Free 30-Day Full-Stack Development Learning Path
30-Day Full-Stack Development Learning Plan (1 Hour Daily) Copy and paste the resource recommendations for each day into search, or research your own with your LLM. Here's a structured plan that combines the best free resources into a cohesive learning journey:### Week 1: React Fundamentals and Replit Integration Day 1: React Environment Setup on Replit Set up a React development environment on Replit Review React component fundamentals Resources: freeCodeCamp React section, Replit documentation Activity: Create a simple React app on Replit Day 2: React Components and Props Learn component hierarchy and composition Master props and state management Resources: React official documentation, freeCodeCamp Activity: Build a component with props and state Day 3: React HooksImplement useState and useEffect Understand React component lifecycle Resources: React Hooks documentation Activity: Convert a class component to a functional component with hooks Day 4: React Forms and Events Handle form submissions and events Implement form validation Resources: freeCodeCamp React forms section Activity: Create a form with validation in your Replit project Day 5: Modern React Styling Explore CSS modules vs. styled components Implement Tailwind CSS in React Resources: Tailwind CSS documentation Activity: Style your existing components with Tailwind Day 6: React RouterSet up client-side routing Implement route parameters and nested routes Resources: React Router documentation Activity: Create a multi-page React application Day 7: Week 1 Review and Mini-Project Consolidate React fundamentals Resources: Review previous materials Activity: Build a multi-page React application with forms, styling, and routing Week 2: Node.js and Backend Development Day 8: Node.js FundamentalsExplore Node.js architecture and event loop Set up npm and manage packages Resources: Node.js documentation, The Odin Project Activity: Create a basic Node.js server on Replit Day 9: Express.js Framework Build Express server routes Implement middleware functions Resources: Express documentation, freeCodeCamp Activity: Create a RESTful API structure Day 10: Advanced Express APIs Design RESTful endpoints Implement CRUD operations Resources: RESTful API design guides Activity: Build a complete API with multiple endpoints Day 11: Database IntegrationConnect to Replit database options Implement data models Resources: Replit database documentation Activity: Add database persistence to your API Day 12: Authentication Implementation Understand JWT authentication Implement secure user authentication Resources: Authentication tutorials, Replit Auth documentation Activity: Add user authentication to your application Day 13: Error Handling and Validation Create error-handling middleware Validate API requests Resources: Express error handling documentation Activity: Implement robust error handling Day 14: Week 2 Review and Backend Project Consolidate backend development concepts Resources: Review previous materials Activity: Complete a functional backend API with authentication Week 3: Next.js and Full-Stack Integration Day 15: Next.js Fundamentals Understand Next.js framework architecture Implement server-side rendering concepts Resources: Next.js documentation, YouTube tutorial[5] Activity: Convert your React app to Next.js Day 16: Next.js Routing System Master file-based routing Implement dynamic routes Resources: Next.js routing documentation Activity: Create a multi-page Next.js application Day 17: Next.js API Routes Build backend functionality within Next.js Connect frontend components to API routes Resources: Next.js API documentation Activity: Create and connect to API routes Day 18: Data Fetching in Next.js Implement getStaticProps and getServerSideProps Handle server-side data loading Resources: Next.js data fetching documentation Activity: Add server-side data loading to your application Day 19: Replit Deployment for Next.js Configure Next.js for Replit deployment Set up environment variables Resources: Replit deployment guide[6] Activity: Deploy your Next.js application on Replit Day 20: Full-Stack Architecture Design efficient client-server communication Implement proper data flow patterns Resources: Full-stack architecture best practices Activity: Connect frontend and backend systems Day 21: Week 3 Review and Project Integration Consolidate Next.js knowledge Resources: Review previous materials Activity: Build a complete full-stack application deployed on Replit Week 4: Shadcn UI and Advanced Topics Day 22: Shadcn UI Introduction Understand Shadcn UI design philosophy Set up Shadcn UI with your Next.js project Resources: Shadcn documentation, YouTube tutorial[2] Activity: Integrate Shadcn UI into your application Day 23: Building Components with Shadcn Explore Shadcn component architecture Implement custom components Resources: Shadcn UI component documentation Activity: Replace existing UI with Shadcn
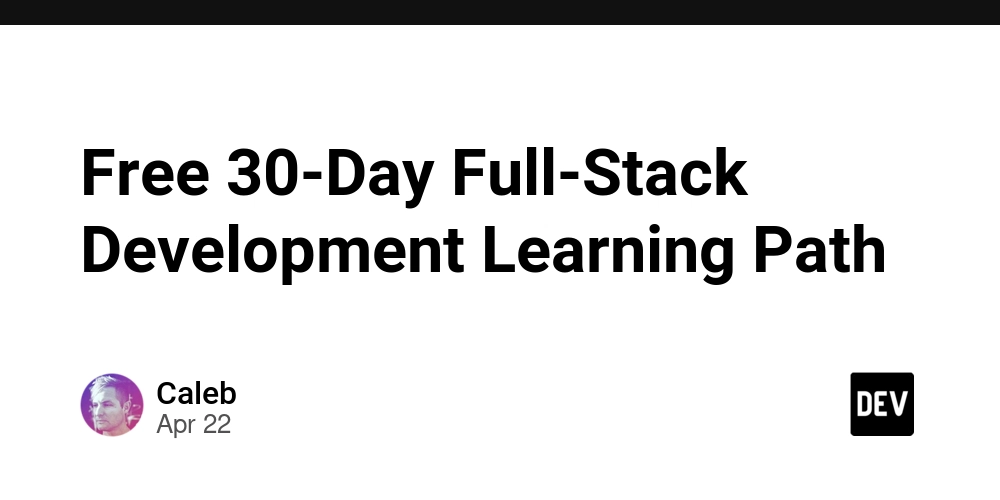
30-Day Full-Stack Development Learning Plan (1 Hour Daily)
Copy and paste the resource recommendations for each day into search, or research your own with your LLM.
