Creating Accessible Form Controls with JavaScript: Expert Implementation Guide
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Building accessible form controls requires careful planning and implementation. Form controls are fundamental interaction points in web applications, making accessibility essential. Let me share practical techniques to create truly accessible form controls with JavaScript. Input Validation Feedback Providing clear validation feedback benefits all users. When implementing validation, we must ensure feedback is perceivable regardless of how someone accesses our application. Effective validation feedback combines visual cues with programmatic announcements. Here's an implementation example: function validateEmail(input) { const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; const isValid = emailRegex.test(input.value); const errorContainer = document.getElementById(`${input.id}-error`); if (!isValid) { input.setAttribute('aria-invalid', 'true'); input.setAttribute('aria-describedby', `${input.id}-error`); errorContainer.textContent = 'Please enter a valid email address'; errorContainer.setAttribute('role', 'alert'); } else { input.removeAttribute('aria-invalid'); input.removeAttribute('aria-describedby'); errorContainer.textContent = ''; errorContainer.removeAttribute('role'); } return isValid; } This approach provides multiple feedback mechanisms: Visual styling through CSS (targeting aria-invalid) Screen reader announcement via the alert role Programmatic association between the field and error message I've found the most effective validation provides immediate feedback but doesn't interrupt the user's flow. Consider implementing validation on blur rather than with every keystroke. Focus Management Focus management is essential for keyboard users. A lack of proper focus can make forms unusable for those who cannot use a mouse. For complex controls like modals, custom dropdowns, or multi-step forms, we need deliberate focus management: class FocusTrap { constructor(element) { this.element = element; this.focusableElements = this.element.querySelectorAll( 'button, [href], input, select, textarea, [tabindex]:not([tabindex="-1"])' ); this.firstElement = this.focusableElements[0]; this.lastElement = this.focusableElements[this.focusableElements.length - 1]; this.handleKeyDown = this.handleKeyDown.bind(this); this.element.addEventListener('keydown', this.handleKeyDown); } handleKeyDown(e) { if (e.key === 'Tab') { if (e.shiftKey && document.activeElement === this.firstElement) { e.preventDefault(); this.lastElement.focus(); } else if (!e.shiftKey && document.activeElement === this.lastElement) { e.preventDefault(); this.firstElement.focus(); } } } activate() { this.previousFocus = document.activeElement; this.firstElement.focus(); } deactivate() { if (this.previousFocus) { this.previousFocus.focus(); } this.element.removeEventListener('keydown', this.handleKeyDown); } } When implementing multi-step forms, I ensure focus moves to the first field or error message in each step. This prevents keyboard users from having to tab through all elements to reach the current step. Custom Control Architecture Custom controls like sliders, toggles, and dropdowns must be built with accessibility in mind from the start. A proper custom control architecture includes: class CustomSelect { constructor(element) { this.container = element; this.button = this.container.querySelector('.select-button'); this.listbox = this.container.querySelector('.select-options'); this.options = Array.from(this.listbox.querySelectorAll('.select-option')); // ARIA setup this.button.setAttribute('aria-haspopup', 'listbox'); this.button.setAttribute('aria-expanded', 'false'); this.listbox.setAttribute('role', 'listbox'); this.listbox.setAttribute('tabindex', '-1'); this.options.forEach(option => { option.setAttribute('role', 'option'); option.setAttribute('tabindex', '-1'); }); // Event listeners this.button.addEventListener('click', () => this.toggleDropdown()); this.button.addEventListener('keydown', (e) => this.handleButtonKeyDown(e)); this.listbox.addEventListener('keydown', (e) => this.handleListboxKeyDown(e)); this.options.forEach(option => { option.addEventListener('click', () => this.selectOption(option)); }); // Close dropdown when clicking outside document.addEventListener('click', (e) => { if (!this.container.contains(e.target)) { this.closeDropdown(); } }); } toggleDropdown() { const isExpanded = this.button.getAttribute('aria-expanded') === 'true'; if (isExpanded) { this.closeDropdown();
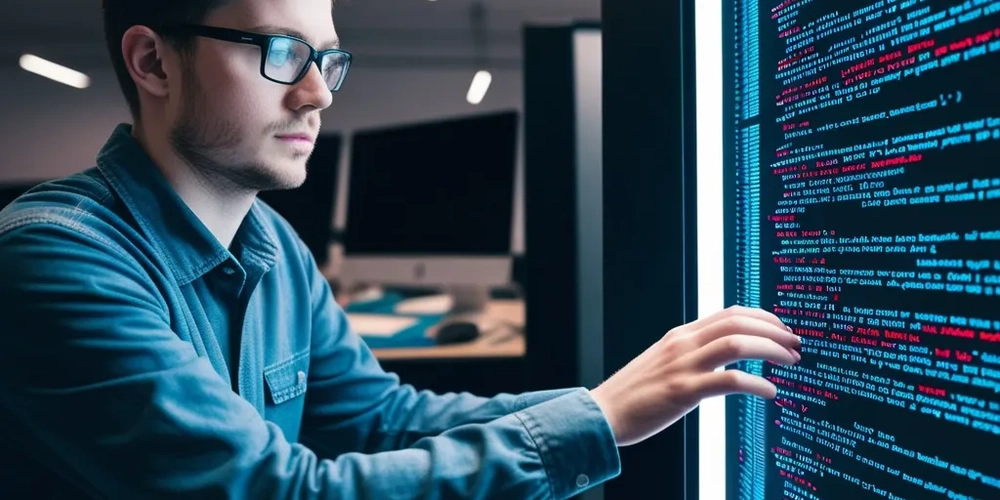
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Building accessible form controls requires careful planning and implementation. Form controls are fundamental interaction points in web applications, making accessibility essential. Let me share practical techniques to create truly accessible form controls with JavaScript.
