Concurrency in Java: A Guide for Beginners!
Overview In modern software development, concurrency is a game-changer. It allows a program to handle multiple tasks at once, improving performance. For example, in a web server, while one thread is serving a user’s request, another can be processing a database query. This makes the system feel fast and responsive, even when there are many things going on behind the scenes. In this article, we’ll dive into concurrency in Java - what it is, how to use it, and how it can be applied in real-world applications. By the end, you’ll have a foundational understanding of how to handle multiple tasks in your Java programs. Concurrency vs. Parallelism: What’s the Difference? Concurrency Concurrency is when a program handles multiple tasks at once, making progress on them even if they aren't running at the same time. For example, a web server handles multiple requests by quickly switching between them, without running them all at once. It’s like a chef cooking several dishes at the same time - stirring one pot, chopping vegetables for another, and cooking rice - without fully focusing on any single task the whole time. Parallelism Parallelism is when tasks are actually executed at the same time, usually with multiple CPU cores or processors. For example, when working with a large dataset, the tasks can be divided and processed simultaneously across different cores. It’s like each chef in a kitchen working on different parts of the same dish at the same time - one chef is chopping vegetables, another is cooking the sauce, and another is grilling the meat - all working together to complete the dish faster. When to Use Concurrency vs. Parallelism? Knowing the difference is great, but when should you actually use concurrency or parallelism in your applications? To put it simply, Use concurrency when tasks need to make progress together but don’t have to run at the exact same time. It’s useful for: Web servers - Handling multiple user requests without blocking. Mobile apps - Loading data while keeping the UI responsive. Chat applications - Sending and receiving messages without delays. Databases - Handling multiple queries without waiting for each to finish. Use parallelism when tasks can run at the exact same time, taking advantage of multiple CPU cores. It’s great for: Image processing - Applying filters to different parts of an image in parallel. Machine learning - Running computations on large datasets simultaneously. Data analysis - Processing big data across multiple threads for faster insights. Video rendering - Encoding multiple frames at the same time to speed up processing. Threads: The Building Blocks of Concurrency A thread is the smallest unit of execution within a program. In Java, a thread is like an individual worker responsible for performing a task. You can either create a thread by extending the Thread class or by implementing the Runnable interface. Here’s a quick comparison: Extending the Thread Class: class MyThread extends Thread { @Override public void run() { System.out.println("Thread is running!"); } public static void main(String[] args) { MyThread thread = new MyThread(); thread.start(); // Start the thread } } Implementing the Runnable Interface: class MyRunnable implements Runnable { @Override public void run() { System.out.println("Runnable is running!"); } public static void main(String[] args) { MyRunnable runnable = new MyRunnable(); Thread thread = new Thread(runnable); thread.start(); // Start the thread } } What Does the run() Method Do? The run() method is where you define the task that you want the thread to perform. When you create a thread, you don’t actually tell it what to do directly. Instead, you put that code inside the run() method, and the thread will execute whatever is inside it when you start the thread. Think of the run() method as the “to-do list” for your worker (thread). When you call the start() method, the thread begins executing the instructions inside the run() method, and that’s when the actual work happens. How Concurrency Works: Context Switching Now that you know what a thread is, let's take a step back to understand how concurrency actually works. When you have multiple threads running on a single CPU, only one thread can execute at a time. But how can multiple threads seem like they're running at once? The answer is context switching. What is Context Switching? Context switching happens when the CPU rapidly switches between multiple threads. It makes it appear that several tasks are being run simultaneously, even though only one is running at any given time. 1.The CPU executes one thread: The CPU runs the instructions of one thread. Time slice ends: After a small period, the operating system interrupts
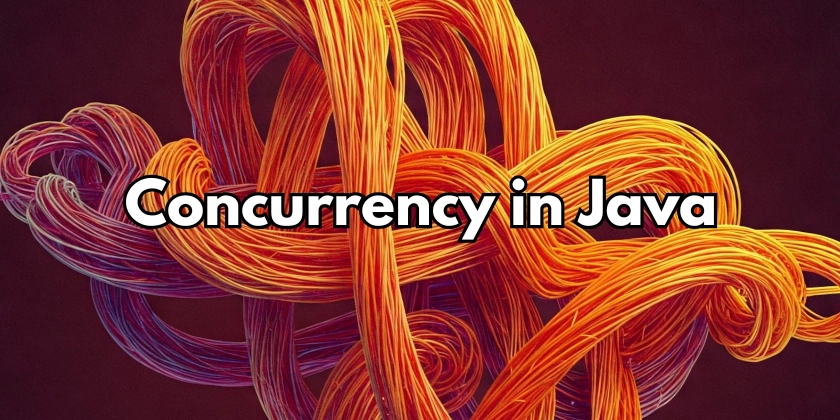
Overview
In modern software development, concurrency is a game-changer. It allows a program to handle multiple tasks at once, improving performance. For example, in a web server, while one thread is serving a user’s request, another can be processing a database query. This makes the system feel fast and responsive, even when there are many things going on behind the scenes.
