# Day 3 Learn JavaScript Basic Syntax & Principles (Beginner Friendly Guide)
Welcome back to Day 3 of our JavaScript learning journey! Today we are diving into the heart of JavaScript → The Basic Syntax & Core Principles you must know before building projects. By the end of this article, you'll write cleaner, better, and more professional JavaScript code. What is JavaScript Syntax? JavaScript syntax refers to the set of rules that defines how we write JavaScript code so that browsers can understand and execute it properly. If you break these rules → You get Errors. If you follow them → Your code runs smoothly. 1. JavaScript Syntax Rules (With Examples) // JavaScript is Case Sensitive let name = "Dhanian"; let Name = "Developer"; console.log(name); // Output: Dhanian console.log(Name); // Output: Developer // Semicolons End Statements (Optional but Recommended) let age = 25; let country = "Kenya"; // Comments Help Explain Code // Single-line Comment /* Multi-line Comment */ // Curly Braces { } Group Code if(age >= 18) { console.log("Adult"); } // White Space is Ignored let a=5; let b=10; console.log(a+b); // Output: 15 // Variable Naming Rules let $money = 1000; let _username = "Dhanian"; let user2 = "New User"; 2. JavaScript Core Principles & Best Practices Writing good code is not just about making it work — It's about making it readable and maintainable. // DRY Principle - Don't Repeat Yourself function greet(name) { console.log("Hello " + name); } greet("Dhanian"); greet("Code Lover"); // KISS Principle - Keep It Simple & Short let price = 500; let quantity = 2; let total = price * quantity; console.log(total); // Output: 1000 // YAGNI Principle - You Aren't Gonna Need It // Don't add unnecessary code // Comment Your Code for clarity // Proper Naming let studentAge = 15; // Good let x = 15; // Bad // Avoid Global Variables 3. JavaScript Output Methods & Statements JavaScript gives us multiple ways to display output or interact with users. // console.log() - Output to Console console.log("Hello World"); // alert() - Pop-up Message alert("Welcome to JavaScript"); // document.write() - Output to Webpage document.write("Hello from JavaScript"); // prompt() - Get User Input let userName = prompt("Enter your name:"); console.log("Hello " + userName); // confirm() - OK or Cancel Pop-up let isSure = confirm("Are you sure?"); console.log(isSure); // true or false // Template Literals - Modern Way let name = "Dhanian"; console.log(`Hello ${name}, Welcome!`); // Escape Characters console.log("He said: \"Learn JavaScript!\""); Final Words Today you’ve learned the core foundations of writing JavaScript properly. → Follow the syntax rules → Apply coding principles → Write clean, simple, and smart code. BONUS GIFT
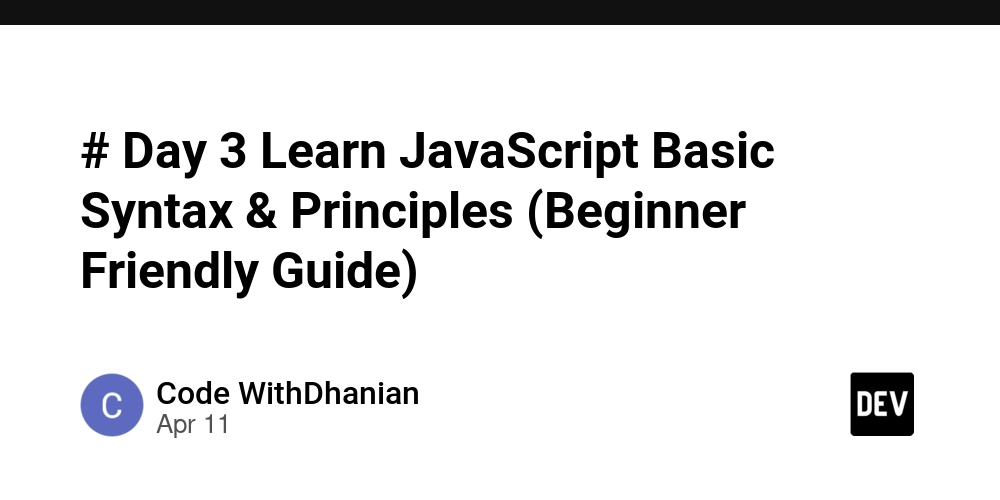
Welcome back to Day 3 of our JavaScript learning journey!
Today we are diving into the heart of JavaScript → The Basic Syntax & Core Principles you must know before building projects.
By the end of this article, you'll write cleaner, better, and more professional JavaScript code.
What is JavaScript Syntax?
JavaScript syntax refers to the set of rules that defines how we write JavaScript code so that browsers can understand and execute it properly.
If you break these rules → You get Errors.
If you follow them → Your code runs smoothly.
1. JavaScript Syntax Rules (With Examples)
// JavaScript is Case Sensitive
let name = "Dhanian";
let Name = "Developer";
console.log(name); // Output: Dhanian
console.log(Name); // Output: Developer
// Semicolons End Statements (Optional but Recommended)
let age = 25;
let country = "Kenya";
// Comments Help Explain Code
// Single-line Comment
/* Multi-line
Comment */
// Curly Braces { } Group Code
if(age >= 18) {
console.log("Adult");
}
// White Space is Ignored
let a=5; let b=10;
console.log(a+b); // Output: 15
// Variable Naming Rules
let $money = 1000;
let _username = "Dhanian";
let user2 = "New User";
2. JavaScript Core Principles & Best Practices
Writing good code is not just about making it work — It's about making it readable and maintainable.
// DRY Principle - Don't Repeat Yourself
function greet(name) {
console.log("Hello " + name);
}
greet("Dhanian");
greet("Code Lover");
// KISS Principle - Keep It Simple & Short
let price = 500;
let quantity = 2;
let total = price * quantity;
console.log(total); // Output: 1000
// YAGNI Principle - You Aren't Gonna Need It
// Don't add unnecessary code
// Comment Your Code for clarity
// Proper Naming
let studentAge = 15; // Good
let x = 15; // Bad
// Avoid Global Variables
3. JavaScript Output Methods & Statements
JavaScript gives us multiple ways to display output or interact with users.
// console.log() - Output to Console
console.log("Hello World");
// alert() - Pop-up Message
alert("Welcome to JavaScript");
// document.write() - Output to Webpage
document.write("Hello from JavaScript
");
// prompt() - Get User Input
let userName = prompt("Enter your name:");
console.log("Hello " + userName);
// confirm() - OK or Cancel Pop-up
let isSure = confirm("Are you sure?");
console.log(isSure); // true or false
// Template Literals - Modern Way
let name = "Dhanian";
console.log(`Hello ${name}, Welcome!`);
// Escape Characters
console.log("He said: \"Learn JavaScript!\"");
Final Words
Today you’ve learned the core foundations of writing JavaScript properly.
→ Follow the syntax rules
→ Apply coding principles
→ Write clean, simple, and smart code.