Populate PDF forms using JSON
When handling PDF forms in modern applications, developers often encounter challenges with the rigid binary structure of PDFs. PDF files are structured as a collection of binary objects, including complex elements like cross-reference tables, streams, and compressed data. This makes programmatically manipulating PDF forms difficult, especially for tasks like filling out form fields. To illustrate the complexity of a PDF's binary structure, here's a simplified snippet of what a PDF file looks like internally: %PDF-1.7 1 0 obj > endobj 2 0 obj > endobj 3 0 obj > >> endobj 4 0 obj > stream BT /F1 24 Tf 72 700 Td (Hello, PDF!) Tj ET endstream endobj 5 0 obj > endobj xref 0 6 0000000000 65535 f 0000000015 00000 n 0000000079 00000 n 0000000150 00000 n 0000000270 00000 n 0000000360 00000 n trailer > startxref 450 %%EOF As shown above, a PDF file consists of objects and cross-reference tables, all managed in binary format. These objects are difficult to manipulate directly and often require specialized libraries to extract or modify data. Furthermore, PDF files usually contain compressed data streams, further complicating programmatic access. Fortunately, JSON provides a flexible, human-readable alternative for populating PDF forms. In this article, we'll walk through how developers can use JSON to fill and update PDF form fields while avoiding the complexities of the traditional binary structure. We'll first cover a manual approach using open-source libraries and then introduce a more streamlined solution using the Joyfill SDK. Why Use JSON for PDF Form Population? With their binary structure, PDFs were designed for document portability and consistent rendering across platforms. However, working with binary data has challenges like complex parsing, lack of human readability, and limited flexibility. Developers have traditionally relied on specialized libraries or manual data entry to manipulate PDFs, which can be inefficient and error-prone. JSON (JavaScript Object Notation) offers a superior solution for form population, and here's why: Human-Readable and Simple: JSON is a text-based format, making it easier for developers to construct and debug compared to the binary structure of PDFs. Separation of Data and Presentation: JSON allows developers to manage data independently from the document layout, making form automation more modular and scalable. Cross-Platform Compatibility: JSON integrates seamlessly with various programming languages and platforms, allowing easy data interchange across systems. Efficiency in Automation: JSON enables developers to automate form-filling tasks at scale, making it ideal for applications requiring frequent data updates or large-scale form processing. Flexibility: JSON's structure is easy to generate and update programmatically, allowing dynamic form population without dealing with binary-encoded data. By using JSON to populate PDFs, developers gain greater flexibility, scalability, and ease of integration while avoiding the complexities of binary formats. This makes JSON a modern, efficient approach to working with PDF forms. How to Populate PDF Forms Using JSON Now, let's dive into the steps for populating PDF forms using JSON. This section will focus on using open-source libraries that don't require proprietary tools, ensuring this solution can be widely applied in different development environments. Step 1: Prepare the PDF Form First, you'll need a PDF form with named fields. This is crucial because JSON data will be mapped to the form fields by their names. If your PDF isn't set up with named fields, you can use tools like Adobe Acrobat or free PDF editors to create and label these fields. For example, in a simple PDF form, you might have fields like name, email, and date. Step 2: Structure Your JSON Data Next, you'll need to structure your JSON data to match the fields in your