Creating Data-Driven Dashboards Without a Backend: The Spreadsheet Developer's Guide
Every developer has faced the "quick dashboard" request: stakeholders need visualizations of business metrics, and they need them yesterday. Traditional approaches often require setting up a database, building an API, and creating a frontend—a process that can take weeks even for experienced developers. But what if you could build powerful, interactive dashboards in a fraction of the time? This guide explores how to leverage spreadsheets as a lightweight backend for data-driven applications, an approach that's surprisingly powerful for prototypes and internal tools. The Traditional Dashboard Development Stack Typically, building a data dashboard involves: Database setup and schema design Server-side API development Data processing logic Frontend UI framework implementation Authentication and authorization Deployment and hosting Each step requires specialized knowledge and time investment. For critical production systems, this comprehensive approach makes sense. But for internal tools, prototypes, or time-sensitive projects, this stack can be overkill. Enter the Spreadsheet-Powered Alternative Spreadsheets—particularly Google Sheets—offer several advantages as a lightweight backend: Zero infrastructure management Familiar interface for non-technical teammates to update data Built-in access controls for sharing and permissions Real-time collaboration features API access through Google Sheets API or third-party tools Version history tracking changes Setting Up the Spreadsheet Backend First, structure your data properly in Google Sheets: For best performance: Use a consistent schema with headers Format data appropriately (dates as dates, numbers as numbers) Create separate sheets for different data categories Name your ranges for easier reference Connecting to Your Frontend You have several options for connecting your spreadsheet to a dashboard frontend: Option 1: Direct API Access Use the Google Sheets API directly with JavaScript: async function fetchSpreadsheetData() { const SHEET_ID = 'your-sheet-id'; const API_KEY = 'your-api-key'; const RANGE = 'Sheet1!A1:D100'; const response = await fetch( `https://sheets.googleapis.com/v4/spreadsheets/${SHEET_ID}/values/${RANGE}?key=${API_KEY}` ); const data = await response.json(); return data.values; } Option 2: Specialized Integration Tools Several tools can simplify the process: Sheetsu or Sheety provide RESTful interfaces to your spreadsheet data Retool or Internal.io offer drag-and-drop interfaces for building admin tools SmartSpreadsheets.co provides automation tools that can transform and expose your data through convenient interfaces Option 3: Spreadsheet Publishing For simple public dashboards, you can publish specific sheets to the web and embed them using iframes or specialized embedding tools. Building the Frontend Dashboard Once your data connection is established, you can build your dashboard using any frontend framework: // Using React with a charting library like Chart.js import { Line } from 'react-chartjs-2'; function Dashboard() { const [data, setData] = useState(null); useEffect(() => { async function loadData() { const spreadsheetData = await fetchSpreadsheetData(); // Transform the data for Chart.js const transformedData = transformForCharts(spreadsheetData); setData(transformedData); } loadData(); }, []); return ( Business Metrics Dashboard {data ? ( ) : ( Loading data... )} ); } Advanced Techniques Real-Time Updates For dashboards that need real-time updates: function setupRealtimeUpdates(callback, intervalMs = 30000) { // Initial load callback(); // Set up polling const intervalId = setInterval(callback, intervalMs); // Clean up on component unmount return () => clearInterval(intervalId); } Data