How can the C language program achieve high readability, easy maintainability and extensibility
I. Top-Down Design Overall Planning Starting from the overall function of the program, define the main module (such as the main() function) first, and clarify the overall goal and input/output of the program. For example, when designing a student management system, first determine the top-level functional modules such as "data entry", "query", and "statistics" that need to be implemented. Step-by-step decomposition Break down complex problems layer by layer into sub-problems to form a tree-like structure. For example, "data entry" can be further divided into sub-modules such as "input student information", "validate data format", and "store to file". Each sub-module is further decomposed until each task is simple enough (typically corresponding to a function within 50 lines). 。 Hierarchical design The hierarchical structure is reflected through the function call relationship. The top-level module calls the lower-level module, and the lower-level module does not depend on the upper-level module in the reverse direction. II. Structured Programming Control Structure Restrictions Only use the three basic structures: sequence, selection (if/switch), and loop (for/while). Avoid using the goto statement. For example, use a for loop to replace complex jump logic. \ \ Single entry, single exit Each function or code block has only one entry and one exit to ensure clear logic. 。 For example, in a function, avoid multiple return statements and uniformly return the result at the end. \ \ Code block normalization Clarify the boundaries of code blocks through indentation, alignment of parentheses, etc., to enhance readability. For example: if (condition) { // Code block } else { // Code block } III. Modular Programming \ Functional Module Division Divide the program into independent functional units, with each module corresponding to a function or a group of related functions. For example: The main module: main() is responsible for process control. Functional modules: such as input_data(), calculate_score(), etc. Tool modules: such as file_io.c for handling file operations. Separation of interface and implementation. Header file (.h): Declare function prototypes, data structures, and define interfaces. For example: // file_io.h int read_file(const char* filename); Source (.c) : Implements the specific functionality, hiding the internal details // file_io.c #include "file_io.h" int read_file(const char* filename) { /* Implementation */ } Module independence principle High cohesion: Functions within modules are closely related (e.g., all mathematical calculations are centralized in math_utils.c) Low coupling: Modules pass data through parameters, avoiding global variables . For example, using function arguments instead of external variables to pass the result of a computation. Module integration and testing The main module is integrated and debugged step by step by including header files to call each sub-module . For example: #include "file_io.h" #include "data_process.h" int main() { read_file("data.txt"); process_data(); return 0; } Ⅳ.Practical Examples Take the "Student Performance Statistics Procedure" as an example: Top-down design: the main function calls input_scores(), calculate_avg(), and output_result(). Structured encoding: Only three control structures are used inside each function, such as a for loop over an array of grades. Modular implementation: io_module.h/c: Handles input and output calc_module.h/c: Implements statistical logic The main program integrates modules through header files . V. Precautions Code size control: A single function is recommended to be no more than 50 lines, and complex logic is split into subfunctions . Naming conventions: Function names should be explicit (e.g., validate_input(), not func1()). Documentation comments: The header should state the module functionality and interface parameters . Through the above methods, the C language program can achieve high readability, easy maintainability and extensibility, which is in line with the core goal of structured programming .
