From Designer to Developer: Build Pixel-Perfect Components from Figma Using AI
Introduction & Context Bridging the gap between Figma designs and production-ready UI components has always been a challenge. Designers work in visual tools. Developers write code. In between? Handoff friction, manual styling, misaligned spacing, and duplicated work. But with the rise of AI-powered development tools and APIs like OpenAI, we now have the ability to automatically translate Figma designs into consistent, styled components—saving time and preserving the original UX intent. In this article, you’ll learn how to: Extract component specs from Figma Use AI to generate frontend code (Vue/React) Match design system tokens and styles Maintain responsive and accessible behavior Iterate faster between design and implementation Why Automate Figma-to-Component Translation? Speed: Cut time spent interpreting spacing, colors, and alignment Accuracy: Get 1:1 styling and layout fidelity Consistency: Reuse your design tokens, avoid design drift Collaboration: Empower designers and developers to speak the same language Tech Stack You’ll Use Figma API or Plugin (e.g., Figma Tokens) OpenAI GPT-4 / Claude / Gemini (via API) Vue 3 with Composition API (or React, optional) Tailwind CSS or your own Design Token System Vite or Storybook for rapid development/testing Step 1: Extract Design Data from Figma Use the Figma API to extract component information such as: Frame sizes and structure Text styles and spacing Border radius, colors, font weights Nested components and variants Or use plugins like Tokens Studio to export design tokens in JSON: { "color": { "primary": "#4f46e5", "surface": "#ffffff" }, "spacing": { "xs": "4px", "sm": "8px", "md": "16px" }, "radii": { "button": "6px" } } You can now feed this data into an AI prompt. Step 2: Craft Your Prompt for Code Generation Use the Figma JSON as part of your system prompt. Here’s an example: "You are a frontend developer assistant. Based on the following Figma design specs, write a Vue 3 component using TailwindCSS. Ensure spacing and colors match the design tokens. Output a fully functional component." Then provide an object block of the layout structure (e.g. Button with icon + label) and style tokens. Let GPT generate something like this: Example 1: Primary Button ... Click Me Example 2: Card Component with Image and Text Card Title This is a short description of the card content. Example 3: Input Field with Label and Error Email Please enter a valid email. Example 4: Modal Dialog Component Confirm Action Are you sure you want to delete this item? Cancel Delete Example 5: Stats Card with Icon ... Total Sales $24,320 Logo Home About Contact ☰ Step 3: Match Design Tokens Automatically Use a transformer function that replaces classnames like bg-primary with Tailwind token utilities (if you're using Tailwind preset with your tokens). Or inject your token map directly into the AI prompt so GPT outputs utility-safe classnames. Step 4: Render and Test the Output You can paste the code into: Storybook or Histoire for preview and documentation Your own component library setup via Vite or Nuxt A local AI preview tool (bonus: use live-edit GPT loops) Add interactivity or props as needed. GPT can even generate stories for different variants: Bonus: Iterate with AI Feedback Loops Add a comment block like: Then prompt the AI: “Refactor this component based on the developer feedback above.” Let GPT rewrite the code with mobile-first fixes and cleaner logic. Challenges and Considerations Responsiveness: Some AI output isn’t mobile-optimized. Add constraints. A11y: Make sure components meet accessibility standards. Validation: Always review AI output and run visual regression tests. Complex Interactions: Multi-state components may require manual tweaking. Summary and Final Thoughts AI won’t replace frontend developers—but it’s already becoming an incredible accelerator. With just Figma exports and a few prompts, you can go from design to working, styled code in minutes. By combining your component system, AI tools, and design specs, you create a powerful workflow that speeds up delivery without sacrificing polish. Call to Action / Community Engagement Have you tried integrating Figma and GPT for your component workflow? Are you building an AI-assisted design system? Share your tools, prompts, and ideas in the comments!
