What is Rate Limiting and How to Add It in Django
Have you ever seen an error like “429 Too Many Requests”? That’s because of rate limiting. In this post, we’ll learn: What is rate limiting Why it’s important How to add rate limiting in a Django project (with simple steps) What is Rate Limiting? Rate limiting means putting a limit on how many times someone can call your API or access a resource in a specific time. Example: “You can only make 5 requests per minute.” It helps keep your website or API safe and fair for everyone. Why is Rate Limiting Important? Stop Abuse Stops bots or attackers from spamming your website. Save Server Resources Avoids server overload and crashes. Fair Usage Makes sure no one uses more than their share. Better Performance Keeps your app fast and smooth. How to Implement Rate Limiting in Django We can use a third-party package called django-ratelimit Step 1: Install the Package pip install django-ratelimit Step 2: Add to Your Django View from django.http import JsonResponse from ratelimit.decorators import ratelimit @ratelimit(key='ip', rate='5/m', block=True) def my_api_view(request): return JsonResponse({"message": "This is a rate-limited API!"}) What this does: key='ip' → limit by user’s IP address rate='5/m' → max 5 requests per minute block=True → block extra requests with 429 error Now, Try hitting the API more than 5 times per minute from the same IP. You will get { "detail": "Request was throttled. Expected available in 60 seconds." } Rate limiting is simple but powerful. It keeps your API healthy and safe. Let me know if you tried this in your Django project or if you want help with a specific use case!
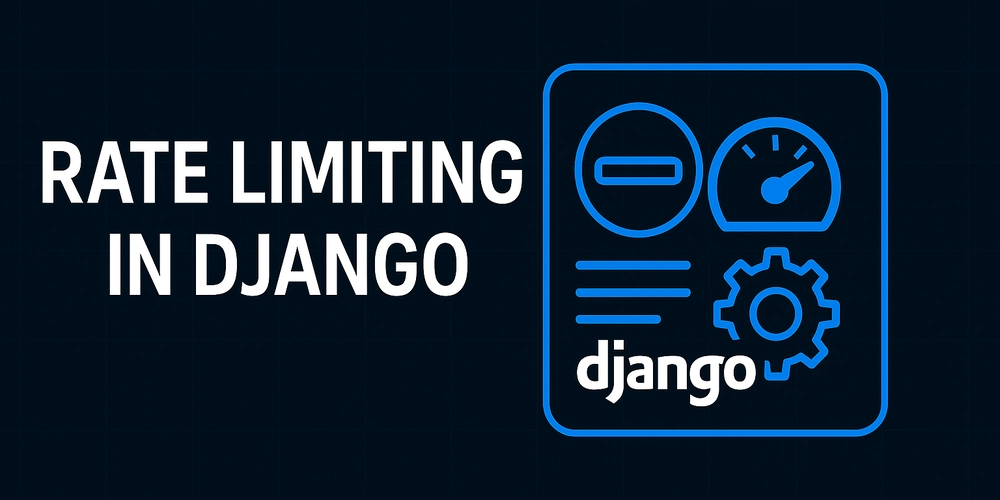
Have you ever seen an error like “429 Too Many Requests”? That’s because of rate limiting. In this post, we’ll learn:
- What is rate limiting
- Why it’s important
- How to add rate limiting in a Django project (with simple steps)
What is Rate Limiting?
Rate limiting means putting a limit on how many times someone can call your API or access a resource in a specific time.
Example:
“You can only make 5 requests per minute.”
It helps keep your website or API safe and fair for everyone.
Why is Rate Limiting Important?
- Stop Abuse
Stops bots or attackers from spamming your website.
- Save Server Resources
Avoids server overload and crashes.
- Fair Usage
Makes sure no one uses more than their share.
- Better Performance
Keeps your app fast and smooth.
How to Implement Rate Limiting in Django
We can use a third-party package called django-ratelimit
Step 1: Install the Package
pip install django-ratelimit
Step 2: Add to Your Django View
from django.http import JsonResponse
from ratelimit.decorators import ratelimit
@ratelimit(key='ip', rate='5/m', block=True)
def my_api_view(request):
return JsonResponse({"message": "This is a rate-limited API!"})
What this does:
- key='ip' → limit by user’s IP address
- rate='5/m' → max 5 requests per minute
- block=True → block extra requests with 429 error
Now, Try hitting the API more than 5 times per minute from the same IP.
You will get
{
"detail": "Request was throttled. Expected available in 60 seconds."
}
Rate limiting is simple but powerful. It keeps your API healthy and safe.
Let me know if you tried this in your Django project or if you want help with a specific use case!