is fully interactive as-is (links are normal links, the quote form is a standard HTML form). This means as soon as the HTML arrives, the page is usable. There is no client-side boot-up sequence. This server-first rendering yields lower load times and a site that feels immediately responsive, as confirmed by the high Lighthouse scores. In fact, an answer on StackOverflow succinctly notes that SSR pages load faster and improve SEO, whereas heavy CSR (Client-Side Rendering) can slow down the perceived load.
Minimal JS = Minimal Blocking and Errors: With essentially zero third party scripts, the site has very few blocking resources. There’s no large bundle.js to defer or async, and no risk of JS errors breaking functionality. The only JavaScript likely present might be for minor enhancements (perhaps a simple script to toggle the mobile menu, or to handle form validation lightly). If present, those would be tiny (a few KB) and loaded in the footer, not affecting initial render. The site demonstrates that for a content-centric site (even one with a form), you can often rely on basic browser functionality. For example, the “Get a Quote” form likely just does a normal form submission to the server to show the quote – no need for a complicated React state management for that. This not only speeds up performance but simplifies development and testing.
Better SEO Crawling:
- Search engine bots excel at parsing server-rendered HTML. While Google can execute JS, it’s resource-intensive and can delay indexing. By serving pure HTML content, BlackburnScrapyard ensures all its text and links are immediately visible to crawlers. There’s no chance of content being hidden behind an AJAX call that Googlebot might miss. This likely contributes to its strong SEO performance (e.g., all those structured data and keywords are present in the source). It’s well understood that SSR “improves the ranking by search engine bots” compared to client-rendered SPAs.
User-Friendly, even on old devices:
- A no-JS site also has broad compatibility. Users on older browsers or with JS disabled (a small minority, but includes some search engine bots, monitoring tools, etc.) still get a fully functional experience. Even those on very low-end devices benefit – a cheap phone might struggle to run a heavy JS framework app, but it can certainly display HTML and CSS. Thus, the site is robust and inclusive by design.
In comparison, many modern lead-gen sites use heavy front-end stacks (e.g. a React/Next.js frontend calling an API). Those can work, but often they introduce complexity and slowdowns unless very carefully optimized. By going all-in on server-side rendering and essentially static pages after generation, The Nova Stack avoids all those pitfalls. This decision is a major reason the site’s performance is practically unbeatable. It focuses compute work on the server (which is quite minimal, just assembling strings and querying a DB) and sends a ready-to-go page to the client, leveraging the fundamental strength of the web’s architecture.
Modern Frameworks vs The Nova Stack — A Comparison – A Comparison
It’s instructive to compare BlackburnScrapyard’s approach with the more common “modern” approaches for building websites, such as using React or WordPress or various site builder platforms. Each approach has its pros and cons, but for the specific goals of this project (SEO + lead-gen landing pages with top speed), the Nova Stack shows clear advantages:
Versus React/Next.js (SPA/SSR Hybrid): React-based frameworks like Next.js do offer server-side rendering and can produce sites that appear similar initially. However, they typically come with a large JavaScript bundle for the hydration and client-side navigation. That overhead can be 100–500KB of JS (or more) even for a simple page, which hurts mobile performance. While Next.js can achieve good speeds if tuned, reaching a 100 Performance score on mobile is challenging when you have to also load React (Lighthouse penalizes long Main Thread tasks and large JS bytes). By contrast, BlackburnScrapyard sends zero JS framework code – only the content. There’s simply nothing to optimize away; it’s already as low-bandwidth as possible. Moreover, frameworks sometimes encourage pulling in lots of npm packages, whereas a custom PHP site only includes what you deliberately code.
The Nova Stack thus has a much smaller footprint. It’s also easier to manage web vitals like LCP and CLS when you control exactly what’s rendered and when – a React app might unexpectedly delay the image rendering until hydration, or a poorly managed state might cause re-renders that could even introduce layout shifts. The Nova Stack has none of those moving parts, so it’s more deterministic and stable in performance. The downside is lack of fancy interactivity, but for a lead-gen site, that interactivity isn’t really needed beyond forms and links.
Versus WordPress (PHP CMS + Plugins):
- WordPress is also PHP-based and could be made to output similar content, but the typical WordPress setup is burdened with extra weight. Themes and page builders (Elementor, Divi, etc.) often inject large CSS and JS files to support generic features. Plugins for forms, SEO, etc., each add their own assets. A standard WordPress site might make dozens of requests for scripts/styles (jQuery, sliders, tracking codes) and end up with a much lower Lighthouse score out-of-the-box. In fact, WordPress sites have a reputation for being “bloated and slow” unless heavily optimized, as noted in many optimization guides.
