Harnessing Warp Terminal: Integrating AI and IDE-like Features into Your Workflow
Harnessing Warp Terminal: Integrating AI and IDE-like Features into Your Workflow Introduction In the rapidly evolving landscape of software development, developers constantly seek tools that streamline their workflows, optimize productivity, and minimize cognitive load. Enter Warp—a terminal designed from the ground-up to integrate advanced functionalities, including AI-powered assistance and IDE-like features. This article comprehensively explores Warp, its historical context, technical details, advantages, alternative comparisons, and practical integration into real-world applications. Historical Context Terminal emulators have long been a staple of software development, evolving from the simple command-line interfaces (CLIs) of the early computing era to the sophisticated tools we use today. Traditionally, terminals were text-based, strictly utilitarian in nature, serving mainly as interfaces for command execution. However, the rise of integrated development environments (IDEs) introduced rich features such as code completion, refactoring tools, and integrated debugging. In 2021, with the emergence of Warp, a paradigm shift began as it sought to combine the efficiency of CLI with the usability of IDEs—intertwining traditional terminal functionalities with modern AI capabilities. Warp utilizes Rust for backend performance and employs Web technology for its user interface, focusing on collaborative usability and enhanced developer experience. Warp's Unique Philosophy: Warp emphasizes the concept of command as a first-class citizen, meaning commands are not merely text inputs but fleshed-out entities With a layer of AI performing contextual interpretations. Technical Overview Architecture Warp operates on a client-server architecture, built with Rust and React. The backend uses Rust for its performance and memory management capabilities while the frontend leverages modern JavaScript frameworks for flexibility in rendering the UI. Core Features AI Power: Warp integrates OpenAI models to assist with command generation, error messages, and even code snippets. Workspace Environment: Features like multi-pane interface, commands history, and stateful sessions provide an IDE-like experience. Collaboration Tools: Sharing command outputs or sessions in real-time enhances collaborative workflows. Exploring Warp's Commands Commands in Warp are more than strings—they encapsulate state and metadata. Here's a deeper breakdown of its command structure: // Sample Warp Command const listFilesCommand = { command: 'ls', arguments: ['-la', '/path/to/directory'], options: {time: 'real', size: 'human-readable'}, onCompletion: (result) => { console.log('Command completed with output:', result); }, }; In this snippet, we can observe how each command can be enriched metadata, enabling enhanced representations in the UI and more powerful completion mechanisms. Code Example: Integrating with Node.js Below is an example demonstrating how one can harness Warp to interact with a Node.js application, providing a seamless interface for file operations: // Importing necessary modules const fs = require('fs'); const { exec } = require('child_process'); // Custom command to read a file const readFileCommand = { command: 'cat', arguments: ['example.txt'], options: { onError: (err) => console.error(err.message) }, onCompletion: (output) => { const jsonData = JSON.parse(output); console.log('Read Data:', jsonData); }, }; // Execution function function executeCommand(command) { exec(`${command.command} ${command.arguments.join(' ')}`, (error, stdout, stderr) => { if (error) command.options.onError(error); else command.onCompletion(stdout); }); } executeCommand(readFileCommand); // Output will be the content of 'example.txt' In this case, exec runs the shell command while managing errors effectively, showcasing error handling in a robust manner. Real-World Use Cases Industry Applications Software Development: Development teams leverage Warp to write, test, and debug code. Its AI capabilities assist in autocompletion and error hinting, reducing the need for flipping between various files. Data Analysis: Analysts use Warp to execute shell scripts for data processing and visualizing results in an IDE-like environment without left the terminal. System Administration: Administrators utilize Warp to manage server configurations with real-time collaboration features, allowing multiple team members to operate under the same terminal session. Edge Case Handling in Warp When working with Warp, potential pitfalls arise: Network Dependencies: Commands requiring network access could fail if the terminal loses connectivity. Implement robust state management or retries. Complex Command Structures: If a command depends on extensive arguments or nested comm
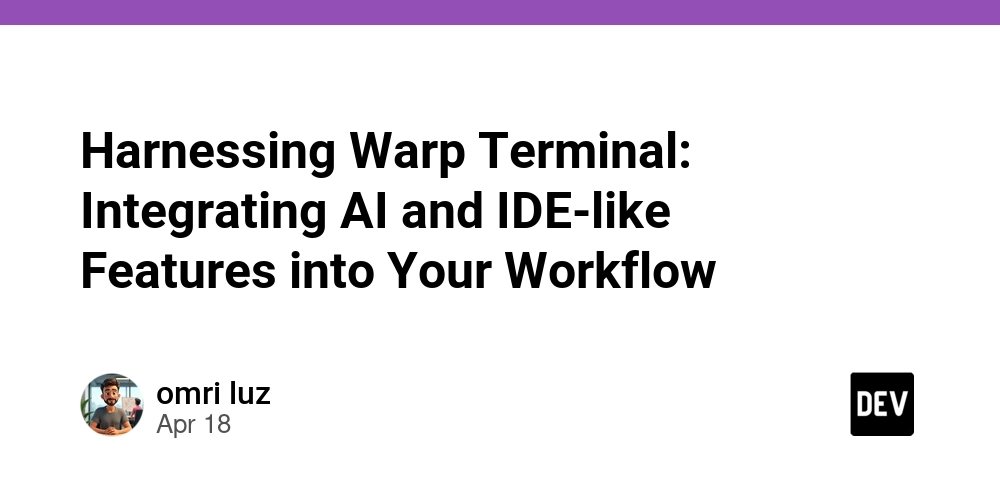
Harnessing Warp Terminal: Integrating AI and IDE-like Features into Your Workflow
Introduction
In the rapidly evolving landscape of software development, developers constantly seek tools that streamline their workflows, optimize productivity, and minimize cognitive load. Enter Warp—a terminal designed from the ground-up to integrate advanced functionalities, including AI-powered assistance and IDE-like features. This article comprehensively explores Warp, its historical context, technical details, advantages, alternative comparisons, and practical integration into real-world applications.
