C++ Tips and Tricks for Advanced Developers
C++ Tips and Tricks for Advanced Developers C++ remains one of the most powerful and versatile programming languages, favored for high-performance applications, game development, and systems programming. While beginners focus on syntax and basic concepts, advanced developers can leverage deeper techniques to optimize performance, improve maintainability, and write more expressive code. In this article, we’ll explore some advanced C++ tips and tricks that can elevate your programming skills. And if you're looking to monetize your web development expertise, consider checking out MillionFormula for opportunities to turn your skills into income. 1. Move Semantics and Perfect Forwarding Move semantics, introduced in C++11, allow for more efficient resource management by avoiding unnecessary copies. Instead of duplicating data, we "move" it, transferring ownership. Example: Using std::move cppCopyDownload#include #include std::vector createLargeVector() { std::vector vec(1000000, 42); // Large vector return vec; // NRVO (Named Return Value Optimization) may apply } int main() { std::vector v = createLargeVector(); // Efficient due to move semantics std::vector v2 = std::move(v); // Explicit move, v is now empty } Perfect Forwarding ensures that arguments passed to a function retain their value category (lvalue or rvalue). This is crucial for template functions: cppCopyDownloadtemplate void wrapper(T&& arg) { // Forward arg as-is (lvalue or rvalue) someOtherFunction(std::forward(arg)); } 2. Smart Pointers for Memory Safety Raw pointers are error-prone. Instead, use smart pointers (std::unique_ptr, std::shared_ptr, std::weak_ptr) for automatic memory management. Example: std::unique_ptr cppCopyDownload#include void processData() { auto ptr = std::make_unique(42); // Ownership is clear // No need to delete, memory is freed when ptr goes out of scope } Example: std::shared_ptr with Custom Deleters cppCopyDownloadauto deleter = [](FILE f) { fclose(f); }; std::shared_ptr filePtr(fopen("data.txt", "r"), deleter); 3. Compile-Time Computation with constexpr C++11 introduced constexpr, allowing computations at compile time for better performance. Example: Compile-Time Factorial cppCopyDownloadconstexpr int factorial(int n) { return (n std::pair { return {42, "Alice"}; } int main() { auto [id, name] = getUser(); // Structured binding // id = 42, name = "Alice" } Works with std::tuple and custom types via std::get specialization. 6. Inline Assembly for Low-Level Optimizations When absolute performance is needed, inline assembly can be used (though compiler intrinsics are often better). Example: Fast Multiply Using Inline ASM (x86) cppCopyDownloadint fastMultiply(int a, int b) { int result; asm("imul %1, %0" : "=r"(result) : "r"(a), "0"(b)); return result; } Note: Inline assembly is compiler-specific. Modern C++ prefers intrinsics ( for SIMD). 7. Benchmarking with std::chrono Optimizing code requires measuring performance. C++11’s provides high-resolution timing. Example: Timing a Function cppCopyDownload#include #include void expensiveFunction() { // Simulate work volatile int x = 0; for (int i = 0; i
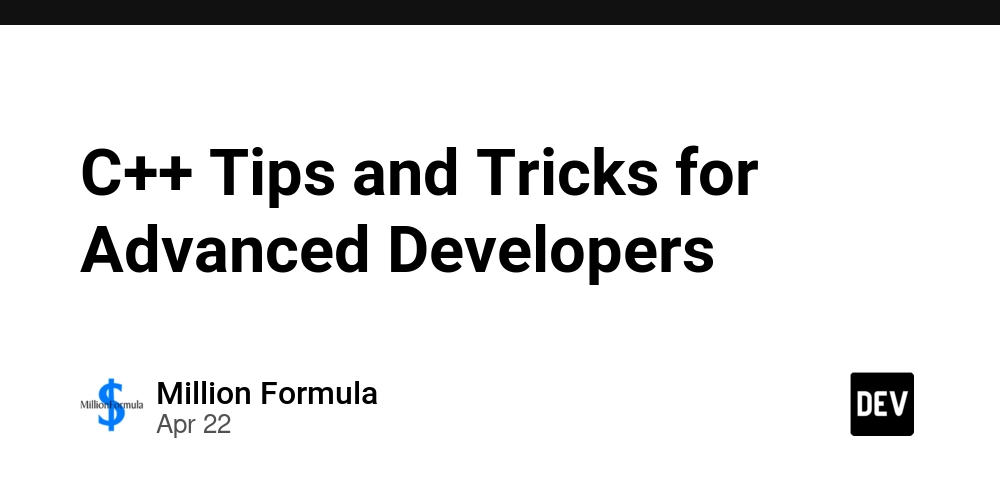
C++ Tips and Tricks for Advanced Developers
C++ remains one of the most powerful and versatile programming languages, favored for high-performance applications, game development, and systems programming. While beginners focus on syntax and basic concepts, advanced developers can leverage deeper techniques to optimize performance, improve maintainability, and write more expressive code.
In this article, we’ll explore some advanced C++ tips and tricks that can elevate your programming skills. And if you're looking to monetize your web development expertise, consider checking out MillionFormula for opportunities to turn your skills into income.
1. Move Semantics and Perfect Forwarding
Move semantics, introduced in C++11, allow for more efficient resource management by avoiding unnecessary copies. Instead of duplicating data, we "move" it, transferring ownership.
