Designing Scalable RESTful APIs for Dairy Farm Intelligence: FarmersMilk API Architecture
Introduction: The Heart of FarmersMilk Imagine running a dairy farm where everything—from tracking each buffalo’s milk production to delivering fresh milk to customers—works together seamlessly through a digital system. That’s the dream behind FarmersMilk, a platform I’m building as its sole developer and architect. At the core of this system are APIs (Application Programming Interfaces), built using .NET Core 8.0, that act like the glue connecting all the pieces: the animals, the farmers, the delivery drivers, the customers, and even smart AI agents. These APIs are the digital heartbeat of FarmersMilk. They let farmers register new buffaloes, log daily milk yields, verify deliveries with QR codes, and even trigger AI to figure out the fastest delivery routes. Whether it’s a farmer checking stats on a mobile app or a customer confirming their milk drop-off, every action flows through these APIs. They’re not just code—they’re what makes this smart dairy farm tick. API Architecture Overview: A Solid Foundation To make FarmersMilk reliable and easy to grow, I’ve built the API with .NET Core 8.0 using a layered structure. Think of it like a well-organized farmhouse with different floors, each handling a specific job: •**Controllers:** These are the front doors. They welcome requests (like “Hey, record this milk yield”) from apps or websites and send them to the right place. •**Services:** This is where the real work happens. Services handle the farm’s rules—like making sure milk amounts make sense—before anything gets saved. •**Repositories:** These are the record keepers, talking to the database (using Entity Framework Core) to store or fetch data, like a Buffalo’s health history. This setup keeps things tidy. If I need to tweak how data is stored, I can adjust the repositories without messing up the services or controllers. It’s like fixing the basement without disturbing the kitchen. I’ve also stuck to REST principles, which are like a universal language for APIs: •GET: To grab info, like a buffalo’s details. •POST: To add new stuff, like a milk record. •PUT: To update things, like a delivery status. •DELETE: To remove outdated entries, like a retired buffalo. Here’s a diagram to picture it: And here’s how the layers fit together: Key API Endpoints: The Everyday Tools Here are some of the main APIs that keep FarmersMilk running smoothly, with examples of what they do: 1.Buffalo Registration (POST /api/buffalo) This is how a new buffalo joins the farm. Farmers send details like breed and RFID tag, and the system saves them. Example Request: JSON POST /api/buffalo { "breed": "Murrah", "rfidTag": "123456789", "healthStatus": "Healthy" } 2.Milk Record (POST /api/milk/record) Every milking session gets logged here—how much milk, which buffalo, and when. It’s key for tracking productivity. Example Request: JSON POST /api/milk/record { "animalId": 1, "liters": 5.5, "timestamp": "2023-10-01T08:00:00Z" } 3.QR Code Verification (POST /api/qr/scan) When milk reaches a customer, they scan a QR code. This API confirms the delivery and logs it. Example Request: JSON POST /api/qr/scan { "deliveryId": 1001, "qrCode": "ABC123", "scanTime": "2023-10-01T09:30:00Z", "location": {"lat": 12.34, "lon": 56.78} } 4.Delivery Status Update (PUT /api/delivery/update) Drivers update this as they go—think “In Transit” or “Delivered”—so everyone knows what’s happening. Example Request: JSON PUT /api/delivery/update { "deliveryId": 1001, "status": "Delivered" } 5.AI Route Optimization Trigger (GET /api/route/optimize) This kicks off the AI to find the best delivery routes based on orders and GPS data. Example Request: JSON GET /api/route/optimize These endpoints are the workhorses of the platform, making sure every task gets done efficiently. Security Measures: Keeping It Safe Farm data and customer info need to stay secure, so I’ve locked down the API with JSON Web Tokens (JWT). Here’s how it works: •Login: Users (farmers, drivers, admins) log in, and if their credentials check out, they get a JWT token—a digital ID card. •Token Check: Every API request includes this token. Middleware (a gatekeeper) verifies it before letting the request through. •Roles: The token says who you are—like “Admin” or “Driver”—and what you can do. Only admins can delete buffaloes, while drivers can update deliveries. Here’s a code snippet showing how it’s enforced: C# Code [Authorize(Roles = "Admin")] [HttpDelete("/api/buffalo/{id}")] public IActionResult DeleteBuffalo(int id) { _buffaloService.DeleteBuffalo(id); return Ok("Buffalo deleted"); } Only admins get past that [Authorize] line. It’s like a “Staff Only” sign for the API. Sample Code Snippets: A Peek Under the Hood Let