Input Validation Feedback
Providing clear validation feedback benefits all users. When implementing validation, we must ensure feedback is perceivable regardless of how someone accesses our application.
Effective validation feedback combines visual cues with programmatic announcements. Here's an implementation example:
function validateEmail(input) {
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
const isValid = emailRegex.test(input.value);
const errorContainer = document.getElementById(`${input.id}-error`);
if (!isValid) {
input.setAttribute('aria-invalid', 'true');
input.setAttribute('aria-describedby', `${input.id}-error`);
errorContainer.textContent = 'Please enter a valid email address';
errorContainer.setAttribute('role', 'alert');
} else {
input.removeAttribute('aria-invalid');
input.removeAttribute('aria-describedby');
errorContainer.textContent = '';
errorContainer.removeAttribute('role');
}
return isValid;
}
This approach provides multiple feedback mechanisms:
- Visual styling through CSS (targeting aria-invalid)
- Screen reader announcement via the alert role
- Programmatic association between the field and error message
I've found the most effective validation provides immediate feedback but doesn't interrupt the user's flow. Consider implementing validation on blur rather than with every keystroke.
Focus Management
Focus management is essential for keyboard users. A lack of proper focus can make forms unusable for those who cannot use a mouse.
For complex controls like modals, custom dropdowns, or multi-step forms, we need deliberate focus management:
class FocusTrap {
constructor(element) {
this.element = element;
this.focusableElements = this.element.querySelectorAll(
'button, [href], input, select, textarea, [tabindex]:not([tabindex="-1"])'
);
this.firstElement = this.focusableElements[0];
this.lastElement = this.focusableElements[this.focusableElements.length - 1];
this.handleKeyDown = this.handleKeyDown.bind(this);
this.element.addEventListener('keydown', this.handleKeyDown);
}
handleKeyDown(e) {
if (e.key === 'Tab') {
if (e.shiftKey && document.activeElement === this.firstElement) {
e.preventDefault();
this.lastElement.focus();
} else if (!e.shiftKey && document.activeElement === this.lastElement) {
e.preventDefault();
this.firstElement.focus();
}
}
}
activate() {
this.previousFocus = document.activeElement;
this.firstElement.focus();
}
deactivate() {
if (this.previousFocus) {
this.previousFocus.focus();
}
this.element.removeEventListener('keydown', this.handleKeyDown);
}
}
When implementing multi-step forms, I ensure focus moves to the first field or error message in each step. This prevents keyboard users from having to tab through all elements to reach the current step.