In this article, we’ll dive into concurrency in Java - what it is, how to use it, and how it can be applied in real-world applications. By the end, you’ll have a foundational understanding of how to handle multiple tasks in your Java programs.
Concurrency vs. Parallelism: What’s the Difference?
Concurrency
Concurrency is when a program handles multiple tasks at once, making progress on them even if they aren't running at the same time. For example, a web server handles multiple requests by quickly switching between them, without running them all at once. It’s like a chef cooking several dishes at the same time - stirring one pot, chopping vegetables for another, and cooking rice - without fully focusing on any single task the whole time.
Parallelism
Parallelism is when tasks are actually executed at the same time, usually with multiple CPU cores or processors. For example, when working with a large dataset, the tasks can be divided and processed simultaneously across different cores. It’s like each chef in a kitchen working on different parts of the same dish at the same time - one chef is chopping vegetables, another is cooking the sauce, and another is grilling the meat - all working together to complete the dish faster.
When to Use Concurrency vs. Parallelism?
Knowing the difference is great, but when should you actually use concurrency or parallelism in your applications? To put it simply,
Use concurrency when tasks need to make progress together but don’t have to run at the exact same time. It’s useful for:
- Web servers - Handling multiple user requests without blocking.
- Mobile apps - Loading data while keeping the UI responsive.
- Chat applications - Sending and receiving messages without delays.
- Databases - Handling multiple queries without waiting for each to finish.
Use parallelism when tasks can run at the exact same time, taking advantage of multiple CPU cores. It’s great for:
- Image processing - Applying filters to different parts of an image in parallel.
- Machine learning - Running computations on large datasets simultaneously.
- Data analysis - Processing big data across multiple threads for faster insights.
- Video rendering - Encoding multiple frames at the same time to speed up processing.
Threads: The Building Blocks of Concurrency
A thread is the smallest unit of execution within a program. In Java, a thread is like an individual worker responsible for performing a task. You can either create a thread by extending the Thread
class or by implementing the Runnable
interface.
Here’s a quick comparison:
Extending the Thread
Class:
class MyThread extends Thread {
@Override
public void run() {
System.out.println("Thread is running!");
}
public static void main(String[] args) {
MyThread thread = new MyThread();
thread.start(); // Start the thread
}
}
Implementing the Runnable
Interface:
class MyRunnable implements Runnable {
@Override
public void run() {
System.out.println("Runnable is running!");
}
public static void main(String[] args) {
MyRunnable runnable = new MyRunnable();
Thread thread = new Thread(runnable);
thread.start(); // Start the thread
}
}
What Does the run()
Method Do?
The run()
method is where you define the task that you want the thread to perform. When you create a thread, you don’t actually tell it what to do directly. Instead, you put that code inside the run()
method, and the thread will execute whatever is inside it when you start the thread.
Think of the run()
method as the “to-do list” for your worker (thread). When you call the start()
method, the thread begins executing the instructions inside the run()
method, and that’s when the actual work happens.
How Concurrency Works: Context Switching
Now that you know what a thread is, let's take a step back to understand how concurrency actually works.
When you have multiple threads running on a single CPU, only one thread can execute at a time. But how can multiple threads seem like they're running at once? The answer is context switching.
What is Context Switching?
