Working with Time in Golang: Essential Utilities
The Go time package provides robust tools for handling timestamps, timezones, and date manipulations. However, certain common utilities like adding days, finding the start or end of a day, and checking for leap years aren't available as direct functions. In this blog, we’ll explore useful time-related functions in Go, ensuring accuracy with proper use of the time package. Additionally, for working with Unix timestamps, you may find this epoch time converter helpful. Adding or Subtracting Days (with Daylight Savings Adjustments) Go provides Add() for adding durations and AddDate() for adding date components (years, months, and days). The latter is preferred for handling date shifts correctly, especially with daylight savings adjustments. package main import ( "fmt" "time" ) // AddDays adds or subtracts days from a given timestamp. func AddDays(t time.Time, days int) time.Time { fmt.Println("Current Time:", t) newTime := t.AddDate(0, 0, days) fmt.Println("New Time:", newTime) return newTime } func main() { now := time.Now() AddDays(now, 2) // Add 2 days AddDays(now, -2) // Subtract 2 days } /* Sample Output: Current Time: 2025-03-27 14:00:00 +0000 UTC New Time: 2025-03-29 14:00:00 +0000 UTC Current Time: 2025-03-27 14:00:00 +0000 UTC New Time: 2025-03-25 14:00:00 +0000 UTC */ Getting the Start of the Day To find when a given day begins, extract the year, month, and day, then reset hours, minutes, and seconds. // StartOfDay returns the start of a given day in a specific timezone. func StartOfDay(t time.Time, timezone string) (time.Time, error) { location, err := time.LoadLocation(timezone) if err != nil { return time.Time{}, err // Handle invalid timezone } year, month, day := t.In(location).Date() dayStartTime := time.Date(year, month, day, 0, 0, 0, 0, location) return dayStartTime, nil } func main() { now := time.Now() start, err := StartOfDay(now, "Asia/Kolkata") if err != nil { fmt.Println("Error:", err) return } fmt.Println("Start of the Day:", start) } Getting the End of the Day Similar to the start of the day, setting the time to 23:59:59 gives the last moment of the day. // EndOfDay returns the end of a given day in a specific timezone. func EndOfDay(t time.Time, timezone string) (time.Time, error) { location, err := time.LoadLocation(timezone) if err != nil { return time.Time{}, err } year, month, day := t.In(location).Date() dayEndTime := time.Date(year, month, day, 23, 59, 59, 0, location) return dayEndTime, nil } Checking if Two Dates Are the Same Day Comparing the day of the year and the year allows you to check if two timestamps fall on the same day. // IsSameDay checks if two timestamps belong to the same calendar day. func IsSameDay(first, second time.Time) bool { return first.YearDay() == second.YearDay() && first.Year() == second.Year() } func main() { t1 := time.Date(2025, 3, 27, 10, 0, 0, 0, time.UTC) t2 := time.Date(2025, 3, 27, 22, 0, 0, 0, time.UTC) fmt.Println("Same day:", IsSameDay(t1, t2)) // Output: true } Calculating the Difference Between Two Dates in Days The Sub() function calculates the duration between two timestamps. To get the number of days, divide by 24 hours. // DiffInDays calculates the number of whole days between two timestamps. func DiffInDays(start, end time.Time) int { return int(end.Sub(start).Hours() / 24) } Counting Specific Weekdays Between Two Dates To find the number of Mondays (or any day) between two dates: // FindNoOfDays counts occurrences of a specific weekday between two dates. func FindNoOfDays(day time.Weekday, start, end time.Time) int { count := 0 for start.Before(end) || start.Equal(end) { if start.Weekday() == day { count++ } start = start.AddDate(0, 0, 1) } return count } Checking if a Year is a Leap Year Leap years occur every 4 years, except for years divisible by 100 unless also divisible by 400. // IsLeapYear checks if a given year is a leap year. func IsLeapYear(year int) bool { return (year%4 == 0 && year%100 != 0) || (year%400 == 0) } func main() { fmt.Println("2024 is leap year:", IsLeapYear(2024)) // Output: true fmt.Println("2025 is leap year:", IsLeapYear(2025)) // Output: false } Conclusion These functions fill some of the gaps in Go's time package while ensuring accuracy. Whether you’re adding days, comparing timestamps, or checking for leap years, these utilities can save you time and effort. Also, if you need to work with Unix timestamps, you can use this epoch time converter to quickly convert between human-readable dates and epoch time. Have any suggestions or improvements? Share your thoughts in the comments!
