Exploring JSON Encoding in Scala: The Role of Macros and Derived Encoders
the entire source code user here can be found at below gist. https://gist.github.com/depareddy/ce6f1500af400f69752beb5a9da05bbf An encoder is like a helper that takes case class turns it into this special code (JSON format).case class in scala can defined like below. case class Person(name: String, age: Int) In our code, we have a special helper called JsonEncoder: JsonEncoder Trait: The JsonEncoder trait defines a type class for encoding values into JSON strings: trait JsonEncoder[T] { def encode(value: T): String } This trait allows for polymorphic behavior, enabling different types to be encoded in a consistent manner. Primitive Type Encoders: Implicit encoders for primitive types are defined using given: given stringEncoder: JsonEncoder[String] with def encode(value: String): String = s""""$value"""" given intEncoder: JsonEncoder[Int] with def encode(value: Int): String = value.toString given booleanEncoder: JsonEncoder[Boolean] with def encode(value: Boolean): String = value.toString List Encoder: A generic encoder for lists is provided, which recursively uses the element encoder: given listEncoder[T](using encoder: JsonEncoder[T]): JsonEncoder[List[T]] with def encode(list: List[T]): String = list.map(encoder.encode).mkString("[", ", ", "]") inline for Compile-Time Resolution What it does: Forces the compiler to evaluate expressions or patterns at compile time instead of runtime. inline: This keyword indicates that the method can be inlined by the compiler, which can lead to performance optimizations. It allows the compiler to replace calls to this method with the method's body at compile time. Let's break down the below given instance in detail, focusing on how it works to automatically generate JSON encoders for case classes in Scala 3. This part of the code is crucial for the functionality of the JSON encoding mechanism. inline given derived[T](using m: Mirror.Of[T]): JsonEncoder[T] = new JsonEncoder[T] { def encode(value: T): String = { inline m match { case p: Mirror.ProductOf[T] => val labels = summonLabels[p.MirroredElemLabels] println(s"labels:$labels") val encoders = summonEncoders[p.MirroredElemTypes] val values = value.asInstanceOf[Product].productIterator.toList val encodedFields = (labels, encoders, values).zipped.map { (label, encoder, value) => s""""$label": ${encoder.asInstanceOf[JsonEncoder[Any]].encode(value)}""" } s"{${encodedFields.mkString(", ")}}" case _ => throw new IllegalArgumentException(s"Unsupported type: ${m.toString}") } } } The derived method automates the creation of type class instances (e.g., JsonEncoder[Person]) for case classes/sealed traits by leveraging compile-time metaprogramming. The Compiler Calls derived When you write val perJson = encode(per) // Requires JsonEncoder[Person] The compiler: Looks for a given JsonEncoder[Person] in scope. If none exists, it tries to derive one using the above derived method 1)Inline Given Instance: inline given derived[T] (using m: Mirror.Of[T]): JsonEncoder[T] Inline Given Instance: This defines an implicit instance of JsonEncoder[T] for any type T that has a corresponding Mirror. using m: Mirror.Of[T]: This means that the method requires an implicit Mirror for type T. The Mirror provides reflection capabilities to inspect the structure of the case class. The Mirror.Of[T] type provides compile-time metadata about T (e.g., field names/types for case classes). Mirror.Of[T] is a type class automatically generated by the Scala 3 compiler for case classes/sealed traits. Is it a case class (product) or sealed trait (sum)? it tells What are the field names and types (for case classes)? similarly What are the subtypes (for sealed traits)? The compiler generates: Mirror.ProductOf[Person] { type MirroredElemLabels = ("name", "age") type MirroredElemTypes = (String, Int) } inline match on m ensures the compiler checks if T is a case class (product type) or a sum type (e.g., sealed trait) during compilation. Without inline, this check would happen at runtime, leading to errors or inefficiency. inline for Recursive Type-Level Computations What it does: Unrolls recursion over type-level tuples (e.g., field labels/types) at compile time. ** 2) Summoning Labels:** val labels = summonLabels[p.MirroredElemLabels] p.MirroredElemLabels is a type-level representation of the names of the fields in the case class T. The summonLabels method retrieves these names as a list of strings. This allows us to know what keys to use in the JSON output. // Helper to summon field labels private inline def summonLabels[T Nil case _: (head *: tail) => constValue[head].toString :: summonLabels[tail] } summonLabels iterates over the type-level tuple of field labels (e.g., ("name", "age") for Person case class). inline ensures th
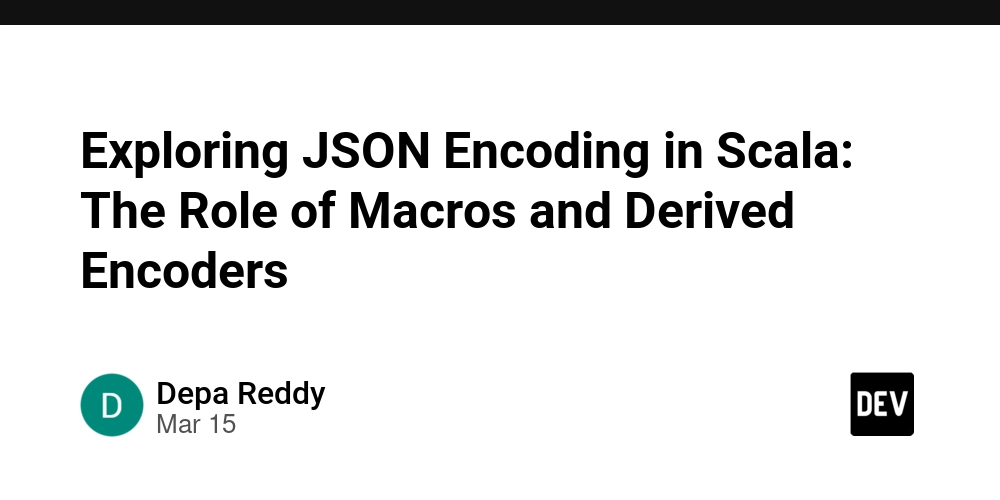
the entire source code user here can be found at below gist.