Context switching happens when the CPU rapidly switches between multiple threads. It makes it appear that several tasks are being run simultaneously, even though only one is running at any given time.
1.The CPU executes one thread: The CPU runs the instructions of one thread.
- Time slice ends: After a small period, the operating system interrupts the running thread and schedules the next one. This is often done in time slices, which can be very short.
- Context switch: The CPU saves the state (or context) of the current thread so it can resume it later. This includes things like the program counter and register values.
- Next thread runs: The CPU loads the state of the next thread and continues executing its instructions.
Thread Lifecycle: Understanding the Process
Threads go through a lifecycle, similar to workers in a production environment. Here’s what happens:
- New: Created but hasn’t started yet.
- Runnable: Ready but might be waiting for CPU time.
- Blocked: Waiting for access to a resource.
- Waiting: Paused until another thread signals it to resume.
- Terminated: Thread has completed its task.
Synchronization: Preventing Conflicts in Multi-threading
When multiple threads access shared resources, you can run into issues like race conditions. For example, if two threads try to update the same data simultaneously, the result could be incorrect or unpredictable.
To handle this, Java provides synchronization
to ensure that only one thread can access a particular resource at a time. This is crucial in real-world applications like banking systems, where two users trying to withdraw money from the same account at the same time could lead to incorrect balances.
Here’s an example of using synchronization in a Java class:
class BankAccount {
private int balance = 0;
public synchronized void deposit(int amount) {
balance += amount;
}
public synchronized void withdraw(int amount) {
balance -= amount;
}
public int getBalance() {
return balance;
}
}
In this example, the synchronized
keyword ensures that no two threads can update the balance at the same time, preventing race conditions.
ExecutorService
: Managing Multiple Tasks Efficiently
In real-world applications, especially ones that need to handle multiple tasks simultaneously (like web servers, data processing pipelines, or task schedulers), managing threads manually can become complex and error-prone. That’s where ExecutorService
comes in.
Imagine you have a file-processing system that needs to handle thousands of files. Instead of creating a new thread for each file (which would be inefficient and resource-intensive), you can use ExecutorService
to manage a pool of worker threads that handle the files concurrently. This makes your application both efficient and scalable.
Here’s an example of using ExecutorService
to handle tasks:
import java.util.concurrent.*;
class FileProcessingTask implements Callable<String> {
@Override
public String call() throws Exception {
// Simulate file processing
return "File processed successfully!";
}
public static void main(String[] args) throws ExecutionException, InterruptedException {
ExecutorService executor = Executors.newFixedThreadPool(5); // Pool of 5 threads
FileProcessingTask task = new FileProcessingTask();
Future<String> result = executor.submit(task);
System.out.println(result.get()); // Output: File processed successfully!
executor.shutdown(); // Close the executor after all tasks are done
}
}
In this example, we’ve created an ExecutorService
with a fixed thread pool. This means that we can process multiple files concurrently, but without overwhelming the system by creating too many threads.
Why Use ExecutorService
?
Using ExecutorService
simplifies task management in production applications. It allows you to:
- Reuse threads: Avoid the overhead of creating new threads every time you need one.
- Schedule tasks: Submit tasks without manually managing threads.
- Handle results: Easily get the results from tasks once they’re completed.
There's More?
While we’ve focused on ExecutorService here, Java provides many other tools to make concurrency easier and more efficient as part of its concurrency utilities. Some of these tools are designed for specific use cases, like handling scheduled tasks (ScheduledExecutorService) or forking tasks to be processed in parallel (ForkJoinPool).
In a future article, I’ll dive into more of these tools to give you a broader understanding of how to manage concurrency in Java. But for now, the ExecutorService is your go-to for most situations.
Conclusion
Java’s concurrency features - such as threads, synchronization
, and ExecutorService
- are crucial for building efficient, scalable applications. By understanding and using these tools, you can build real-world systems that handle multiple tasks concurrently, ensuring your application is responsive and optimized.
With ExecutorService
, you can easily manage concurrent tasks in production scenarios, from web servers to file-processing systems. While there’s much more to explore, this foundation will set you up for success as you dive deeper into Java’s powerful concurrency tools.