Here's a structured plan that combines the best free resources into a cohesive learning journey:### Week 1: React Fundamentals and Replit Integration
Day 1: React Environment Setup on Replit
Set up a React development environment on Replit
Review React component fundamentals
Resources: freeCodeCamp React section, Replit documentation
Activity: Create a simple React app on Replit
Day 2: React Components and Props
Learn component hierarchy and composition
Master props and state management
Resources: React official documentation, freeCodeCamp
Activity: Build a component with props and state
Day 3: React HooksImplement useState and useEffect
Understand React component lifecycle
Resources: React Hooks documentation
Activity: Convert a class component to a functional component with hooks
Day 4: React Forms and Events
Handle form submissions and events
Implement form validation
Resources: freeCodeCamp React forms section
Activity: Create a form with validation in your Replit project
Day 5: Modern React Styling
Explore CSS modules vs. styled components
Implement Tailwind CSS in React
Resources: Tailwind CSS documentation
Activity: Style your existing components with Tailwind
Day 6: React RouterSet up client-side routing
Implement route parameters and nested routes
Resources: React Router documentation
Activity: Create a multi-page React application
Day 7: Week 1 Review and Mini-Project
Consolidate React fundamentals
Resources: Review previous materials
Activity: Build a multi-page React application with forms, styling, and routing
Week 2: Node.js and Backend Development
Day 8: Node.js FundamentalsExplore Node.js architecture and event loop
Set up npm and manage packages
Resources: Node.js documentation, The Odin Project
Activity: Create a basic Node.js server on Replit
Day 9: Express.js Framework
Build Express server routes
Implement middleware functions
Resources: Express documentation, freeCodeCamp
Activity: Create a RESTful API structure
Day 10: Advanced Express APIs
Design RESTful endpoints
Implement CRUD operations
Resources: RESTful API design guides
Activity: Build a complete API with multiple endpoints
Day 11: Database IntegrationConnect to Replit database options
Implement data models
Resources: Replit database documentation
Activity: Add database persistence to your API
Day 12: Authentication Implementation
Understand JWT authentication
Implement secure user authentication
Resources: Authentication tutorials, Replit Auth documentation
Activity: Add user authentication to your application
Day 13: Error Handling and Validation
Create error-handling middleware
Validate API requests
Resources: Express error handling documentation
Activity: Implement robust error handling
Day 14: Week 2 Review and Backend Project
Consolidate backend development concepts
Resources: Review previous materials
Activity: Complete a functional backend API with authentication
Week 3: Next.js and Full-Stack Integration
Day 15: Next.js Fundamentals
Understand Next.js framework architecture
Implement server-side rendering concepts
Resources: Next.js documentation, YouTube tutorial[5]
Activity: Convert your React app to Next.js
Day 16: Next.js Routing System
Master file-based routing
Implement dynamic routes
Resources: Next.js routing documentation
Activity: Create a multi-page Next.js application
Day 17: Next.js API Routes
Build backend functionality within Next.js
Connect frontend components to API routes
Resources: Next.js API documentation
Activity: Create and connect to API routes
Day 18: Data Fetching in Next.js
Implement getStaticProps and getServerSideProps
Handle server-side data loading
Resources: Next.js data fetching documentation
Activity: Add server-side data loading to your application
Day 19: Replit Deployment for Next.js
Configure Next.js for Replit deployment
Set up environment variables
Resources: Replit deployment guide[6]
Activity: Deploy your Next.js application on Replit
Day 20: Full-Stack Architecture
Design efficient client-server communication
Implement proper data flow patterns
Resources: Full-stack architecture best practices
Activity: Connect frontend and backend systems
Day 21: Week 3 Review and Project Integration
Consolidate Next.js knowledge
Resources: Review previous materials
Activity: Build a complete full-stack application deployed on Replit
Week 4: Shadcn UI and Advanced Topics
Day 22: Shadcn UI Introduction
Understand Shadcn UI design philosophy
Set up Shadcn UI with your Next.js project
Resources: Shadcn documentation, YouTube tutorial[2]
Activity: Integrate Shadcn UI into your application
Day 23: Building Components with Shadcn
Explore Shadcn component architecture
Implement custom components
Resources: Shadcn UI component documentation
Activity: Replace existing UI with Shadcn components
Day 24: Shadcn Theme Customization
Implement light and dark modes
Customize component themes
Resources: Shadcn theming documentation
Activity: Add theme customization to your application
Day 25: Advanced UI Patterns
Implement modals, drawers, and tooltips
Create responsive layouts
Resources: Shadcn UI advanced components documentation
Activity: Add complex UI interactions to your application
Day 26: Performance Optimization
Implement code splitting and lazy loading
Optimize assets and API calls
Resources: Next.js performance documentation
Activity: Measure and improve application performance
Day 27: Testing Full-Stack Applications
Set up testing frameworks
Write component and API tests
Resources: Testing documentation for your stack
Activity: Add test coverage to critical components
Day 28: Debugging and Troubleshooting
Use browser and Node.js debugging tools
Identify and fix common issues
Resources: Debugging guides for React and Next.js
Activity: Debug and fix issues in your application
Day 29: Advanced Replit Features
Leverage Replit AI assistance
Use Replit collaboration tools
Resources: Replit advanced features documentation[3]
Activity: Implement advanced Replit features in your project
Day 30: Final Project Completion
Polish your full-stack application
Implement final features
Resources: All previous materials
Activity: Complete your full-featured application with React, Next.js, Node.js, and Shadcn UI on Replit