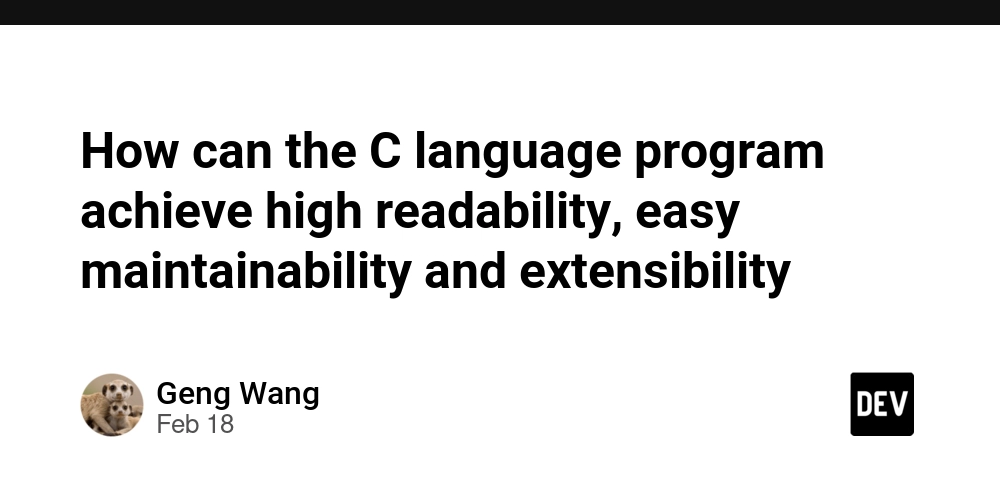
I. Top-Down Design
Overall Planning
Starting from the overall function of the program, define the main module (such as the main() function) first, and clarify the overall goal and input/output of the program. For example, when designing a student management system, first determine the top-level functional modules such as "data entry", "query", and "statistics" that need to be implemented.
Step-by-step decomposition
Break down complex problems layer by layer into sub-problems to form a tree-like structure. For example, "data entry" can be further divided into sub-modules such as "input student information", "validate data format", and "store to file". Each sub-module is further decomposed until each task is simple enough (typically corresponding to a function within 50 lines). 。
Hierarchical design
The hierarchical structure is reflected through the function call relationship. The top-level module calls the lower-level module, and the lower-level module does not depend on the upper-level module in the reverse direction.
II. Structured Programming
Control Structure Restrictions
Only use the three basic structures: sequence, selection (if/switch), and loop (for/while). Avoid using the goto statement. For example, use a for loop to replace complex jump logic. \
\
Single entry, single exit
Each function or code block has only one entry and one exit to ensure clear logic. 。 For example, in a function, avoid multiple return statements and uniformly return the result at the end. \
\
Code block normalization
Clarify the boundaries of code blocks through indentation, alignment of parentheses, etc., to enhance readability. For example:
if (condition) {
// Code block
} else {
// Code block
}
III. Modular Programming
\
Functional Module Division
Divide the program into independent functional units, with each module corresponding to a function or a group of related functions. For example:
- The main module: main() is responsible for process control.
- Functional modules: such as input_data(), calculate_score(), etc.
- Tool modules: such as file_io.c for handling file operations.
Separation of interface and implementation.
- Header file (.h): Declare function prototypes, data structures, and define interfaces. For example:
// file_io.h
int read_file(const char* filename);
- Source (.c) : Implements the specific functionality, hiding the internal details
// file_io.c
#include "file_io.h"
int read_file(const char* filename) { /* Implementation */ }
Module independence principle
- High cohesion: Functions within modules are closely related (e.g., all mathematical calculations are centralized in math_utils.c)
- Low coupling: Modules pass data through parameters, avoiding global variables . For example, using function arguments instead of external variables to pass the result of a computation.
Module integration and testing
The main module is integrated and debugged step by step by including header files to call each sub-module . For example:
#include "file_io.h"
#include "data_process.h"
int main() {
read_file("data.txt");
process_data();
return 0;
}
Ⅳ.Practical Examples
Take the "Student Performance Statistics Procedure" as an example:
- Top-down design: the main function calls input_scores(), calculate_avg(), and output_result().
- Structured encoding: Only three control structures are used inside each function, such as a for loop over an array of grades.
- Modular implementation: io_module.h/c: Handles input and output calc_module.h/c: Implements statistical logic The main program integrates modules through header files .
V. Precautions
- Code size control: A single function is recommended to be no more than 50 lines, and complex logic is split into subfunctions .
- Naming conventions: Function names should be explicit (e.g., validate_input(), not func1()).
- Documentation comments: The header should state the module functionality and interface parameters .
Through the above methods, the C language program can achieve high readability, easy maintainability and extensibility, which is in line with the core goal of structured programming .