Git Branching and Merging: A Data Engineer's Guide to Efficient Code Management
Git Branching and Merging: A Step-By-Step Guide As a data engineer, managing and collaborating on code for data pipelines, transformations, and infrastructure is crucial. Git branching and merging are fundamental practices that enable us to work efficiently and safely on our projects. This guide outlines the key concepts and steps involved in Git branching and merging, which are highly applicable to our daily workflows. What is Git Branching? Git branching allows us to diverge from the production version of our codebase to work on bug fixes or new features in isolation. We create branches to have a separate copy of the code, ensuring that our changes don't directly impact the stable, production-ready code. This isolation is particularly valuable when developing new data ingestion processes, implementing complex data transformations, or experimenting with different data storage solutions. We can test our changes thoroughly in a branch before integrating them into the main codebase. The main branch is typically the initial branch created when a Git repository is initialized. When we create a new branch, Git essentially creates a new pointer to the same commit that the main branch is currently pointing to. As we make commits in our new branch, Git tracks these changes with new pointers, and our branch's commit history starts to diverge from the main branch. Git uses a special pointer called HEAD to know which branch we currently have checked out. When we create a new branch, HEAD doesn't automatically switch to it; we need to explicitly check out the new branch to start working on it. Branch Naming Strategies for Data Engineering Projects: While branch names can technically be anything, establishing a consistent naming strategy within a data engineering team is essential for organization. Some useful strategies include: `•feature/: For developing new data pipelines or adding new data processing capabilities (e.g., feature/add-salesforce-ingestion). •bugfix/: For addressing issues or errors in existing data pipelines or infrastructure code (e.g., bugfix/resolve-null-value-error). •hotfix/: For critical and immediate fixes needed in the production environment (e.g., hotfix/fix-data-duplication-issue). •environment/: Potentially for tracking environment-specific configurations or deployments (e.g., environment/staging). •username/: Useful for individual developers to track their work on specific tasks (e.g., john.doe/etl-pipeline-optimization).` How to Create a Branch in Git: There are several ways to create branches in Git: 1. Using git branch: To create a branch without immediately switching to it, we use the git branch command followed by the desired branch name. This will show all available branches, with an asterisk indicating the currently active branch. 2. Using git checkout -b: To create a branch and immediately switch to it, we use the git checkout -b command followed by the new branch name. After this command, Git's HEAD pointer will be moved to the data-model-update branch. 3. Creating a Branch from a Commit: We can create a branch based on a specific historical commit. This is useful if we need to revisit a previous state of our data engineering code. First, we need to find the SHA-1 identifier of the commit using git log. Then, we use git branch . 4. Creating a Branch from Another Branch: It's common in data engineering workflows to create a new feature or bugfix branch from an existing development or feature branch. The process is the same as creating from the main branch, but we specify the name of the starting branch. 5. Downloading a Branch from a Remote Repository: When collaborating with other data engineers, we may need to work on branches that exist in the remote repository but not locally. We can use git pull origin to retrieve the branch. After pulling, we might need to explicitly check out the branch using git checkout to start working on it. Merging Branches: Once the work on a branch is complete and tested, we need to merge it back into the main branch or another relevant branch to integrate our changes. Git uses two primary types of merges: Fast-Forward Merge: If the branch we are merging has a direct ancestor commit in the target branch (e.g., main), Git simply moves the target branch's pointer forward to the latest commit of the merging branch. This often happens with short-lived feature or hotfix branches. To perform a local fast-forward merge, we first switch to the target branch (e.g., git checkout main) and then use git merge (e.g., git merge feature-branch). Three-Way Merge: If the commit history of the merging branch has diverged from the target branch (meaning new commits have been added to the target branch since the merging branch was created), Git performs a three-way merge. Git identifies a common ancestor commit, the latest commit of the merging branch, and the latest commit of the target branch. It then creates a new "merge commit" that combines
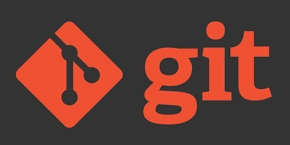
Git Branching and Merging: A Step-By-Step Guide
As a data engineer, managing and collaborating on code for data pipelines, transformations, and infrastructure is crucial. Git branching and merging are fundamental practices that enable us to work efficiently and safely on our projects. This guide outlines the key concepts and steps involved in Git branching and merging, which are highly applicable to our daily workflows.
What is Git Branching?
- Git branching allows us to diverge from the production version of our codebase to work on bug fixes or new features in isolation.
- We create branches to have a separate copy of the code, ensuring that our changes don't directly impact the stable, production-ready code. This isolation is particularly valuable when developing new data ingestion processes, implementing complex data transformations, or experimenting with different data storage solutions.