Transformation and Caching When working with larger datasets, implement client-side data processing: function processAndCacheData(rawData) { // Transform data const processed = rawData.map(/* transformation logic */); // Cache in localStorage for performance localStorage.setItem('dashboardData', JSON.stringify({ timestamp: Date.now(), data: processed })); return processed; } Automation for Data Aggregation For more complex data needs, you can automate the collection and processing within the spreadsheet itself: Use Google Apps Script for custom functions and triggers Set up automated data import from external sources Create spreadsheet formulas for data aggregation and analysis Tools like SmartSpreadsheets.co enhance these capabilities by providing automation tools specifically designed for Google Sheets, allowing for web scraping, AI-powered data processing, and dynamic content generation all within
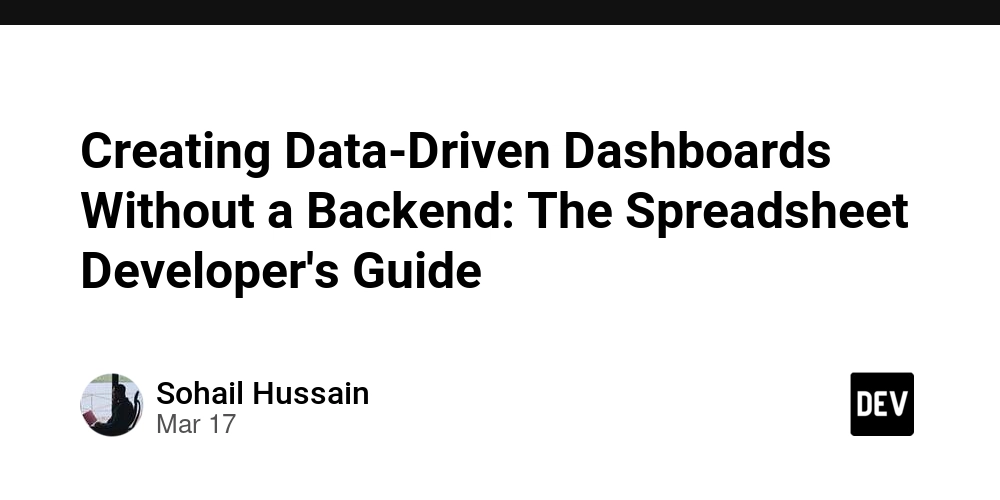
Every developer has faced the "quick dashboard" request: stakeholders need visualizations of business metrics, and they need them yesterday. Traditional approaches often require setting up a database, building an API, and creating a frontend—a process that can take weeks even for experienced developers.
But what if you could build powerful, interactive dashboards in a fraction of the time? This guide explores how to leverage spreadsheets as a lightweight backend for data-driven applications, an approach that's surprisingly powerful for prototypes and internal tools.
The Traditional Dashboard Development Stack
Typically, building a data dashboard involves:
- Database setup and schema design
- Server-side API development
- Data processing logic
- Frontend UI framework implementation
- Authentication and authorization
- Deployment and hosting
Each step requires specialized knowledge and time investment. For critical production systems, this comprehensive approach makes sense. But for internal tools, prototypes, or time-sensitive projects, this stack can be overkill.
Enter the Spreadsheet-Powered Alternative
Spreadsheets—particularly Google Sheets—offer several advantages as a lightweight backend:
- Zero infrastructure management
- Familiar interface for non-technical teammates to update data
- Built-in access controls for sharing and permissions
- Real-time collaboration features
- API access through Google Sheets API or third-party tools
- Version history tracking changes
Setting Up the Spreadsheet Backend
First, structure your data properly in Google Sheets:
For best performance:
- Use a consistent schema with headers
- Format data appropriately (dates as dates, numbers as numbers)
- Create separate sheets for different data categories
- Name your ranges for easier reference
Connecting to Your Frontend
You have several options for connecting your spreadsheet to a dashboard frontend:
Option 1: Direct API Access
Use the Google Sheets API directly with JavaScript:
async function fetchSpreadsheetData() {
const SHEET_ID = 'your-sheet-id';
const API_KEY = 'your-api-key';
const RANGE = 'Sheet1!A1:D100';
const response = await fetch(
`https://sheets.googleapis.com/v4/spreadsheets/${SHEET_ID}/values/${RANGE}?key=${API_KEY}`
);
const data = await response.json();
return data.values;
}
Option 2: Specialized Integration Tools
Several tools can simplify the process:
- Sheetsu or Sheety provide RESTful interfaces to your spreadsheet data
- Retool or Internal.io offer drag-and-drop interfaces for building admin tools
- SmartSpreadsheets.co provides automation tools that can transform and expose your data through convenient interfaces
Option 3: Spreadsheet Publishing
For simple public dashboards, you can publish specific sheets to the web and embed them using iframes or specialized embedding tools.
