All React Concepts in One Read: Interview Insights
If you're diving into React, you might have come across some confusing concepts. Don't worry—I'm here to break it all down for you! With 8 years of experience under my belt, I’ll make sure you understand React in a clear, digestible way. Let’s go over the essential React concepts one by one. 1️⃣ Components: The Building Blocks of React In React, components are the heart and soul of your app. They're the modular units that represent parts of your UI, like buttons, inputs, or even entire pages. What are they? Components are JavaScript functions that return markup. Think of them as the "Lego blocks" of your app—you can reuse them as many times as you want. The more components you create, the more manageable your app becomes. 2️⃣ JSX: JavaScript with a Touch of HTML React uses JSX, which is a special syntax that looks similar to HTML but has some unique properties. JSX allows you to write components with HTML-like syntax in your JavaScript code. Why use it? While JSX is optional, it’s preferred because using plain createElement calls can get cumbersome. Plus, JSX enables you to combine HTML structure with JavaScript logic seamlessly. Important note: In JSX, you need to use camelCase for attributes, so class becomes className, and for becomes htmlFor. 3️⃣ Curly Braces: Making React Dynamic React allows you to use dynamic values in JSX through curly braces {}. This makes it easier to inject JavaScript variables and expressions into your markup. Example: If you have a variable holding a user’s name, you can display it in your JSX with curly braces: Hello, {userName}! You can also use curly braces to pass dynamic values to attributes and apply inline styles. 4️⃣ Fragments: Avoid Extra DOM Elements In React, every component must return a single parent element. This can be restrictive if you don’t want to add unnecessary wrapping elements like . Solution: Fragments let you return multiple elements without introducing extra nodes in the DOM. You can use or the shorthand to wrap your elements. Example: Title Some content here. 5️⃣ Props: Passing Data Between Components Props (short for "properties") allow you to pass data from a parent component to a child component. They’re immutable, meaning the child cannot modify them. How to use: Pass props like attributes when rendering components. In the child component, you access them as parameters. const WelcomeMessage = (props) => Hello, {props.name}!; 6️⃣ Children: Passing Components as Props React allows you to pass components as props using the children prop. This is extremely useful for composing UI elements and creating flexible components. Example: const Card = ({ children }) => {children}; Card Title Card content goes here. 7️⃣ Keys: Helping React Identify Elements When you render a list of components, you need to assign a key prop to each one. This helps React identify which items have changed, are added, or are removed. Why is it important? It improves performance and ensures React can efficiently re-render the list. const items = ['Item 1', 'Item 2', 'Item 3']; const listItems = items.map((item, index) => {item}); 8️⃣ Rendering: How React Renders Components Rendering is the process where React updates the user interface. React uses the Virtual DOM (VDOM) to perform efficient updates: React changes the virtual DOM when state or props change. It compares the new VDOM to the old one (called diffing). React updates only the parts of the actual DOM that have changed (this is called reconciliation). 9️⃣ Event Handling: Reacting to User Interactions React provides event handling to detect and respond to user actions like clicks, key presses, and form submissions. Common events include onClick, onChange, and onSubmit. const handleClick = () => alert('Button clicked!'); Click Me
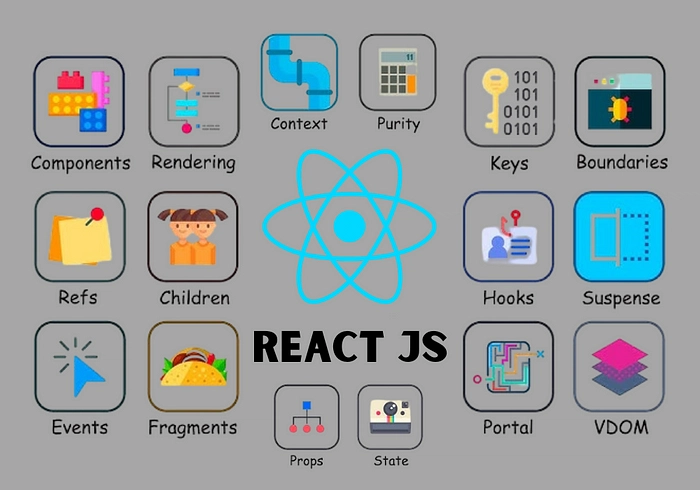
If you're diving into React, you might have come across some confusing concepts. Don't worry—I'm here to break it all down for you! With 8 years of experience under my belt, I’ll make sure you understand React in a clear, digestible way.
Let’s go over the essential React concepts one by one.
1️⃣ Components: The Building Blocks of React
In React, components are the heart and soul of your app. They're the modular units that represent parts of your UI, like buttons, inputs, or even entire pages.
- What are they? Components are JavaScript functions that return markup. Think of them as the "Lego blocks" of your app—you can reuse them as many times as you want. The more components you create, the more manageable your app becomes.
2️⃣ JSX: JavaScript with a Touch of HTML
React uses JSX, which is a special syntax that looks similar to HTML but has some unique properties. JSX allows you to write components with HTML-like syntax in your JavaScript code.
-
Why use it? While JSX is optional, it’s preferred because using plain
createElement
calls can get cumbersome. Plus, JSX enables you to combine HTML structure with JavaScript logic seamlessly. -
Important note: In JSX, you need to use camelCase for attributes, so
class
becomesclassName
, andfor
becomeshtmlFor
.
3️⃣ Curly Braces: Making React Dynamic
React allows you to use dynamic values in JSX through curly braces {}
. This makes it easier to inject JavaScript variables and expressions into your markup.
- Example: If you have a variable holding a user’s name, you can display it in your JSX with curly braces:
<p>Hello, {userName}!p>
You can also use curly braces to pass dynamic values to attributes and apply inline styles.
4️⃣ Fragments: Avoid Extra DOM Elements
In React, every component must return a single parent element. This can be restrictive if you don’t want to add unnecessary wrapping elements like Example: Props (short for "properties") allow you to pass data from a parent component to a child component. They’re immutable, meaning the child cannot modify them.
React allows you to pass components as props using the When you render a list of components, you need to assign a key prop to each one. This helps React identify which items have changed, are added, or are removed.
Rendering is the process where React updates the user interface. React uses the Virtual DOM (VDOM) to perform efficient updates:
React provides event handling to detect and respond to user actions like clicks, key presses, and form submissions.
or the shorthand <> >
to wrap your elements.
<React.Fragment>
<h1>Titleh1>
<p>Some content here.p>
React.Fragment>
5️⃣ Props: Passing Data Between Components
const WelcomeMessage = (props) => <h1>Hello, {props.name}!h1>;
<WelcomeMessage name="John" />
6️⃣ Children: Passing Components as Props
children
prop. This is extremely useful for composing UI elements and creating flexible components.
const Card = ({ children }) => <div className="card">{children}div>;
<Card>
<h2>Card Titleh2>
<p>Card content goes here.p>
Card>
7️⃣ Keys: Helping React Identify Elements
const items = ['Item 1', 'Item 2', 'Item 3'];
const listItems = items.map((item, index) => <li key={index}>{item}li>);
8️⃣ Rendering: How React Renders Components
9️⃣ Event Handling: Reacting to User Interactions
onClick
, onChange
, and onSubmit
.
const handleClick = () => alert('Button clicked!');
<button onClick={handleClick}>Click Mebutton>