DAY -5 JAVA METHODS
Java Methods A method in Java is a block of code that performs a specific task. It helps in code reusability and modularity. Types of Methods in Java Predefined Methods (Built-in methods like Math.sqrt(), System.out.println()) User-defined Methods (Methods created by the user) Static Methods (Methods that belong to a class, not to instances of a class) Instance Methods (Methods that belong to an instance of a class) Parameterized Methods (Methods with parameters) Method Overloading (Multiple methods with the same name but different parameters) Method Overriding (Redefining a method in a subclass) Basic Example of a Java Method public class Example { // User-defined method static void greet() { System.out.println("Hello, welcome to Java!"); } public static void main(String[] args) { greet(); // Calling the method } } Output: Hello, welcome to Java! Parameterized Method public class SumCalculator { // Method with parameters static int add(int a, int b) { return a + b; } public static void main(String[] args) { int result = add(5, 10); // Calling the method System.out.println("Sum: " + result); } } Output: Sum: 15 Method Overloading Example public class OverloadingExample { // Method with two int parameters static int multiply(int a, int b) { return a * b; } // Overloaded method with three int parameters static int multiply(int a, int b, int c) { return a * b * c; } public static void main(String[] args) { System.out.println("Multiplication (2 values): " + multiply(5, 10)); System.out.println("Multiplication (3 values): " + multiply(2, 3, 4)); } } Output: Multiplication (2 values): 50 Multiplication (3 values): 24 Method Overriding Example class Animal { void makeSound() { System.out.println("Animal makes a sound"); } } class Dog extends Animal { @override void makeSound() { System.out.println("Dog barks"); } } public class MethodOverriding { public static void main(String[] args) { Animal myDog = new Dog(); // Upcasting myDog.makeSound(); } } Output: Dog barks Static vs Instance Methods public class StaticInstanceExample { // Static method static void staticMethod() { System.out.println("Static method called"); } // Instance method void instanceMethod() { System.out.println("Instance method called"); } public static void main(String[] args) { staticMethod(); // Call without object StaticInstanceExample obj = new StaticInstanceExample(); obj.instanceMethod(); // Call with object } } Output: Static method called Instance method called Static vs Non-Static Summary Static Block and Static Variables Static Block: Executes once when the class is loaded into memory. Used to initialize static variables. Runs before the main() method or any object creation. Static Variables: Declared using the static keyword. Shared among all objects of the class (class-level variables). Initialized once when the class is loaded. Example: Static Block and Static Variable class StaticExample { static int staticVar; // Static variable // Static block static { System.out.println("Static block executed."); staticVar = 10; } public static void main(String[] args) { System.out.println("Main method executed."); System.out.println("Static Variable: " + staticVar); } } Output: Static block executed. Main method executed. Static Variable: 10 Key Points: The static block runs before the main() method. staticVar is initialized in the static block. The static variable is shared among all instances of the class. Non-Static Block and Instance Variables Non-Static Block (Instance Initializer Block): Runs before the constructor when an object is created. Used to initialize instance variables. Executes each time an object is instantiated. Instance Variables: Defined without the static keyword. Each object has its own copy of the variable. Example: Non-Static Block and Instance Variables class NonStaticExample { int instanceVar; // Instance variable // Non-static block { System.out.println("Non-static block executed."); instanceVar = 20; } // Constructor NonStaticExample() { System.out.println("Constructor executed."); } public static void main(String[] args) { System.out.println("Main method executed."); NonStaticExample obj1 = new NonStaticExample(); NonStaticExample obj2 = new NonStaticExample(); System.out.println("Instance Variable obj1: " + obj1.instanceVar); System.out.println("Instance Variable obj2: " + obj2.instanceVar); } } Output: Main method executed. Non-static block executed. Constructor executed. Non-static block executed. Constructor executed. Instance Variable obj1: 20 Instance Variable obj2: 20 Key Points: Non-static blocks execute every time an object is created (before the c
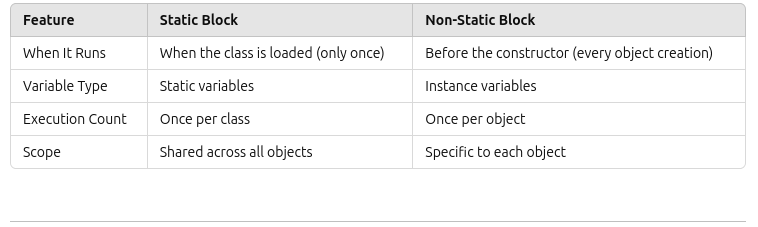
Java Methods
A method in Java is a block of code that performs a specific task. It helps in code reusability and modularity.
