File and Blob API in Depth
File and Blob API in Depth: A Comprehensive Exploration Introduction The File and Blob APIs are integral to modern web development, especially as the web increasingly moves towards being a platform that supports complex file uploads, downloads, and manipulation of binary data. This article will explore the historical context, technical implementations, real-world use cases, and edge-case handling of these APIs. It is designed to be a definitive guide for senior developers who want to deeply understand how to leverage these technologies effectively. Historical and Technical Context Prior to the introduction of the File and Blob APIs, handling files in web environments was complex and cumbersome. Before HTML5, uploads were typically handled via forms with input elements of type "file," and the processing was performed server-side. The introduction of the File API (part of the larger HTML5 specification) in 2011 greatly enhanced the web's capability to handle file uploads directly in the browser. The Blob object was introduced to handle binary data more easily. A Blob represents a file-like object of immutable, raw data. It can be used to represent data that doesn't necessarily conform to a specific format, or to create files programmatically in the browser. The evolution of these APIs indicated a move toward client-side file handling, reducing server load and improving user experience. Core Concepts Blob: Represents a chunk of raw binary data; handles binary files such as images and documents. File: A specialized type of Blob that represents an actual file on the user's filesystem. FileReader: An interface that provides methods to asynchronously read the contents of files (or Blobs) stored on the user's computer. URL.createObjectURL(): Creates a URL that references the given Blob or File, allowing you to use it as an image source or link. Detailed Technical Exploration Creating a Blob Creating a Blob is straightforward. It takes an array of data and optional options for the Blob's type. const myBlob = new Blob(['Hello, world!'], { type: 'text/plain' }); console.log(myBlob.size); // 13 console.log(myBlob.type); // 'text/plain' The type parameter specifies the MIME type, which is fundamental for the browser to know how to handle the data. Working with Files The File object is derived from Blob, and it contains additional properties such as name and lastModified. To create a File object, typically you'll be dealing with file input: Using JavaScript to read the file: const input = document.getElementById('fileInput'); input.addEventListener('change', function(event) { const file = event.target.files[0]; console.log(file.name); // name of the file console.log(file.size); // size in bytes console.log(file.type); // MIME type }); Reading Files with FileReader The FileReader API provides a way to asynchronously read the contents of files. Here’s a comprehensive example: const reader = new FileReader(); reader.onload = (event) => { console.log('File content:', event.target.result); }; reader.onerror = (event) => { console.error('Error reading file:', event.target.error); }; // Assuming `file` is a File object obtained from an input reader.readAsText(file); // Read file as text Converting File and Blob objects to URLs Using URL.createObjectURL() can allow you to generate temporary URLs for Blob and File objects. const blobUrl = URL.createObjectURL(myBlob); const imgElement = document.createElement('img'); imgElement.src = blobUrl; document.body.appendChild(imgElement); Examples of Complex Scenarios 1. Uploading Multiple Files This example showcases a multi-file upload with progress tracking: const input = document.getElementById('fileInput'); input.addEventListener('change', async (e) => { const files = e.target.files; for (const file of files) { const readFile = new Promise((resolve, reject) => { const reader = new FileReader(); reader.onload = (event) => { console.log(`Content of ${file.name}: ${event.target.result}`); resolve(); }; reader.onerror = reject; reader.readAsText(file); }); await readFile; } }); 2. Chunked Upload with Progress For larger files, upload them in chunks, allowing display progress: async function uploadChunks(file, chunkSize = 1024 * 1024) { const totalChunks = Math.ceil(file.size / chunkSize); for (let i = 0; i { setTimeout(() => resolve(), 1000); // Dummy delay for the simulation }); } Real-World Use Cases Image/CSS/JavaScript Uploaders: Applications like code editors, where the user can upload images or Sass files for use; examples include CodePen and JSFiddle. Cloud Storage Interfaces: S