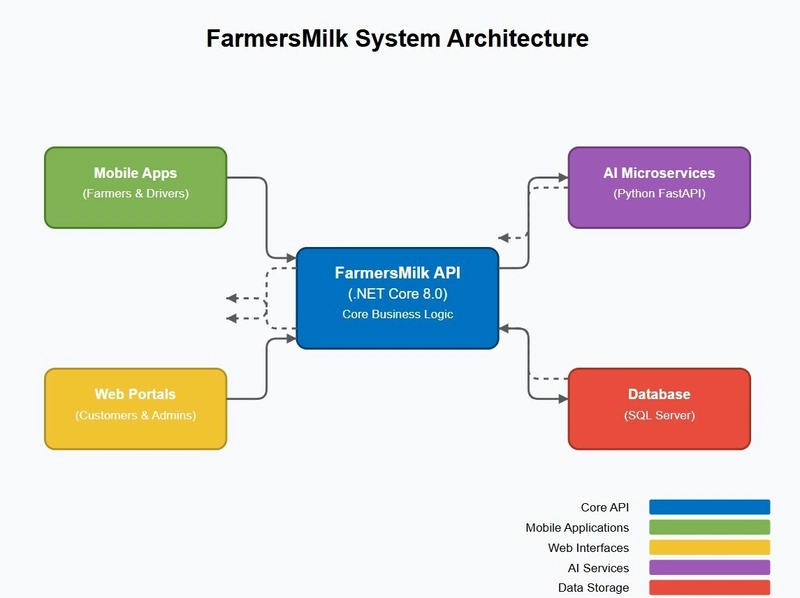
Introduction: The Heart of FarmersMilk
Imagine running a dairy farm where everything—from tracking each buffalo’s milk production to delivering fresh milk to customers—works together seamlessly through a digital system. That’s the dream behind FarmersMilk, a platform I’m building as its sole developer and architect. At the core of this system are APIs (Application Programming Interfaces), built using .NET Core 8.0, that act like the glue connecting all the pieces: the animals, the farmers, the delivery drivers, the customers, and even smart AI agents.
These APIs are the digital heartbeat of FarmersMilk. They let farmers register new buffaloes, log daily milk yields, verify deliveries with QR codes, and even trigger AI to figure out the fastest delivery routes. Whether it’s a farmer checking stats on a mobile app or a customer confirming their milk drop-off, every action flows through these APIs. They’re not just code—they’re what makes this smart dairy farm tick.
API Architecture Overview: A Solid Foundation
To make FarmersMilk reliable and easy to grow, I’ve built the API with .NET Core 8.0 using a layered structure. Think of it like a well-organized farmhouse with different floors, each handling a specific job:
•**Controllers:** These are the front doors. They welcome requests
(like “Hey, record this milk yield”) from apps or websites and
send them to the right place.
•**Services:** This is where the real work happens. Services handle
the farm’s rules—like making sure milk amounts make sense—before
anything gets saved.
•**Repositories:** These are the record keepers, talking to the
database (using Entity Framework Core) to store or fetch data,
like a Buffalo’s health history.
This setup keeps things tidy. If I need to tweak how data is stored, I can adjust the repositories without messing up the services or controllers. It’s like fixing the basement without disturbing the kitchen.
I’ve also stuck to REST principles, which are like a universal language for APIs:
•GET: To grab info, like a buffalo’s details.
•POST: To add new stuff, like a milk record.
•PUT: To update things, like a delivery status.
•DELETE: To remove outdated entries, like a retired buffalo.
Here’s a diagram to picture it:
And here’s how the layers fit together:
Key API Endpoints: The Everyday Tools
Here are some of the main APIs that keep FarmersMilk running smoothly, with examples of what they do:
1.Buffalo Registration (POST /api/buffalo)
This is how a new buffalo joins the farm. Farmers send details like breed and RFID tag, and the system saves them.
Example Request:
JSON
POST /api/buffalo
{
"breed": "Murrah",
"rfidTag": "123456789",
"healthStatus": "Healthy"
}
2.Milk Record (POST /api/milk/record)
Every milking session gets logged here—how much milk, which buffalo, and when. It’s key for tracking productivity.
Example Request:
JSON
POST /api/milk/record
{
"animalId": 1,
"liters": 5.5,
"timestamp": "2023-10-01T08:00:00Z"
}
3.QR Code Verification (POST /api/qr/scan)
When milk reaches a customer, they scan a QR code. This API confirms the delivery and logs it.
Example Request:
JSON
POST /api/qr/scan
{
"deliveryId": 1001,
"qrCode": "ABC123",
"scanTime": "2023-10-01T09:30:00Z",
"location": {"lat": 12.34, "lon": 56.78}
}
4.Delivery Status Update (PUT /api/delivery/update)
Drivers update this as they go—think “In Transit” or “Delivered”—so everyone knows what’s happening.
Example Request:
JSON
PUT /api/delivery/update
{
"deliveryId": 1001,
"status": "Delivered"
}
5.AI Route Optimization Trigger (GET /api/route/optimize)
This kicks off the AI to find the best delivery routes based on orders and GPS data.