https://gist.github.com/depareddy/ce6f1500af400f69752beb5a9da05bbf
An encoder is like a helper that takes case class turns it into this special code (JSON format).case class in scala can defined like below.
case class Person(name: String, age: Int)
In our code, we have a special helper called JsonEncoder:
JsonEncoder Trait: The JsonEncoder trait defines a type class for encoding values into JSON strings:
trait JsonEncoder[T] {
def encode(value: T): String
}
This trait allows for polymorphic behavior, enabling different types to be encoded in a consistent manner.
Primitive Type Encoders: Implicit encoders for primitive types are defined using given:
given stringEncoder: JsonEncoder[String] with
def encode(value: String): String = s""""$value""""
given intEncoder: JsonEncoder[Int] with
def encode(value: Int): String = value.toString
given booleanEncoder: JsonEncoder[Boolean] with
def encode(value: Boolean): String = value.toString
List Encoder: A generic encoder for lists is provided, which recursively uses the element encoder:
given listEncoder[T](using encoder: JsonEncoder[T]): JsonEncoder[List[T]] with
def encode(list: List[T]): String =
list.map(encoder.encode).mkString("[", ", ", "]")
inline for Compile-Time Resolution
What it does: Forces the compiler to evaluate expressions or patterns at compile time instead of runtime.
inline: This keyword indicates that the method can be inlined by the compiler, which can lead to performance optimizations. It allows the compiler to replace calls to this method with the method's body at compile time.
Let's break down the below given instance in detail, focusing on how it works to automatically generate JSON encoders for case classes in Scala 3. This part of the code is crucial for the functionality of the JSON encoding mechanism.
inline given derived[T](using m: Mirror.Of[T]): JsonEncoder[T] = new JsonEncoder[T] {
def encode(value: T): String = {
inline m match {
case p: Mirror.ProductOf[T] =>
val labels = summonLabels[p.MirroredElemLabels]
println(s"labels:$labels")
val encoders = summonEncoders[p.MirroredElemTypes]
val values = value.asInstanceOf[Product].productIterator.toList
val encodedFields = (labels, encoders, values).zipped.map {
(label, encoder, value) =>
s""""$label": ${encoder.asInstanceOf[JsonEncoder[Any]].encode(value)}"""
}
s"{${encodedFields.mkString(", ")}}"
case _ => throw new IllegalArgumentException(s"Unsupported type: ${m.toString}")
}
}
}
The derived method automates the creation of type class instances (e.g., JsonEncoder[Person]) for case classes/sealed traits by leveraging compile-time metaprogramming.
The Compiler Calls derived
When you write
val perJson = encode(per) // Requires JsonEncoder[Person]
The compiler:
Looks for a given JsonEncoder[Person] in scope.
If none exists, it tries to derive one using the above derived method
1)Inline Given Instance:
inline given derived[T] (using m: Mirror.Of[T]): JsonEncoder[T]
Inline Given Instance:
This defines an implicit instance of JsonEncoder[T] for any type T that has a corresponding Mirror.
using m: Mirror.Of[T]: This means that the method requires an implicit Mirror for type T. The Mirror provides reflection capabilities to inspect the structure of the case class.
The Mirror.Of[T] type provides compile-time metadata about T (e.g., field names/types for case classes).
Mirror.Of[T] is a type class automatically generated by the Scala 3 compiler for case classes/sealed traits.
Is it a case class (product) or sealed trait (sum)?
it tells What are the field names and types (for case classes)?
similarly What are the subtypes (for sealed traits)?
The compiler generates:
Mirror.ProductOf[Person] {
type MirroredElemLabels = ("name", "age")
type MirroredElemTypes = (String, Int)
}
inline match on m ensures the compiler checks if T is a case class (product type) or a sum type (e.g., sealed trait) during compilation.
Without inline, this check would happen at runtime, leading to errors or inefficiency.
inline for Recursive Type-Level Computations
What it does: Unrolls recursion over type-level tuples (e.g., field labels/types) at compile time.
