Day 7: polymorphism method overloading
What is method overloading? Method Overloading is when a class has multiple methods with the same name but different parameters (different type, number, or order of parameters). Why: To increase code readability and reusability by performing similar operations with different inputs. When is Method Overloading used? when: When we need to perform similar operations with different types or numbers of inputs. Example: In a calculator class: add(int a, int b) add(double a, double b) add(int a, int b, int c) All methods are named add but work with different arguments. Which methods can be overloaded?#$ Any instance or static method can be overloaded, but constructors can also be overloaded. Method overloading is not based on return type — only the method signature (name + parameters). Real-Time Java Program: ATM Balance Checker (Method Overloading) class ATM { // Method 1: Check balance using account number void checkBalance(int accountNumber) { System.out.println("Welcome to the ATM."); System.out.println("Account Number: " + accountNumber); System.out.println("Balance: ₹25,000"); System.out.println("----------------------------------"); } // Method 2: Check balance using account number and PIN void checkBalance(int accountNumber, int pin) { if (pin == 1234) { System.out.println("Authentication Successful!"); System.out.println("Account Number: " + accountNumber); System.out.println("Balance: ₹25,000"); } else { System.out.println("Invalid PIN. Please try again."); } System.out.println("----------------------------------"); } // Method 3: Check balance using card number void checkBalance(String cardNumber) { System.out.println("Card Number: " + cardNumber); System.out.println("Balance: ₹25,000"); System.out.println("----------------------------------"); } } public class BankSystem { public static void main(String[] args) { ATM atm = new ATM(); // Using account number only atm.checkBalance(100112233); // Using account number and correct PIN atm.checkBalance(100112233, 1234); // Using account number and wrong PIN atm.checkBalance(100112233, 1111); // Using card number atm.checkBalance("CARD456789123"); } } Output: Welcome to the ATM. Account Number: 100112233 Balance: ₹25,000 ---------------------------------- Authentication Successful! Account Number: 100112233 Balance: ₹25,000 ---------------------------------- Invalid PIN. Please try again. ---------------------------------- Card Number: CARD456789123 Balance: ₹25,000 ---------------------------------- This real-time simulation shows how the same method name checkBalance() behaves differently based on the input. This is a practical use of Method Overloading.
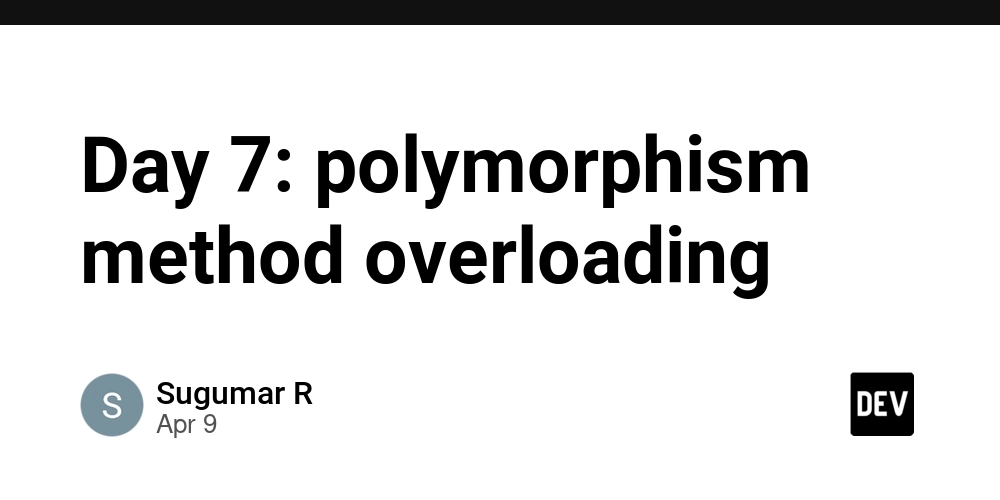
What is method overloading?
Method Overloading is when a class has multiple methods with the same name but different parameters (different type, number, or order of parameters).
Why:
To increase code readability and reusability by performing similar operations with different inputs.
When is Method Overloading used?
when:
When we need to perform similar operations with different types or numbers of inputs.
Example:
In a calculator class:
add(int a, int b)
add(double a, double b)
add(int a, int b, int c)
All methods are named add but work with different arguments.
Which methods can be overloaded?#$
Any instance or static method can be overloaded, but constructors can also be overloaded.
Method overloading is not based on return type — only the method signature (name + parameters).
Real-Time Java Program: ATM Balance Checker (Method Overloading)
class ATM {
// Method 1: Check balance using account number
void checkBalance(int accountNumber) {
System.out.println("Welcome to the ATM.");
System.out.println("Account Number: " + accountNumber);
System.out.println("Balance: ₹25,000");
System.out.println("----------------------------------");
}
// Method 2: Check balance using account number and PIN
void checkBalance(int accountNumber, int pin) {
if (pin == 1234) {
System.out.println("Authentication Successful!");
System.out.println("Account Number: " + accountNumber);
System.out.println("Balance: ₹25,000");
} else {
System.out.println("Invalid PIN. Please try again.");
}
System.out.println("----------------------------------");
}
// Method 3: Check balance using card number
void checkBalance(String cardNumber) {
System.out.println("Card Number: " + cardNumber);
System.out.println("Balance: ₹25,000");
System.out.println("----------------------------------");
}
}
public class BankSystem {
public static void main(String[] args) {
ATM atm = new ATM();
// Using account number only
atm.checkBalance(100112233);
// Using account number and correct PIN
atm.checkBalance(100112233, 1234);
// Using account number and wrong PIN
atm.checkBalance(100112233, 1111);
// Using card number
atm.checkBalance("CARD456789123");
}
}
Output:
Welcome to the ATM.
Account Number: 100112233
Balance: ₹25,000
----------------------------------
Authentication Successful!
Account Number: 100112233
Balance: ₹25,000
----------------------------------
Invalid PIN. Please try again.
----------------------------------
Card Number: CARD456789123
Balance: ₹25,000
----------------------------------
This real-time simulation shows how the same method name checkBalance() behaves differently based on the input. This is a practical use of Method Overloading.