Day : 18 Return Data types and programmes
Return Data Types The return type must be specified before the method's signature when declaring the method. There are several different data types that can be used as a method return type in Java, including primitive data types (e.g. int, double, char), object data types (e.g. Strings, Objects) and the void data typ. Void The void keyword in Java is used to specify that a method does not return any value. It is a return type that indicates the method performs an action but does not produce a result that can be used elsewhere in the code. Important in Java 1.class 2.object 3.method 4.Arguments 5.return datatypes questions for your reference (FUN ACTIVITY :).. can we pass multiple arguments to a method. (TRUE/FALSE) can we return multiple values from a method. (TRUE/FALSE) Can we have multiple class in java. (TRUE/FALSE) can we have a same name in same class. (TRUE/FALSE) Example programs public class Calculator2 { //void - nothing is returned public int add(int no1, int no2) { // parameter declearation return no1+no2; // return value } public int multiply(int no1, int no2) { // nothing to retuen so void return no1*no2; } public static void main(String[] args) { Calculator2 calc = new Calculator2(); int result = calc.add(20,30); // argument declearation System.out.println(result); int multiply_output = calc.multiply(result, 2); System.out.println(multiply_output); Calculator3 calculator3 = new Calculator3(); int subtractor = calculator3.subtractor(result, 5); System.out.println(subtractor); int subtractor1 = calculator3.subtractor(multiply_output, 7); System.out.println("this is subtractor by 60: " +subtractor1); } } ----------------------------------------------------------------------------public class Calculator3 { public static void cal_information() { System.out.println("Calculator information"); } public int subtractor(int no1, int no2) { // parameter declearation return no1-no2; // return value } } OUTPUT sangamithra@ideapad:~/Documents/mar20$ java Calculator2 50 100 45 this is subtractor by 60: 93 Answers: 1.true 2.true 3.true 4.true
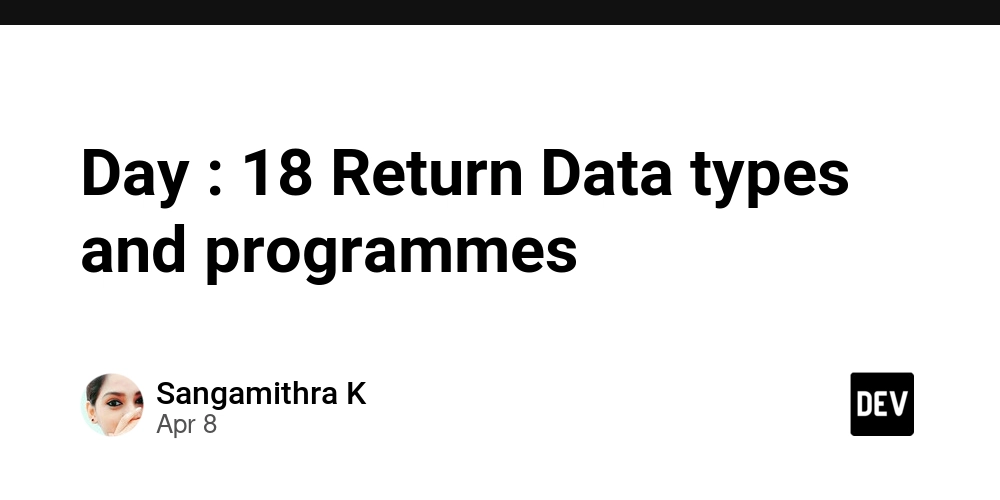
Return Data Types
The return type must be specified before the method's signature when declaring the method. There are several different data types that can be used as a method return type in Java, including primitive data types (e.g. int, double, char), object data types (e.g. Strings, Objects) and the void data typ.
Void
The void keyword in Java is used to specify that a method does not return any value. It is a return type that indicates the method performs an action but does not produce a result that can be used elsewhere in the code.
Important in Java
1.class
2.object
3.method
4.Arguments
5.return datatypes
questions for your reference (FUN ACTIVITY :)..
- can we pass multiple arguments to a method. (TRUE/FALSE)
- can we return multiple values from a method. (TRUE/FALSE)
- Can we have multiple class in java. (TRUE/FALSE)
- can we have a same name in same class. (TRUE/FALSE)
Example programs
public class Calculator2 {
//void - nothing is returned
public int add(int no1, int no2) { // parameter declearation
return no1+no2; // return value
}
public int multiply(int no1, int no2) { // nothing to retuen so void
return no1*no2;
}
public static void main(String[] args) {
Calculator2 calc = new Calculator2();
int result = calc.add(20,30); // argument declearation
System.out.println(result);
int multiply_output = calc.multiply(result, 2);
System.out.println(multiply_output);
Calculator3 calculator3 = new Calculator3();
int subtractor = calculator3.subtractor(result, 5);
System.out.println(subtractor);
int subtractor1 = calculator3.subtractor(multiply_output, 7);
System.out.println("this is subtractor by 60: " +subtractor1);
}
}
----------------------------------------------------------------------------public class Calculator3 {
public static void cal_information() {
System.out.println("Calculator information");
}
public int subtractor(int no1, int no2) { // parameter declearation
return no1-no2; // return value
}
}
OUTPUT
sangamithra@ideapad:~/Documents/mar20$ java Calculator2
50
100
45
this is subtractor by 60: 93
Answers:
1.true
2.true
3.true
4.true