Custom Control Architecture
Custom controls like sliders, toggles, and dropdowns must be built with accessibility in mind from the start. A proper custom control architecture includes:
class CustomSelect {
constructor(element) {
this.container = element;
this.button = this.container.querySelector('.select-button');
this.listbox = this.container.querySelector('.select-options');
this.options = Array.from(this.listbox.querySelectorAll('.select-option'));
// ARIA setup
this.button.setAttribute('aria-haspopup', 'listbox');
this.button.setAttribute('aria-expanded', 'false');
this.listbox.setAttribute('role', 'listbox');
this.listbox.setAttribute('tabindex', '-1');
this.options.forEach(option => {
option.setAttribute('role', 'option');
option.setAttribute('tabindex', '-1');
});
// Event listeners
this.button.addEventListener('click', () => this.toggleDropdown());
this.button.addEventListener('keydown', (e) => this.handleButtonKeyDown(e));
this.listbox.addEventListener('keydown', (e) => this.handleListboxKeyDown(e));
this.options.forEach(option => {
option.addEventListener('click', () => this.selectOption(option));
});
// Close dropdown when clicking outside
document.addEventListener('click', (e) => {
if (!this.container.contains(e.target)) {
this.closeDropdown();
}
});
}
toggleDropdown() {
const isExpanded = this.button.getAttribute('aria-expanded') === 'true';
if (isExpanded) {
this.closeDropdown();
} else {
this.openDropdown();
}
}
openDropdown() {
this.button.setAttribute('aria-expanded', 'true');
this.listbox.classList.add('visible');
// If we have a selected option, focus it, otherwise focus the first option
const selectedOption = this.options.find(opt => opt.getAttribute('aria-selected') === 'true');
(selectedOption || this.options[0]).focus();
}
closeDropdown() {
this.button.setAttribute('aria-expanded', 'false');
this.listbox.classList.remove('visible');
this.button.focus();
}
selectOption(option) {
// Update ARIA states
this.options.forEach(opt => opt.setAttribute('aria-selected', 'false'));
option.setAttribute('aria-selected', 'true');
// Update button text
this.button.textContent = option.textContent;
// Close dropdown
this.closeDropdown();
// Dispatch change event
const event = new CustomEvent('change', {
bubbles: true,
detail: { value: option.dataset.value }
});
this.container.dispatchEvent(event);
}
handleButtonKeyDown(e) {
switch(e.key) {
case 'ArrowDown':
case 'Enter':
case ' ':
e.preventDefault();
this.openDropdown();
break;
case 'Escape':
this.closeDropdown();
break;
}
}
handleListboxKeyDown(e) {
const currentIndex = this.options.indexOf(document.activeElement);
switch(e.key) {
case 'ArrowDown':
e.preventDefault();
if (currentIndex < this.options.length - 1) {
this.options[currentIndex + 1].focus();
}
break;
case 'ArrowUp':
e.preventDefault();
if (currentIndex > 0) {
this.options[currentIndex - 1].focus();
}
break;
case 'Home':
e.preventDefault();
this.options[0].focus();
break;
case 'End':
e.preventDefault();
this.options[this.options.length - 1].focus();
break;
case 'Enter':
case ' ':
e.preventDefault();
this.selectOption(document.activeElement);
break;
case 'Escape':
e.preventDefault();
this.closeDropdown();
break;
}
}
}
When building custom controls, I always test them with a keyboard and screen reader. I find this reveals interaction issues that might not be obvious during development.
Label Association
Every form control must have a programmatically associated label. This is fundamental for accessibility yet often overlooked:
function createDynamicFormField(fieldType, id, labelText) {
const fieldContainer = document.createElement('div');
fieldContainer.className = 'form-field';
// Create label
const label = document.createElement('label');
label.setAttribute('for', id);
label.textContent = labelText;
// Create input
const input = document.createElement(fieldType === 'textarea' ? 'textarea' : 'input');
input.setAttribute('id', id);
input.setAttribute('name', id);
if (fieldType !== 'textarea') {
input.setAttribute('type', fieldType);
}
// Add error message container
const errorContainer = document.createElement('div');
errorContainer.setAttribute('id', `${id}-error`);
errorContainer.className = 'error-message';
// Assemble field
fieldContainer.appendChild(label);
fieldContainer.appendChild(input);
fieldContainer.appendChild(errorContainer);
return fieldContainer;
}
For more complex controls where traditional labels aren't sufficient, I use ARIA attributes:
function createRatingControl(id, labelText) {
const container = document.createElement('div');
container.className = 'rating-control';
const groupLabel = document.createElement('span');
groupLabel.setAttribute('id', `${id}-label`);
groupLabel.textContent = labelText;
const ratingGroup = document.createElement('div');
ratingGroup.setAttribute('role', 'radiogroup');
ratingGroup.setAttribute('aria-labelledby', `${id}-label`);
