Go Event Bus Best Practices: Implementing Loose Coupling with NSQite
Event Bus Best Practices: Implementing Loose Coupling with NSQite What is Event Bus? Event Bus is a messaging pattern that allows different components of an application to communicate through a publish/subscribe mechanism without direct dependencies. This pattern is particularly suitable for implementing loosely coupled architectural designs. Why Do We Need Event Bus? Decoupling: Components communicate through events without direct dependencies Extensibility: New features can be easily added by subscribing to existing events Maintainability: Code becomes easier to understand and maintain Asynchronous Processing: Supports asynchronous message handling, improving system responsiveness Best Practice Case: User Registration Notification System Background Let's assume we're developing an e-commerce system where new user registration requires: Sending a welcome email Creating a user points account Pushing system notifications Recording user behavior logs Traditional implementation would result in tight coupling between the registration service and these features, making the code difficult to maintain. NSQite Solution // Define event structure type UserRegisteredEvent struct { UserID string Username string Email string Time time.Time } // Email service handler type EmailHandler struct{} func (h *EmailHandler) HandleMessage(message *EventMessage[UserRegisteredEvent]) error { event := message.Body // Send welcome email return sendWelcomeEmail(event.Email) } // Points service handler type PointsHandler struct{} func (h *PointsHandler) HandleMessage(message *EventMessage[UserRegisteredEvent]) error { event := message.Body // Create user points account return createUserPoints(event.UserID) } // Registration service const topic = "user.registered" var publisher = NewPublisher[UserRegisteredEvent]() func RegisterUser(username, email string) error { // Create user userID := createUser(username, email) // Publish user registration event event := UserRegisteredEvent{ UserID: userID, Username: username, Email: email, Time: time.Now(), } return publisher.Publish(topic, event) } // Main program func main() { // Initialize email subscriber const emailChannel = "email" emailSub := NewSubscriber(topic, emailChannel) emailSub.AddConcurrentHandlers(&EmailHandler{}, 2) // Initialize points subscriber const pointsChannel = "points" pointsSub := NewSubscriber(topic, pointsChannel) pointsSub.AddConcurrentHandlers(&PointsHandler{}, 2) // Register new user RegisterUser("testuser", "test@example.com") } Code Explanation We define a user.registered Topic Create two Channels: email and points Each Channel has independent subscribers with different concurrency levels When publishing a message, it's replicated to all Channels Subscribers in each Channel process messages independently Advantages Decoupling: Registration service doesn't need to know specific notification logic Extensibility: Adding new notification methods only requires new consumers Reliability: NSQite guarantees at-least-once message delivery Performance: Supports concurrent processing, improving system throughput Summary NSQite Event Bus Design Principles NSQite consists of two parts: Event Bus and Transactional Message Queue. The design is inspired by NSQ's philosophy. The Event Bus is suitable for monolithic architectures, while the Transactional Message Queue is ideal for early-stage projects where you might not need large message queue systems like NSQ or Pulsar. It implements a two-level message distribution mechanism with Topic and Channel: Topic: Message classification, where one Topic can contain multiple Channels Channel: Messages are replicated to all Channels Subscriber: Subscribers can start concurrent goroutines to process channel messages quickly Core advantages of this design: Messages can be processed in parallel by multiple types of consumers Each Channel can independently set its concurrency level Supports both broadcast and point-to-point communication Implements simple load balancing Using github.com/ixugo/nsqite to implement the Event Bus pattern helps us build more flexible and maintainable system architectures. If you find this project helpful, please give it a Star! Project URL: https://github.com/ixugo/nsqite
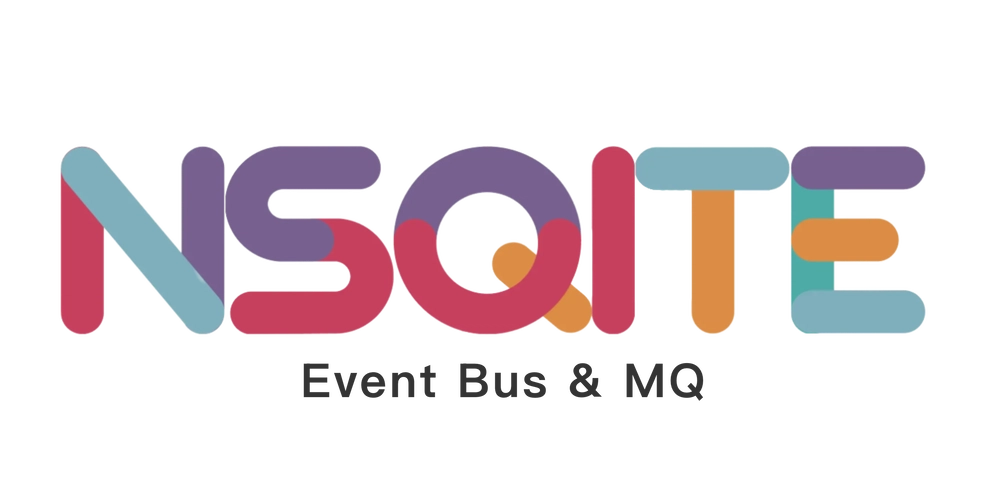
Event Bus Best Practices: Implementing Loose Coupling with NSQite
What is Event Bus?