Types of Methods in Java
Predefined Methods (Built-in methods like Math.sqrt(), System.out.println())
User-defined Methods (Methods created by the user)
Static Methods (Methods that belong to a class, not to instances of a class)
Instance Methods (Methods that belong to an instance of a class)
Parameterized Methods (Methods with parameters)
Method Overloading (Multiple methods with the same name but different parameters)
Method Overriding (Redefining a method in a subclass)
Basic Example of a Java Method
public class Example {
// User-defined method
static void greet() {
System.out.println("Hello, welcome to Java!");
}
public static void main(String[] args) {
greet(); // Calling the method
}
}
Output:
Hello, welcome to Java!
Parameterized Method
public class SumCalculator {
// Method with parameters
static int add(int a, int b) {
return a + b;
}
public static void main(String[] args) {
int result = add(5, 10); // Calling the method
System.out.println("Sum: " + result);
}
}
Output:
Sum: 15
Method Overloading Example
public class OverloadingExample {
// Method with two int parameters
static int multiply(int a, int b) {
return a * b;
}
// Overloaded method with three int parameters
static int multiply(int a, int b, int c) {
return a * b * c;
}
public static void main(String[] args) {
System.out.println("Multiplication (2 values): " + multiply(5, 10));
System.out.println("Multiplication (3 values): " + multiply(2, 3, 4));
}
}
Output:
Multiplication (2 values): 50
Multiplication (3 values): 24
Method Overriding Example
class Animal {
void makeSound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@override
void makeSound() {
System.out.println("Dog barks");
}
}
public class MethodOverriding {
public static void main(String[] args) {
Animal myDog = new Dog(); // Upcasting
myDog.makeSound();
}
}
Output:
Dog barks
Static vs Instance Methods
public class StaticInstanceExample {
// Static method
static void staticMethod() {
System.out.println("Static method called");
}
// Instance method
void instanceMethod() {
System.out.println("Instance method called");
}
public static void main(String[] args) {
staticMethod(); // Call without object
StaticInstanceExample obj = new StaticInstanceExample();
obj.instanceMethod(); // Call with object
}
}
Output:
Static method called
Instance method called
Static vs Non-Static Summary
-
Static Block and Static Variables
Static Block:Executes once when the class is loaded into memory.
Used to initialize static variables.
Runs before the main() method or any object creation.
Static Variables:
Declared using the static keyword.
Shared among all objects of the class (class-level variables).
Initialized once when the class is loaded.
Example: Static Block and Static Variable
class StaticExample {
static int staticVar; // Static variable
// Static block
static {
System.out.println("Static block executed.");
staticVar = 10;
}
public static void main(String[] args) {
System.out.println("Main method executed.");
System.out.println("Static Variable: " + staticVar);
}
}
Output:
Static block executed.
Main method executed.
Static Variable: 10
Key Points:
The static block runs before the main() method.
staticVar is initialized in the static block.
The static variable is shared among all instances of the class.
-
Non-Static Block and Instance Variables
Non-Static Block (Instance Initializer Block):Runs before the constructor when an object is created.
Used to initialize instance variables.
Executes each time an object is instantiated.
Instance Variables:
Defined without the static keyword.
Each object has its own copy of the variable.
Example: Non-Static Block and Instance Variables
class NonStaticExample {
int instanceVar; // Instance variable
// Non-static block
{
System.out.println("Non-static block executed.");
instanceVar = 20;
}
// Constructor
NonStaticExample() {
System.out.println("Constructor executed.");
}
public static void main(String[] args) {
System.out.println("Main method executed.");
NonStaticExample obj1 = new NonStaticExample();
NonStaticExample obj2 = new NonStaticExample();
System.out.println("Instance Variable obj1: " + obj1.instanceVar);
System.out.println("Instance Variable obj2: " + obj2.instanceVar);
}
}
Output:
Main method executed.
Non-static block executed.
Constructor executed.
Non-static block executed.
Constructor executed.
Instance Variable obj1: 20
Instance Variable obj2: 20
Key Points:
Non-static blocks execute every time an object is created (before the constructor).
Each object gets a new instance of the instance variable.
The constructor runs after the non-static block.