for (let i = 1; i <= 5; i++) {
const star = document.createElement('span');
star.setAttribute('role', 'radio');
star.setAttribute('tabindex', i === 1 ? '0' : '-1');
star.setAttribute('aria-checked', 'false');
star.setAttribute('aria-label', `${i} star${i > 1 ? 's' : ''}`);
star.className = 'star';
star.dataset.value = i;
star.addEventListener('click', () => selectRating(ratingGroup, star));
star.addEventListener('keydown', (e) => handleRatingKeyDown(e, ratingGroup));
ratingGroup.appendChild(star);
}
container.appendChild(groupLabel);
container.appendChild(ratingGroup);
return container;
}
State Communication
Communicating state changes is critical for accessibility. I use ARIA live regions to announce dynamic changes:
function createLiveRegion(id, politeness = 'polite') {
const region = document.createElement('div');
region.setAttribute('id', id);
region.setAttribute('aria-live', politeness);
region.setAttribute('role', politeness === 'assertive' ? 'alert' : 'status');
region.className = 'sr-only'; // Visually hidden but available to screen readers
return region;
}
function updateFormStatus(message, isError = false) {
const statusRegion = document.getElementById('form-status');
statusRegion.textContent = message;
if (isError) {
statusRegion.setAttribute('aria-live', 'assertive');
} else {
statusRegion.setAttribute('aria-live', 'polite');
}
}
// Example usage
document.getElementById('submit-button').addEventListener('click', function(e) {
e.preventDefault();
const form = document.getElementById('contact-form');
if (validateForm(form)) {
updateFormStatus('Form submitted successfully! We will contact you soon.');
// Process form submission
} else {
updateFormStatus('Please correct the errors in the form to continue.', true);
}
});
For controls that update frequently, I'm careful about how often I send announcements to avoid overwhelming users with too many notifications.
Keyboard Navigation
Keyboard navigation patterns should follow established conventions:
function setupTabPanels(tabContainer) {
const tabs = Array.from(tabContainer.querySelectorAll('[role="tab"]'));
const panels = Array.from(tabContainer.querySelectorAll('[role="tabpanel"]'));
// Set up keyboard navigation
tabs.forEach(tab => {
tab.addEventListener('keydown', (e) => {
const currentIndex = tabs.indexOf(tab);
let newIndex;
switch(e.key) {
case 'ArrowLeft':
e.preventDefault();
newIndex = currentIndex > 0 ? currentIndex - 1 : tabs.length - 1;
break;
case 'ArrowRight':
e.preventDefault();
newIndex = currentIndex < tabs.length - 1 ? currentIndex + 1 : 0;
break;
case 'Home':
e.preventDefault();
newIndex = 0;
break;
case 'End':
e.preventDefault();
newIndex = tabs.length - 1;
break;
default:
return;
}
tabs[newIndex].focus();
tabs[newIndex].click();
});
tab.addEventListener('click', () => activateTab(tab, tabs, panels));
});
}
function activateTab(selectedTab, tabs, panels) {
// Update tab states
tabs.forEach(tab => {
const isSelected = tab === selectedTab;
tab.setAttribute('aria-selected', isSelected ? 'true' : 'false');
tab.setAttribute('tabindex', isSelected ? '0' : '-1');
});
// Show the selected panel, hide others
const selectedPanelId = selectedTab.getAttribute('aria-controls');
panels.forEach(panel => {
panel.setAttribute('hidden', panel.id !== selectedPanelId);
});
}
I always implement keyboard shortcuts that align with user expectations. Using familiar patterns helps reduce the cognitive load for all users.
Touch Target Optimization
Form controls need to be easy to interact with on touch devices:
function createAccessibleButton(id, labelText, iconClass) {
const button = document.createElement('button');
button.setAttribute('id', id);
button.className = 'accessible-button';
// Create the visual container to ensure adequate touch target
const touchTarget = document.createElement('span');
touchTarget.className = 'touch-target';
// Create icon if provided
if (iconClass) {
const icon = document.createElement('span');
icon.className = `icon ${iconClass}`;
// Add aria-hidden to decorative icons
icon.setAttribute('aria-hidden', 'true');
touchTarget.appendChild(icon);
}
// Add text (and optional screen reader text)
const textNode = document.createElement('span');
textNode.textContent = labelText;
touchTarget.appendChild(textNode);
button.appendChild(touchTarget);
return button;
}
// CSS to go with this component
/*
.accessible-button {
position: relative;
border: none;
background: transparent;
padding: 0;
}
.touch-target {
display: flex;
align-items: center;
justify-content: center;
min-width: 44px;
min-height: 44px;
padding: 8px 16px;
}
@media (pointer: fine) {
.touch-target {
min-width: 32px;
min-height: 32px;
padding: 4px 12px;
}
}
*/
The 44x44 pixel minimum touch target size follows WCAG recommendations for touch interactions, while the media query adjusts sizes for devices with fine pointer capabilities.
