Creating a Countdown Timer with Tkinter in Python
Introduction: In this post, we’ll walk through how to build a simple Countdown Timer using Tkinter, the standard GUI library for Python. Whether you're working on a small personal project or looking to improve your Python skills, this project is a great starting point. Project Overview: Objective: Create a countdown timer with start, pause, and reset functionality. Skills Covered: Python programming, Tkinter GUI design, time manipulation. Tools Needed: Python 3.x, Tkinter. Step 1: Setting Up the Environment Before we start coding, ensure you have Python installed with Tkinter. Most versions of Python come with Tkinter pre-installed. Check if Tkinter is installed: Open Python in the terminal and run: import tkinter If there’s no error, you’re good to go! Step 2: Designing the Timer UI Let’s create the user interface (UI) for our countdown timer using Tkinter. Components: Label for displaying the time. Entry field for setting the countdown time. Buttons for starting, pausing, and resetting the timer. Here’s the code for the UI layout: from tkinter import * # Import all components from tkinter from tkinter import messagebox # Create the main window (Master Window B) B = Tk() B.title("Countdown Timer") # Set window size B.geometry("300x200") # Time variable time_left = 0 # Timer label timer_label = Label(B, text="00:00", font=("Helvetica", 48)) timer_label.pack() # Time input entry time_input = Entry(B, font=("Helvetica", 14)) time_input.pack(pady=10) # Helper functions def start_timer(): global time_left try: time_left = int(time_input.get()) * 60 # Convert minutes to seconds countdown() except ValueError: messagebox.showerror("Invalid Input", "Please enter a valid number.") def countdown(): global time_left if time_left > 0: mins, secs = divmod(time_left, 60) timer_label.config(text=f"{mins:02d}:{secs:02d}") time_left -= 1 B.after(1000, countdown) # Update every second else: messagebox.showinfo("Time's Up!", "The timer has finished.") def reset_timer(): global time_left time_left = 0 timer_label.config(text="00:00") time_input.delete(0, END) # Buttons start_button = Button(B, text="Start", font=("Helvetica", 14), command=start_timer) start_button.pack(side="left", padx=20) reset_button = Button(B, text="Reset", font=("Helvetica", 14), command=reset_timer) reset_button.pack(side="right", padx=20) B.mainloop() Step 3: Explanation of the Code 1. Importing Tkinter: from tkinter import * imports all components from Tkinter. 2. Creating the Master Window: B = Tk() initializes the window (Master Window B), and B.title() sets its title. 3. Creating the Timer Label: timer_label is a Label widget that displays the remaining time. 4. Handling Time Input: time_input = Entry(B) allows the user to input minutes for countdown. 5. Start Timer Function: Retrieves input, converts to seconds, and begins countdown. 6. Countdown Function: Updates the timer every second using B.after(). 7. Reset Timer: Clears the countdown and resets display. Step 4: Enhancing the Project Now that we have a basic countdown timer, let’s add some enhancements: Add a Pause Button: Allow users to pause and resume the countdown. Custom Timer Settings: Background color, fonts, or sound alerts. Better Error Handling: Restrict input to valid integers only. Step 5: Conclusion By following this guide, you've created a simple yet functional countdown timer using Python and Tkinter. This helps you get hands-on with GUIs, time events, and user interaction. Feel free to extend it with: Alarm sounds A visual progress bar Mobile app version Call to Action: If you enjoyed this project, explore more tutorials on Python and Tkinter. Share your version of the timer, and comment below if you add new features! Final Thoughts This longer post with code and explanations helps beginners follow along and understand each step. Add screenshots to make it even better!
