Day-19:Encapsulation
Encapsulation: Encapsulation describes the ability of an object to hide its data and methods from the rest of the world and is one of the fundamental principles of object-oriented programming. In Java, a class encapsulates the fields, which hold the state of an object, and the methods, which define the actions of the object. Encapsulation enables you to write reusable programs[TBD]. It also enables you to restrict access only to those features of an object that are declared public. All other fields and methods are private and can be used for internal object processing. **Data Encapsulation **can be defined as wrapping the code or methods(properties) and the related fields or variables together as a single unit. In object-oriented programming, we call this single unit – a class, interface, etc. We can visualize it like a medical capsule (as the name suggests, too), wherein the enclosed medicine can be compared to fields and methods of a class. ** Setter and Getter Method** Getter and setter methods are used to access and modify the private variables (fields) of a class, respectively. They help achieve encapsulation by providing controlled access to the class’s attributes. Example public class Person { private String name; private int age; // Getter for name public String getName() { return name; } // Setter for name public void setName(String name) { this.name = name; } // Getter for age public int getAge() { return age; } // Setter for age public void setAge(int age) { this.age = age; } } public class Main { public static void main(String[] args) { Person person = new Person(); // Set values using setter methods person.setName("John"); person.setAge(30); // Retrieve values using getter methods System.out.println("Name: " + person.getName()); System.out.println("Age: " + person.getAge()); } } Output Name: John Age: 30 The main() method creates an instance of the Person class named person. The setter methods setName("John") and setAge(30) are used to set the values of the name and age attributes of the person object. The getter methods getName() and getAge() are used to retrieve the values of the name and age attributes, respectively. The retrieved values are then printed to the console. Implementation of Encapsulation Example public class Person { private String name; private int age; // Constructor public Person(String name, int age) { this.name = name; this.age = age; } // Getter for name public String getName() { return name; } // Setter for name public void setName(String name) { this.name = name; } // Getter for age public int getAge() { return age; } // Setter for age public void setAge(int age) { if (age >= 0) { this.age = age; } else { System.out.println("Age cannot be negative."); } } // Method to display person details public void display() { System.out.println("Name: " + name); System.out.println("Age: " + age); } } public class Main { public static void main(String[] args) { Person person = new Person("John", 30); // Display initial details person.display(); // Update name person.setName("Alice"); // Update age person.setAge(25); // Display updated details person.display(); } } Output Name: John Age: 30 Name: Alice Age: 25 name and age are private instance variables, accessible only within the Person class. getName() and setName() methods provide access to the name variable, ensuring encapsulation by controlling how the name can be accessed and modified. getAge() and setAge() methods provide access to the age variable, with additional validation to ensure the age is non-negative. display() method allows displaying the person’s details without exposing the internal implementation. Data Hiding[TBD] Data hiding, also known as information hiding, is a fundamental principle of object-oriented programming and encapsulation . It refers to the concept of hiding the implementation details of a class’s attributes (data) and exposing only the necessary information or interfaces to interact with those attributes. Data hiding is achieved by declaring class attributes as private, meaning they are accessible only within the class itself. Outside classes cannot directly access or modify these private attributes. Instead, interactions with these attributes are typically mediated through public methods such as getters and setters, which provide controlled access to the data. Private Attributes: Attributes of a class are declared as private to restrict direct access from outside the class. public class Person { private Stri
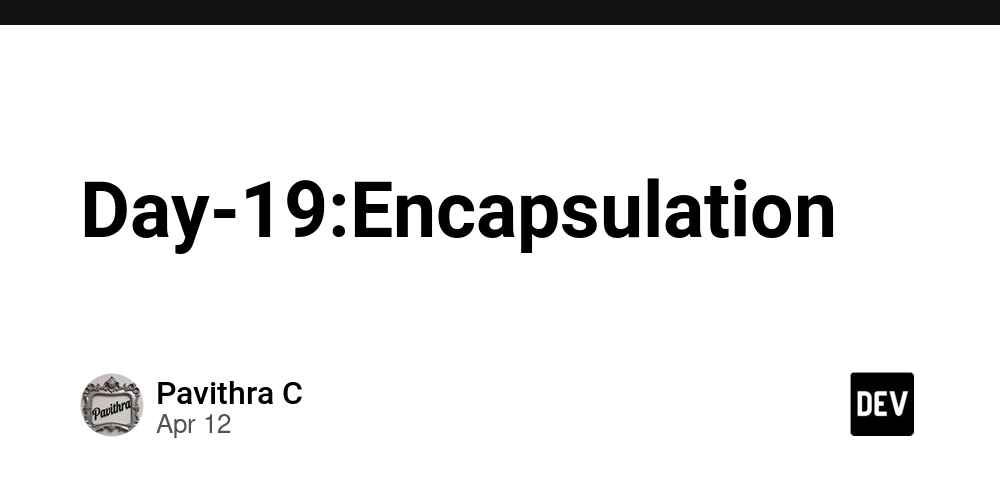
Encapsulation:
Encapsulation describes the ability of an object to hide its data and methods from the rest of the world and is one of the fundamental principles of object-oriented programming. In Java, a class encapsulates the fields, which hold the state of an object, and the methods, which define the actions of the object. Encapsulation enables you to write reusable programs[TBD]. It also enables you to restrict access only to those features of an object that are declared public. All other fields and methods are private and can be used for internal object processing.
