Debugging a 500 Error in Amplify Gen 2 SSR with DynamoDB (Solved)
I am relatively new to coding but studied AWS in college, so fixing this issue with SSR on Amplify felt really rewarding. I am working on developing an E-comm web app for the company I work for, its a NextJS 15 stack, hosted through amplify and using DynamoDB for SSR. I made a feature for adding and managing product listings on the site where you can upload an excel CSV file with product info, title, price, etc to S3 -> lambda parses it and sends it to dynamoDB. I used GetStaticProps to render the product data on MUI tables on one page, and [...slug] pages that are linked to the MUI tables. I think people have a common issue with having SSR working perfectly fine on local, but when pushing it to amplify gen 2 getting a "500 internal server error". I'll share how I solved my issue and hope it steers some people in the right direction to solve theirs. Things to note NextJS 15 stack, running Node 20.x On local, I had my .env.local with AWS access keys & region. When I got the 500 error I checked cloudwatch logs for the app and found the log for when I tried visiting the pages that were giving me the 500 error. I saw a repeated error in the logs "Syntax Error: Unexpected token 'export'" Which is pretty non descript, it'd be easier if AWS could provide more details but that was all I had to go off of. I went through a few checklist things making sure my amplify.yml specified the correct Node version to build the app with on deployment. Lesson to self: Always look at what directory is mentioned in the error so I don't go rummaging through my code like a chicken with its head cut off trying to find what the cause is. It seemed the error was pointing to one or more of the components I was using was not transpiling which I though was weird because all the builds worked locally and on amplify before hand, just not now that I am using SSR with dynamo. So I added a BUNCH of components and packages I was using into my next.config.js transpilePackages list. No luck. When I looked at exactly where cloudwatch was saying the error was happening (the log right under the SyntaxError), I saw it mention my .next/server/pages/catagorypage.js which when I opened it from my vs code all I saw was a bunch of heaped together code I didn't know what to do with. This is where I learned a new little trick: I learned you can disable webpack minification temporarily by putting this line in next.config.js: config.optimization.minimize = false; I rebuilt .next and returned to the file cloudwatch logs was claiming created the syntax error. In the file all the heaped together code was in neat readable code format and I could finally look at which "export" statement was bringing in a package that wasn't transpiling. I noticed one particular statement "rc-pagination/es/locale/en_US". The folder /es/ was a tell that the rc package (which I believe is support for ant d components) contain native exports that werent being transpiled. Solution: I jumped back into next.config.js and added everything RC-* related I could find on that file into the transpilePackages list, did a clean rebuild on the nextjs app (rm -rf node_modules .next -> npm install -> npm build) everything worked good on local like before, pushed to github & amplify, and the 500 error was no more! The pages rendered like they should (after reversing some fixes I thought would help but made it worse). Key takeaway: I learned a lot about debugging in this process, especially to always look for the clues in the logs and to start there before trying something that could be completely unrelated. I feel a lot more confident that in future debugging situations I can hunt down the issue and resolve it better, especially when I can learn new tricks like disabling webpack minification. I hope this post may help some people if they are having issues with SSR on amplify, I know AWS logs in amplify and cloudwatch can be vague.
