#2 Refactoring Fidely & Tackling Side Projects
Hello everyone, It’s been a while since my last update in The 10-Minute Company series. I originally thought I’d be able to share progress sooner, but the holiday season (and a few unexpected projects) absorbed much of my free time. Nevertheless, I made some good progress—both on Fidely and on a smaller side project that popped up in early January. A Quick Detour: Building a Landing Page with Next.js During the first two weeks of January, I helped a friend who offers online consultations by building a simple landing page for her services. The project was straightforward but fun, and it gave me a chance to work with: Next.js for the frontend, Brevo (formerly Sendinblue) for handling newsletter subscriptions and automated emails, Brevo Meeting for scheduling online consultations, Clerk.com for authentication and an admin dashboard, Stripe for handling payments and checkout. One of the most interesting parts of the project was setting up an automated workflow: when someone subscribes to the newsletter and completes the double opt-in, they receive a welcome coupon code via email. This ensures only verified users get access to the special offer, adding an extra layer of engagement and trust. Now, back to Fidely. Returning to Fidely: Cleaning Up the Backend With the side project wrapped up, I finally got back to working on Fidely. The focus of the last few days has been restructuring the backend to improve maintainability and scalability. Here’s what I worked on: 1. Refactoring API Routes I split API endpoints more logically, ensuring that different resources and functionalities were correctly separated. This cleanup makes the backend easier to navigate and will help with future expansions. Here’s a quick look at the updated routing system: import { Router } from "express"; import authenticate from "../../middlewares/authenticate"; import authRoutes from "./auth"; import companiesRoutes from "./companies"; import campaignsRoutes from "./campaigns"; import cardCampaignsRoutes from "./card-campaigns"; import cardsRoutes from "./cards"; import transactionsRoutes from "./transactions"; import campaignBuildersRoutes from "./campaign-builders"; const v1Routes = Router(); // Public routes (no auth required) v1Routes.use("/auth", authRoutes); // Protected routes v1Routes.use("/companies", authenticate, companiesRoutes); v1Routes.use("/campaigns", authenticate, campaignsRoutes); v1Routes.use("/card-campaigns", authenticate, cardCampaignsRoutes); v1Routes.use("/cards", authenticate, cardsRoutes); v1Routes.use("/transactions", authenticate, transactionsRoutes); v1Routes.use("/campaign-builders", authenticate, campaignBuildersRoutes); export default v1Routes; 2. Creating a Simple Seeding System Manually setting up test data was becoming a hassle, so I implemented an automated seeding script that: Clears the database, Inserts fake data for testing and demo purposes. Here’s the updated seed system: import { SeedContext } from './types'; import { seedUsers } from './users'; import { seedCompanies } from './companies'; import { seedCampaigns } from './campaigns'; import { seedCards } from './cards'; async function main() { const context: SeedContext = { users: {}, companies: {}, campaigns: {}, cards: {}, catalogs: {} }; try { console.log('
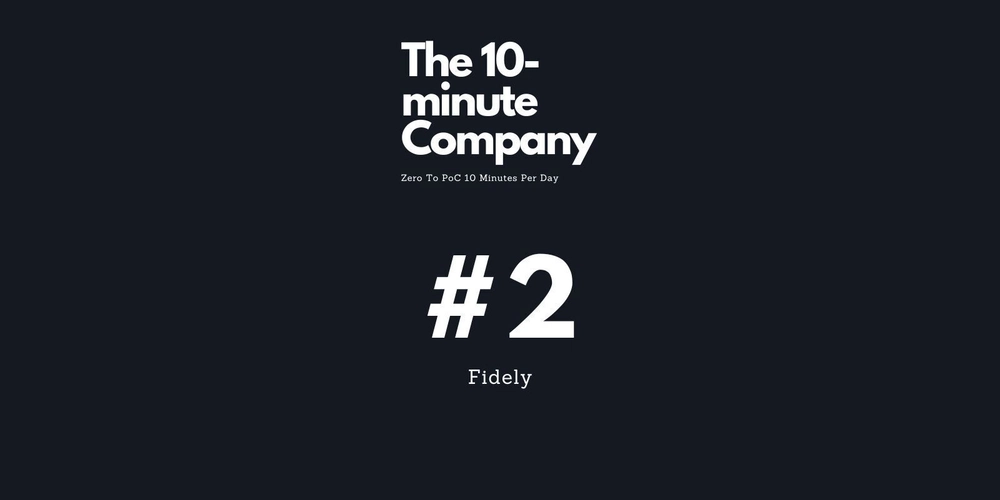
Hello everyone,
It’s been a while since my last update in The 10-Minute Company series. I originally thought I’d be able to share progress sooner, but the holiday season (and a few unexpected projects) absorbed much of my free time. Nevertheless, I made some good progress—both on Fidely and on a smaller side project that popped up in early January.
A Quick Detour: Building a Landing Page with Next.js
During the first two weeks of January, I helped a friend who offers online consultations by building a simple landing page for her services. The project was straightforward but fun, and it gave me a chance to work with:
Next.js for the frontend,
Brevo (formerly Sendinblue) for handling newsletter subscriptions and automated emails,
Brevo Meeting for scheduling online consultations,
Clerk.com for authentication and an admin dashboard,
Stripe for handling payments and checkout.
One of the most interesting parts of the project was setting up an automated workflow: when someone subscribes to the newsletter and completes the double opt-in, they receive a welcome coupon code via email. This ensures only verified users get access to the special offer, adding an extra layer of engagement and trust.
Now, back to Fidely.
Returning to Fidely: Cleaning Up the Backend
With the side project wrapped up, I finally got back to working on Fidely. The focus of the last few days has been restructuring the backend to improve maintainability and scalability. Here’s what I worked on:
1. Refactoring API Routes
I split API endpoints more logically, ensuring that different resources and functionalities were correctly separated. This cleanup makes the backend easier to navigate and will help with future expansions.
Here’s a quick look at the updated routing system:
import { Router } from "express";
import authenticate from "../../middlewares/authenticate";
import authRoutes from "./auth";
import companiesRoutes from "./companies";
import campaignsRoutes from "./campaigns";
import cardCampaignsRoutes from "./card-campaigns";
import cardsRoutes from "./cards";
import transactionsRoutes from "./transactions";
import campaignBuildersRoutes from "./campaign-builders";
const v1Routes = Router();
// Public routes (no auth required)
v1Routes.use("/auth", authRoutes);
// Protected routes
v1Routes.use("/companies", authenticate, companiesRoutes);
v1Routes.use("/campaigns", authenticate, campaignsRoutes);
v1Routes.use("/card-campaigns", authenticate, cardCampaignsRoutes);
v1Routes.use("/cards", authenticate, cardsRoutes);
v1Routes.use("/transactions", authenticate, transactionsRoutes);
v1Routes.use("/campaign-builders", authenticate, campaignBuildersRoutes);
export default v1Routes;
2. Creating a Simple Seeding System
Manually setting up test data was becoming a hassle, so I implemented an automated seeding script that:
Clears the database,
Inserts fake data for testing and demo purposes.
Here’s the updated seed system:
import { SeedContext } from './types';
import { seedUsers } from './users';
import { seedCompanies } from './companies';
import { seedCampaigns } from './campaigns';
import { seedCards } from './cards';
async function main() {
const context: SeedContext = {
users: {},
companies: {},
campaigns: {},
cards: {},
catalogs: {}
};
try {
console.log('