When creating custom touch-friendly controls, I'm careful to maintain visual design while improving usability. CSS techniques like transparent extended hitareas help create controls that are functionally larger than they appear visually.
Applying These Techniques in Practice
Creating accessible forms requires integrating these techniques into a cohesive system. Here's how I approach building a complete form:
class AccessibleForm {
constructor(formElement) {
this.form = formElement;
this.fields = Array.from(this.form.querySelectorAll('input, select, textarea'));
this.submitButton = this.form.querySelector('[type="submit"]');
// Create status region
this.statusRegion = createLiveRegion('form-status');
this.form.appendChild(this.statusRegion);
// Set up event listeners
this.form.addEventListener('submit', (e) => this.handleSubmit(e));
this.fields.forEach(field => {
field.addEventListener('blur', () => this.validateField(field));
field.addEventListener('input', () => this.clearFieldError(field));
});
// Initialize custom controls
this.initializeCustomControls();
}
initializeCustomControls() {
// Initialize custom select dropdowns
const customSelects = this.form.querySelectorAll('.custom-select');
customSelects.forEach(select => new CustomSelect(select));
// Initialize other custom controls
// ...
}
validateField(field) {
const errorContainer = document.getElementById(`${field.id}-error`);
if (!errorContainer) return true;
let isValid = field.checkValidity();
let errorMessage = '';
// Handle specific validation types
if (!isValid) {
if (field.validity.valueMissing) {
errorMessage = `${field.labels[0].textContent} is required.`;
} else if (field.validity.typeMismatch) {
errorMessage = `Please enter a valid ${field.type}.`;
} else if (field.validity.patternMismatch) {
errorMessage = field.dataset.patternError || 'Please match the requested format.';
} else {
errorMessage = field.validationMessage;
}
// Set aria attributes for accessibility
field.setAttribute('aria-invalid', 'true');
field.setAttribute('aria-describedby', `${field.id}-error`);
errorContainer.textContent = errorMessage;
errorContainer.setAttribute('role', 'alert');
} else {
this.clearFieldError(field);
}
return isValid;
}
clearFieldError(field) {
const errorContainer = document.getElementById(`${field.id}-error`);
if (errorContainer) {
errorContainer.textContent = '';
errorContainer.removeAttribute('role');
}
field.removeAttribute('aria-invalid');
field.removeAttribute('aria-describedby');
}
validateForm() {
let isValid = true;
this.fields.forEach(field => {
if (!this.validateField(field)) {
isValid = false;
}
});
if (!isValid) {
// Focus the first invalid field
const firstInvalidField = this.form.querySelector('[aria-invalid="true"]');
if (firstInvalidField) {
firstInvalidField.focus();
}
// Update status for screen readers
this.updateStatus('Form has errors. Please correct them and try again.', true);
}
return isValid;
}
updateStatus(message, isError = false) {
this.statusRegion.textContent = message;
this.statusRegion.setAttribute('aria-live', isError ? 'assertive' : 'polite');
}
async handleSubmit(e) {
e.preventDefault();
if (!this.validateForm()) {
return;
}
try {
this.submitButton.disabled = true;
this.updateStatus('Submitting form...');
// Simulating form submission
await new Promise(resolve => setTimeout(resolve, 1000));
// Success handling
this.updateStatus('Form submitted successfully!');
this.form.reset();
} catch (error) {
this.updateStatus('There was an error submitting the form. Please try again.', true);
} finally {
this.submitButton.disabled = false;
}
}
}
// Initialize all forms on the page
document.addEventListener('DOMContentLoaded', () => {
const forms = document.querySelectorAll('form');
forms.forEach(form => new AccessibleForm(form));
});
Through my experience working with diverse user groups, I've found that accessible forms benefit everyone - not just those with disabilities. Clearer error messages, better keyboard support, and more thoughtful interactions make forms easier for all users.
Accessibility isn't a feature to be added after development - it's a fundamental aspect of quality web development. By incorporating these seven techniques into your development workflow, you'll create form controls that work for everyone, regardless of how they interact with your application.
Testing is crucial. I regularly test with keyboard-only navigation, screen readers like NVDA or VoiceOver, and high-contrast modes. Each testing approach reveals different aspects of accessibility that might need improvement.
The most important thing is to start incorporating these practices today. Even implementing one or two techniques will improve the experience for your users. Over time, accessible development becomes second nature, allowing you to create inclusive experiences with minimal additional effort.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva