5 Ways to Center a Div in CSS
Centering a div in CSS is a common task that can be accomplished in multiple ways, depending on your requirements. Whether you need to center it horizontally, vertically, or both, here are five effective methods to achieve this: 1. Using Flexbox Flexbox is one of the easiest and most powerful ways to center a div. .container { display: flex; justify-content: center; /* Centers horizontally */ align-items: center; /* Centers vertically */ height: 100vh; /* Full viewport height */ } This method works perfectly for centering both horizontally and vertically within a parent container. 2. Using Grid Layout CSS Grid also provides a simple way to center a div. .container { display: grid; place-items: center; height: 100vh; } The place-items: center; property centers the child both horizontally and vertically. 3. Using Position and Transform If you prefer using absolute positioning, you can achieve centering with transform. .container { position: relative; height: 100vh; } .child { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); } This moves the div to the center while ensuring it stays perfectly aligned. 4. Using Margin Auto (For Horizontal Centering Only) If you only need to center a block-level element horizontally, margin: auto; is a simple approach. .child { width: 200px; margin: 0 auto; } This method works because block elements by default take up the full width of their container. 5. Using Table Display Setting the parent as a table and the child as a table cell enables vertical centering. .container { display: table; width: 100%; height: 100vh; } .child { display: table-cell; vertical-align: middle; text-align: center; } This method is a legacy technique but can still be useful in some cases. Conclusion Each of these methods has its use cases. Flexbox and Grid are modern and highly recommended for most applications, while positioning and table display methods are useful in specific scenarios. Choose the one that best fits your design needs!
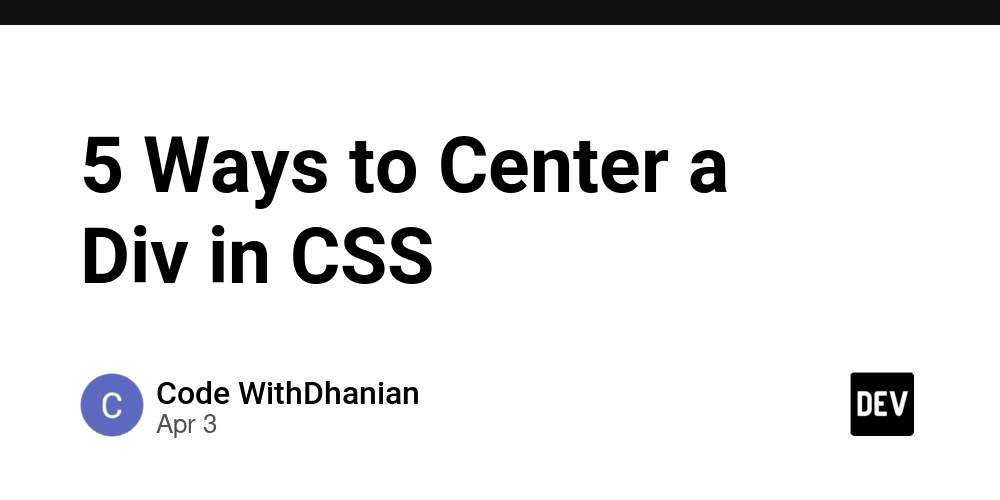
Centering a div
in CSS is a common task that can be accomplished in multiple ways, depending on your requirements. Whether you need to center it horizontally, vertically, or both, here are five effective methods to achieve this:
1. Using Flexbox
Flexbox is one of the easiest and most powerful ways to center a div
.
.container {
display: flex;
justify-content: center; /* Centers horizontally */
align-items: center; /* Centers vertically */
height: 100vh; /* Full viewport height */
}
This method works perfectly for centering both horizontally and vertically within a parent container.
2. Using Grid Layout
CSS Grid also provides a simple way to center a div
.
.container {
display: grid;
place-items: center;
height: 100vh;
}
The place-items: center;
property centers the child both horizontally and vertically.
3. Using Position and Transform
If you prefer using absolute positioning, you can achieve centering with transform
.
.container {
position: relative;
height: 100vh;
}
.child {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
This moves the div
to the center while ensuring it stays perfectly aligned.
4. Using Margin Auto (For Horizontal Centering Only)
If you only need to center a block-level element horizontally, margin: auto;
is a simple approach.
.child {
width: 200px;
margin: 0 auto;
}
This method works because block elements by default take up the full width of their container.
5. Using Table Display
Setting the parent as a table and the child as a table cell enables vertical centering.
.container {
display: table;
width: 100%;
height: 100vh;
}
.child {
display: table-cell;
vertical-align: middle;
text-align: center;
}
This method is a legacy technique but can still be useful in some cases.
Conclusion
Each of these methods has its use cases. Flexbox and Grid are modern and highly recommended for most applications, while positioning and table display methods are useful in specific scenarios. Choose the one that best fits your design needs!