Example Request:
JSON
GET /api/route/optimize
These endpoints are the workhorses of the platform, making sure every task gets done efficiently.
Security Measures: Keeping It Safe
Farm data and customer info need to stay secure, so I’ve locked down the API with JSON Web Tokens (JWT). Here’s how it works:
•Login: Users (farmers, drivers, admins) log in, and if their
credentials check out, they get a JWT token—a digital ID card.
•Token Check: Every API request includes this token. Middleware
(a gatekeeper) verifies it before letting the request through.
•Roles: The token says who you are—like “Admin” or “Driver”—and
what you can do. Only admins can delete buffaloes, while drivers
can update deliveries.
Here’s a code snippet showing how it’s enforced:
C# Code
[Authorize(Roles = "Admin")]
[HttpDelete("/api/buffalo/{id}")]
public IActionResult DeleteBuffalo(int id)
{
_buffaloService.DeleteBuffalo(id);
return Ok("Buffalo deleted");
}
Only admins get past that [Authorize] line. It’s like a “Staff Only” sign for the API.
Sample Code Snippets: A Peek Under the Hood
Let’s look at some code to see how this all comes together.
Milk Controller Example
This handles milk-related requests:
C# Code
[ApiController]
[Route("api/milk")]
public class MilkController : ControllerBase
{
private readonly IMilkService _milkService;
public MilkController(IMilkService milkService)
{
_milkService = milkService;
}
[HttpPost("record")]
public IActionResult AddMilkRecord([FromBody] MilkInputModel input)
{
if (input.Liters <= 0 || input.AnimalId == 0)
{
return BadRequest("Invalid data: Liters must be positive and AnimalId required.");
}
_milkService.AddMilkRecord(input);
return Ok("Milk record saved!");
}
[HttpGet("yield/{animalId}")]
public IActionResult GetMilkYield(int animalId)
{
var yield = _milkService.GetMilkYield(animalId);
return Ok(yield);
}
}
The controller uses MilkService (injected via dependency injection) to do the heavy lifting.
Milk Service Example
Here’s how the service processes that data:
C# Code
public class MilkService : IMilkService
{
private readonly ApplicationDbContext _context;
public MilkService(ApplicationDbContext context)
{
_context = context;
}
public void AddMilkRecord(MilkInputModel input)
{
var record = new MilkRecord
{
AnimalId = input.AnimalId,
Liters = input.Liters,
Timestamp = input.Timestamp ?? DateTime.UtcNow
};
_context.MilkRecords.Add(record);
_context.SaveChanges();
}
public decimal GetMilkYield(int animalId)
{
return _context.MilkRecords
.Where(m => m.AnimalId == animalId)
.Sum(m => m.Liters);
}
}
And in Program.cs, I tell the system to provide MilkService when needed:
builder.Services.AddScoped();
This keeps the code clean and reusable.
Real-Time Communication: Teaming Up with AI
The APIs don’t work alone—they team up with AI agents I built in Python using FastAPI. For example:
•**Health Alerts**: If the AI spots trouble (like a buffalo producing less milk), it sends:
JSON CODE
POST /api/health/alert
{
"animalId": 1,
"alertType": "LowMilkYield",
"description": "Milk yield dropped 30% in 3 days."
}
•Route Updates: After optimizing routes, the AI updates the system:
JSON CODE
PUT /api/route/update
{
"driverId": 5,
"route": [
{"customerId": 101, "address": "123 Farm Lane"},
{"customerId": 102, "address": "456 Country Rd"}
]
}
In the future, I’ll add Webhooks and SignalR for instant updates—like live delivery tracking.
Testing & Scalability: Ready for Growth
To keep the API solid and scalable:
•Testing: I use xUnit for unit tests (checking each piece works) and
Postman to test the full API flow. Example test:
C# Code
[Fact]
public void AddMilkRecord_ShouldSave()
{
var context = new ApplicationDbContext(new DbContextOptionsBuilder().UseInMemoryDatabase("TestDb").Options);
var service = new MilkService(context);
var input = new MilkInputModel { AnimalId = 1, Liters = 5.0 };
service.AddMilkRecord(input);
Assert.Equal(5.0, context.MilkRecords.First().Liters);
}
Scalability: Async database calls, lightweight DTOs, and Azure App Services with auto-scaling handle up to 10,000 requests daily without breaking a sweat.
Conclusion: The Digital Dairy Future
The FarmersMilk API isn’t just tech—it’s the backbone of a smarter dairy farm. It ties everything together, from milk records to delivery routes, making the farm more efficient and profitable. As I add more features—like better AI or slicker apps—this API will keep it all connected and growing smoothly.