Historical Context
Terminal emulators have long been a staple of software development, evolving from the simple command-line interfaces (CLIs) of the early computing era to the sophisticated tools we use today. Traditionally, terminals were text-based, strictly utilitarian in nature, serving mainly as interfaces for command execution. However, the rise of integrated development environments (IDEs) introduced rich features such as code completion, refactoring tools, and integrated debugging.
In 2021, with the emergence of Warp, a paradigm shift began as it sought to combine the efficiency of CLI with the usability of IDEs—intertwining traditional terminal functionalities with modern AI capabilities. Warp utilizes Rust for backend performance and employs Web technology for its user interface, focusing on collaborative usability and enhanced developer experience.
- Warp's Unique Philosophy: Warp emphasizes the concept of command as a first-class citizen, meaning commands are not merely text inputs but fleshed-out entities With a layer of AI performing contextual interpretations.
Technical Overview
Architecture
Warp operates on a client-server architecture, built with Rust and React. The backend uses Rust for its performance and memory management capabilities while the frontend leverages modern JavaScript frameworks for flexibility in rendering the UI.
Core Features
- AI Power: Warp integrates OpenAI models to assist with command generation, error messages, and even code snippets.
- Workspace Environment: Features like multi-pane interface, commands history, and stateful sessions provide an IDE-like experience.
- Collaboration Tools: Sharing command outputs or sessions in real-time enhances collaborative workflows.
Exploring Warp's Commands
Commands in Warp are more than strings—they encapsulate state and metadata. Here's a deeper breakdown of its command structure:
// Sample Warp Command
const listFilesCommand = {
command: 'ls',
arguments: ['-la', '/path/to/directory'],
options: {time: 'real', size: 'human-readable'},
onCompletion: (result) => {
console.log('Command completed with output:', result);
},
};
In this snippet, we can observe how each command can be enriched metadata, enabling enhanced representations in the UI and more powerful completion mechanisms.
Code Example: Integrating with Node.js
Below is an example demonstrating how one can harness Warp to interact with a Node.js application, providing a seamless interface for file operations:
// Importing necessary modules
const fs = require('fs');
const { exec } = require('child_process');
// Custom command to read a file
const readFileCommand = {
command: 'cat',
arguments: ['example.txt'],
options: { onError: (err) => console.error(err.message) },
onCompletion: (output) => {
const jsonData = JSON.parse(output);
console.log('Read Data:', jsonData);
},
};
// Execution function
function executeCommand(command) {
exec(`${command.command} ${command.arguments.join(' ')}`, (error, stdout, stderr) => {
if (error) command.options.onError(error);
else command.onCompletion(stdout);
});
}
executeCommand(readFileCommand); // Output will be the content of 'example.txt'
In this case, exec
runs the shell command while managing errors effectively, showcasing error handling in a robust manner.
Real-World Use Cases
Industry Applications
Software Development: Development teams leverage Warp to write, test, and debug code. Its AI capabilities assist in autocompletion and error hinting, reducing the need for flipping between various files.
Data Analysis: Analysts use Warp to execute shell scripts for data processing and visualizing results in an IDE-like environment without left the terminal.
System Administration: Administrators utilize Warp to manage server configurations with real-time collaboration features, allowing multiple team members to operate under the same terminal session.
Edge Case Handling in Warp
When working with Warp, potential pitfalls arise:
- Network Dependencies: Commands requiring network access could fail if the terminal loses connectivity. Implement robust state management or retries.
- Complex Command Structures: If a command depends on extensive arguments or nested commands, clarity in user commands is essential to prevent errors.
Advanced Debugging Techniques
Debugging Features
Warp provides built-in logging and monitoring tools that can be vital when debugging complex workflows:
- Event Logging: Log all executed commands and responses to trace issues.
- UI Visual Feedback: The command interface provides real-time feedback; errors often highlight troublesome commands.
Performance Considerations
Warp is engineered for performance:
- Optimized Context Switching: By maintaining state between commands, Warp reduces the overhead associated with context switching.
- Resource Management: Employing Rust minimizes memory leaks, keeping the terminal responsive under high demand.
Optimization Strategies
- Batch Commands: Grouping commands into a single execution can reduce context switching and save on execution overhead.
- Utilizing Built-in Aliases: Warp supports custom aliases, which can streamline commonly used commands into quicker executions, enhancing efficiency.
Alternative Approaches
Comparing with Traditional Terminals
Advanced Features
While traditional terminals like GNOME Terminal or iTerm2 provide basic functionalities, they lack integrated AI capabilities. In contrast, Warp’s smart command completion and collaborative features set it apart.
Performance
Compared with traditional terminals, Warp’s Rust backend heralds faster execution and lower memory usage. However, traditional terminals might boast broader community support and plugin ecosystems.
Conclusion
Warp is redefining our approach to terminal use by integrating AI and IDE features into a singular cohesive environment beneficial to various domains in software development. As this tool continues to evolve, its focus on enhancing developer workflows will further revolutionize how we interact with code at the terminal level.
References
By leveraging Warp's powerful features, developers can significantly enhance their workflows, marry the command line's power with the comforts of an IDE, and foster collaboration in real-time. As Warp continues to advance, its potential impact on the software development landscape is poised to be profound.