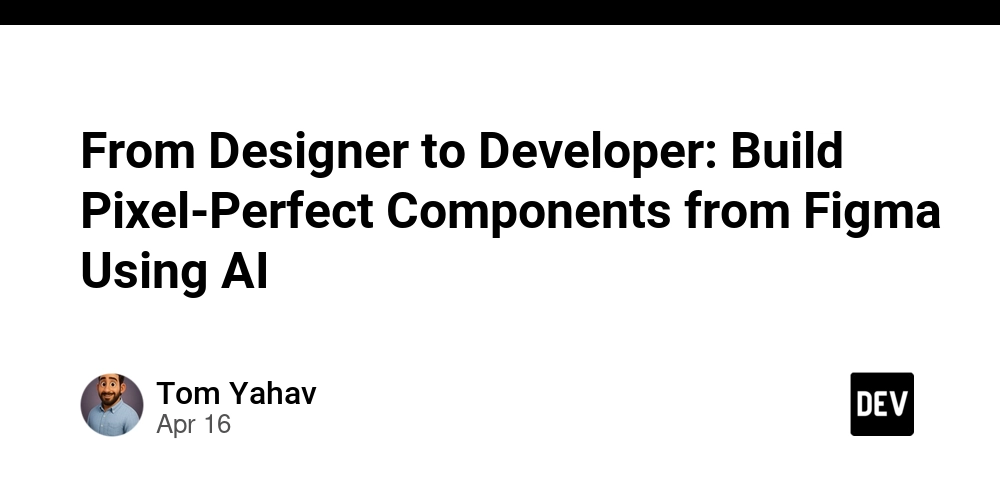
Introduction & Context
Bridging the gap between Figma designs and production-ready UI components has always been a challenge. Designers work in visual tools. Developers write code. In between? Handoff friction, manual styling, misaligned spacing, and duplicated work.
But with the rise of AI-powered development tools and APIs like OpenAI, we now have the ability to automatically translate Figma designs into consistent, styled components—saving time and preserving the original UX intent.
In this article, you’ll learn how to:
- Extract component specs from Figma
- Use AI to generate frontend code (Vue/React)
- Match design system tokens and styles
- Maintain responsive and accessible behavior
- Iterate faster between design and implementation
Why Automate Figma-to-Component Translation?
- Speed: Cut time spent interpreting spacing, colors, and alignment
- Accuracy: Get 1:1 styling and layout fidelity
- Consistency: Reuse your design tokens, avoid design drift
- Collaboration: Empower designers and developers to speak the same language
Tech Stack You’ll Use
- Figma API or Plugin (e.g., Figma Tokens)
- OpenAI GPT-4 / Claude / Gemini (via API)
- Vue 3 with Composition API (or React, optional)
- Tailwind CSS or your own Design Token System
- Vite or Storybook for rapid development/testing
Step 1: Extract Design Data from Figma
Use the Figma API to extract component information such as:
- Frame sizes and structure
- Text styles and spacing
- Border radius, colors, font weights
- Nested components and variants
Or use plugins like Tokens Studio to export design tokens in JSON:
{
"color": {
"primary": "#4f46e5",
"surface": "#ffffff"
},
"spacing": {
"xs": "4px",
"sm": "8px",
"md": "16px"
},
"radii": {
"button": "6px"
}
}
You can now feed this data into an AI prompt.
Step 2: Craft Your Prompt for Code Generation
Use the Figma JSON as part of your system prompt. Here’s an example:
"You are a frontend developer assistant. Based on the following Figma design specs, write a Vue 3 component using TailwindCSS. Ensure spacing and colors match the design tokens. Output a fully functional component."
Then provide an object block of the layout structure (e.g. Button with icon + label) and style tokens.
Let GPT generate something like this:
Example 1: Primary Button
Example 2: Card Component with Image and Text
Card Title
This is a short description of the card content.
Example 3: Input Field with Label and Error
Please enter a valid email.
Example 4: Modal Dialog Component
Confirm Action
Are you sure you want to delete this item?
Example 5: Stats Card with Icon
Total Sales
$24,320
Step 3: Match Design Tokens Automatically
Use a transformer function that replaces classnames like bg-primary
with Tailwind token utilities (if you're using Tailwind preset with your tokens).
Or inject your token map directly into the AI prompt so GPT outputs utility-safe classnames.
Step 4: Render and Test the Output
You can paste the code into:
- Storybook or Histoire for preview and documentation
- Your own component library setup via Vite or Nuxt
- A local AI preview tool (bonus: use live-edit GPT loops)
Add interactivity or props as needed. GPT can even generate stories for different variants:
Bonus: Iterate with AI Feedback Loops
Add a comment block like:
Then prompt the AI:
“Refactor this component based on the developer feedback above.”
Let GPT rewrite the code with mobile-first fixes and cleaner logic.
Challenges and Considerations
- Responsiveness: Some AI output isn’t mobile-optimized. Add constraints.
- A11y: Make sure components meet accessibility standards.
- Validation: Always review AI output and run visual regression tests.
- Complex Interactions: Multi-state components may require manual tweaking.
Summary and Final Thoughts
AI won’t replace frontend developers—but it’s already becoming an incredible accelerator
. With just Figma exports and a few prompts, you can go from design to working, styled code in minutes.
By combining your component system, AI tools, and design specs, you create a powerful workflow that speeds up delivery without sacrificing polish.
Call to Action / Community Engagement
Have you tried integrating Figma and GPT for your component workflow? Are you building an AI-assisted design system? Share your tools, prompts, and ideas in the comments!