PDF. Each key in the JSON object corresponds to a field name in the PDF, and each value represents the data that will populate that field. Here's an example JSON structure: { "name": "John Doe", "email": "[john.doe@example.com](mailto:john.doe@example.com)", "date": "2024-09-23" } Step 3: Populate PDF Using Other Libraries Now that your JSON data is prepared, you can use libraries like pdf-lib or pdf-fill-form to map the JSON values to the corresponding PDF fields. Here's how you can do it using pdf-lib, an open-source library for working with PDFs in JavaScript: import { PDFDocument } from 'pdf-lib'; import fs from 'fs'; const pdfDoc = await PDFDocument.load(fs.readFileSync('template.pdf')); const form = pdfDoc.getForm(); const jsonData = { "name": "John Doe", "email": "[john.doe@example.com](mailto:john.doe@example.com)", "date": "2024-09-23" }; form.getTextField('name').setText([jsonData.name](http://jsondata.name/)); form.getTextField('email').setText(jsonData.email); form.getTextField('date
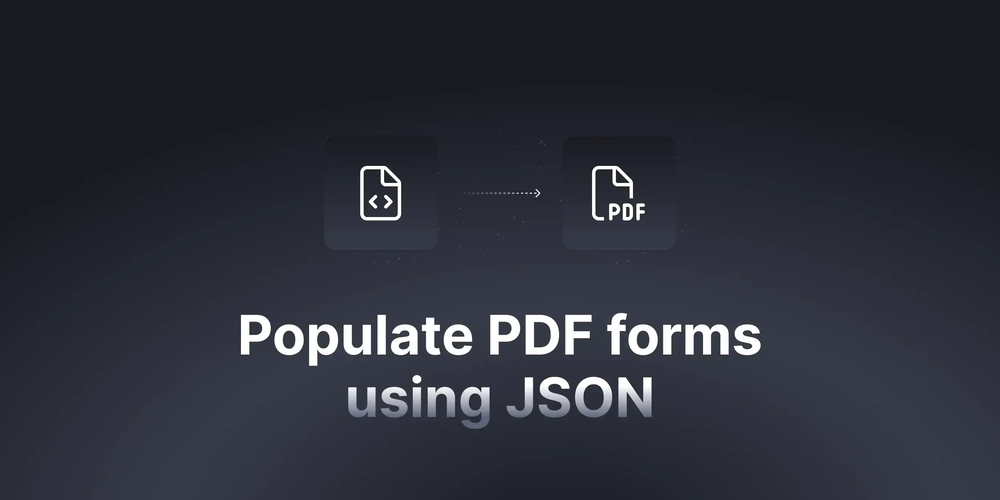
When handling PDF forms in modern applications, developers often encounter challenges with the rigid binary structure of PDFs. PDF files are structured as a collection of binary objects, including complex elements like cross-reference tables, streams, and compressed data. This makes programmatically manipulating PDF forms difficult, especially for tasks like filling out form fields.
To illustrate the complexity of a PDF's binary structure, here's a simplified snippet of what a PDF file looks like internally:
%PDF-1.7
1 0 obj
<<
/Type /Catalog
/Pages 2 0 R
>>
endobj
2 0 obj
<<
/Type /Pages
/Kids [3 0 R]
/Count 1
>>
endobj
3 0 obj
<<
/Type /Page
/Parent 2 0 R
/MediaBox [0 0 595 842]
/Contents 4 0 R
/Resources << /Font << /F1 5 0 R >> >>
>>
endobj
4 0 obj
<<
/Length 50
>>
stream
BT
/F1 24 Tf
72 700 Td
(Hello, PDF!) Tj
ET
endstream
endobj
5 0 obj
<<
/Type /Font
/Subtype /Type1
/BaseFont /Helvetica
>>
endobj
xref
0 6
0000000000 65535 f
0000000015 00000 n
0000000079 00000 n
0000000150 00000 n
0000000270 00000 n
0000000360 00000 n
trailer
<<
/Root 1 0 R
/Size 6
/ID [<42841c13bbf709d79a200fa1691836f8>]
>>
startxref
450
%%EOF
As shown above, a PDF file consists of objects and cross-reference tables, all managed in binary format. These objects are difficult to manipulate directly and often require specialized libraries to extract or modify data. Furthermore, PDF files usually contain compressed data streams, further complicating programmatic access.
Fortunately, JSON provides a flexible, human-readable alternative for populating PDF forms. In this article, we'll walk through how developers can use JSON to fill and update PDF form fields while avoiding the complexities of the traditional binary structure. We'll first cover a manual approach using open-source libraries and then introduce a more streamlined solution using the Joyfill SDK.
Why Use JSON for PDF Form Population?
With their binary structure, PDFs were designed for document portability and consistent rendering across platforms. However, working with binary data has challenges like complex parsing, lack of human readability, and limited flexibility. Developers have traditionally relied on specialized libraries or manual data entry to manipulate PDFs, which can be inefficient and error-prone.
JSON (JavaScript Object Notation) offers a superior solution for form population, and here's why:
- Human-Readable and Simple: JSON is a text-based format, making it easier for developers to construct and debug compared to the binary structure of PDFs.
- Separation of Data and Presentation: JSON allows developers to manage data independently from the document layout, making form automation more modular and scalable.
- Cross-Platform Compatibility: JSON integrates seamlessly with various programming languages and platforms, allowing easy data interchange across systems.
