Why SimpleDateFormat throws java.lang.NumberFormatException
Introduction Recently, while fixing a DEFECT at work, I encountered a perplexing issue in the PREF environment. This defect never appeared during normal operations but consistently surfaced during load testing. The error message was: Exception in thread : java.lang.NumberFormatException: For input string: "" Upon inspecting the stack trace, it turned out that the exception was thrown during a conversion of a String to Timestamp. The issue seemed to stem from an empty string ("") in the input, causing the conversion to fail. However, the strange part was that the code already used StringUtils.isNotBlank() to filter out empty or null strings. Logically, an empty string should never reach the sdf.parse() method. So, what was really going on? The Mystery Deepens Even more strangely, every time the load test ran, the method call stack leading to the exception was different. However, the ultimate error always pointed to the date parsing (**parse**) method. I first suspected that StringUtils.isNotBlank() might not be filtering out "" correctly, but after verifying its behavior, it became evident that the method was indeed strict and working as expected. Then, I considered whether an incorrect date format might be causing the issue. But that still didn’t explain why the stack trace varied every time the error occurred or why it only happened under heavy load. The Root Cause: SimpleDateFormat is Not Thread-safe While investigating the code, I noticed that the project used a static instance of SimpleDateFormat to avoid frequent object instantiation. Here’s an example: import java.text.SimpleDateFormat; import java.text.ParseException; import java.util.Date; public class SimpleDateFormatTest { private static final SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); public static String formatDate(Date date) throws ParseException { return sdf.format(date); } public static Date parse(String strDate) throws ParseException { return sdf.parse(strDate); } public static void main(String[] args) throws ParseException { System.out.println(sdf.format(new Date())); } } While this code might work fine in single-threaded scenarios, it fails horribly under multi-threading, as shown in the following example: import java.text.DateFormat; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class TestSimpleDateFormat { private static final DateFormat DATE_FORMAT = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); public static Date parse(String date) throws ParseException { return DATE_FORMAT.parse(date); } public static void main(String[] args) { ExecutorService executorService = Executors.newFixedThreadPool(500); for (int i = 0; i { for (int j = 0; j > 8; int count = compiledPattern[i++] & 0xff; if (count == 255) { count = compiledPattern[i++]
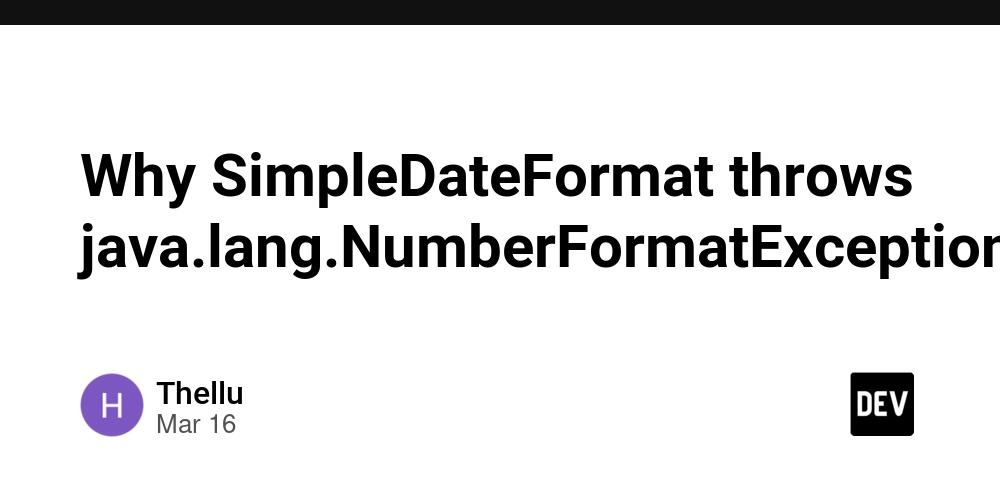
Introduction
Recently, while fixing a DEFECT at work, I encountered a perplexing issue in the PREF environment. This defect never appeared during normal operations but consistently surfaced during load testing. The error message was:
Exception in thread : java.lang.NumberFormatException: For input string: ""
Upon inspecting the stack trace, it turned out that the exception was thrown during a conversion of a String to Timestamp. The issue seemed to stem from an empty string (""
) in the input, causing the conversion to fail.
However, the strange part was that the code already used StringUtils.isNotBlank()
to filter out empty or null strings. Logically, an empty string should never reach the sdf.parse()
method. So, what was really going on?
The Mystery Deepens
Even more strangely, every time the load test ran, the method call stack leading to the exception was different. However, the ultimate error always pointed to the date parsing (**parse**
) method.