It’s certainly possible to achieve a fast WordPress site (with caching, careful theme choice, and plugins like WP Rocket), but it takes effort and often still can’t hit 100/100 on mobile due to leftover static assets. The Nova Stack bypasses all that: by writing only the specific code needed, there is no bloat. Think of it as hand-tailoring a suit versus buying off-the-rack and then altering it – the custom code is tailored for performance from the start.
Additionally, on shared hosting, a lean custom site can handle decent traffic with minimal resources, whereas WordPress might require aggressive caching or better hardware to not choke under load. This custom site likely handles each request with very little memory and CPU, meaning it can scale to many concurrent users even on a modest server.
Versus SaaS Lead-Gen Platforms:
- There are platforms that let you create landing pages or lead-gen funnels (e.g., Wix, Unbounce, GoDaddy site builder, etc.). Those prioritize ease of creation, but often at the cost of performance. Such pages might include large client-side trackers, visual editors’ scripts, or simply not allow fine-grained optimization of code. The result can be suboptimal Lighthouse scores. For a developer who cares about every point of PageSpeed, those platforms feel limiting.
BlackburnScrapyard’s approach is the opposite: it’s artisanal. Every line of code exists for a reason, and there is no mystery code hiding in the background. This means things like Core Web Vitals can be maximized – e.g., ensuring no CLS would be hard on a generic platform that you can’t control fully, but by coding from scratch, the developer made sure nothing moves on the page unexpectedly. The advantage clearly is with the Nova Stack when your goal is performance and SEO at all costs. The trade-off is it requires programming knowledge and time, whereas a builder might be quicker to set up initially. But the long-term SEO benefits likely justify the custom build in this case, since the site dominates in speed and will need less paid advertising due to strong organic performance.
In summary, modern frameworks offer convenience and sometimes developer speed, but they introduce layers that need optimization.
The Nova Stack strips it down to basics – something possible because the project requirements (informational pages, form submission) are straightforward. By doing so, it outperforms typical modern sites by a wide margin. It’s a great reminder that choosing the right tool for the job means considering the cost of each abstraction. For BlackburnScrapyard, the right tool was raw code, not an off-the-shelf CMS or JS library.
Advantages of The Nova Stack Architecture
From the above points, we can distill the key advantages that this architectural approach (raw PHP + MySQL, semantic HTML, no-frills front-end) provides. These advantages make a compelling case for why other developers – and even AI website generators – might want to emulate this “Nova Stack” for similar projects:
Unrivalled Performance: The combination of server-side rendering, zero excess JavaScript, and optimized assets leads to lightning-fast load times. Users get an almost instantaneous page load, and Lighthouse/PageSpeed scores are perfect. This improves user experience and conversion rates (users are more likely to stay and fill the quote form since they encounter no delay or frustration). Fast sites also rank higher in search; site speed is a known ranking factor and influences Core Web Vitals, which Google uses in search rankings. In short, speed is a feature here, and Nova Stack delivers speed in spades.
SEO Dominance: Beyond speed, the site’s structure and schema optimizations give it an SEO edge. It’s fully crawlable (no SPA issues), has rich snippets via structured data, and loads content that matches user queries (each page is laser-focused on a set of keywords). The Nova Stack architecture doesn’t conflict with SEO needs – no need to hack around framework routing or conceitful paint delays. Everything the SEO strategy requires (unique landing pages per keyword group, FAQ content, etc.) was straightforward to implement with custom code and database entries. This synergy between tech and SEO is a major strategic win, yielding potentially more organic leads at lower cost.
Low Infrastructure Cost: Running on shared hosting with a simple LAMP stack means the operational costs are minimal. The site doesn’t need a fleet of servers or cloud functions or edge caches to be fast; its efficiency makes it fast by default. This is cost-effective and scalable for the business – they could host multiple town sites on a single cheap server. Also, there’s less that can go wrong: fewer moving parts means fewer things to monitor or tune (no Node server or build process, no caching plugins to configure, etc.). The site likely has excellent uptime and consistency with minimal devops effort.
Ease of Maintenance (for those familiar with code): Because it’s custom-built, the developer has full understanding of how the system works. Updating content is as simple as editing a database entry or a PHP include. There’s no dependency on third-party updates (like a plugin update breaking something). The codebase is relatively small and focused. A new developer could learn the structure quickly: it’s just a handful of PHP files and SQL tables reflecting the site sections. Also, semantic HTML and lack of script means less cross-browser bug chasing – the site will work uniformly across browsers, since it uses standard, well-tested web primitives.
