Building a Sequential Data Fetching API with JavaScript
The Challenge: Taming Async Operations Ever tried juggling multiple API calls and ended up with spaghetti code? You're not alone! In this project, we'll transform chaotic API requests into a clean, sequential flow using the Pokémon API. What's This Async/Await Thing Anyway? Before we dive in, let's break down some terms that might be new to beginners: Asynchronous (async) is like that friend who says "I'll get back to you later!" When your code makes an async request, it doesn't sit around waiting - it continues running other tasks while that request works in the background. Await is like saying "hold up, I really need this information before moving on." It tells your code to pause at that specific spot until the async task finishes. Together, async/await creates code that reads like a step-by-step recipe, even though some steps might take time to complete. It's JavaScript's way of handling things that don't happen instantly (like fetching data from the internet). What We're Building We're creating a simple API client that fetches Pokémon data in a specific order. No more callback hell or promise confusion—just clean, readable code that even your non-technical friends could understand (almost!). Key Features Clean Async Code: Using modern async/await syntax that's actually readable Sequential Fetching: Controlling the exact order of our API requests (like waiting for your first Pokémon's data before getting the next one) Error Handling: Because Pokémon servers sometimes need a rest too! Project Structure at a Glance day-5-project/ ├── src/ │ ├── services/ │ │ └── fetchSequentially.js # Where the magic happens │ ├── utils/ │ │ └── Logger.js # Keeps our console logs tidy │ └── index.js # The starting point ├── package.json # Our dependencies └── README.md # You are here! The Code: Step-by-Step Breakdown 1. The Fetch Service: Where Data Comes to Life In fetchSequentially.js, we define our core fetching logic: // This function is labeled "async" which is like telling JavaScript // "Hey, I'll need to wait for some things inside this function" const fetchSequentiallyWithAsyncAwait = async () => { const endpoint = "https://pokeapi.co/api/v2/pokemon/species/1"; try { console.log(`Fetching data from ${endpoint}...`); // The "await" keyword here is like saying "pause here until we get a response" // Without it, response would be a Promise object, not the actual data const response = await fetch(endpoint); // Same here - wait until the JSON data is extracted before continuing const data = await response.json(); return data; } catch (error) { // If anything goes wrong above, we land here console.error(`Error fetching data: ${error.message}`); return null; } }; module.exports = { fetchSequentiallyWithAsyncAwait }; 2. The Main Function: Putting It All Together Our index.js file orchestrates the entire process: const { fetchSequentiallyWithAsyncAwait } = require('./services/fetchSequentially'); const { logResults } = require('./utils/Logger'); // Another async function since we need to await our fetch results const main = async () => { console.log('Starting our Pokémon data adventure...'); // Wait for the Pokémon data before moving on const results = await fetchSequentiallyWithAsyncAwait(); // Once we have the data, then log it logResults(results); }; // Start the program and handle any major errors main().catch(error => console.error('Something went wrong:', error)); 3. The Logger: Making Sense of the Data To keep things organized, we use a simple logger in Logger.js: const logResults = (results) => { if (!results) { console.log('No results to display'); return; } console.log('✅ Successfully fetched Pokémon data:'); console.log(`Name: ${results.name}`); console.log(`Generation: ${results.generation.name}`); // Add more specific data points you want to highlight }; module.exports = { logResults }; Running Your Creation Fire up your terminal and run: node src/index.js You'll see your console light up with Pokémon data, neatly presented and error-free! What's Really Happening Behind the Scenes? Think of our code like ordering food at a restaurant: You place your order (make an API request) Instead of just standing at the counter waiting, you sit down at your table (your code continues running) When your order is ready, the server brings it to you (the Promise resolves) Now you can enjoy your meal (use the data) The async/await syntax handles all this complexity for us, making our code read almost like synchronous code even though it's dealing with operations that take time. What's Next? Now
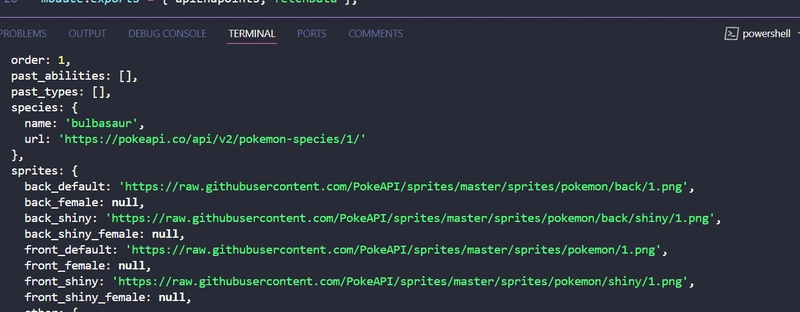
The Challenge: Taming Async Operations
Ever tried juggling multiple API calls and ended up with spaghetti code? You're not alone! In this project, we'll transform chaotic API requests into a clean, sequential flow using the Pokémon API.
