End-to-End Testing in Angular with Cypress
End-to-end (E2E) testing is essential for ensuring your Angular applications behave correctly in real-world scenarios. Cypress is a modern, powerful testing tool that simplifies E2E testing. This guide covers how to effectively set up and use Cypress for testing your Angular apps. What is Cypress? Cypress is a JavaScript-based front-end testing tool designed to make E2E testing fast, reliable, and intuitive. It offers real-time reloading, automatic waiting, and easy debugging. Setting Up Cypress with Angular Step 1: Install Cypress First, install Cypress as a development dependency: npm install cypress --save-dev Step 2: Open Cypress Run Cypress to generate the initial setup: npx cypress open This command creates a cypress folder with configuration and example tests. Step 3: Configure Cypress for Angular In your Angular app, ensure the base URL is set correctly in cypress.json: { "baseUrl": "http://localhost:4200" } Writing Your First Cypress Test Create a simple test in cypress/integration/example.spec.js: describe('Angular app E2E test', () => { it('Visits the homepage', () => { cy.visit('/'); cy.contains('Welcome'); }); }); Run the Angular app: ng serve Open Cypress again to run your test: npx cypress open Cypress Features Automatic Waiting Cypress automatically waits for elements to become available, eliminating flaky tests: cy.get('.button').click(); Real-time Reloading Cypress instantly reloads tests as you edit, speeding up development and testing cycles. Easy Debugging Cypress includes built-in debugging tools, including snapshots and logs: cy.get('input').type('Cypress debugging'); cy.screenshot(); Advanced Cypress Testing Tips Custom Commands Create reusable custom commands in cypress/support/commands.js: Cypress.Commands.add('login', (email, password) => { cy.visit('/login'); cy.get('#email').type(email); cy.get('#password').type(password); cy.get('form').submit(); }); Then use your custom commands: cy.login('user@example.com', 'password'); Environment Variables Set up environment variables for dynamic testing scenarios: // cypress.json { "env": { "apiUrl": "https://api.example.com" } } Access environment variables in tests: cy.request(Cypress.env('apiUrl') + '/endpoint'); Best Practices Write small, focused tests for reliability and ease of debugging. Regularly run E2E tests in your CI/CD pipeline. Mock API responses to isolate frontend issues. Conclusion Integrating Cypress into your Angular testing workflow significantly enhances your application's quality, reliability, and user experience. With Cypress, E2E testing becomes simpler, more powerful, and more effective. Have you used Cypress for Angular E2E testing? Share your experiences and tips below!
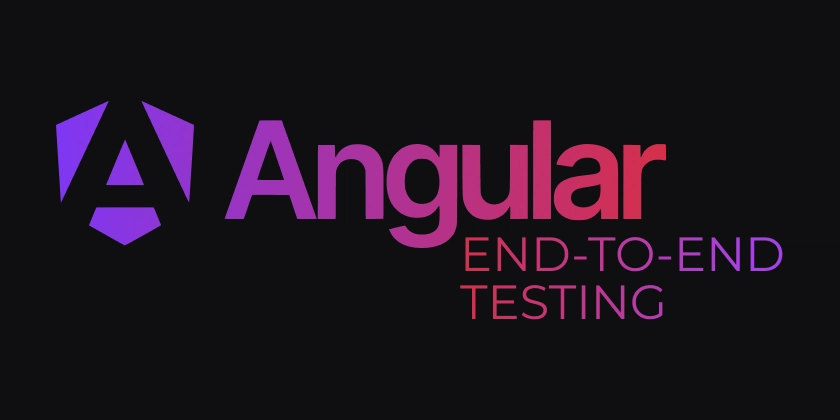
End-to-end (E2E) testing is essential for ensuring your Angular applications behave correctly in real-world scenarios. Cypress is a modern, powerful testing tool that simplifies E2E testing. This guide covers how to effectively set up and use Cypress for testing your Angular apps.
What is Cypress?
Cypress is a JavaScript-based front-end testing tool designed to make E2E testing fast, reliable, and intuitive. It offers real-time reloading, automatic waiting, and easy debugging.
Setting Up Cypress with Angular
Step 1: Install Cypress
First, install Cypress as a development dependency:
npm install cypress --save-dev
Step 2: Open Cypress
Run Cypress to generate the initial setup:
npx cypress open
This command creates a cypress
folder with configuration and example tests.
Step 3: Configure Cypress for Angular
In your Angular app, ensure the base URL is set correctly in cypress.json
:
{
"baseUrl": "http://localhost:4200"
}
Writing Your First Cypress Test
Create a simple test in cypress/integration/example.spec.js
:
describe('Angular app E2E test', () => {
it('Visits the homepage', () => {
cy.visit('/');
cy.contains('Welcome');
});
});
Run the Angular app:
ng serve
Open Cypress again to run your test:
npx cypress open
Cypress Features
Automatic Waiting
Cypress automatically waits for elements to become available, eliminating flaky tests:
cy.get('.button').click();
Real-time Reloading
Cypress instantly reloads tests as you edit, speeding up development and testing cycles.
Easy Debugging
Cypress includes built-in debugging tools, including snapshots and logs:
cy.get('input').type('Cypress debugging');
cy.screenshot();
Advanced Cypress Testing Tips
Custom Commands
Create reusable custom commands in cypress/support/commands.js
:
Cypress.Commands.add('login', (email, password) => {
cy.visit('/login');
cy.get('#email').type(email);
cy.get('#password').type(password);
cy.get('form').submit();
});
Then use your custom commands:
cy.login('user@example.com', 'password');
Environment Variables
Set up environment variables for dynamic testing scenarios:
// cypress.json
{
"env": {
"apiUrl": "https://api.example.com"
}
}
Access environment variables in tests:
cy.request(Cypress.env('apiUrl') + '/endpoint');
Best Practices
- Write small, focused tests for reliability and ease of debugging.
- Regularly run E2E tests in your CI/CD pipeline.
- Mock API responses to isolate frontend issues.
Conclusion
Integrating Cypress into your Angular testing workflow significantly enhances your application's quality, reliability, and user experience. With Cypress, E2E testing becomes simpler, more powerful, and more effective.
Have you used Cypress for Angular E2E testing? Share your experiences and tips below!