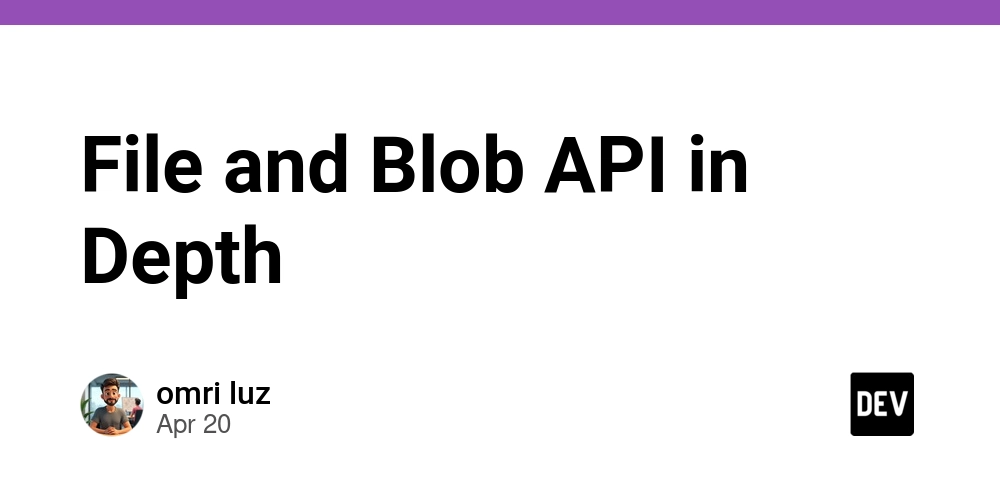
File and Blob API in Depth: A Comprehensive Exploration
Introduction
The File and Blob APIs are integral to modern web development, especially as the web increasingly moves towards being a platform that supports complex file uploads, downloads, and manipulation of binary data. This article will explore the historical context, technical implementations, real-world use cases, and edge-case handling of these APIs. It is designed to be a definitive guide for senior developers who want to deeply understand how to leverage these technologies effectively.
Historical and Technical Context
Prior to the introduction of the File and Blob APIs, handling files in web environments was complex and cumbersome. Before HTML5, uploads were typically handled via forms with input elements of type "file," and the processing was performed server-side. The introduction of the File API (part of the larger HTML5 specification) in 2011 greatly enhanced the web's capability to handle file uploads directly in the browser.
The Blob
object was introduced to handle binary data more easily. A Blob
represents a file-like object of immutable, raw data. It can be used to represent data that doesn't necessarily conform to a specific format, or to create files programmatically in the browser.
The evolution of these APIs indicated a move toward client-side file handling, reducing server load and improving user experience.
Core Concepts
- Blob: Represents a chunk of raw binary data; handles binary files such as images and documents.
- File: A specialized type of Blob that represents an actual file on the user's filesystem.
- FileReader: An interface that provides methods to asynchronously read the contents of files (or Blobs) stored on the user's computer.
- URL.createObjectURL(): Creates a URL that references the given Blob or File, allowing you to use it as an image source or link.
Detailed Technical Exploration
Creating a Blob
Creating a Blob
is straightforward. It takes an array of data and optional options for the Blob's type.
const myBlob = new Blob(['Hello, world!'], { type: 'text/plain' });
console.log(myBlob.size); // 13
console.log(myBlob.type); // 'text/plain'
The type
parameter specifies the MIME type, which is fundamental for the browser to know how to handle the data.
Working with Files
The File
object is derived from Blob
, and it contains additional properties such as name
and lastModified
.
To create a File
object, typically you'll be dealing with file input:
type="file" id="fileInput">
Using JavaScript to read the file:
const input = document.getElementById('fileInput');
input.addEventListener('change', function(event) {
const file = event.target.files[0];
console.log(file.name); // name of the file
console.log(file.size); // size in bytes
console.log(file.type); // MIME type
});
Reading Files with FileReader
The FileReader
API provides a way to asynchronously read the contents of files. Here’s a comprehensive example:
const reader = new FileReader();
reader.onload = (event) => {
console.log('File content:', event.target.result);
};
reader.onerror = (event) => {
console.error('Error reading file:', event.target.error);
};
// Assuming `file` is a File object obtained from an input
reader.readAsText(file); // Read file as text
Converting File and Blob objects to URLs
Using URL.createObjectURL()
can allow you to generate temporary URLs for Blob and File objects.
