10 Powerful Bash Tricks Every Beginner Should Know
Bash is a must-know tool for developers, but many only use basic commands. Here are 10 powerful Bash tricks to speed up your workflow and make you feel like a terminal ninja. 1. Use !! to Repeat the Last Command Typed a command but forgot sudo? Instead of retyping, just run: sudo !! This reruns the last command with sudo. 2. Use !$ to Reuse the Last Argument Instead of retyping the last argument, use !$: mkdir my_project cd !$ This changes into the newly created directory. 3. Find Large Files Quickly Need to free up space? Find large files with: find . -type f -size +100M This lists all files larger than 100MB in the current directory. 4. Extract Any Compressed File with One Command No need to remember different extract commands. Just use: extract() { if [ -f "$1" ]; then case "$1" in *.tar.bz2) tar xjf "$1" ;; *.tar.gz) tar xzf "$1" ;; *.zip) unzip "$1" ;; *.rar) unrar x "$1" ;; *.7z) 7z x "$1" ;; *) echo "Unknown file format" ;; esac else echo "'$1' is not a valid file" fi } Save this in your .bashrc or .bash_profile and run extract filename.zip. 5. Monitor Real-Time File Changes To see live changes in a file, use: tail -f filename.log Great for monitoring logs or debugging. 6. Rename Multiple Files at Once Easily rename .txt files to .md using: for file in *.txt; do mv "$file" "${file%.txt}.md"; done This renames all .txt files to .md. 7. Kill a Process by Name Instead of finding and killing a process manually, run: pkill -f process_name This kills any process matching process_name. 8. Copy Files Over SSH Like a Pro Instead of scp every time, mount a remote folder using SSH: sshfs user@remote:/path/to/folder /local/mount Now, you can access remote files like they’re local! 9. Create a Quick and Simple HTTP Server Serve the current directory over HTTP with: python3 -m http.server 8000 Now, open http://localhost:8000 in your browser. 10. Make Your cd Command Smarter Tired of typing long paths? Add this to .bashrc: shopt -s autocd Now, typing a directory name alone will cd into it. Final Thoughts These Bash tricks will speed up your workflow and help you work like a pro. Got your own favorite Bash trick? Share it in the comments!
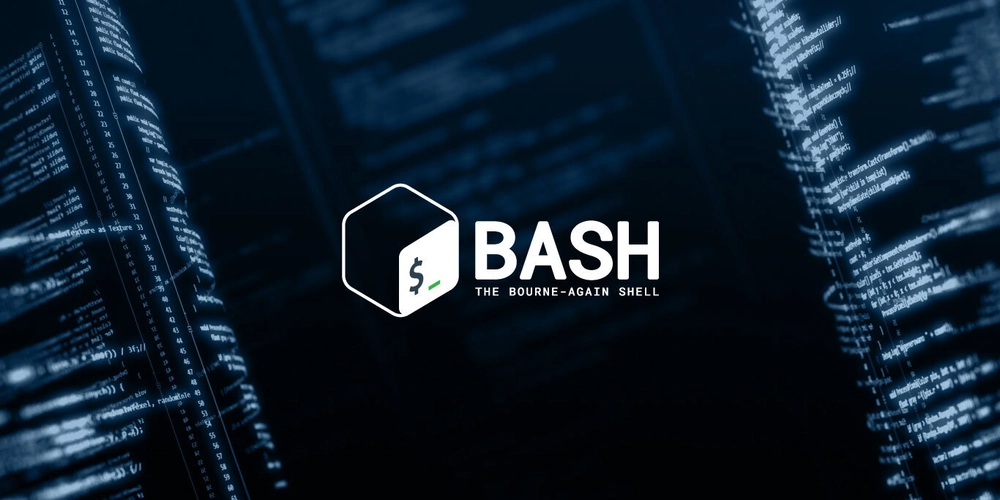
Bash is a must-know tool for developers, but many only use basic commands. Here are 10 powerful Bash tricks to speed up your workflow and make you feel like a terminal ninja.
1. Use !!
to Repeat the Last Command
Typed a command but forgot sudo
? Instead of retyping, just run:
sudo !!
This reruns the last command with sudo
.
2. Use !$
to Reuse the Last Argument
Instead of retyping the last argument, use !$
:
mkdir my_project
cd !$
This changes into the newly created directory.
3. Find Large Files Quickly
Need to free up space? Find large files with:
find . -type f -size +100M
This lists all files larger than 100MB in the current directory.
4. Extract Any Compressed File with One Command
No need to remember different extract commands. Just use:
extract() {
if [ -f "$1" ]; then
case "$1" in
*.tar.bz2) tar xjf "$1" ;;
*.tar.gz) tar xzf "$1" ;;
*.zip) unzip "$1" ;;
*.rar) unrar x "$1" ;;
*.7z) 7z x "$1" ;;
*) echo "Unknown file format" ;;
esac
else
echo "'$1' is not a valid file"
fi
}
Save this in your .bashrc
or .bash_profile
and run extract filename.zip
.
5. Monitor Real-Time File Changes
To see live changes in a file, use:
tail -f filename.log
Great for monitoring logs or debugging.
6. Rename Multiple Files at Once
Easily rename .txt
files to .md
using:
for file in *.txt; do mv "$file" "${file%.txt}.md"; done
This renames all .txt
files to .md
.
7. Kill a Process by Name
Instead of finding and killing a process manually, run:
pkill -f process_name
This kills any process matching process_name
.
8. Copy Files Over SSH Like a Pro
Instead of scp
every time, mount a remote folder using SSH:
sshfs user@remote:/path/to/folder /local/mount
Now, you can access remote files like they’re local!
9. Create a Quick and Simple HTTP Server
Serve the current directory over HTTP with:
python3 -m http.server 8000
Now, open http://localhost:8000
in your browser.
10. Make Your cd
Command Smarter
Tired of typing long paths? Add this to .bashrc
:
shopt -s autocd
Now, typing a directory name alone will cd
into it.
Final Thoughts
These Bash tricks will speed up your workflow and help you work like a pro. Got your own favorite Bash trick? Share it in the comments!