- We can test our changes thoroughly in a branch before integrating them into the main codebase.
- The main branch is typically the initial branch created when a Git repository is initialized. When we create a new branch, Git essentially creates a new pointer to the same commit that the main branch is currently pointing to. As we make commits in our new branch, Git tracks these changes with new pointers, and our branch's commit history starts to diverge from the main branch.
- Git uses a special pointer called HEAD to know which branch we currently have checked out. When we create a new branch, HEAD doesn't automatically switch to it; we need to explicitly check out the new branch to start working on it.
Branch Naming Strategies for Data Engineering Projects:
While branch names can technically be anything, establishing a consistent naming strategy within a data engineering team is essential for organization.
Some useful strategies include:
`•feature/: For developing new data pipelines or adding new data processing capabilities (e.g., feature/add-salesforce-ingestion).
•bugfix/: For addressing issues or errors in existing data pipelines or infrastructure code (e.g., bugfix/resolve-null-value-error).
•hotfix/: For critical and immediate fixes needed in the production environment (e.g., hotfix/fix-data-duplication-issue).
•environment/: Potentially for tracking environment-specific configurations or deployments (e.g., environment/staging).
•username/: Useful for individual developers to track their work on specific tasks (e.g., john.doe/etl-pipeline-optimization).`
How to Create a Branch in Git:
There are several ways to create branches in Git:
1. Using git branch: To create a branch without immediately switching to it, we use the git branch
command followed by the desired branch name.
This will show all available branches, with an asterisk indicating the currently active branch.
2. Using git checkout -b: To create a branch and immediately switch to it, we use the git checkout -b
command followed by the new branch name.
After this command, Git's HEAD pointer will be moved to the data-model-update branch.
3. Creating a Branch from a Commit: We can create a branch based on a specific historical commit. This is useful if we need to revisit a previous state of our data engineering code. First, we need to find the SHA-1 identifier of the commit using git log. Then, we use
git branch
.
4. Creating a Branch from Another Branch: It's common in data engineering workflows to create a new feature or bugfix branch from an existing development or feature branch. The process is the same as creating from the main branch, but we specify the name of the starting branch.
5. Downloading a Branch from a Remote Repository: When collaborating with other data engineers, we may need to work on branches that exist in the remote repository but not locally. We can use git pull origin
to retrieve the branch. After pulling, we might need to explicitly check out the branch using git checkout
to start working on it.
Merging Branches:
Once the work on a branch is complete and tested, we need to merge it back into the main branch or another relevant branch to integrate our changes. Git uses two primary types of merges:
Fast-Forward Merge: If the branch we are merging has a direct ancestor commit in the target branch (e.g., main), Git simply moves the target branch's pointer forward to the latest commit of the merging branch. This often happens with short-lived feature or hotfix branches. To perform a local fast-forward merge, we first switch to the target branch (e.g., git checkout main)
and then use git merge
(e.g., git merge feature-branch)
.
Three-Way Merge: If the commit history of the merging branch has diverged from the target branch (meaning new commits have been added to the target branch since the merging branch was created), Git performs a three-way merge. Git identifies a common ancestor commit, the latest commit of the merging branch, and the latest commit of the target branch. It then creates a new "merge commit" that combines the changes from both branches. To perform a local three-way merge, the process is the same: switch to the target branch (git checkout main)
and then use git merge
Merging Branches to a Remote Repository:
After merging a branch locally into the main branch (or another integration branch), we need to push these changes to the remote repository so that other team members can access them. If the branch we merged was a local branch that didn't exist remotely, we need to set the upstream branch using git push --set-upstream origin
. For subsequent pushes on that branch, a simple git push will suffice.
Merging Main into a Branch:
While working on a long-lived feature branch, it's good practice to periodically merge the latest changes from the main branch into your feature branch. This helps to avoid significant merge conflicts later on and ensures your feature branch stays relatively up-to-date. To do this, switch to your feature branch (git checkout
and then run git merge main
.
Key Takeaways for Data Engineers:
- Isolate Work: Use branches to isolate development work on new data pipelines, transformations, or infrastructure changes.
- Manage Environments: Consider using branches or GitFlow workflows to manage different development, staging, and production environments.
- Collaborate Effectively: Branches allow multiple data engineers to work on different aspects of a project simultaneously without interfering with each other's work.
- Review Code Changes: Branches facilitate code reviews before merging changes into the main branch, ensuring code quality and reducing the risk of introducing errors into production data pipelines.
- Version Control: Git branching and merging provide a robust history of changes, allowing us to easily revert to previous states if needed.
By understanding and effectively utilizing Git branching and merging strategies, data engineering teams can streamline their development processes, improve collaboration, and maintain a stable and reliable data infrastructure.