I first suspected that StringUtils.isNotBlank()
might not be filtering out ""
correctly, but after verifying its behavior, it became evident that the method was indeed strict and working as expected.
Then, I considered whether an incorrect date format might be causing the issue. But that still didn’t explain why the stack trace varied every time the error occurred or why it only happened under heavy load.
The Root Cause: SimpleDateFormat is Not Thread-safe
While investigating the code, I noticed that the project used a static instance of SimpleDateFormat to avoid frequent object instantiation. Here’s an example:
import java.text.SimpleDateFormat;
import java.text.ParseException;
import java.util.Date;
public class SimpleDateFormatTest {
private static final SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
public static String formatDate(Date date) throws ParseException {
return sdf.format(date);
}
public static Date parse(String strDate) throws ParseException {
return sdf.parse(strDate);
}
public static void main(String[] args) throws ParseException {
System.out.println(sdf.format(new Date()));
}
}
While this code might work fine in single-threaded scenarios, it fails horribly under multi-threading, as shown in the following example:
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class TestSimpleDateFormat {
private static final DateFormat DATE_FORMAT = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
public static Date parse(String date) throws ParseException {
return DATE_FORMAT.parse(date);
}
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(500);
for (int i = 0; i < 500; i++) {
executorService.execute(() -> {
for (int j = 0; j < 1000000; j++) {
try {
DATE_FORMAT.parse("2025-01-01 00:00:00");
} catch (ParseException e) {
e.printStackTrace();
}
}
});
}
executorService.shutdown();
}
}
What Happens Under Load?
During load testing, multiple threads attempt to use the same instance of SimpleDateFormat
, leading to race conditions. This results in:
-
Corrupted date parsing leading to
NumberFormatException
- Incorrect timestamps due to shared state modifications
- Application crashes due to unpredictable behavior
Understanding the Issue via Source Code
If we look at the SimpleDateFormat.format()
method in the JDK source code:
@Override
public StringBuffer format(Date date, StringBuffer toAppendTo, FieldPosition pos) {
pos.beginIndex = pos.endIndex = 0;
return format(date, toAppendTo, pos.getFieldDelegate());
}
private StringBuffer format(Date date, StringBuffer toAppendTo, FieldDelegate delegate) {
calendar.setTime(date);
boolean useDateFormatSymbols = useDateFormatSymbols();
for (int i = 0; i < compiledPattern.length; ) {
int tag = compiledPattern[i] >>> 8;
int count = compiledPattern[i++] & 0xff;
if (count == 255) {
count = compiledPattern[i++] << 16;
count |= compiledPattern[i++];
}
switch (tag) {
case TAG_QUOTE_ASCII_CHAR:
toAppendTo.append((char)count);
break;
case TAG_QUOTE_CHARS:
toAppendTo.append(compiledPattern, i, count);
i += count;
break;
default:
subFormat(tag, count, delegate, toAppendTo, useDateFormatSymbols);
break;
}
}
return toAppendTo;
}
Notice the line:
calendar.setTime(date);
Since we declared SimpleDateFormat as a static variable, its internal Calendar instance is shared across multiple threads, leading to inconsistent state modifications and thread interference.
For example:
-
Thread A sets the time to
2019-01-02
and gets paused. -
Thread B changes the time to
2019-01-03
and gets paused. - Thread A resumes, but the calendar state has changed, leading to incorrect formatting and potential exceptions.
The Solution
There are multiple ways to fix this issue:
- Create a New Instance Per Use
✅ No thread safety issues, ❌ but increases object creation overhead.
public static Date parse(String date) throws ParseException {
return new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").parse(date);
}
- Use ThreadLocal to Store a Separate Instance for Each Thread
✅ Avoids excessive object creation, ✅ Ensures thread safety.
private static final ThreadLocal<DateFormat> DATE_FORMAT = ThreadLocal.withInitial(() -> new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"));
public static Date parse(String date) throws ParseException {
return DATE_FORMAT.get().parse(date);
}
- Use DateTimeFormatter (Recommended)
Java 8 introduced DateTimeFormatter
, which is immutable and thread-safe:
✅ Best practice, ✅ Thread-safe, ✅ More efficient.
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateTimeFormatterExample {
private static final DateTimeFormatter FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
public static void main(String[] args) {
String formattedDate = LocalDateTime.now().format(FORMATTER);
System.out.println(formattedDate);
}
}
Conclusion
Using SimpleDateFormat
as a static shared instance in multi-threaded applications is a disaster waiting to happen. Instead, consider using ThreadLocal or DateTimeFormatter to ensure thread safety and reliable date parsing in Java applications.
Reference
For further reading, check out this article: CSDN - SimpleDateFormat Thread Safety Issue