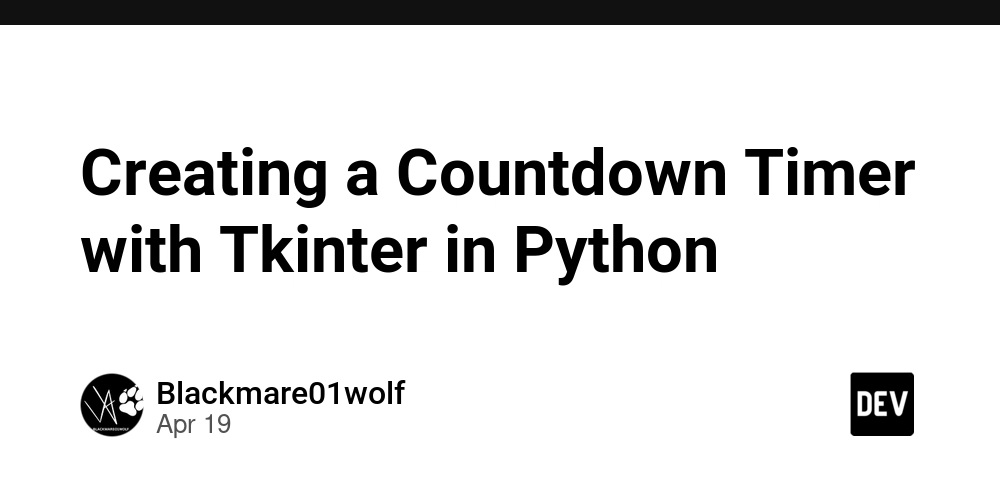
Introduction:
In this post, we’ll walk through how to build a simple Countdown Timer using Tkinter, the standard GUI library for Python. Whether you're working on a small personal project or looking to improve your Python skills, this project is a great starting point.
Project Overview:
Objective: Create a countdown timer with start, pause, and reset functionality.
Skills Covered: Python programming, Tkinter GUI design, time manipulation.
Tools Needed: Python 3.x, Tkinter.
Step 1: Setting Up the Environment
Before we start coding, ensure you have Python installed with Tkinter. Most versions of Python come with Tkinter pre-installed.
Check if Tkinter is installed: Open Python in the terminal and run:
import tkinter
If there’s no error, you’re good to go!
Step 2: Designing the Timer UI
Let’s create the user interface (UI) for our countdown timer using Tkinter.
Components:
- Label for displaying the time.
- Entry field for setting the countdown time.
- Buttons for starting, pausing, and resetting the timer.
Here’s the code for the UI layout:
from tkinter import * # Import all components from tkinter
from tkinter import messagebox
# Create the main window (Master Window B)
B = Tk()
B.title("Countdown Timer")
# Set window size
B.geometry("300x200")
# Time variable
time_left = 0
# Timer label
timer_label = Label(B, text="00:00", font=("Helvetica", 48))
timer_label.pack()
# Time input entry
time_input = Entry(B, font=("Helvetica", 14))
time_input.pack(pady=10)
# Helper functions
def start_timer():
global time_left
try:
time_left = int(time_input.get()) * 60 # Convert minutes to seconds
countdown()
except ValueError:
messagebox.showerror("Invalid Input", "Please enter a valid number.")
def countdown():
global time_left
if time_left > 0:
mins, secs = divmod(time_left, 60)
timer_label.config(text=f"{mins:02d}:{secs:02d}")
time_left -= 1
B.after(1000, countdown) # Update every second
else:
messagebox.showinfo("Time's Up!", "The timer has finished.")
def reset_timer():
global time_left
time_left = 0
timer_label.config(text="00:00")
time_input.delete(0, END)
# Buttons
start_button = Button(B, text="Start", font=("Helvetica", 14), command=start_timer)
start_button.pack(side="left", padx=20)
reset_button = Button(B, text="Reset", font=("Helvetica", 14), command=reset_timer)
reset_button.pack(side="right", padx=20)
B.mainloop()
Step 3: Explanation of the Code
1. Importing Tkinter:
from tkinter import *
imports all components from Tkinter.
2. Creating the Master Window:
B = Tk()
initializes the window (Master Window B), and B.title()
sets its title.
3. Creating the Timer Label:
timer_label
is a Label widget that displays the remaining time.
4. Handling Time Input:
time_input = Entry(B)
allows the user to input minutes for countdown.
5. Start Timer Function:
Retrieves input, converts to seconds, and begins countdown.
6. Countdown Function:
Updates the timer every second using B.after()
.
7. Reset Timer:
Clears the countdown and resets display.
Step 4: Enhancing the Project
Now that we have a basic countdown timer, let’s add some enhancements:
Add a Pause Button:
Allow users to pause and resume the countdown.Custom Timer Settings:
Background color, fonts, or sound alerts.Better Error Handling:
Restrict input to valid integers only.
Step 5: Conclusion
By following this guide, you've created a simple yet functional countdown timer using Python and Tkinter. This helps you get hands-on with GUIs, time events, and user interaction.
Feel free to extend it with:
- Alarm sounds
- A visual progress bar
- Mobile app version
Call to Action:
If you enjoyed this project, explore more tutorials on Python and Tkinter. Share your version of the timer, and comment below if you add new features!
Final Thoughts
This longer post with code and explanations helps beginners follow along and understand each step. Add screenshots to make it even better!