Clean Up Your React Native Imports: A Guide to Absolute Paths
Tired of messy relative paths like ../../../../components cluttering your React Native project? Absolute imports offer a cleaner, more maintainable alternative. This guide will walk you through setting them up seamlessly. Why Switch to Absolute Paths? ✅ Cleaner Code – No more counting ../ to navigate directories ✅ Better Refactoring – Move files without breaking imports ✅ Improved Readability – Instantly see where components come from ✅ Faster Development – Spend less time fixing broken paths Step 1: Install the Required Package Install babel-plugin-module-resolver to enable path aliasing: npm install babel-plugin-module-resolver --save-dev # or yarn add babel-plugin-module-resolver --dev Step 2: Configure Your Project - For JavaScript Projects (jsconfig.json) Create a jsconfig.json file in your project root (delete tsconfig.json if it exists): { "compilerOptions": { "baseUrl": "./", "paths": { "app-components/*": ["src/components/*"], "app-screens/*": ["src/screens/*"], "app-assets/*": ["src/assets/*"], "app-navigation/*": ["src/navigation/*"] } }, "exclude": ["node_modules"] } - For TypeScript Projects (tsconfig.json) If using TypeScript, create a similar tsconfig.json with matching aliases. Step 3: Update Babel Configuration - Modify babel.config.js to include the resolver plugin: module.exports = { presets: ['module:@react-native/babel-preset'], plugins: [ 'react-native-reanimated/plugin', // Keep this first if using Reanimated [ 'module-resolver', { root: ['./src'], extensions: ['.js', '.jsx', '.json'], alias: { 'app-components': './src/components', 'app-screens': './src/screens', 'app-assets': './src/assets', 'app-navigation': './src/navigation', }, }, ], ], }; Note: Remove react-native-reanimated/plugin if not using Reanimated. Example: Before vs. After ❌ Old Way (Relative Paths) import LanguageComponent from '../../../../../components/LanguageComponent'; ✅ New Way (Absolute Paths) import LanguageComponent from 'app-components/LanguageComponent'; Troubleshooting If VS Code doesn’t recognize the paths (can’t jump to file on hover/click): 1. Restart Everything Close VS Code completely Run these commands: rm -rf node_modules npm install npm start -- --reset-cache Reopen VS Code and wait for indexing 2. Verify Configurations Ensure jsconfig.json/tsconfig.json and babel.config.js match Check for typos in aliases Customization Tips
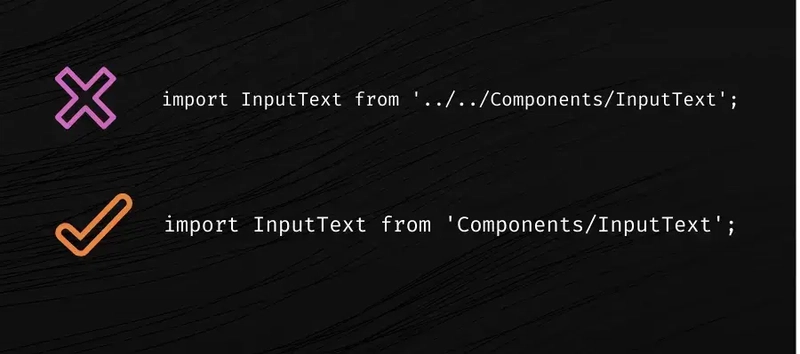
Tired of messy relative paths like ../../../../components
cluttering your React Native project? Absolute imports offer a cleaner, more maintainable alternative. This guide will walk you through setting them up seamlessly.
Why Switch to Absolute Paths?
✅ Cleaner Code – No more counting ../ to navigate directories
✅ Better Refactoring – Move files without breaking imports
✅ Improved Readability – Instantly see where components come from
✅ Faster Development – Spend less time fixing broken paths
Step 1: Install the Required Package
Install babel-plugin-module-resolver
to enable path aliasing:
npm install babel-plugin-module-resolver --save-dev
# or
yarn add babel-plugin-module-resolver --dev
Step 2: Configure Your Project
- For JavaScript Projects (jsconfig.json)
Create a jsconfig.json
file in your project root (delete tsconfig.json
if it exists):
{
"compilerOptions": {
"baseUrl": "./",
"paths": {
"app-components/*": ["src/components/*"],
"app-screens/*": ["src/screens/*"],
"app-assets/*": ["src/assets/*"],
"app-navigation/*": ["src/navigation/*"]
}
},
"exclude": ["node_modules"]
}
- For TypeScript Projects (tsconfig.json)
If using TypeScript, create a similar tsconfig.json with matching aliases.
Step 3: Update Babel Configuration
- Modify babel.config.js
to include the resolver plugin:
module.exports = {
presets: ['module:@react-native/babel-preset'],
plugins: [
'react-native-reanimated/plugin', // Keep this first if using Reanimated
[
'module-resolver',
{
root: ['./src'],
extensions: ['.js', '.jsx', '.json'],
alias: {
'app-components': './src/components',
'app-screens': './src/screens',
'app-assets': './src/assets',
'app-navigation': './src/navigation',
},
},
],
],
};
Note: Remove react-native-reanimated/plugin if not using Reanimated.
Example: Before vs. After
❌ Old Way (Relative Paths)
import LanguageComponent from '../../../../../components/LanguageComponent';
✅ New Way (Absolute Paths)
import LanguageComponent from 'app-components/LanguageComponent';
Troubleshooting
If VS Code doesn’t recognize the paths (can’t jump to file on hover/click):
1. Restart Everything
- Close VS Code completely
- Run these commands:
rm -rf node_modules
npm install
npm start -- --reset-cache
- Reopen VS Code and wait for indexing
2. Verify Configurations
- Ensure
jsconfig.json/tsconfig.json
andbabel.config.js
match - Check for typos in aliases
Customization Tips