Advantages of Java Stream API
Java Stream API was introduced in Java 8 to process collections of data in a functional programming style. It provides a way to perform operations like filtering, mapping, reducing, and sorting on collections efficiently. Advantages of Java Stream API Concise and Readable Code Reduces boilerplate code by replacing traditional for and while loops with declarative operations like filter(), map(), and forEach(). Example: List names = List.of("Alice", "Bob", "Charlie"); names.stream() .filter(name -> name.startsWith("A")) .forEach(System.out::println); // Alice Improved Performance with Lazy Evaluation Streams use lazy evaluation, meaning operations are only executed when needed, optimizing performance. Intermediate operations (filter(), map()) are not executed immediately; they wait until a terminal operation (collect(), forEach(), count()) is called. Parallel Processing (Better Performance on Multi-Core CPUs) Streams support parallel execution with parallelStream(), enabling better performance on multi-core processors. Example: List numbers = List.of(1, 2, 3, 4, 5); int sum = numbers.parallelStream().mapToInt(n -> n * 2).sum(); System.out.println(sum); // 30 Functional and Declarative Programming Style Encourages functional programming by allowing the use of lambda expressions and method references. No need for external iteration using loops. Reduces Side Effects and Mutability Issues Streams work on immutable data, reducing the chances of unexpected side effects and making the code thread-safe in many cases. Better Memory Efficiency Streams process elements on-demand, avoiding unnecessary memory usage (compared to creating new collections for intermediate results). Wide Range of Built-in Operations Supports various operations like: Filtering (filter()) Transformation (map()) Aggregation (reduce(), count(), sum()) Sorting (sorted()) Collecting results (collect()) Easier Data Transformation The map() function allows transforming one type of data into another easily. Example: List names = List.of("Alice", "Bob", "Charlie"); List nameLengths = names.stream() .map(String::length) .toList(); System.out.println(nameLengths); // [5, 3, 7] Built-in Support for Aggregation Allows easy counting, summing, and reducing operations. Example: int total = List.of(1, 2, 3, 4, 5).stream().reduce(0, Integer::sum); System.out.println(total); // 15 Conclusion Java Stream API provides concise, efficient, and parallelizable ways to work with collections, making code more readable and maintainable while improving performance in many cases. However, developers should use parallel streams cautiously to avoid unnecessary overhead in small datasets.
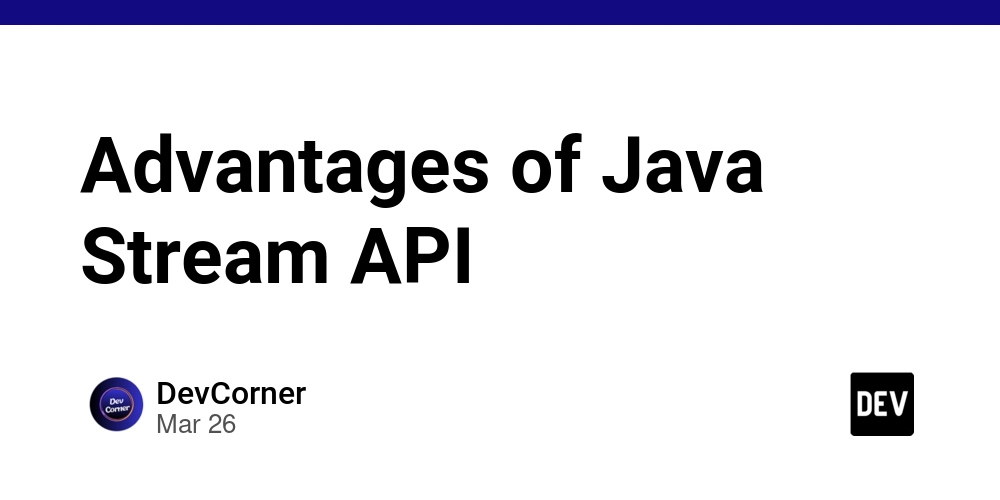
Java Stream API was introduced in Java 8 to process collections of data in a functional programming style. It provides a way to perform operations like filtering, mapping, reducing, and sorting on collections efficiently.
Advantages of Java Stream API
-
Concise and Readable Code
- Reduces boilerplate code by replacing traditional
for
andwhile
loops with declarative operations likefilter()
,map()
, andforEach()
. - Example:
List<String> names = List.of("Alice", "Bob", "Charlie"); names.stream() .filter(name -> name.startsWith("A")) .forEach(System.out::println); // Alice
- Reduces boilerplate code by replacing traditional
-
Improved Performance with Lazy Evaluation
- Streams use lazy evaluation, meaning operations are only executed when needed, optimizing performance.
- Intermediate operations (
filter()
,map()
) are not executed immediately; they wait until a terminal operation (collect()
,forEach()
,count()
) is called.
-
Parallel Processing (Better Performance on Multi-Core CPUs)
- Streams support parallel execution with
parallelStream()
, enabling better performance on multi-core processors. - Example:
List<Integer> numbers = List.of(1, 2, 3, 4, 5); int sum = numbers.parallelStream().mapToInt(n -> n * 2).sum(); System.out.println(sum); // 30
- Streams support parallel execution with
-
Functional and Declarative Programming Style
- Encourages functional programming by allowing the use of lambda expressions and method references.
- No need for external iteration using loops.
-
Reduces Side Effects and Mutability Issues
- Streams work on immutable data, reducing the chances of unexpected side effects and making the code thread-safe in many cases.
-
Better Memory Efficiency
- Streams process elements on-demand, avoiding unnecessary memory usage (compared to creating new collections for intermediate results).
-
Wide Range of Built-in Operations
- Supports various operations like:
-
Filtering (
filter()
) -
Transformation (
map()
) -
Aggregation (
reduce()
,count()
,sum()
) -
Sorting (
sorted()
) -
Collecting results (
collect()
)
-
Filtering (
- Supports various operations like:
-
Easier Data Transformation
- The
map()
function allows transforming one type of data into another easily. - Example:
List<String> names = List.of("Alice", "Bob", "Charlie"); List<Integer> nameLengths = names.stream() .map(String::length) .toList(); System.out.println(nameLengths); // [5, 3, 7]
- The
-
Built-in Support for Aggregation
- Allows easy counting, summing, and reducing operations.
- Example:
int total = List.of(1, 2, 3, 4, 5).stream().reduce(0, Integer::sum); System.out.println(total); // 15
Conclusion
Java Stream API provides concise, efficient, and parallelizable ways to work with collections, making code more readable and maintainable while improving performance in many cases. However, developers should use parallel streams cautiously to avoid unnecessary overhead in small datasets.