**Data Encapsulation **can be defined as wrapping the code or methods(properties) and the related fields or variables together as a single unit. In object-oriented programming, we call this single unit – a class, interface, etc. We can visualize it like a medical capsule (as the name suggests, too), wherein the enclosed medicine can be compared to fields and methods of a class.
** Setter and Getter Method**
Getter and setter methods are used to access and modify the private variables (fields) of a class, respectively. They help achieve encapsulation by providing controlled access to the class’s attributes.
Example
public class Person {
private String name;
private int age;
// Getter for name
public String getName() {
return name;
}
// Setter for name
public void setName(String name) {
this.name = name;
}
// Getter for age
public int getAge() {
return age;
}
// Setter for age
public void setAge(int age) {
this.age = age;
}
}
public class Main {
public static void main(String[] args) {
Person person = new Person();
// Set values using setter methods
person.setName("John");
person.setAge(30);
// Retrieve values using getter methods
System.out.println("Name: " + person.getName());
System.out.println("Age: " + person.getAge());
}
}
Output
Name: John
Age: 30
- The main() method creates an instance of the Person class named person.
- The setter methods setName("John") and setAge(30) are used to set the values of the name and age attributes of the person object.
- The getter methods getName() and getAge() are used to retrieve the values of the name and age attributes, respectively.
- The retrieved values are then printed to the console.
Implementation of Encapsulation
Example
public class Person {
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getter for name
public String getName() {
return name;
}
// Setter for name
public void setName(String name) {
this.name = name;
}
// Getter for age
public int getAge() {
return age;
}
// Setter for age
public void setAge(int age) {
if (age >= 0) {
this.age = age;
} else {
System.out.println("Age cannot be negative.");
}
}
// Method to display person details
public void display() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
public class Main {
public static void main(String[] args) {
Person person = new Person("John", 30);
// Display initial details
person.display();
// Update name
person.setName("Alice");
// Update age
person.setAge(25);
// Display updated details
person.display();
}
}
Output
Name: John
Age: 30
Name: Alice
Age: 25
- name and age are private instance variables, accessible only within the Person class.
- getName() and setName() methods provide access to the name variable, ensuring encapsulation by controlling how the name can be accessed and modified.
- getAge() and setAge() methods provide access to the age variable, with additional validation to ensure the age is non-negative.
- display() method allows displaying the person’s details without exposing the internal implementation.
Data Hiding[TBD]
Data hiding, also known as information hiding, is a fundamental principle of object-oriented programming and encapsulation . It refers to the concept of hiding the implementation details of a class’s attributes (data) and exposing only the necessary information or interfaces to interact with those attributes.
Data hiding is achieved by declaring class attributes as private, meaning they are accessible only within the class itself. Outside classes cannot directly access or modify these private attributes. Instead, interactions with these attributes are typically mediated through public methods such as getters and setters, which provide controlled access to the data.
- Private Attributes: Attributes of a class are declared as private to restrict direct access from outside the class.
public class Person {
private String name; // private attribute
private int age; // private attribute
// other code...
}
- Public Methods: Public methods, such as getters and setters, are provided to access and modify the private attributes.
public class Person {
private String name;
private int age;
// Getter for name
public String getName() {
return name;
}
// Setter for name
public void setName(String name) {
this.name = name;
}
// Getter for age
public int getAge() {
return age;
}
// Setter for age
public void setAge(int age) {
this.age = age;
}
// other code...
}
- Controlled Access: Outside classes interact with the object’s attributes only through these public methods. This way, the internal representation of data is hidden, and the class can enforce its own rules and validations.
public class Main {
public static void main(String[] args) {
Person person = new Person();
// Access and modify attributes via public methods
person.setName("John");
person.setAge(30);
// Retrieve attribute values
String name = person.getName();
int age = person.getAge();
// Display information
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
Reference link
https://www.geekster.in/articles/encapsulation-in-java/
https://docs.oracle.com/en/database/oracle/oracle-database/19/jjdev/Java-overview.html#GUID-061CB7CD-144F-4B3C-9409-748B94C25A09