Event Bus is a messaging pattern that allows different components of an application to communicate through a publish/subscribe mechanism without direct dependencies. This pattern is particularly suitable for implementing loosely coupled architectural designs.
Why Do We Need Event Bus?
- Decoupling: Components communicate through events without direct dependencies
- Extensibility: New features can be easily added by subscribing to existing events
- Maintainability: Code becomes easier to understand and maintain
- Asynchronous Processing: Supports asynchronous message handling, improving system responsiveness
Best Practice Case: User Registration Notification System
Background
Let's assume we're developing an e-commerce system where new user registration requires:
- Sending a welcome email
- Creating a user points account
- Pushing system notifications
- Recording user behavior logs
Traditional implementation would result in tight coupling between the registration service and these features, making the code difficult to maintain.
NSQite Solution
// Define event structure
type UserRegisteredEvent struct {
UserID string
Username string
Email string
Time time.Time
}
// Email service handler
type EmailHandler struct{}
func (h *EmailHandler) HandleMessage(message *EventMessage[UserRegisteredEvent]) error {
event := message.Body
// Send welcome email
return sendWelcomeEmail(event.Email)
}
// Points service handler
type PointsHandler struct{}
func (h *PointsHandler) HandleMessage(message *EventMessage[UserRegisteredEvent]) error {
event := message.Body
// Create user points account
return createUserPoints(event.UserID)
}
// Registration service
const topic = "user.registered"
var publisher = NewPublisher[UserRegisteredEvent]()
func RegisterUser(username, email string) error {
// Create user
userID := createUser(username, email)
// Publish user registration event
event := UserRegisteredEvent{
UserID: userID,
Username: username,
Email: email,
Time: time.Now(),
}
return publisher.Publish(topic, event)
}
// Main program
func main() {
// Initialize email subscriber
const emailChannel = "email"
emailSub := NewSubscriber(topic, emailChannel)
emailSub.AddConcurrentHandlers(&EmailHandler{}, 2)
// Initialize points subscriber
const pointsChannel = "points"
pointsSub := NewSubscriber(topic, pointsChannel)
pointsSub.AddConcurrentHandlers(&PointsHandler{}, 2)
// Register new user
RegisterUser("testuser", "test@example.com")
}
Code Explanation
- We define a
user.registered
Topic - Create two Channels:
email
andpoints
- Each Channel has independent subscribers with different concurrency levels
- When publishing a message, it's replicated to all Channels
- Subscribers in each Channel process messages independently
Advantages
- Decoupling: Registration service doesn't need to know specific notification logic
- Extensibility: Adding new notification methods only requires new consumers
- Reliability: NSQite guarantees at-least-once message delivery
- Performance: Supports concurrent processing, improving system throughput
Summary
NSQite Event Bus Design Principles
NSQite consists of two parts: Event Bus and Transactional Message Queue. The design is inspired by NSQ's philosophy. The Event Bus is suitable for monolithic architectures, while the Transactional Message Queue is ideal for early-stage projects where you might not need large message queue systems like NSQ or Pulsar.
It implements a two-level message distribution mechanism with Topic and Channel:
- Topic: Message classification, where one Topic can contain multiple Channels
- Channel: Messages are replicated to all Channels
- Subscriber: Subscribers can start concurrent goroutines to process channel messages quickly
Core advantages of this design:
- Messages can be processed in parallel by multiple types of consumers
- Each Channel can independently set its concurrency level
- Supports both broadcast and point-to-point communication
- Implements simple load balancing
Using github.com/ixugo/nsqite to implement the Event Bus pattern helps us build more flexible and maintainable system architectures. If you find this project helpful, please give it a Star!
Project URL: https://github.com/ixugo/nsqite