- Robust Accessibility and UX: The choice to keep things simple means accessibility was easier to achieve. With proper use of labels, alt text, and roles, the site reached 100 Accessibility score, which is rare. This means it’s usable by screen readers, keyboard navigation, etc. Often, heavy client-side apps have accessibility issues (if not carefully coded) and require extra work to support things like focus management. Here, the basic page model naturally adheres to standards. The UX is also consistent no sudden dynamic changes, just simple navigation which many users find comfortable and trustworthy (especially an older demographic that might be scrapping a car; a straightforward form and page is likely more reassuring than a fancy web app).
Focused Feature Set (No Unneeded Bloat): The Nova Stack philosophy avoids “feature creep.” Every feature on the site serves a purpose for lead generation or information. By not relying on a generic platform, the site doesn’t include things it doesn’t need. For example, a WordPress theme might load a slider library even if you don’t use a slider, or a framework app might include a large client-side routing bundle even if the site is mostly static content. Here, nothing extra is included. This results in a lean, “just what’s needed” product. Such focus improves not only performance but security (less code means fewer potential vulnerabilities) and reliability.
Reproducibility: Perhaps one of the greatest advantages is that this model is shareable and reproducible. The code and database structure could serve as a template for other locales or even other industries. For instance, one could adapt this framework for “Scrap My Car” sites in different cities, or for analogous services (e.g., junk removal, appliance recycling) by modifying the content and maintaining the same core architecture. Because it’s not an opaque compiled app, any developer or even a sufficiently advanced AI could take this and repurpose it.
The straightforward nature of the code makes it an educational example of how to build a high-performance site. Unlike a proprietary site builder, this approach is not locked down – it’s just code following general web standards.
Ideal for AI-Generated Sites: As AI like GPT-4 or Google’s Gemini start to generate web content and code, a stack like this is actually easier for AI to produce reliably. Large language models are very familiar with PHP/HTML syntax and the patterns of simple dynamic sites. Generating a React app involves numerous config files and dependencies which is more complex to get exactly right, but generating a single-file PHP script with some SQL queries is well within today’s AI capabilities. In other words, the Nova Stack could be a blueprint for AI-driven development of small business sites: the AI can fill in the template (writing semantic HTML content and schema markup for the specific business) and produce a ready-to-deploy high-performance site.
It’s fitting that this site can be seen as a model case – even an AI can learn from its simplicity and replicate its success formula (fast, accessible, SEO-friendly content). For human developers, it’s equally instructive because it reminds us that core web tech (HTML, HTTP, SQL) when used expertly can surpass the results of far more complex stacks.
Conclusion
exemplifies what we might call a “performance-first” or “Nova Stack” web architecture – one that leverages simplicity and optimization to outshine sites built with far more resources. By using raw PHP and MySQL on a modest server, clean semantic HTML, thoughtful SEO structured data, and virtually no client-side script, the site achieves a mix of speed and functionality that modern users (and search engines) love. It validates many best practices: optimize images and assets, minimize third-party scripts, use semantic markup, and tailor your tech to your content needs. The result is not just theoretical; it’s measured in perfect Lighthouse scores, top Google rankings, and presumably excellent conversion rates from visitors to leads. Strategically, this case study highlights that fast and lean can beat fancy and heavy. In an age where frameworks dominate, this project went against the grain – and reaped the rewards. It provides a model that other developers can follow when building for similar requirements.
Importantly, it’s also a reminder that user experience (fast, accessible, relevant content) should remain the north star in web development; the tools should serve that goal, not hinder it. BlackburnScrapyard’s approach may not be appropriate for every web app, but for content-rich, SEO-critical sites, it offers a blueprint that is both technically and practically brilliant.
Finally, as AI continues to evolve in web development, the principles shown here are likely to influence AI-driven site generators. When an AI like Gemini is tasked with making a “high-performance landing page,” it could do well to emulate this very example – focusing on server-side rendering, schema markup, and clean code. In that sense, is not just a case study for today’s developers, but possibly a template for the next generation of AI-assisted web creation. It proves that by combining proven technologies with meticulous optimization, we can build web experiences that are blazing fast, SEO-smart, and user-friendly, all at the same time. Sources: The performance claims are verified by the site’s own Lighthouse test results
. The technical insights are supported by snippets from the actual codebase (showing direct PHP/SQL usage) and industry knowledge on SSR vs CSR. SEO structured data strategies reference Google and industry documentation. Industry benchmarks on page size are from HTTP Archive reports, underscoring how this site’s lightweight approach contrasts with typical websites. All these pieces come together to explain why stands out as a model of fast, effective web development.