- Efficiency in Automation: JSON enables developers to automate form-filling tasks at scale, making it ideal for applications requiring frequent data updates or large-scale form processing.
- Flexibility: JSON's structure is easy to generate and update programmatically, allowing dynamic form population without dealing with binary-encoded data.
By using JSON to populate PDFs, developers gain greater flexibility, scalability, and ease of integration while avoiding the complexities of binary formats. This makes JSON a modern, efficient approach to working with PDF forms.
How to Populate PDF Forms Using JSON
Now, let's dive into the steps for populating PDF forms using JSON. This section will focus on using open-source libraries that don't require proprietary tools, ensuring this solution can be widely applied in different development environments.
Step 1: Prepare the PDF Form
First, you'll need a PDF form with named fields. This is crucial because JSON data will be mapped to the form fields by their names. If your PDF isn't set up with named fields, you can use tools like Adobe Acrobat or free PDF editors to create and label these fields.
For example, in a simple PDF form, you might have fields like name, email, and date.
Step 2: Structure Your JSON Data
Next, you'll need to structure your JSON data to match the fields in your PDF. Each key in the JSON object corresponds to a field name in the PDF, and each value represents the data that will populate that field.
Here's an example JSON structure:
{
"name": "John Doe",
"email": "[john.doe@example.com](mailto:john.doe@example.com)",
"date": "2024-09-23"
}
Step 3: Populate PDF Using Other Libraries
Now that your JSON data is prepared, you can use libraries like pdf-lib or pdf-fill-form to map the JSON values to the corresponding PDF fields. Here's how you can do it using pdf-lib, an open-source library for working with PDFs in JavaScript:
import { PDFDocument } from 'pdf-lib';
import fs from 'fs';
const pdfDoc = await PDFDocument.load(fs.readFileSync('template.pdf'));
const form = pdfDoc.getForm();
const jsonData = {
"name": "John Doe",
"email": "[john.doe@example.com](mailto:john.doe@example.com)",
"date": "2024-09-23"
};
form.getTextField('name').setText([jsonData.name](http://jsondata.name/));
form.getTextField('email').setText(jsonData.email);
form.getTextField('date').setText(jsonData.date);
const filledPdfBytes = await pdfDoc.save();
fs.writeFileSync('filled.pdf', filledPdfBytes);
In this example, we load the PDF form, map the JSON data to the appropriate fields, and save the filled PDF to a new file. This method works across multiple platforms and is easy to implement using JavaScript.
Step 4: Export the PDF
After populating the form fields with your JSON data, the last step is exporting the PDF. The pdf-lib library allows you to save the completed form and either download it, email it, or save it to a file system, depending on your application's needs.
Below are the final two lines of code from the previous example, which handle exporting the PDF filled with your JSON data:
const filledPdfBytes = await pdfDoc.save();
fs.writeFileSync('filled.pdf', filledPdfBytes);
At this point, your PDF is ready for use and complete with the data you populated using JSON.
Simplifying PDF data population with Joyfill
While the manual approach using open-source libraries like pdf-lib gets the job done, it can be time-consuming and requires careful management of field names and data structures.
While the manual approach gives you complete control, the Joyfill SDK eliminates the need to manage the technical details, saving development time and reducing potential errors.
This is where Joyfill comes in, offering a more streamlined solution. With Joyfill's SDK, developers can automate form population in minutes, thanks to pre-built components that seamlessly handle form field mapping and PDF data injection.
Here's an example of how Joyfill's SDK simplifies the process:
import { fillPDF } from '@joyfill/components';
const pdfData = {
"name": "John Doe",
"email": "[john.doe@example.com](mailto:john.doe@example.com)",
"date": "2024-09-23"
};
fillPDF('path/to/pdf', pdfData)
.then(filledPDF => {
// PDF with populated fields
})
.catch(error => {
console.error("Error filling PDF:", error);
});
With the Joyfill PDF form filler SDK, you don't need to manually manage the mapping of JSON data to form fields or handle the intricacies of PDF export. Everything is taken care of, allowing you to focus on building your app rather than dealing with low-level PDF form management.
By following these steps, you can easily populate PDF forms using JSON, whether you prefer a manual approach with open-source libraries or a faster, more streamlined solution with Joyfill. Either way, JSON gives you the flexibility to automate and scale your form-filling processes without getting bogged down by the complexity of PDF formats.
If you want to learn more about PDF form management, check out Joyfill.