Example: Using std::move
cppCopyDownload#include
#includestd::vector<int> createLargeVector() {
std::vector<int> vec(1000000, 42); // Large vector
return vec; // NRVO (Named Return Value Optimization) may apply
}int main() {
std::vector<int> v = createLargeVector(); // Efficient due to move semantics
std::vector<int> v2 = std::move(v); // Explicit move, v is now empty
}
Perfect Forwarding ensures that arguments passed to a function retain their value category (lvalue
or rvalue
). This is crucial for template functions:
cppCopyDownload
template<typename T>
void wrapper(T&& arg) {
// Forward arg as-is (lvalue or rvalue)
someOtherFunction(std::forward<T>(arg));
}
2. Smart Pointers for Memory Safety
Raw pointers are error-prone. Instead, use smart pointers (std::unique_ptr
, std::shared_ptr
, std::weak_ptr
) for automatic memory management.
Example: std::unique_ptr
cppCopyDownload#includevoid processData() {
auto ptr = std::make_unique<int>(42); // Ownership is clear
// No need to delete, memory is freed when ptr goes out of scope
}
Example: std::shared_ptr
with Custom Deleters
cppCopyDownloadauto deleter = [](FILE f) { fclose(f); };
std::shared_ptr<FILE> filePtr(fopen("data.txt", "r"), deleter);
3. Compile-Time Computation with constexpr
C++11 introduced constexpr
, allowing computations at compile time for better performance.
Example: Compile-Time Factorial
cppCopyDownloadconstexpr int factorial(int n) {
return (n <= 1) ? 1 : (n factorial(n - 1));
}int main() {
constexpr int val = factorial(5); // Evaluated at compile-time
static_assert(val == 120, "Factorial of 5 should be 120");
}
C++20 expands this with consteval
(immediate functions) and constinit
.
4. Metaprogramming with Templates and Concepts (C++20)
Templates enable generic programming, but C++20’s Concepts make them more readable and enforceable.
Example: Using Concepts
cppCopyDownload#includetemplate<typename T>
requires std::integral<T>
T square(T x) {
return x * x;
}int main() {
auto result = square(5); // Works
// auto fail = square(3.14); // Compile-time error (floating-point not allowed)
}
5. Structured Bindings for Cleaner Code
C++17 introduced structured bindings, allowing multiple return values to be unpacked elegantly.
Example: Decomposing a std::pair
cppCopyDownload#includeauto getUser() -> std::pair<int, std::string> {
return {42, "Alice"};
}int main() {
auto [id, name] = getUser(); // Structured binding
// id = 42, name = "Alice"
}
Works with std::tuple
and custom types via std::get
specialization.
6. Inline Assembly for Low-Level Optimizations
When absolute performance is needed, inline assembly can be used (though compiler intrinsics are often better).
Example: Fast Multiply Using Inline ASM (x86)
cppCopyDownloadint fastMultiply(int a, int b) {
int result;
asm("imul %1, %0" : "=r"(result) : "r"(a), "0"(b));
return result;
}
Note: Inline assembly is compiler-specific. Modern C++ prefers intrinsics (
for SIMD).
7. Benchmarking with std::chrono
Optimizing code requires measuring performance. C++11’s
provides high-resolution timing.
Example: Timing a Function
cppCopyDownload#include
#includevoid expensiveFunction() {
// Simulate work
volatile int x = 0;
for (int i = 0; i < 1000000; ++i) x += i;
}int main() {
auto start = std::chrono::high_resolution_clock::now();
expensiveFunction();
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::microseconds>(end - start);
std::cout << "Time taken: " << duration.count() << "μs\n";
}
For advanced profiling, consider tools like Google Benchmark.
8. Custom Allocators for Performance-Critical Code
The default new
/delete
may not be optimal for all use cases. Custom allocators can reduce fragmentation.
Example: Using a Pool Allocator
cppCopyDownload#include
#includetemplate<typename T>
struct PoolAllocator {
using value_type = T;T* allocate(size_t n) { // Custom allocation logic (e.g., memory pool) return static_cast<T*>(::operator new(n * sizeof(T))); } void deallocate(T* p, size_t n) { ::operator delete(p); }
};
int main() {
std::vector<int, PoolAllocator<int>> vec;
vec.push_back(42); // Uses custom allocator
}
9. Coroutines for Asynchronous Code (C++20)
Coroutines simplify asynchronous programming by allowing suspension/resumption of functions.
Example: Simple Coroutine
cppCopyDownload#include
#includestruct Task {
struct promise_type {
Task get_return_object() { return {}; }
std::suspend_never initial_suspend() { return {}; }
std::suspend_never final_suspend() noexcept { return {}; }
void return_void() {}
void unhandled_exception() {}
};
};Task myCoroutine() {
std::cout << "Coroutine running\n";
co_return; // Suspends and resumes
}int main() {
myCoroutine();
}
For production use, consider cppcoro.
10. Undefined Behavior Sanitizers (UBSan) for Debugging
C++ is prone to undefined behavior (UB). Tools like UBSan catch these issues at runtime.
Compiling with UBSan (GCC/Clang)
shCopyDownloadg++ -fsanitize=undefined -fno-sanitize-recover program.cpp -o program
Detects issues like:
Null pointer dereferences
Integer overflow
Misaligned accesses
Final Thoughts
Mastering these advanced C++ techniques can significantly improve your code’s efficiency, safety, and maintainability. Whether you're working on game engines, high-frequency trading systems, or embedded applications, these tricks will give you an edge.
And if you're looking to leverage your programming skills for financial gain, don’t forget to explore MillionFormula for ways to monetize your expertise in web development.
Happy coding!