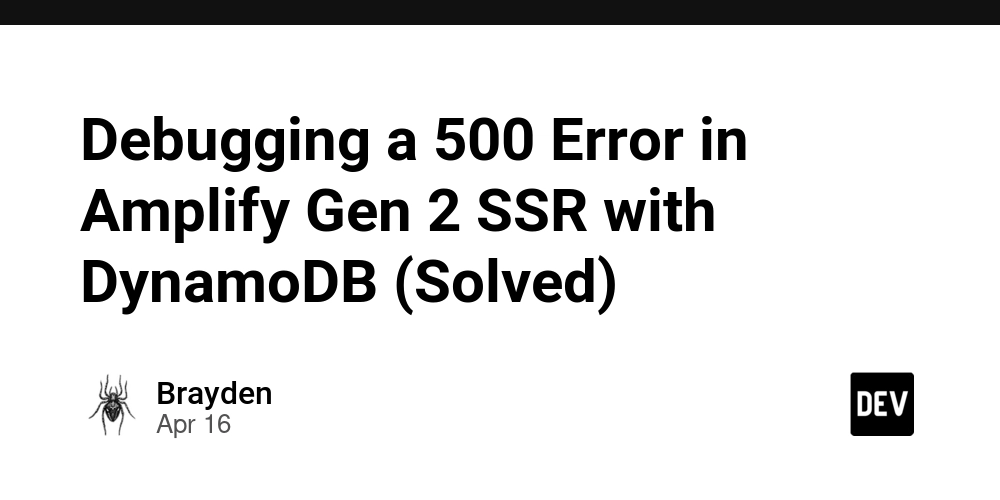
I am relatively new to coding but studied AWS in college, so fixing this issue with SSR on Amplify felt really rewarding.
I am working on developing an E-comm web app for the company I work for, its a NextJS 15 stack, hosted through amplify and using DynamoDB for SSR. I made a feature for adding and managing product listings on the site where you can upload an excel CSV file with product info, title, price, etc to S3 -> lambda parses it and sends it to dynamoDB. I used GetStaticProps to render the product data on MUI tables on one page, and [...slug] pages that are linked to the MUI tables.
I think people have a common issue with having SSR working perfectly fine on local, but when pushing it to amplify gen 2 getting a "500 internal server error". I'll share how I solved my issue and hope it steers some people in the right direction to solve theirs.
Things to note
NextJS 15 stack, running Node 20.x
On local, I had my .env.local with AWS access keys & region.
When I got the 500 error I checked cloudwatch logs for the app and found the log for when I tried visiting the pages that were giving me the 500 error. I saw a repeated error in the logs "Syntax Error: Unexpected token 'export'" Which is pretty non descript, it'd be easier if AWS could provide more details but that was all I had to go off of. I went through a few checklist things making sure my amplify.yml specified the correct Node version to build the app with on deployment.
Lesson to self: Always look at what directory is mentioned in the error so I don't go rummaging through my code like a chicken with its head cut off trying to find what the cause is.
It seemed the error was pointing to one or more of the components I was using was not transpiling which I though was weird because all the builds worked locally and on amplify before hand, just not now that I am using SSR with dynamo. So I added a BUNCH of components and packages I was using into my next.config.js transpilePackages list. No luck.
When I looked at exactly where cloudwatch was saying the error was happening (the log right under the SyntaxError), I saw it mention my .next/server/pages/catagorypage.js which when I opened it from my vs code all I saw was a bunch of heaped together code I didn't know what to do with.
This is where I learned a new little trick: I learned you can disable webpack minification temporarily by putting this line in next.config.js:
config.optimization.minimize = false;
I rebuilt .next and returned to the file cloudwatch logs was claiming created the syntax error. In the file all the heaped together code was in neat readable code format and I could finally look at which "export" statement was bringing in a package that wasn't transpiling. I noticed one particular statement "rc-pagination/es/locale/en_US". The folder /es/ was a tell that the rc package (which I believe is support for ant d components) contain native exports that werent being transpiled.
Solution:
I jumped back into next.config.js and added everything RC-* related I could find on that file into the transpilePackages list, did a clean rebuild on the nextjs app (rm -rf node_modules .next -> npm install -> npm build) everything worked good on local like before, pushed to github & amplify, and the 500 error was no more! The pages rendered like they should (after reversing some fixes I thought would help but made it worse).
Key takeaway:
I learned a lot about debugging in this process, especially to always look for the clues in the logs and to start there before trying something that could be completely unrelated. I feel a lot more confident that in future debugging situations I can hunt down the issue and resolve it better, especially when I can learn new tricks like disabling webpack minification.
I hope this post may help some people if they are having issues with SSR on amplify, I know AWS logs in amplify and cloudwatch can be vague.