day-22: Getter Methods in Java: Best Practices for Clean and Secure Code
Getter Method in Java (Simple Explanation) A getter method is a public method that provides controlled read access to a private field in a class. Why Use a Getter? Encapsulation → Keeps fields (private) hidden but allows safe access. Security → Prevents direct modification of sensitive data. Flexibility → You can later add logic (e.g., formatting, validation) without breaking existing code. Example: Getter for goldRate public class SriKumaranTangaMaligai { private int goldRate = 5000; // Private field (hidden) // Getter method (read-only access) public int getGoldRate() { return goldRate; // Returns the value of goldRate } } How to Use the Getter? public class User { public static void main(String[] args) { SriKumaranTangaMaligai shop = new SriKumaranTangaMaligai(); // Access goldRate via getter (not directly) System.out.println("Gold Rate: ₹" + shop.getGoldRate()); } } Key Points ✔ Naming Convention: Getters start with get + FieldName (e.g., getGoldRate). ✔ Return Type: Matches the field’s type (int, String, etc.). ✔ No Parameters: Getters only return values (no arguments). Getter vs. Direct Public Access Approach Pros Cons Getter (getGoldRate()) Safe, controlled, future-proof Slightly more code Public Field (goldRate) Shorter, faster No validation, breaks encapsulation When to Avoid Getters? In high-performance code where direct access is critical. For simple data containers (use Java record instead)
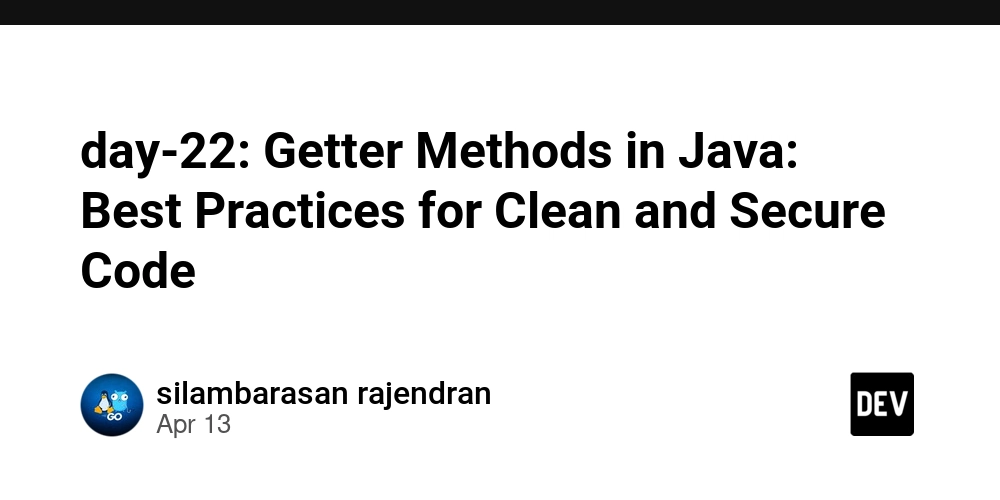
Getter Method in Java (Simple Explanation)
A getter method is a public method that provides controlled read access to a private field in a class.
Why Use a Getter?
Encapsulation → Keeps fields (private) hidden but allows safe access.
Security → Prevents direct modification of sensitive data.
Flexibility → You can later add logic (e.g., formatting, validation) without breaking existing code.
Example: Getter for goldRate
public class SriKumaranTangaMaligai {
private int goldRate = 5000; // Private field (hidden)
// Getter method (read-only access)
public int getGoldRate() {
return goldRate; // Returns the value of goldRate
}
}
How to Use the Getter?
public class User {
public static void main(String[] args) {
SriKumaranTangaMaligai shop = new SriKumaranTangaMaligai();
// Access goldRate via getter (not directly)
System.out.println("Gold Rate: ₹" + shop.getGoldRate());
}
}
Key Points
✔ Naming Convention: Getters start with get + FieldName (e.g., getGoldRate).
✔ Return Type: Matches the field’s type (int, String, etc.).
✔ No Parameters: Getters only return values (no arguments).
Getter vs. Direct Public Access
Approach Pros Cons
Getter (getGoldRate()) Safe, controlled, future-proof Slightly more code
Public Field (goldRate) Shorter, faster No validation, breaks encapsulation
When to Avoid Getters?
In high-performance code where direct access is critical.
For simple data containers (use Java record instead)