day-14: Building a Theatre Simulation in Java: Static vs Non-Static Methods Explained
Method: A method is a block of code that performs a specific task. It is a collection of instructions grouped together to perform a particular operation. Methods can be used to perform actions, return values, or modify data. Static and Non-static Methods: Static Method: A method declared with the static keyword, which belongs to the class and can be called without an object. Non-static Method: A method that does not have the static keyword and requires an object of the class to be invoked. Key Points: Static methods are associated with the class and do not require an instance to be called. They can only access static variables and other static methods. public static void myStaticMethod() { // code } Non-static methods are associated with objects (instances) and require an object to be called. They can access both instance variables and static variables. public void myNonStaticMethod() { // code } Example Programme: public class Theatre { static String owner = "Sethu"; //class specific variable int ticket_fare; //int //non-static float show_time; //float String movie_name; //String boolean on_screen; //boolean //Method Definition public static void open_theatre() { System.out.println("Open Counters"); System.out.println("Clean All Areas"); } //Non-static method definition public void cast_movie() { System.out.println("Watching Movie " +movie_name); } public static void main(String[] args) { Theatre screen1 = new Theatre(); Theatre screen2 = new Theatre(); Theatre screen3 = new Theatre(); screen1.movie_name = "Leo"; screen2.movie_name = "Vidaamuyarchi"; screen3.movie_name = "VDS Part 2"; // screen1.show_time = 11.30f; // ticket_fare = 120; Theatre.open_theatre(); //Method Calling Statement or Static method screen1.cast_movie(); //Object - non-static screen2.cast_movie(); screen3.cast_movie(); } } Note: The document formatting and structure were assisted by ChatGPT. ------------------- End of the Blog -------------------------------
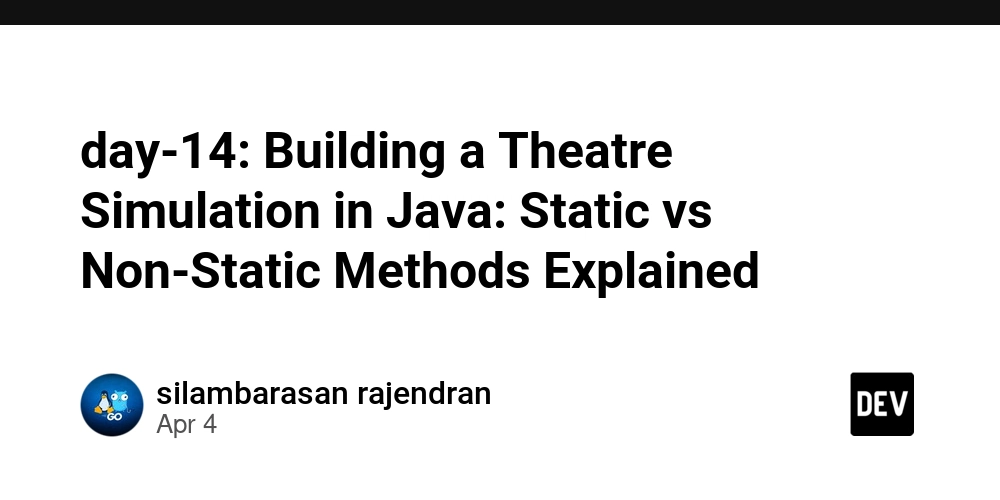
Method:
A method is a block of code that performs a specific task. It is a collection of instructions grouped together to perform a particular operation. Methods can be used to perform actions, return values, or modify data.
Static and Non-static Methods:
Static Method: A method declared with the static keyword, which belongs to the class and can be called without an object.
Non-static Method: A method that does not have the static keyword and requires an object of the class to be invoked.
Key Points:
Static methods are associated with the class and do not require an instance to be called.
They can only access static variables and other static methods.
public static void myStaticMethod() {
// code
}
Non-static methods are associated with objects (instances) and require an object to be called.
They can access both instance variables and static variables.
public void myNonStaticMethod() {
// code
}
Example Programme:
public class Theatre {
static String owner = "Sethu"; //class specific variable
int ticket_fare; //int //non-static
float show_time; //float
String movie_name; //String
boolean on_screen; //boolean
//Method Definition
public static void open_theatre() {
System.out.println("Open Counters");
System.out.println("Clean All Areas");
}
//Non-static method definition
public void cast_movie() {
System.out.println("Watching Movie " +movie_name);
}
public static void main(String[] args) {
Theatre screen1 = new Theatre();
Theatre screen2 = new Theatre();
Theatre screen3 = new Theatre();
screen1.movie_name = "Leo";
screen2.movie_name = "Vidaamuyarchi";
screen3.movie_name = "VDS Part 2";
// screen1.show_time = 11.30f;
// ticket_fare = 120;
Theatre.open_theatre(); //Method Calling Statement or Static method
screen1.cast_movie(); //Object - non-static
screen2.cast_movie();
screen3.cast_movie();
}
}
Note: The document formatting and structure were assisted by ChatGPT.
------------------- End of the Blog -------------------------------