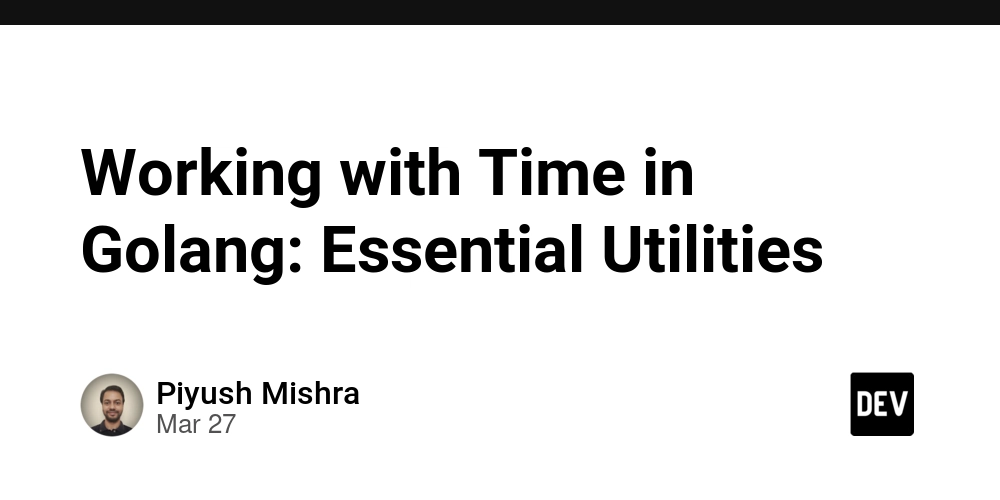
The Go time
package provides robust tools for handling timestamps, timezones, and date manipulations. However, certain common utilities like adding days, finding the start or end of a day, and checking for leap years aren't available as direct functions.
In this blog, we’ll explore useful time-related functions in Go, ensuring accuracy with proper use of the time
package. Additionally, for working with Unix timestamps, you may find this epoch time converter helpful.
Adding or Subtracting Days (with Daylight Savings Adjustments)
Go provides Add()
for adding durations and AddDate()
for adding date components (years, months, and days). The latter is preferred for handling date shifts correctly, especially with daylight savings adjustments.
package main
import (
"fmt"
"time"
)
// AddDays adds or subtracts days from a given timestamp.
func AddDays(t time.Time, days int) time.Time {
fmt.Println("Current Time:", t)
newTime := t.AddDate(0, 0, days)
fmt.Println("New Time:", newTime)
return newTime
}
func main() {
now := time.Now()
AddDays(now, 2) // Add 2 days
AddDays(now, -2) // Subtract 2 days
}
/*
Sample Output:
Current Time: 2025-03-27 14:00:00 +0000 UTC
New Time: 2025-03-29 14:00:00 +0000 UTC
Current Time: 2025-03-27 14:00:00 +0000 UTC
New Time: 2025-03-25 14:00:00 +0000 UTC
*/
Getting the Start of the Day
To find when a given day begins, extract the year, month, and day, then reset hours, minutes, and seconds.
// StartOfDay returns the start of a given day in a specific timezone.
func StartOfDay(t time.Time, timezone string) (time.Time, error) {
location, err := time.LoadLocation(timezone)
if err != nil {
return time.Time{}, err // Handle invalid timezone
}
year, month, day := t.In(location).Date()
dayStartTime := time.Date(year, month, day, 0, 0, 0, 0, location)
return dayStartTime, nil
}
func main() {
now := time.Now()
start, err := StartOfDay(now, "Asia/Kolkata")
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println("Start of the Day:", start)
}
Getting the End of the Day
Similar to the start of the day, setting the time to 23:59:59 gives the last moment of the day.
// EndOfDay returns the end of a given day in a specific timezone.
func EndOfDay(t time.Time, timezone string) (time.Time, error) {
location, err := time.LoadLocation(timezone)
if err != nil {
return time.Time{}, err
}
year, month, day := t.In(location).Date()
dayEndTime := time.Date(year, month, day, 23, 59, 59, 0, location)
return dayEndTime, nil
}
Checking if Two Dates Are the Same Day
Comparing the day of the year and the year allows you to check if two timestamps fall on the same day.
// IsSameDay checks if two timestamps belong to the same calendar day.
func IsSameDay(first, second time.Time) bool {
return first.YearDay() == second.YearDay() && first.Year() == second.Year()
}
func main() {
t1 := time.Date(2025, 3, 27, 10, 0, 0, 0, time.UTC)
t2 := time.Date(2025, 3, 27, 22, 0, 0, 0, time.UTC)
fmt.Println("Same day:", IsSameDay(t1, t2)) // Output: true
}
Calculating the Difference Between Two Dates in Days
The Sub()
function calculates the duration between two timestamps. To get the number of days, divide by 24 hours.
// DiffInDays calculates the number of whole days between two timestamps.
func DiffInDays(start, end time.Time) int {
return int(end.Sub(start).Hours() / 24)
}
Counting Specific Weekdays Between Two Dates
To find the number of Mondays (or any day) between two dates:
// FindNoOfDays counts occurrences of a specific weekday between two dates.
func FindNoOfDays(day time.Weekday, start, end time.Time) int {
count := 0
for start.Before(end) || start.Equal(end) {
if start.Weekday() == day {
count++
}
start = start.AddDate(0, 0, 1)
}
return count
}
Checking if a Year is a Leap Year
Leap years occur every 4 years, except for years divisible by 100 unless also divisible by 400.
// IsLeapYear checks if a given year is a leap year.
func IsLeapYear(year int) bool {
return (year%4 == 0 && year%100 != 0) || (year%400 == 0)
}
func main() {
fmt.Println("2024 is leap year:", IsLeapYear(2024)) // Output: true
fmt.Println("2025 is leap year:", IsLeapYear(2025)) // Output: false
}
Conclusion
These functions fill some of the gaps in Go's time
package while ensuring accuracy. Whether you’re adding days, comparing timestamps, or checking for leap years, these utilities can save you time and effort.
Also, if you need to work with Unix timestamps, you can use this epoch time converter to quickly convert between human-readable dates and epoch time.
Have any suggestions or improvements? Share your thoughts in the comments!