DAY : 27 Abstract and Interface in Java – Simplified
Abstraction 1.Abstraction in Java is a fundamental concept in object-oriented programming (OOP) that hides implementation details and only shows essential features. 2.if at least one method is abstract in a class, then the entire class should be abstract Key features of abstraction: Abstraction hides the complex details and shows only essential features. Abstract classes may have methods without implementation and must be implemented by subclasses. By abstracting functionality, changes in the implementation do not affect the code that depends on the abstraction. Important rules for abstract methods are mentioned below: Any class that contains one or more abstract methods must also be declared abstract. If a class contains an abstract method it needs to be abstract and vice versa is not true. The following are various illegal combinations of other modifiers for methods with respect to abstract modifiers: final abstract native abstract synchronized abstract static abstract private abstract strictfp How to Achieve Abstraction in Java? Java provides two ways to implement abstraction, which are listed below: Abstract Classes (Partial Abstraction) Interface (100% Abstraction) Interface In Java, an interface defines a contract for classes to implement, specifying a set of abstract methods and constants. It's a blueprint for classes to adhere to, ensuring they provide certain functionalities. Interfaces allow for abstraction and enable multiple inheritance in Java. Why use interfaces? Interfaces fulfill two goals: They allow the programmer to be more abstract when referencing objects (for example, var vehicle : Vehicle, can reference any car, truck, etc... anything that is a vehicle (and not care what type it is.) This occurs at "program time".
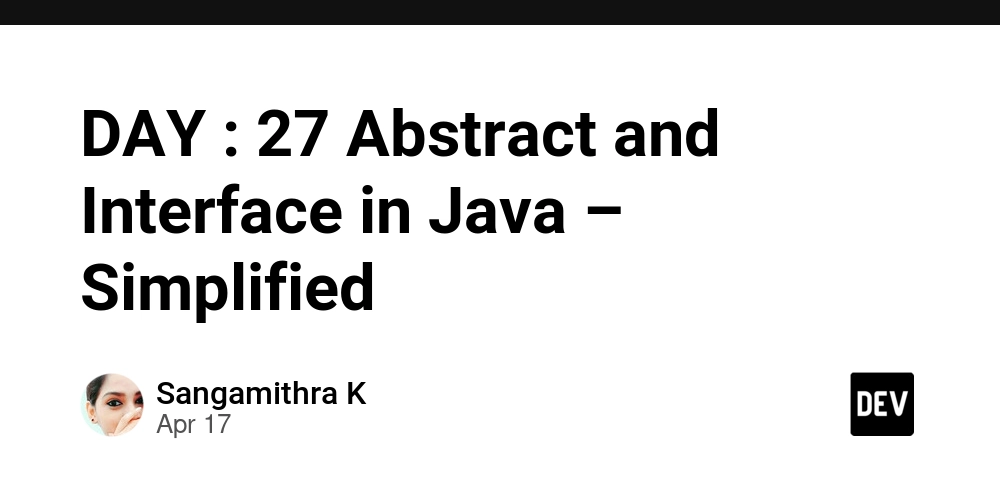
Abstraction
1.Abstraction in Java is a fundamental concept in object-oriented programming (OOP) that hides implementation details and only shows essential features.
2.if at least one method is abstract in a class, then the entire class should be abstract
Key features of abstraction:
- Abstraction hides the complex details and shows only essential features.
- Abstract classes may have methods without implementation and must be implemented by subclasses.
- By abstracting functionality, changes in the implementation do not affect the code that depends on the abstraction.
Important rules for abstract methods are mentioned below:
- Any class that contains one or more abstract methods must also be declared abstract.
-
If a class contains an abstract method it needs to be abstract and vice versa is not true.
The following are various illegal combinations of other modifiers for methods with respect to abstract modifiers:
- final
- abstract native
- abstract synchronized
- abstract static
- abstract private
- abstract strictfp
How to Achieve Abstraction in Java?
Java provides two ways to implement abstraction, which are listed below:
- Abstract Classes (Partial Abstraction)
- Interface (100% Abstraction)
Interface
In Java, an interface defines a contract for classes to implement, specifying a set of abstract methods and constants. It's a blueprint for classes to adhere to, ensuring they provide certain functionalities. Interfaces allow for abstraction and enable multiple inheritance in Java.
Why use interfaces?
Interfaces fulfill two goals: They allow the programmer to be more abstract when referencing objects (for example, var vehicle : Vehicle, can reference any car, truck, etc... anything that is a vehicle (and not care what type it is.) This occurs at "program time".