What's This Async/Await Thing Anyway?
Before we dive in, let's break down some terms that might be new to beginners:
Asynchronous (async) is like that friend who says "I'll get back to you later!" When your code makes an async request, it doesn't sit around waiting - it continues running other tasks while that request works in the background.
Await is like saying "hold up, I really need this information before moving on." It tells your code to pause at that specific spot until the async task finishes.
Together, async/await creates code that reads like a step-by-step recipe, even though some steps might take time to complete. It's JavaScript's way of handling things that don't happen instantly (like fetching data from the internet).
What We're Building
We're creating a simple API client that fetches Pokémon data in a specific order. No more callback hell or promise confusion—just clean, readable code that even your non-technical friends could understand (almost!).
Key Features
- Clean Async Code: Using modern async/await syntax that's actually readable
- Sequential Fetching: Controlling the exact order of our API requests (like waiting for your first Pokémon's data before getting the next one)
- Error Handling: Because Pokémon servers sometimes need a rest too!
Project Structure at a Glance
day-5-project/
├── src/
│ ├── services/
│ │ └── fetchSequentially.js # Where the magic happens
│ ├── utils/
│ │ └── Logger.js # Keeps our console logs tidy
│ └── index.js # The starting point
├── package.json # Our dependencies
└── README.md # You are here!
The Code: Step-by-Step Breakdown
1. The Fetch Service: Where Data Comes to Life
In fetchSequentially.js
, we define our core fetching logic:
// This function is labeled "async" which is like telling JavaScript
// "Hey, I'll need to wait for some things inside this function"
const fetchSequentiallyWithAsyncAwait = async () => {
const endpoint = "https://pokeapi.co/api/v2/pokemon/species/1";
try {
console.log(`Fetching data from ${endpoint}...`);
// The "await" keyword here is like saying "pause here until we get a response"
// Without it, response would be a Promise object, not the actual data
const response = await fetch(endpoint);
// Same here - wait until the JSON data is extracted before continuing
const data = await response.json();
return data;
} catch (error) {
// If anything goes wrong above, we land here
console.error(`Error fetching data: ${error.message}`);
return null;
}
};
module.exports = { fetchSequentiallyWithAsyncAwait };
2. The Main Function: Putting It All Together
Our index.js
file orchestrates the entire process:
const { fetchSequentiallyWithAsyncAwait } = require('./services/fetchSequentially');
const { logResults } = require('./utils/Logger');
// Another async function since we need to await our fetch results
const main = async () => {
console.log('Starting our Pokémon data adventure...');
// Wait for the Pokémon data before moving on
const results = await fetchSequentiallyWithAsyncAwait();
// Once we have the data, then log it
logResults(results);
};
// Start the program and handle any major errors
main().catch(error => console.error('Something went wrong:', error));
3. The Logger: Making Sense of the Data
To keep things organized, we use a simple logger in Logger.js
:
const logResults = (results) => {
if (!results) {
console.log('No results to display');
return;
}
console.log('✅ Successfully fetched Pokémon data:');
console.log(`Name: ${results.name}`);
console.log(`Generation: ${results.generation.name}`);
// Add more specific data points you want to highlight
};
module.exports = { logResults };
Running Your Creation
Fire up your terminal and run:
node src/index.js
You'll see your console light up with Pokémon data, neatly presented and error-free!
What's Really Happening Behind the Scenes?
Think of our code like ordering food at a restaurant:
- You place your order (make an API request)
- Instead of just standing at the counter waiting, you sit down at your table (your code continues running)
- When your order is ready, the server brings it to you (the Promise resolves)
- Now you can enjoy your meal (use the data)
The async/await
syntax handles all this complexity for us, making our code read almost like synchronous code even though it's dealing with operations that take time.
What's Next?
Now that you've mastered the basics:
- Try fetching multiple Pokémon in sequence
- Add a simple UI to display your Pokémon data
- Create a comparison feature between different Pokémon types
Key Takeaways
- Async/await makes asynchronous code feel synchronous
- Proper error handling prevents your app from crashing
- Structured code organization makes future extensions easier
Happy coding, and may your API requests always return 200 OK!