Building the Frontend Dashboard
Once your data connection is established, you can build your dashboard using any frontend framework:
// Using React with a charting library like Chart.js
import { Line } from 'react-chartjs-2';
function Dashboard() {
const [data, setData] = useState(null);
useEffect(() => {
async function loadData() {
const spreadsheetData = await fetchSpreadsheetData();
// Transform the data for Chart.js
const transformedData = transformForCharts(spreadsheetData);
setData(transformedData);
}
loadData();
}, []);
return (
<div className="dashboard">
<h1>Business Metrics Dashboard</h1>
{data ? (
<Line data={data.revenueData} options={chartOptions} />
) : (
<p>Loading data...</p>
)}
</div>
);
}
Advanced Techniques
Real-Time Updates
For dashboards that need real-time updates:
function setupRealtimeUpdates(callback, intervalMs = 30000) {
// Initial load
callback();
// Set up polling
const intervalId = setInterval(callback, intervalMs);
// Clean up on component unmount
return () => clearInterval(intervalId);
}
Data Transformation and Caching
When working with larger datasets, implement client-side data processing:
function processAndCacheData(rawData) {
// Transform data
const processed = rawData.map(/* transformation logic */);
// Cache in localStorage for performance
localStorage.setItem('dashboardData', JSON.stringify({
timestamp: Date.now(),
data: processed
}));
return processed;
}
Automation for Data Aggregation
For more complex data needs, you can automate the collection and processing within the spreadsheet itself:
- Use Google Apps Script for custom functions and triggers
- Set up automated data import from external sources
- Create spreadsheet formulas for data aggregation and analysis
Tools like SmartSpreadsheets.co enhance these capabilities by providing automation tools specifically designed for Google Sheets, allowing for web scraping, AI-powered data processing, and dynamic content generation all within your spreadsheet backend.
Case Study: Analytics Dashboard in 2 Hours
Recently, I needed to create a content performance dashboard for a marketing team. Instead of the traditional stack, I:
- Created a Google Sheet with structured analytics data
- Used SmartSpreadsheets.co's Web Scraper Pro to automatically import real-time performance metrics from various platforms
- Set up a simple React app that consumed the spreadsheet data via API
- Deployed to Vercel in minutes
The entire process took under 2 hours, compared to days using traditional methods. The marketing team could update data sources directly in the familiar spreadsheet interface, while developers maintained control over the presentation layer.
When to Use This Approach
This spreadsheet-as-a-backend approach works best for:
- Internal dashboards where polish matters less than functionality
- Rapid prototyping to validate ideas before full implementation
- Small-to-medium datasets (up to tens of thousands of rows)
- Teams with mixed technical skills who need to collaborate on data
- Projects with tight deadlines that can't afford a full stack implementation
Limitations to Consider
Be aware of certain constraints:
- API quotas can limit high-volume applications
- Performance issues with very large datasets
- Limited complex querying capabilities compared to real databases
- Security considerations for sensitive data
Conclusion
Building data-driven dashboards doesn't always require complex infrastructure. By leveraging spreadsheets as a backend, developers can create functional, collaborative dashboard solutions in hours rather than weeks. This approach bridges the gap between developers and non-technical stakeholders, allowing for rapid iteration and validation before investing in more robust solutions.
As you explore this approach, consider tools like SmartSpreadsheets.co that extend the native capabilities of Google Sheets with automation and integration features specifically designed for developers looking to build sophisticated solutions with minimal infrastructure.
What other creative ways have you found to use spreadsheets in your development workflow? Share your experiences in the comments!