**
2) Summoning Labels:**
val labels = summonLabels[p.MirroredElemLabels]
p.MirroredElemLabels is a type-level representation of the names of the fields in the case class T. The summonLabels method retrieves these names as a list of strings.
This allows us to know what keys to use in the JSON output.
// Helper to summon field labels
private inline def summonLabels[T <: Tuple]: List[String] = inline erasedValue[T] match {
case _: EmptyTuple => Nil
case _: (head *: tail) => constValue[head].toString :: summonLabels[tail]
}
summonLabels iterates over the type-level tuple of field labels (e.g., ("name", "age") for Person case class).
inline ensures the compiler recursively resolves each label to a String and builds a List[String] during compilation.
Without inline, the recursion would fail because type-level tuples exist only at compile time.
erasedValue and Type Safety
erasedValue[T] Creates a "fake" value of type T that exists only at compile time.This is necessary because in Scala, pattern matching typically works on values, not types. By using erasedValue, the compiler can inspect the structure of T.
The compiler pretends to create a runtime value of type T (e.g., ("name", "age")), even though T is a type.
This "fake" value is used to pattern-match on the structure of T.
The compiler inspects the structure of T (e.g., is it a tuple?).
For a tuple T = ("name", "age"):
Match head *: tail, where head = "name", tail = ("age").
Extract head as a value using constValuehead.
Recurse on tail to process "age".
For Person, the compiler:
Materializes T = ("name", "age") via erasedValue[T].
Matches head *: tail to decompose the tuple.
Extracts "name" and "age" using constValue.
Returns List("name", "age").
3) Summoning Encoders:
val encoders = summonEncoders[p.MirroredElemTypes]
Similar to labels, p.MirroredElemTypes provides the types of the fields in the case class. The summonEncoders method retrieves the corresponding JsonEncoder instances for each field type.
This is crucial for encoding each field correctly based on its type.
// Helper to summon encoders for each field type
private inline def summonEncoders[T <: Tuple]: List[JsonEncoder[_]] =
inline erasedValue[T] match {
case _: EmptyTuple => Nil
case _: (t *: ts) => summonInline[JsonEncoder[t]] :: summonEncoders[ts]
}
Usage:
val encoder: JsonEncoder[String] =summonInline[JsonEncoder[String]]
Retrieves the JsonEncoder[String] instance at compile time.
If no instance exists, compilation fails.
Recursively summons encoders for each type in a tuple T.
Example: For T = (String, Int), it returns List(JsonEncoder[String], JsonEncoder[Int]).
SummonEncoders uses summonInline to fetch encoders for String and Int.If an encoder for String or Int is missing, compilation fails immediately.
Why Use summonInline Over summon?
Guaranteed Compile-Time Resolution:
summon might resolve instances at runtime in generic contexts, but summonInline forces resolution during compilation.
Integration with inline:Required for code generation in inline methods (e.g., deriving JSON encoders for case classes).
Example: Deriving a JSON Encoder
// Derive encoder for Person
given JsonEncoder[Person] with {
def encode(p: Person): String = {
val labels = summonLabels[Person.derived.MirroredElemLabels] // ("name", "age")
val encoders = summonEncoders[Person.derived.MirroredElemTypes] // (JsonEncoder[String], JsonEncoder[Int])
// Use labels/encoders to generate JSON
}
}
4) Extracting Values:
val values = value.asInstanceOf[Product].productIterator.toList
The value is cast to Product, which is the base trait for all case classes. The productIterator method returns an iterator over the fields of the case class.
The toList method converts this iterator into a list of values, which corresponds to the fields of the case class.
5) Encoding Fields:
val encodedFields = (labels, encoders, values).zipped.map {
(label, encoder, value) =>
s""""$label": ${encoder.asInstanceOf[JsonEncoder[Any]].encode(value)}"""
}
The zipped method combines the three lists: labels, encoders, and values into tuples. Each tuple contains a label, an encoder, and a value.
The map function iterates over these tuples, using the encoder to encode each value and formatting it as a JSON key-value pair.
The result is a list of strings representing the encoded fields.
5) Constructing the JSON Object:
s"{${encodedFields.mkString(", ")}}"
Finally, the encoded fields are joined together with commas and wrapped in curly braces to form a valid JSON object.
6) Full Derivation Flow
Request an Encoder:
encode(Person("Alice", 25)) // Requires JsonEncoder[Person]
1) Compiler Searches for JsonEncoder[Person]:
2) Checks implicit/given instances in scope.
3) Falls back to JsonEncoder.derived if none found.
4) Compiler Derives the Encoder:
5) Generates a Mirror.Of[Person].
6) Calls derived[Person] with the Mirror instance.
7) Uses inline to detect Person is a product type (case class).
8) Recursively resolves field labels ("name", "age") and types (String, Int).
9) Summons JsonEncoder[String] and JsonEncoder[Int] at compile time.
10) Generates code to serialize Person as {"name": "value", "age": 21}.