const blobUrl = URL.createObjectURL(myBlob);
const imgElement = document.createElement('img');
imgElement.src = blobUrl;
document.body.appendChild(imgElement);
Examples of Complex Scenarios
1. Uploading Multiple Files
This example showcases a multi-file upload with progress tracking:
type="file" id="fileInput" multiple>
const input = document.getElementById('fileInput');
input.addEventListener('change', async (e) => {
const files = e.target.files;
for (const file of files) {
const readFile = new Promise((resolve, reject) => {
const reader = new FileReader();
reader.onload = (event) => {
console.log(`Content of ${file.name}: ${event.target.result}`);
resolve();
};
reader.onerror = reject;
reader.readAsText(file);
});
await readFile;
}
});
2. Chunked Upload with Progress
For larger files, upload them in chunks, allowing display progress:
async function uploadChunks(file, chunkSize = 1024 * 1024) {
const totalChunks = Math.ceil(file.size / chunkSize);
for (let i = 0; i < totalChunks; i++) {
const start = i * chunkSize;
const end = Math.min(start + chunkSize, file.size);
const chunk = file.slice(start, end);
// Simulated upload function
await simulateUpload(chunk);
console.log(`Uploaded chunk ${i+1} of ${totalChunks}`);
}
}
function simulateUpload(chunk) {
return new Promise(resolve => {
setTimeout(() => resolve(), 1000); // Dummy delay for the simulation
});
}
Real-World Use Cases
Image/CSS/JavaScript Uploaders: Applications like code editors, where the user can upload images or Sass files for use; examples include CodePen and JSFiddle.
Cloud Storage Interfaces: Services such as Dropbox or Google Drive enable users to upload and manage files with the help of the File and Blob APIs.
Data Transfer in Web Applications: Modern web applications leverage Blob for large data transfers, such as exporting data in CSV or PDF formats.
Performance Considerations and Optimization Strategies
-
Memory Management:
Blob
and File objects consume memory, particularly for large files. Ensure to release memory by revoking object URLs when finished:
URL.revokeObjectURL(blobUrl);
Efficiency: For larger files, consider chunking uploads or using Web Workers to process data in the background, avoiding blocking the main thread.
Compression: When uploading files, consider using compression algorithms on the client-side (e.g., using libraries like
pako
) to reduce upload sizes and times.
Potential Pitfalls and Advanced Debugging Techniques
Asynchronous Behavior: Ensure to handle asynchronous operations appropriately using promises, async/await, or callbacks; overlooking these can lead to race conditions or unexpected behavior.
File Type Restrictions: Implement robust error handling around file types and sizes to enhance user experience and avoid unnecessary uploads.
Debugging FileReader Issues: Utilize the
onerror
event handler to manage and log potential issues when reading files.
reader.onerror = (event) => {
console.error("File could not be read! Code: " + event.target.error.code);
};
- Handling Large Files: Test applications on devices with different hardware capabilities to ensure consistent performance when dealing with large file uploads.
Alternatives to File and Blob API
WebSockets: For real-time file uploads and updates, consider using WebSockets for continuous connections. This is especially useful for applications that require low latency.
Service Workers: For background synchronization of files and offline capabilities, Service Workers can be utilized to enhance file management in applications like PWAs (Progressive Web Applications).
Third-Party Libraries: Libraries like Dropzone.js or FineUploader simplify file uploads but abstract the underlying Blob/File API, come at the cost of flexibility and control.
Conclusion
The File and Blob APIs have dramatically transformed how modern web applications handle files. Understanding their complexities allows developers to build efficient, robust, and user-friendly applications. The case studies and examples discussed offer connectivity between theoretical concepts and practical implementation, ensuring that developers can harness the full potential of these APIs in real-world scenarios.
References
- MDN Web Docs - File API
- MDN Web Docs - Blob API
- MDN Web Docs - FileReader API
- HTML Living Standard: Blob
- HTML Living Standard: File
This article serves as a comprehensive guide, designed to elevate the understanding of the File and Blob APIs to a level suitable for proficient developers aiming to master advanced JavaScript concepts.